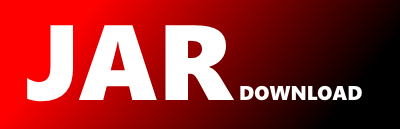
com.innovenso.townplanner.io.concepts.ITContainerExcelIO.scala Maven / Gradle / Ivy
package com.innovenso.townplanner.io.concepts
import com.innovenso.townplanner.io.concepts.ExcelFields._
import com.innovenso.townplanner.model.EnterpriseArchitecture
import com.innovenso.townplanner.model.concepts.{
Batch,
Cache,
Database,
DesktopUI,
EventStream,
Filesystem,
Firewall,
Gateway,
GenericContainer,
ItContainer,
ItContainerConfigurer,
Microservice,
MobileUI,
Queue,
Service,
SmartTVUI,
TerminalUI,
Topic,
WatchUI,
WebUI
}
object ITContainerExcelIO extends ConceptExcelIO[ItContainer] {
override def conceptClass: Class[ItContainer] = classOf[ItContainer]
override def sheetName: String = "IT Containers"
override def headerLabels: List[String] = List(
TITLE,
DESCRIPTION,
TYPE,
CRITICALITY,
CRITICALITY_CONSEQUENCES,
ARCHITECTURE_VERDICT,
ARCHITECTURE_VERDICT_EXPLANATION,
API_STYLE,
API_STYLE_DESCRIPTION,
API_AUTH_STYLE,
API_AUTH_DESCRIPTION,
API_SCOPE,
API_SCOPE_DESCRIPTION,
DDOS_PROTECTION,
DDOS_PROTECTION_DESCRIPTION,
RATE_LIMITING,
RATE_LIMITING_DESCRIPTION
)
override def cellValues(concept: ItContainer): List[String] = List(
concept.title,
concept.descriptions.map(_.value).mkString("\n\n"),
concept.containerType,
concept.criticality.name,
concept.criticality.consequences,
concept.architectureVerdict.name,
concept.architectureVerdict.description,
concept.api.map(_.style.title).getOrElse(""),
concept.api.flatMap(_.style.description).getOrElse(""),
concept.api.map(_.authentication.title).getOrElse(""),
concept.api.flatMap(_.authentication.description).getOrElse(""),
concept.api.map(_.scope.title).getOrElse(""),
concept.api.flatMap(_.scope.description).getOrElse(""),
concept.api.map(_.ddoSProtection.title).getOrElse(""),
concept.api.flatMap(_.ddoSProtection.description).getOrElse(""),
concept.api.map(_.rateLimiting.title).getOrElse(""),
concept.api.flatMap(_.rateLimiting.description).getOrElse("")
)
override def concept(
props: ConceptProperties,
enterpriseArchitecture: EnterpriseArchitecture
): Unit = {
val container = props(TYPE).toLowerCase match {
case "microservice" =>
enterpriseArchitecture describes Microservice(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "database" =>
enterpriseArchitecture describes Database(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "queue" =>
enterpriseArchitecture describes Queue(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "topic" =>
enterpriseArchitecture describes Topic(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "event stream" =>
enterpriseArchitecture describes EventStream(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "service" =>
enterpriseArchitecture describes Service(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "function" =>
enterpriseArchitecture describes com.innovenso.townplanner.model.concepts
.Function(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "filesystem" =>
enterpriseArchitecture describes Filesystem(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "gateway" =>
enterpriseArchitecture describes Gateway(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "proxy" =>
enterpriseArchitecture describes com.innovenso.townplanner.model.concepts
.Proxy(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "cache" =>
enterpriseArchitecture describes Cache(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "firewall" =>
enterpriseArchitecture describes Firewall(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "web ui" =>
enterpriseArchitecture describes WebUI(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "mobile ui" =>
enterpriseArchitecture describes MobileUI(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "watch ui" =>
enterpriseArchitecture describes WatchUI(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "desktop ui" =>
enterpriseArchitecture describes DesktopUI(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "terminal ui" =>
enterpriseArchitecture describes TerminalUI(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "smart tv ui" =>
enterpriseArchitecture describes SmartTVUI(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case "batch" =>
enterpriseArchitecture describes Batch(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
case _ =>
enterpriseArchitecture describes GenericContainer(
key = props.key,
sortKey = props.sortKey,
title = props.title
) as { it => configureContainer(props, it) }
}
println(s"imported $container")
}
private def configureContainer(
props: ConceptProperties,
it: ItContainerConfigurer[_ <: ItContainer]
) = {
props.descriptions.foreach(d => it has d)
it should props.architectureVerdict
it ratesImpactAs props.criticality
props.api.foreach(a => it has a)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy