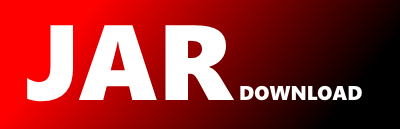
com.insightfullogic.lambdabehave.expectations.Expect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda-behave Show documentation
Show all versions of lambda-behave Show documentation
A modern testing and behavioural specification framework for Java 8
package com.insightfullogic.lambdabehave.expectations;
import com.insightfullogic.lambdabehave.Block;
import org.junit.Assert;
import org.mockito.Mockito;
import org.mockito.stubbing.Stubber;
import java.util.Collection;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
public final class Expect {
public BoundExpectation that(final T value) {
return new BoundExpectation(value, true);
}
public CollectionExpectation that(final Collection collection) {
return new CollectionExpectation<>(collection, true);
}
public > ComparableExpectation that(final T comparable) {
return new ComparableExpectation(comparable, true);
}
public StringExpectation that(final String str) {
return new StringExpectation(str, true);
}
public DoubleExpectation that(final Double value) {
return new DoubleExpectation(value, true);
}
public ArrayExpectation that(final T[] array) {
return new ArrayExpectation<>(array, true);
}
public void exception(final Class extends Throwable> expectedException, final Block block) throws Exception {
String expectedName = expectedException.getName();
try {
block.run();
} catch (final Throwable throwable) {
if (!expectedException.isInstance(throwable)) {
String name = throwable.getClass().getName();
failure("Expected exception: " + expectedName + ", but " + name + " was thrown");
}
return;
}
failure("Expected exception: " + expectedName + ", but no exception was thrown");
}
// NB: no failure without a message because its a bad idea to not have test failure diagnostics
public void failure(final String message) {
Assert.fail(message);
}
@SuppressWarnings("unchecked")
public Stubber toAnswer(final Runnable method) {
return Mockito.doAnswer(invocation -> {
Object[] arguments = invocation.getArguments();
method.run();
return null;
});
}
@SuppressWarnings("unchecked")
public Stubber toAnswer(final Consumer method) {
return doAnswer(arguments -> method.accept((T) arguments[0]), 1);
}
@SuppressWarnings("unchecked")
public Stubber toAnswer(final BiConsumer method) {
return doAnswer(arguments ->
method.accept((F) arguments[0], (S) arguments[1]), 2);
}
@SuppressWarnings("unchecked")
public Stubber toAnswer(final TriConsumer method) {
return doAnswer(arguments ->
method.accept((F) arguments[0], (S) arguments[1], (T) arguments[2]), 3);
}
private Stubber doAnswer(final Consumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy