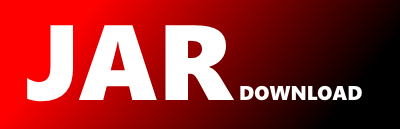
com.inrupt.client.parser.WwwAuthenticateParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of inrupt-client-parser Show documentation
Show all versions of inrupt-client-parser Show documentation
Parsing utilities for the Inrupt Java Client Libraries.
// Generated from com/inrupt/client/parser/WwwAuthenticate.g4 by ANTLR 4.13.0
package com.inrupt.client.parser;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast", "CheckReturnValue"})
public class WwwAuthenticateParser extends Parser {
static { RuntimeMetaData.checkVersion("4.13.0", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
T__0=1, AuthParam=2, AuthScheme=3, QuotedString=4, Token=5, Token68=6,
WS=7;
public static final int
RULE_wwwAuthenticate = 0, RULE_challenge = 1;
private static String[] makeRuleNames() {
return new String[] {
"wwwAuthenticate", "challenge"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, "','"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, null, "AuthParam", "AuthScheme", "QuotedString", "Token", "Token68",
"WS"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "WwwAuthenticate.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public WwwAuthenticateParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
@SuppressWarnings("CheckReturnValue")
public static class WwwAuthenticateContext extends ParserRuleContext {
public List challenge() {
return getRuleContexts(ChallengeContext.class);
}
public ChallengeContext challenge(int i) {
return getRuleContext(ChallengeContext.class,i);
}
public List WS() { return getTokens(WwwAuthenticateParser.WS); }
public TerminalNode WS(int i) {
return getToken(WwwAuthenticateParser.WS, i);
}
public WwwAuthenticateContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_wwwAuthenticate; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof WwwAuthenticateListener ) ((WwwAuthenticateListener)listener).enterWwwAuthenticate(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof WwwAuthenticateListener ) ((WwwAuthenticateListener)listener).exitWwwAuthenticate(this);
}
}
public final WwwAuthenticateContext wwwAuthenticate() throws RecognitionException {
WwwAuthenticateContext _localctx = new WwwAuthenticateContext(_ctx, getState());
enterRule(_localctx, 0, RULE_wwwAuthenticate);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(4);
challenge();
setState(12);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==T__0) {
{
{
setState(5);
match(T__0);
setState(7);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WS) {
{
setState(6);
match(WS);
}
}
setState(9);
challenge();
}
}
setState(14);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ChallengeContext extends ParserRuleContext {
public TerminalNode AuthScheme() { return getToken(WwwAuthenticateParser.AuthScheme, 0); }
public List WS() { return getTokens(WwwAuthenticateParser.WS); }
public TerminalNode WS(int i) {
return getToken(WwwAuthenticateParser.WS, i);
}
public List Token68() { return getTokens(WwwAuthenticateParser.Token68); }
public TerminalNode Token68(int i) {
return getToken(WwwAuthenticateParser.Token68, i);
}
public List AuthParam() { return getTokens(WwwAuthenticateParser.AuthParam); }
public TerminalNode AuthParam(int i) {
return getToken(WwwAuthenticateParser.AuthParam, i);
}
public ChallengeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_challenge; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof WwwAuthenticateListener ) ((WwwAuthenticateListener)listener).enterChallenge(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof WwwAuthenticateListener ) ((WwwAuthenticateListener)listener).exitChallenge(this);
}
}
public final ChallengeContext challenge() throws RecognitionException {
ChallengeContext _localctx = new ChallengeContext(_ctx, getState());
enterRule(_localctx, 2, RULE_challenge);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(15);
match(AuthScheme);
setState(36);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WS) {
{
{
setState(16);
match(WS);
setState(32);
_errHandler.sync(this);
switch (_input.LA(1)) {
case Token68:
{
setState(17);
match(Token68);
}
break;
case AuthParam:
{
setState(18);
match(AuthParam);
setState(29);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,4,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(20);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WS) {
{
setState(19);
match(WS);
}
}
setState(22);
match(T__0);
setState(24);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WS) {
{
setState(23);
match(WS);
}
}
setState(26);
match(AuthParam);
}
}
}
setState(31);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,4,_ctx);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(38);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\u0004\u0001\u0007(\u0002\u0000\u0007\u0000\u0002\u0001\u0007\u0001\u0001"+
"\u0000\u0001\u0000\u0001\u0000\u0003\u0000\b\b\u0000\u0001\u0000\u0005"+
"\u0000\u000b\b\u0000\n\u0000\f\u0000\u000e\t\u0000\u0001\u0001\u0001\u0001"+
"\u0001\u0001\u0001\u0001\u0001\u0001\u0003\u0001\u0015\b\u0001\u0001\u0001"+
"\u0001\u0001\u0003\u0001\u0019\b\u0001\u0001\u0001\u0005\u0001\u001c\b"+
"\u0001\n\u0001\f\u0001\u001f\t\u0001\u0003\u0001!\b\u0001\u0005\u0001"+
"#\b\u0001\n\u0001\f\u0001&\t\u0001\u0001\u0001\u0000\u0000\u0002\u0000"+
"\u0002\u0000\u0000,\u0000\u0004\u0001\u0000\u0000\u0000\u0002\u000f\u0001"+
"\u0000\u0000\u0000\u0004\f\u0003\u0002\u0001\u0000\u0005\u0007\u0005\u0001"+
"\u0000\u0000\u0006\b\u0005\u0007\u0000\u0000\u0007\u0006\u0001\u0000\u0000"+
"\u0000\u0007\b\u0001\u0000\u0000\u0000\b\t\u0001\u0000\u0000\u0000\t\u000b"+
"\u0003\u0002\u0001\u0000\n\u0005\u0001\u0000\u0000\u0000\u000b\u000e\u0001"+
"\u0000\u0000\u0000\f\n\u0001\u0000\u0000\u0000\f\r\u0001\u0000\u0000\u0000"+
"\r\u0001\u0001\u0000\u0000\u0000\u000e\f\u0001\u0000\u0000\u0000\u000f"+
"$\u0005\u0003\u0000\u0000\u0010 \u0005\u0007\u0000\u0000\u0011!\u0005"+
"\u0006\u0000\u0000\u0012\u001d\u0005\u0002\u0000\u0000\u0013\u0015\u0005"+
"\u0007\u0000\u0000\u0014\u0013\u0001\u0000\u0000\u0000\u0014\u0015\u0001"+
"\u0000\u0000\u0000\u0015\u0016\u0001\u0000\u0000\u0000\u0016\u0018\u0005"+
"\u0001\u0000\u0000\u0017\u0019\u0005\u0007\u0000\u0000\u0018\u0017\u0001"+
"\u0000\u0000\u0000\u0018\u0019\u0001\u0000\u0000\u0000\u0019\u001a\u0001"+
"\u0000\u0000\u0000\u001a\u001c\u0005\u0002\u0000\u0000\u001b\u0014\u0001"+
"\u0000\u0000\u0000\u001c\u001f\u0001\u0000\u0000\u0000\u001d\u001b\u0001"+
"\u0000\u0000\u0000\u001d\u001e\u0001\u0000\u0000\u0000\u001e!\u0001\u0000"+
"\u0000\u0000\u001f\u001d\u0001\u0000\u0000\u0000 \u0011\u0001\u0000\u0000"+
"\u0000 \u0012\u0001\u0000\u0000\u0000!#\u0001\u0000\u0000\u0000\"\u0010"+
"\u0001\u0000\u0000\u0000#&\u0001\u0000\u0000\u0000$\"\u0001\u0000\u0000"+
"\u0000$%\u0001\u0000\u0000\u0000%\u0003\u0001\u0000\u0000\u0000&$\u0001"+
"\u0000\u0000\u0000\u0007\u0007\f\u0014\u0018\u001d $";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy