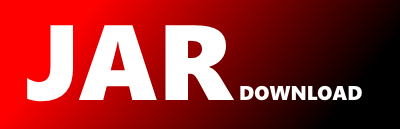
com.innovenso.townplan.dsl.traits.HasRaciRelationships.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of innovenso-townplanner-dsl Show documentation
Show all versions of innovenso-townplanner-dsl Show documentation
Domain Specific Language for the Innovenso Townplanner
package com.innovenso.townplan.dsl.traits
import com.innovenso.townplan.api.value.RelationshipType
import com.innovenso.townplan.dsl.TownPlanConfigurer
import com.innovenso.townplan.dsl.concepts.AbstractConceptConfigurer
import com.innovenso.townplan.dsl.concepts.relationship.RelationshipConfigurer
import lombok.NonNull
import static groovy.lang.Closure.DELEGATE_ONLY
trait HasRaciRelationships extends CanConfigureConfigurer {
abstract String getKey();
abstract TownPlanConfigurer getTownPlanConfigurer();
RelationshipConfigurer isResponsibleFor(@DelegatesTo(value = RelationshipConfigurer, strategy = DELEGATE_ONLY) Closure configurer) {
getTownPlanConfigurer().relationship configureConfigurer(new RelationshipConfigurer(townPlanConfigurer, RelationshipType.RESPONSIBLE, getKey()), configurer)
}
RelationshipConfigurer isResponsibleFor(@NonNull String targetKey) {
isResponsibleFor(targetKey, "is responsible for")
}
RelationshipConfigurer isResponsibleFor(@NonNull String targetKey, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.RESPONSIBLE, getKey(), targetKey, title)
}
RelationshipConfigurer isResponsibleFor(@NonNull AbstractConceptConfigurer target) {
isResponsibleFor(target, "is responsible for")
}
RelationshipConfigurer isResponsibleFor(@NonNull AbstractConceptConfigurer target, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.RESPONSIBLE, getKey(), target.key, title)
}
RelationshipConfigurer isAccountableFor(@DelegatesTo(value = RelationshipConfigurer, strategy = DELEGATE_ONLY) Closure configurer) {
getTownPlanConfigurer().relationship configureConfigurer(new RelationshipConfigurer(townPlanConfigurer, RelationshipType.ACCOUNTABLE, getKey()), configurer)
}
RelationshipConfigurer isAccountableFor(@NonNull String targetKey) {
isAccountableFor(targetKey, "is accountable for")
}
RelationshipConfigurer isAccountableFor(@NonNull String targetKey, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.ACCOUNTABLE, getKey(), targetKey, title)
}
RelationshipConfigurer isAccountableFor(@NonNull AbstractConceptConfigurer target) {
isAccountableFor(target, "is accountable for")
}
RelationshipConfigurer isAccountableFor(@NonNull AbstractConceptConfigurer target, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.ACCOUNTABLE, getKey(), target.key, title)
}
RelationshipConfigurer hasBeenConsultedAbout(@DelegatesTo(value = RelationshipConfigurer, strategy = DELEGATE_ONLY) Closure configurer) {
getTownPlanConfigurer().relationship configureConfigurer(new RelationshipConfigurer(townPlanConfigurer, RelationshipType.HAS_BEEN_CONSULTED, getKey()), configurer)
}
RelationshipConfigurer hasBeenConsultedAbout(@NonNull String targetKey) {
hasBeenConsultedAbout(targetKey, "has been consulted about")
}
RelationshipConfigurer hasBeenConsultedAbout(@NonNull String targetKey, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.HAS_BEEN_CONSULTED, getKey(), targetKey, title)
}
RelationshipConfigurer hasBeenConsultedAbout(@NonNull AbstractConceptConfigurer target) {
hasBeenConsultedAbout(target, "has been consulted about")
}
RelationshipConfigurer hasBeenConsultedAbout(@NonNull AbstractConceptConfigurer target, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.HAS_BEEN_CONSULTED, getKey(), target.key, title)
}
RelationshipConfigurer isToBeConsultedAbout(@DelegatesTo(value = RelationshipConfigurer, strategy = DELEGATE_ONLY) Closure configurer) {
getTownPlanConfigurer().relationship configureConfigurer(new RelationshipConfigurer(townPlanConfigurer, RelationshipType.TO_BE_CONSULTED, getKey()), configurer)
}
RelationshipConfigurer isToBeConsultedAbout(@NonNull String targetKey) {
isToBeConsultedAbout(targetKey, "is to be consulted about")
}
RelationshipConfigurer isToBeConsultedAbout(@NonNull String targetKey, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.TO_BE_CONSULTED, getKey(), targetKey, title)
}
RelationshipConfigurer isToBeConsultedAbout(@NonNull AbstractConceptConfigurer target) {
isToBeConsultedAbout(target, "is to be consulted about")
}
RelationshipConfigurer isToBeConsultedAbout(@NonNull AbstractConceptConfigurer target, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.TO_BE_CONSULTED, getKey(), target.key, title)
}
RelationshipConfigurer hasBeenInformedAbout(@DelegatesTo(value = RelationshipConfigurer, strategy = DELEGATE_ONLY) Closure configurer) {
getTownPlanConfigurer().relationship configureConfigurer(new RelationshipConfigurer(townPlanConfigurer, RelationshipType.HAS_BEEN_INFORMED, getKey()), configurer)
}
RelationshipConfigurer hasBeenInformedAbout(@NonNull String targetKey) {
hasBeenInformedAbout(targetKey, "has been informed about")
}
RelationshipConfigurer hasBeenInformedAbout(@NonNull String targetKey, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.HAS_BEEN_INFORMED, getKey(), targetKey, title)
}
RelationshipConfigurer hasBeenInformedAbout(@NonNull AbstractConceptConfigurer target) {
hasBeenInformedAbout(target, "has been informed about")
}
RelationshipConfigurer hasBeenInformedAbout(@NonNull AbstractConceptConfigurer target, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.HAS_BEEN_INFORMED, getKey(), target.key, title)
}
RelationshipConfigurer isToBeInformedAbout(@DelegatesTo(value = RelationshipConfigurer, strategy = DELEGATE_ONLY) Closure configurer) {
getTownPlanConfigurer().relationship configureConfigurer(new RelationshipConfigurer(townPlanConfigurer, RelationshipType.TO_BE_INFORMED, getKey()), configurer)
}
RelationshipConfigurer isToBeInformedAbout(@NonNull String targetKey) {
isToBeInformedAbout(targetKey, "is to be informed about")
}
RelationshipConfigurer isToBeInformedAbout(@NonNull String targetKey, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.TO_BE_INFORMED, getKey(), targetKey, title)
}
RelationshipConfigurer isToBeInformedAbout(@NonNull AbstractConceptConfigurer target) {
isToBeInformedAbout(target, "is to be informed about")
}
RelationshipConfigurer isToBeInformedAbout(@NonNull AbstractConceptConfigurer target, @NonNull String title) {
getTownPlanConfigurer().relationship new RelationshipConfigurer(townPlanConfigurer, RelationshipType.TO_BE_INFORMED, getKey(), target.key, title)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy