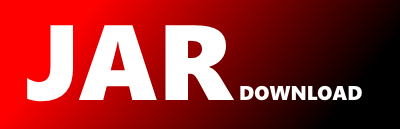
com.infotel.seleniumrobot.grid.tasks.CommandTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of seleniumRobot-grid Show documentation
Show all versions of seleniumRobot-grid Show documentation
Selenium grid extension for mobile testing
package com.infotel.seleniumrobot.grid.tasks;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.apache.log4j.Logger;
import com.infotel.seleniumrobot.grid.exceptions.TaskException;
import com.infotel.seleniumrobot.grid.servlets.server.NodeTaskServlet;
import com.seleniumtests.util.osutility.OSCommand;
import com.seleniumtests.util.osutility.OSUtility;
public class CommandTask implements Task {
private static final Logger logger = Logger.getLogger(NodeTaskServlet.class);
private static final List WINDOWS_COMMAND_WHITE_LIST = Arrays.asList("echo", "cmdkey");
private static final List LINUX_COMMAND_WHITE_LIST = Arrays.asList("echo");
private static final List MAC_COMMAND_WHITE_LIST = Arrays.asList("echo");
private String command = "";
private String result = "";
private List args = new ArrayList();
public void setCommand(String command, List args) {
this.command = command;
this.args = args;
}
@Override
public void execute() {
result = "";
if (command == null || command.isEmpty()) {
throw new TaskException("No command provided");
} else if (OSUtility.isLinux() && LINUX_COMMAND_WHITE_LIST.contains(command)
|| OSUtility.isWindows() && WINDOWS_COMMAND_WHITE_LIST.contains(command)
|| OSUtility.isMac() && MAC_COMMAND_WHITE_LIST.contains(command)) {
logger.error(String.format("Executing command %s", command));
args.add(0, command);
result = OSCommand.executeCommandAndWait(args.toArray(new String[] {}));
} else {
throw new TaskException(String.format("Command %s is not supported", command));
}
}
public String getResult() {
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy