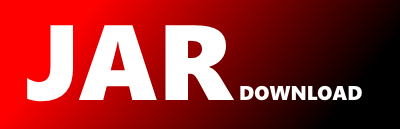
com.seleniumtests.reporter.SeleniumTestsReporter Maven / Gradle / Ivy
/**
* Orignal work: Copyright 2015 www.seleniumtests.com
* Modified work: Copyright 2016 www.infotel.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.seleniumtests.reporter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.lang.reflect.Method;
import java.net.URISyntaxException;
import java.net.URL;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Comparator;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.TreeSet;
import org.apache.commons.lang.StringUtils;
import org.apache.velocity.Template;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.app.VelocityEngine;
import org.testng.IInvokedMethod;
import org.testng.IReporter;
import org.testng.IResultMap;
import org.testng.ISuite;
import org.testng.ISuiteResult;
import org.testng.ITestContext;
import org.testng.ITestNGMethod;
import org.testng.ITestResult;
import org.testng.Reporter;
import org.testng.xml.XmlSuite;
import com.seleniumtests.core.SeleniumTestsContext;
import com.seleniumtests.core.SeleniumTestsContextManager;
import com.seleniumtests.core.SeleniumTestsPageListener;
import com.seleniumtests.customexception.ScenarioException;
import com.seleniumtests.driver.DriverMode;
import com.seleniumtests.driver.TestType;
import com.seleniumtests.util.StringUtility;
import com.thoughtworks.qdox.JavaDocBuilder;
import com.thoughtworks.qdox.model.JavaClass;
import com.thoughtworks.qdox.model.JavaMethod;
import com.thoughtworks.qdox.model.Type;
public class SeleniumTestsReporter extends CommonReporter implements IReporter {
private static final String IMAGES_DIR = "images";
private static final String LIGHTBOX_DIR = "lightbox";
private static final String MKTREE_DIR = "mktree";
private static final String YUKONTOOLBOX_DIR = "yukontoolbox";
private static final String REPORTER_DIR = "reporter";
protected PrintWriter mOut;
private int mTreeId = 0;
private String outputDirectory;
private String resources;
private JavaDocBuilder builder = null;
private String generationErrorMessage = null;
Map methodsByGroup = null;
protected class TestMethodSorter implements Comparator {
/**
* Arrange methods by class and method name.
*/
@Override
public int compare(final T o1, final T o2) {
int r = o1.getTestClass().getName().compareTo(o2.getTestClass().getName());
if (r == 0) {
r = o1.getMethodName().compareTo(o2.getMethodName());
}
return r;
}
}
protected class TestResultSorter implements Comparator {
/**
* Arrange methods by class and method name.
*/
@Override
public int compare(final T o1, final T o2) {
String sig1 = StringUtility.constructMethodSignature(o1.getMethod().getConstructorOrMethod().getMethod(), o1.getParameters());
String sig2 = StringUtility.constructMethodSignature(o2.getMethod().getConstructorOrMethod().getMethod(), o2.getParameters());
return sig1.compareTo(sig2);
}
}
public static String escape(final String string) {
if (null == string) {
return string;
}
return string.replaceAll("\n", "
");
}
public static void writeResourceToFile(final File file, final String resourceName, final Class> aClass)
throws IOException {
InputStream inputStream = aClass.getResourceAsStream("/" + resourceName);
if (inputStream == null) {
logger.error("can not find resource on the class path: " + resourceName);
} else {
try (
FileOutputStream outputStream = new FileOutputStream(file);
){
int i;
byte[] buffer = new byte[4096];
while (0 < (i = inputStream.read(buffer))) {
outputStream.write(buffer, 0, i);
}
} finally {
inputStream.close();
}
}
}
private void addAllTestResults(final Set testResults, final IResultMap resultMap) {
if (resultMap != null) {
testResults.addAll(resultMap.getAllResults());
}
}
public void copyResources() throws IOException {
new File(outputDirectory + File.separator + RESOURCES_DIR).mkdir();
new File(outputDirectory + File.separator + RESOURCES_DIR + File.separator + "css").mkdir();
new File(outputDirectory + File.separator + RESOURCES_DIR + File.separator + IMAGES_DIR).mkdir();
new File(outputDirectory + File.separator + RESOURCES_DIR + File.separator + IMAGES_DIR + File.separator
+ LIGHTBOX_DIR).mkdir();
new File(outputDirectory + File.separator + RESOURCES_DIR + File.separator + IMAGES_DIR + File.separator + MKTREE_DIR)
.mkdir();
new File(outputDirectory + File.separator + RESOURCES_DIR + File.separator + IMAGES_DIR + File.separator
+ YUKONTOOLBOX_DIR).mkdir();
new File(outputDirectory + File.separator + RESOURCES_DIR + File.separator + "js").mkdir();
List resourceList = new ArrayList<>();
resourceList.add(REPORTER_DIR + File.separator + "css" + File.separator + "report.css");
resourceList.add(REPORTER_DIR + File.separator + "css" + File.separator + "jquery.lightbox-0.5.css");
resourceList.add(REPORTER_DIR + File.separator + "css" + File.separator + "mktree.css");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + LIGHTBOX_DIR + File.separator
+ "seleniumtests_lightbox-blank.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + LIGHTBOX_DIR + File.separator
+ "seleniumtests_lightbox-btn-close.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + LIGHTBOX_DIR + File.separator
+ "seleniumtests_lightbox-btn-next.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + LIGHTBOX_DIR + File.separator
+ "seleniumtests_lightbox-btn-prev.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + LIGHTBOX_DIR + File.separator
+ "seleniumtests_lightbox-ico-loading.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + MKTREE_DIR + File.separator
+ "seleniumtests_bullet.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + MKTREE_DIR + File.separator
+ "seleniumtests_minus.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + MKTREE_DIR + File.separator
+ "seleniumtests_plus.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + MKTREE_DIR + File.separator
+ "seleniumtests_test1.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + MKTREE_DIR + File.separator
+ "seleniumtests_test2.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + MKTREE_DIR + File.separator
+ "seleniumtests_test3.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + MKTREE_DIR + File.separator
+ "seleniumtests_test3.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_footer_grad.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_grey_bl.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_grey_br.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_hovertab_l.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_hovertab_r.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_tabbed_nav_goldgradbg.png");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_table_sep_left.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_table_sep_right.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_table_zebrastripe_left.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_table_zebrastripe_right.gif");
resourceList.add(REPORTER_DIR + File.separator + IMAGES_DIR + File.separator + YUKONTOOLBOX_DIR + File.separator
+ "seleniumtests_yellow_tr.gif");
resourceList.add(REPORTER_DIR + File.separator + "js" + File.separator + "jquery-1.10.2.min.js");
resourceList.add(REPORTER_DIR + File.separator + "js" + File.separator + "jquery.lightbox-0.5.min.js");
resourceList.add(REPORTER_DIR + File.separator + "js" + File.separator + "mktree.js");
resourceList.add(REPORTER_DIR + File.separator + "js" + File.separator + "report.js");
resourceList.add(REPORTER_DIR + File.separator + "js" + File.separator + "browserdetect.js");
for (String resourceName : resourceList) {
File f = new File(outputDirectory, resourceName.replace(REPORTER_DIR, RESOURCES_DIR));
resourceName = resourceName.replaceAll("\\\\", "/");
logger.debug("Begin to write resource " + resourceName + " to file " + f.getAbsolutePath());
writeResourceToFile(f, resourceName, SeleniumTestsReporter.class);
}
}
/**
* Completes HTML stream.
*
* @param out
*/
protected void endHtml(final PrintWriter out) {
out.println("