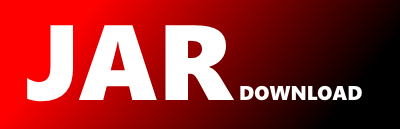
com.insightfullogic.lambdabehave.expectations.CollectionExpectation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda-behave Show documentation
Show all versions of lambda-behave Show documentation
A modern testing and behavioural specification framework for Java 8
package com.insightfullogic.lambdabehave.expectations;
import org.hamcrest.Matcher;
import org.hamcrest.Matchers;
import java.util.Collection;
import java.util.List;
import static org.hamcrest.Matchers.empty;
import static org.junit.Assert.assertThat;
public final class CollectionExpectation extends BoundExpectation> {
CollectionExpectation(final Collection objectUnderTest, final boolean positive) {
super(objectUnderTest, positive);
}
public CollectionExpectation isEmpty() {
return matches(empty());
}
public CollectionExpectation hasItem(final T item) {
return matches(Matchers.hasItem(item));
}
public CollectionExpectation hasItem(final Matcher super T> item) {
return matches(Matchers.hasItem(item));
}
public CollectionExpectation hasItems(final T ... items) {
return matches(Matchers.hasItems(items));
}
public CollectionExpectation contains(final T ... items) {
return matches(Matchers.contains(items));
}
public CollectionExpectation contains(final Matcher super T> ... items) {
return matches(Matchers.contains(items));
}
public CollectionExpectation contains(final List> items) {
return matches(Matchers.contains(items));
}
public CollectionExpectation containsInAnyOrder(final T ... items) {
return matches(Matchers.containsInAnyOrder(items));
}
public CollectionExpectation containsInAnyOrder(final Matcher super T> ... items) {
return matches(Matchers.containsInAnyOrder(items));
}
public CollectionExpectation containsInAnyOrder(final Collection> items) {
return matches(Matchers.containsInAnyOrder(items));
}
public CollectionExpectation emptyCollectionOf(final Class type) {
return matches(Matchers.emptyCollectionOf(type));
}
public CollectionExpectation hasSize(final int size) {
return matches(Matchers.hasSize(size));
}
public CollectionExpectation hasSize(final Matcher super Integer> size) {
return matches(Matchers.hasSize(size));
}
public CollectionExpectation hasItems(final Matcher super T> ... items) {
return matches(Matchers.hasItems(items));
}
private CollectionExpectation matches(final Matcher super Collection> matcher) {
assertThat(objectUnderTest, negatedIfNeeded(matcher));
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy