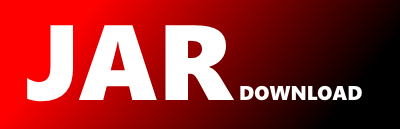
com.seleniumtests.uipage.ExpectedConditionsC Maven / Gradle / Ivy
package com.seleniumtests.uipage;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedCondition;
import com.seleniumtests.uipage.htmlelements.HtmlElement;
public class ExpectedConditionsC {
private ExpectedConditionsC() {
}
/**
* An expectation for checking that an element is present on the DOM of a page. This does not
* necessarily mean that the element is visible.
*
* @param element the HtmlElement to locate
* @return the WebElement once it is located
*/
public static ExpectedCondition presenceOfElementLocated(final HtmlElement element) {
return new ExpectedCondition() {
@Override
public WebElement apply(WebDriver driver) {
element.findElement(false, false);
return element.getRealElement();
}
@Override
public String toString() {
return "presence of element: " + element;
}
};
}
public static ExpectedCondition absenceOfElementLocated(final HtmlElement element) {
return new ExpectedCondition() {
@Override
public Boolean apply(WebDriver driver) {
try {
element.findElement(false, false);
element.getRealElement();
// element is there, this is not what we want
return false;
} catch (Exception e) {
return true;
}
}
@Override
public String toString() {
return "absence of element: " + element;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy