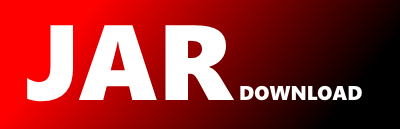
com.infobip.model.WhatsAppResultWhatsAppInboundContent Maven / Gradle / Ivy
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ [email protected].
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
import java.util.Objects;
/**
* Collection of reports, one per every received message.
*/
public class WhatsAppResultWhatsAppInboundContent {
private String from;
private String to;
private String integrationType;
private OffsetDateTime receivedAt;
private String messageId;
private String pairedMessageId;
private String callbackData;
private WhatsAppWebhookInboundContent message;
private MessagePrice price;
/**
* Sets from.
*
* Field description:
* Number which sent the message.
*
* @param from
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent from(String from) {
this.from = from;
return this;
}
/**
* Returns from.
*
* Field description:
* Number which sent the message.
*
* @return from
*/
@JsonProperty("from")
public String getFrom() {
return from;
}
/**
* Sets from.
*
* Field description:
* Number which sent the message.
*
* @param from
*/
@JsonProperty("from")
public void setFrom(String from) {
this.from = from;
}
/**
* Sets to.
*
* Field description:
* Sender provided during the activation process.
*
* @param to
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent to(String to) {
this.to = to;
return this;
}
/**
* Returns to.
*
* Field description:
* Sender provided during the activation process.
*
* @return to
*/
@JsonProperty("to")
public String getTo() {
return to;
}
/**
* Sets to.
*
* Field description:
* Sender provided during the activation process.
*
* @param to
*/
@JsonProperty("to")
public void setTo(String to) {
this.to = to;
}
/**
* Sets integrationType.
*
* Field description:
* Integration type.
*
* @param integrationType
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent integrationType(String integrationType) {
this.integrationType = integrationType;
return this;
}
/**
* Returns integrationType.
*
* Field description:
* Integration type.
*
* @return integrationType
*/
@JsonProperty("integrationType")
public String getIntegrationType() {
return integrationType;
}
/**
* Sets integrationType.
*
* Field description:
* Integration type.
*
* @param integrationType
*/
@JsonProperty("integrationType")
public void setIntegrationType(String integrationType) {
this.integrationType = integrationType;
}
/**
* Sets receivedAt.
*
* Field description:
* Date and time when Infobip received the message.
*
* @param receivedAt
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent receivedAt(OffsetDateTime receivedAt) {
this.receivedAt = receivedAt;
return this;
}
/**
* Returns receivedAt.
*
* Field description:
* Date and time when Infobip received the message.
*
* @return receivedAt
*/
@JsonProperty("receivedAt")
public OffsetDateTime getReceivedAt() {
return receivedAt;
}
/**
* Sets receivedAt.
*
* Field description:
* Date and time when Infobip received the message.
*
* @param receivedAt
*/
@JsonProperty("receivedAt")
public void setReceivedAt(OffsetDateTime receivedAt) {
this.receivedAt = receivedAt;
}
/**
* Sets messageId.
*
* Field description:
* The ID that uniquely identifies the received message.
*
* @param messageId
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Returns messageId.
*
* Field description:
* The ID that uniquely identifies the received message.
*
* @return messageId
*/
@JsonProperty("messageId")
public String getMessageId() {
return messageId;
}
/**
* Sets messageId.
*
* Field description:
* The ID that uniquely identifies the received message.
*
* @param messageId
*/
@JsonProperty("messageId")
public void setMessageId(String messageId) {
this.messageId = messageId;
}
/**
* Sets pairedMessageId.
*
* Field description:
* Message ID of paired outgoing message if matched by Infobip platform.
*
* @param pairedMessageId
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent pairedMessageId(String pairedMessageId) {
this.pairedMessageId = pairedMessageId;
return this;
}
/**
* Returns pairedMessageId.
*
* Field description:
* Message ID of paired outgoing message if matched by Infobip platform.
*
* @return pairedMessageId
*/
@JsonProperty("pairedMessageId")
public String getPairedMessageId() {
return pairedMessageId;
}
/**
* Sets pairedMessageId.
*
* Field description:
* Message ID of paired outgoing message if matched by Infobip platform.
*
* @param pairedMessageId
*/
@JsonProperty("pairedMessageId")
public void setPairedMessageId(String pairedMessageId) {
this.pairedMessageId = pairedMessageId;
}
/**
* Sets callbackData.
*
* Field description:
* Callback data sent through ‛callbackData‛ field when sending message.
*
* @param callbackData
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent callbackData(String callbackData) {
this.callbackData = callbackData;
return this;
}
/**
* Returns callbackData.
*
* Field description:
* Callback data sent through ‛callbackData‛ field when sending message.
*
* @return callbackData
*/
@JsonProperty("callbackData")
public String getCallbackData() {
return callbackData;
}
/**
* Sets callbackData.
*
* Field description:
* Callback data sent through ‛callbackData‛ field when sending message.
*
* @param callbackData
*/
@JsonProperty("callbackData")
public void setCallbackData(String callbackData) {
this.callbackData = callbackData;
}
/**
* Sets message.
*
* @param message
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent message(WhatsAppWebhookInboundContent message) {
this.message = message;
return this;
}
/**
* Returns message.
*
* @return message
*/
@JsonProperty("message")
public WhatsAppWebhookInboundContent getMessage() {
return message;
}
/**
* Sets message.
*
* @param message
*/
@JsonProperty("message")
public void setMessage(WhatsAppWebhookInboundContent message) {
this.message = message;
}
/**
* Sets price.
*
* @param price
* @return This {@link WhatsAppResultWhatsAppInboundContent instance}.
*/
public WhatsAppResultWhatsAppInboundContent price(MessagePrice price) {
this.price = price;
return this;
}
/**
* Returns price.
*
* @return price
*/
@JsonProperty("price")
public MessagePrice getPrice() {
return price;
}
/**
* Sets price.
*
* @param price
*/
@JsonProperty("price")
public void setPrice(MessagePrice price) {
this.price = price;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
WhatsAppResultWhatsAppInboundContent whatsAppResultWhatsAppInboundContent =
(WhatsAppResultWhatsAppInboundContent) o;
return Objects.equals(this.from, whatsAppResultWhatsAppInboundContent.from)
&& Objects.equals(this.to, whatsAppResultWhatsAppInboundContent.to)
&& Objects.equals(this.integrationType, whatsAppResultWhatsAppInboundContent.integrationType)
&& Objects.equals(this.receivedAt, whatsAppResultWhatsAppInboundContent.receivedAt)
&& Objects.equals(this.messageId, whatsAppResultWhatsAppInboundContent.messageId)
&& Objects.equals(this.pairedMessageId, whatsAppResultWhatsAppInboundContent.pairedMessageId)
&& Objects.equals(this.callbackData, whatsAppResultWhatsAppInboundContent.callbackData)
&& Objects.equals(this.message, whatsAppResultWhatsAppInboundContent.message)
&& Objects.equals(this.price, whatsAppResultWhatsAppInboundContent.price);
}
@Override
public int hashCode() {
return Objects.hash(
from, to, integrationType, receivedAt, messageId, pairedMessageId, callbackData, message, price);
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class WhatsAppResultWhatsAppInboundContent {")
.append(newLine)
.append(" from: ")
.append(toIndentedString(from))
.append(newLine)
.append(" to: ")
.append(toIndentedString(to))
.append(newLine)
.append(" integrationType: ")
.append(toIndentedString(integrationType))
.append(newLine)
.append(" receivedAt: ")
.append(toIndentedString(receivedAt))
.append(newLine)
.append(" messageId: ")
.append(toIndentedString(messageId))
.append(newLine)
.append(" pairedMessageId: ")
.append(toIndentedString(pairedMessageId))
.append(newLine)
.append(" callbackData: ")
.append(toIndentedString(callbackData))
.append(newLine)
.append(" message: ")
.append(toIndentedString(message))
.append(newLine)
.append(" price: ")
.append(toIndentedString(price))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}