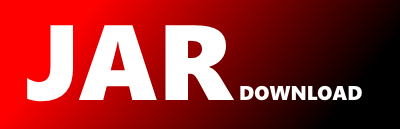
com.infomaximum.database.engine.BaseIntervalRecordIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rdao Show documentation
Show all versions of rdao Show documentation
Library for creating a light cluster
The newest version!
package com.infomaximum.database.engine;
import com.infomaximum.database.Record;
import com.infomaximum.database.domainobject.filter.BaseIntervalFilter;
import com.infomaximum.database.domainobject.filter.SortDirection;
import com.infomaximum.database.exception.DatabaseException;
import com.infomaximum.database.exception.IllegalTypeException;
import com.infomaximum.database.provider.DBDataReader;
import com.infomaximum.database.provider.DBIterator;
import com.infomaximum.database.provider.KeyPattern;
import com.infomaximum.database.provider.KeyValue;
import com.infomaximum.database.schema.dbstruct.DBBaseIntervalIndex;
import com.infomaximum.database.schema.dbstruct.DBField;
import com.infomaximum.database.schema.dbstruct.DBTable;
import com.infomaximum.database.utils.HashIndexUtils;
import com.infomaximum.database.utils.IntervalIndexUtils;
import com.infomaximum.database.utils.key.BaseIntervalIndexKey;
import java.util.*;
abstract class BaseIntervalRecordIterator extends BaseIndexRecordIterator {
private final List checkedFilterFields;
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy