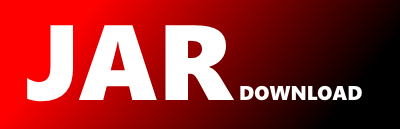
com.innovativeastrosolutions.astrosoftcore.beans.AstroData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AstrosoftCore Show documentation
Show all versions of AstrosoftCore Show documentation
https://github.com/erajasekar/AstrosoftCore
The newest version!
/**
* AstroData.java
*
* Created on December 14, 2002, 12:08 PM
*
* @author E. Rajasekar
*/
package com.innovativeastrosolutions.astrosoftcore.beans;
import java.util.Calendar;
import java.util.GregorianCalendar;
import com.innovativeastrosolutions.astrosoftcore.consts.Sex;
import com.innovativeastrosolutions.astrosoftcore.util.AstroUtil;
import com.innovativeastrosolutions.astrosoftcore.util.Options;
public class AstroData {
private String personName;
private Sex sex;
private Place place;
private int year;
private int month;
private int date;
private int hour;
private int minutes;
private int seconds;
private double time;
private double timeGMT;
private Calendar calendar;
private Options options;
public AstroData(
String name, Sex sex, int date, int month, int year, int hr, int min, int secs,
Place place, Options options) {
personName = name;
this.sex = sex;
this.date = date;
this.month = month;
this.year = year;
hour = hr;
minutes = min;
seconds = secs;
this.place = place;
this.options = options;
time = AstroUtil.decimal(hr, min, 0);
timeGMT = time - place.timeZone();
calendar = new GregorianCalendar(year, month - 1, date, hour, minutes, seconds);
}
public AstroData(
String name, int date, int month, int year, int hr, int min, int secs,
Place place, Options options){
this(name,null,date,month,year,hr,min,secs,place, options);
}
public String name() {
return personName;
}
public int year() {
return year;
}
public int month() {
return month;
}
public int date() {
return date;
}
public int hour() {
return hour;
}
public int minutes() {
return minutes;
}
public double latitude() {
return place.latitude();
}
public double longitude() {
return place.longitude();
}
public String place() {
return place.city();
}
public double timeZone() {
return place.timeZone();
}
public double time() {
return time;
}
public double timeGMT(){
return timeGMT;
}
public int seconds(){
return seconds;
}
public Calendar calender() {
return calendar;
}
public Place getPlace() {
return place;
}
public String birthDayString(){
return AstroUtil.formatDate(calendar.getTime());
}
public Sex sex(){
return sex;
}
public Options options() {
return options;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy