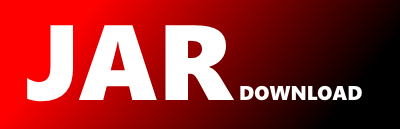
com.infobip.model.ViberPlatform Maven / Gradle / Ivy
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ [email protected].
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Objects;
/**
* Platform options. For more details, see [documentation](https://www.infobip.com/docs/cpaas-x/application-and-entity-management).
*/
public class ViberPlatform {
private String entityId;
private String applicationId;
/**
* Sets entityId.
*
* Field description:
* Required to use entity in a send request for outbound traffic. Returned in notification events. For more details, see [documentation](https://www.infobip.com/docs/cpaas-x/application-and-entity-management).
*
* @param entityId
* @return This {@link ViberPlatform instance}.
*/
public ViberPlatform entityId(String entityId) {
this.entityId = entityId;
return this;
}
/**
* Returns entityId.
*
* Field description:
* Required to use entity in a send request for outbound traffic. Returned in notification events. For more details, see [documentation](https://www.infobip.com/docs/cpaas-x/application-and-entity-management).
*
* @return entityId
*/
@JsonProperty("entityId")
public String getEntityId() {
return entityId;
}
/**
* Sets entityId.
*
* Field description:
* Required to use entity in a send request for outbound traffic. Returned in notification events. For more details, see [documentation](https://www.infobip.com/docs/cpaas-x/application-and-entity-management).
*
* @param entityId
*/
@JsonProperty("entityId")
public void setEntityId(String entityId) {
this.entityId = entityId;
}
/**
* Sets applicationId.
*
* Field description:
* Required for application use in a send request for outbound traffic. Returned in notification events. For more details, see [documentation](https://www.infobip.com/docs/cpaas-x/application-and-entity-management).
*
* @param applicationId
* @return This {@link ViberPlatform instance}.
*/
public ViberPlatform applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* Returns applicationId.
*
* Field description:
* Required for application use in a send request for outbound traffic. Returned in notification events. For more details, see [documentation](https://www.infobip.com/docs/cpaas-x/application-and-entity-management).
*
* @return applicationId
*/
@JsonProperty("applicationId")
public String getApplicationId() {
return applicationId;
}
/**
* Sets applicationId.
*
* Field description:
* Required for application use in a send request for outbound traffic. Returned in notification events. For more details, see [documentation](https://www.infobip.com/docs/cpaas-x/application-and-entity-management).
*
* @param applicationId
*/
@JsonProperty("applicationId")
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ViberPlatform viberPlatform = (ViberPlatform) o;
return Objects.equals(this.entityId, viberPlatform.entityId)
&& Objects.equals(this.applicationId, viberPlatform.applicationId);
}
@Override
public int hashCode() {
return Objects.hash(entityId, applicationId);
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class ViberPlatform {")
.append(newLine)
.append(" entityId: ")
.append(toIndentedString(entityId))
.append(newLine)
.append(" applicationId: ")
.append(toIndentedString(applicationId))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}