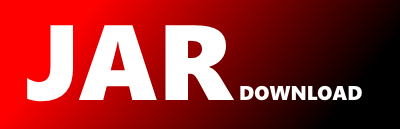
com.infobip.model.WhatsAppContactContent Maven / Gradle / Ivy
Show all versions of infobip-api-java-client Show documentation
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ support@infobip.com.
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* An array of contacts sent in a WhatsApp message.
*/
public class WhatsAppContactContent {
private List addresses = null;
private String birthday;
private List emails = null;
private WhatsAppNameContent name;
private WhatsAppOrganizationContent org;
private List phones = null;
private List urls = null;
/**
* Sets addresses.
*
* Field description:
* Array of addresses information.
*
* @param addresses
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent addresses(List addresses) {
this.addresses = addresses;
return this;
}
/**
* Adds and item into addresses.
*
* Field description:
* Array of addresses information.
*
* @param addressesItem The item to be added to the list.
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent addAddressesItem(WhatsAppAddressContent addressesItem) {
if (this.addresses == null) {
this.addresses = new ArrayList<>();
}
this.addresses.add(addressesItem);
return this;
}
/**
* Returns addresses.
*
* Field description:
* Array of addresses information.
*
* @return addresses
*/
@JsonProperty("addresses")
public List getAddresses() {
return addresses;
}
/**
* Sets addresses.
*
* Field description:
* Array of addresses information.
*
* @param addresses
*/
@JsonProperty("addresses")
public void setAddresses(List addresses) {
this.addresses = addresses;
}
/**
* Sets birthday.
*
* Field description:
* Date of birth in `YYYY-MM-DD` format.
*
* @param birthday
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent birthday(String birthday) {
this.birthday = birthday;
return this;
}
/**
* Returns birthday.
*
* Field description:
* Date of birth in `YYYY-MM-DD` format.
*
* @return birthday
*/
@JsonProperty("birthday")
public String getBirthday() {
return birthday;
}
/**
* Sets birthday.
*
* Field description:
* Date of birth in `YYYY-MM-DD` format.
*
* @param birthday
*/
@JsonProperty("birthday")
public void setBirthday(String birthday) {
this.birthday = birthday;
}
/**
* Sets emails.
*
* Field description:
* Array of emails information.
*
* @param emails
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent emails(List emails) {
this.emails = emails;
return this;
}
/**
* Adds and item into emails.
*
* Field description:
* Array of emails information.
*
* @param emailsItem The item to be added to the list.
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent addEmailsItem(WhatsAppEmailContent emailsItem) {
if (this.emails == null) {
this.emails = new ArrayList<>();
}
this.emails.add(emailsItem);
return this;
}
/**
* Returns emails.
*
* Field description:
* Array of emails information.
*
* @return emails
*/
@JsonProperty("emails")
public List getEmails() {
return emails;
}
/**
* Sets emails.
*
* Field description:
* Array of emails information.
*
* @param emails
*/
@JsonProperty("emails")
public void setEmails(List emails) {
this.emails = emails;
}
/**
* Sets name.
*
* The field is required.
*
* @param name
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent name(WhatsAppNameContent name) {
this.name = name;
return this;
}
/**
* Returns name.
*
* The field is required.
*
* @return name
*/
@JsonProperty("name")
public WhatsAppNameContent getName() {
return name;
}
/**
* Sets name.
*
* The field is required.
*
* @param name
*/
@JsonProperty("name")
public void setName(WhatsAppNameContent name) {
this.name = name;
}
/**
* Sets org.
*
* @param org
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent org(WhatsAppOrganizationContent org) {
this.org = org;
return this;
}
/**
* Returns org.
*
* @return org
*/
@JsonProperty("org")
public WhatsAppOrganizationContent getOrg() {
return org;
}
/**
* Sets org.
*
* @param org
*/
@JsonProperty("org")
public void setOrg(WhatsAppOrganizationContent org) {
this.org = org;
}
/**
* Sets phones.
*
* Field description:
* Array of phones information.
*
* @param phones
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent phones(List phones) {
this.phones = phones;
return this;
}
/**
* Adds and item into phones.
*
* Field description:
* Array of phones information.
*
* @param phonesItem The item to be added to the list.
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent addPhonesItem(WhatsAppPhoneContent phonesItem) {
if (this.phones == null) {
this.phones = new ArrayList<>();
}
this.phones.add(phonesItem);
return this;
}
/**
* Returns phones.
*
* Field description:
* Array of phones information.
*
* @return phones
*/
@JsonProperty("phones")
public List getPhones() {
return phones;
}
/**
* Sets phones.
*
* Field description:
* Array of phones information.
*
* @param phones
*/
@JsonProperty("phones")
public void setPhones(List phones) {
this.phones = phones;
}
/**
* Sets urls.
*
* Field description:
* Array of urls information.
*
* @param urls
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent urls(List urls) {
this.urls = urls;
return this;
}
/**
* Adds and item into urls.
*
* Field description:
* Array of urls information.
*
* @param urlsItem The item to be added to the list.
* @return This {@link WhatsAppContactContent instance}.
*/
public WhatsAppContactContent addUrlsItem(WhatsAppUrlContent urlsItem) {
if (this.urls == null) {
this.urls = new ArrayList<>();
}
this.urls.add(urlsItem);
return this;
}
/**
* Returns urls.
*
* Field description:
* Array of urls information.
*
* @return urls
*/
@JsonProperty("urls")
public List getUrls() {
return urls;
}
/**
* Sets urls.
*
* Field description:
* Array of urls information.
*
* @param urls
*/
@JsonProperty("urls")
public void setUrls(List urls) {
this.urls = urls;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
WhatsAppContactContent whatsAppContactContent = (WhatsAppContactContent) o;
return Objects.equals(this.addresses, whatsAppContactContent.addresses)
&& Objects.equals(this.birthday, whatsAppContactContent.birthday)
&& Objects.equals(this.emails, whatsAppContactContent.emails)
&& Objects.equals(this.name, whatsAppContactContent.name)
&& Objects.equals(this.org, whatsAppContactContent.org)
&& Objects.equals(this.phones, whatsAppContactContent.phones)
&& Objects.equals(this.urls, whatsAppContactContent.urls);
}
@Override
public int hashCode() {
return Objects.hash(addresses, birthday, emails, name, org, phones, urls);
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class WhatsAppContactContent {")
.append(newLine)
.append(" addresses: ")
.append(toIndentedString(addresses))
.append(newLine)
.append(" birthday: ")
.append(toIndentedString(birthday))
.append(newLine)
.append(" emails: ")
.append(toIndentedString(emails))
.append(newLine)
.append(" name: ")
.append(toIndentedString(name))
.append(newLine)
.append(" org: ")
.append(toIndentedString(org))
.append(newLine)
.append(" phones: ")
.append(toIndentedString(phones))
.append(newLine)
.append(" urls: ")
.append(toIndentedString(urls))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}