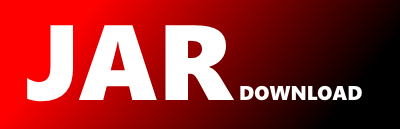
com.seleniumtests.util.osutility.OSUtilityWindows Maven / Gradle / Ivy
/**
* Orignal work: Copyright 2015 www.seleniumtests.com
* Modified work: Copyright 2016 www.infotel.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.seleniumtests.util.osutility;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import com.seleniumtests.driver.BrowserType;
import com.sun.jna.platform.win32.Advapi32Util;
import com.sun.jna.platform.win32.Win32Exception;
import com.sun.jna.platform.win32.WinReg;
public class OSUtilityWindows extends OSUtility {
Pattern versionPattern = Pattern.compile(".*?(\\d+\\.\\d+\\.\\d+).*?");
@Override
public int getIEVersion() {
String output = OSCommand.executeCommandAndWait("reg query \"HKLM\\Software\\Microsoft\\Internet Explorer\" /v svcVersion");
if (output.split("\n").length < 3) {
output = OSCommand.executeCommandAndWait("reg query \"HKLM\\Software\\Microsoft\\Internet Explorer\" /v Version");
}
String internetExplorerValue = output.split("\n")[2];
String version = internetExplorerValue.trim().split(" ")[2];
version = version.trim().split("\\.")[0];
return Integer.parseInt(version);
}
/**
* @param fast : true gets only Image Name and pId of the process
* @return list of ProcessInfo
*/
@Override
public List getRunningProcessList(){
/*
* Output command : Image name ; PID ; Session name ; Session# ; Mem Usage .
* In Windows 7, windir = C:\Windows\
* /NH will not display column headers
* and /V displays also : Status ; Username ; CPU time ; Windows title .
* or /SVC displays only : Image name ; PID ; Services .
*/
String command = System.getenv("windir") + "\\system32\\" + "tasklist.exe /NH /SVC";
List strProcessList = Arrays.asList(OSCommand.executeCommandAndWait(command).split("\n"));
List processInfoList = new ArrayList<>();
for (String sentence : strProcessList) {
sentence = sentence.toLowerCase();
ProcessInfo processInfo = new ProcessInfo();
Pattern pTasklist = Pattern.compile("(system|.*\\.exe)\\s+(\\d+).*");
Matcher m = pTasklist.matcher(sentence);
if (m.matches()) {
processInfo.setName(m.group(1).replaceAll(".exe", ""));
processInfo.setPid(m.group(2));
processInfoList.add(processInfo);
}
}
return processInfoList;
}
/**
* Terminate process from command line terminal.
* @param process
* @param force to kill the process
* @return
* @throws IOException
*/
@Override
public String killProcess(String pid, boolean force) {
if (force) {
return OSCommand.executeCommandAndWait("taskkill /F /PID " + pid);
} else {
return OSCommand.executeCommandAndWait("taskkill /PID " + pid);
}
}
@Override
public String getProgramExtension() {
return ".exe";
}
@Override
public String getOSBuild() {
String version = OSCommand.executeCommandAndWait("cmd /C ver").replace("\r", "").replace("\n", "").trim();
Matcher versionMatcher = versionPattern.matcher(version);
if (versionMatcher.matches()) {
return versionMatcher.group(1);
} else {
logger.error("could not get Windows version");
return "5000";
}
}
@Override
public List getInstalledBrowsers() {
List browserList = new ArrayList<>();
// look for Firefox
try {
Advapi32Util.registryGetStringValue(WinReg.HKEY_CLASSES_ROOT, "FirefoxHTML\\shell\\open\\command", "");
browserList.add(BrowserType.FIREFOX);
} catch (Win32Exception e) {}
// look for chrome
try {
Advapi32Util.registryGetStringValue(WinReg.HKEY_LOCAL_MACHINE, "Software\\Classes\\ChromeHTML\\shell\\open\\command", "");
browserList.add(BrowserType.CHROME);
} catch (Win32Exception e) {}
// look for safari
try {
Advapi32Util.registryGetStringValue(WinReg.HKEY_LOCAL_MACHINE, "Software\\Classes\\SafariHTML\\shell\\open\\command", "");
browserList.add(BrowserType.SAFARI);
} catch (Win32Exception e) {}
// look for ie
try {
Advapi32Util.registryGetStringValue(WinReg.HKEY_LOCAL_MACHINE, "Software\\Microsoft\\Windows\\CurrentVersion\\App Paths\\IEXPLORE.EXE", "");
browserList.add(BrowserType.INTERNET_EXPLORER);
} catch (Win32Exception e) {}
// look for edge
try {
Advapi32Util.registryGetStringValue(WinReg.HKEY_CURRENT_USER, "Software\\Microsoft\\MicrosoftEdge\\Main", "EdgeSwitchingOSBuildNumber");
browserList.add(BrowserType.EDGE);
} catch (Win32Exception e) {}
return browserList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy