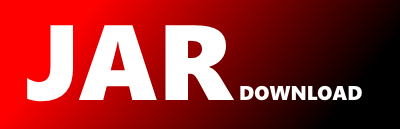
com.inspiresoftware.lib.dto.geda.interceptor.impl.RuntimeAdviceConfigResolverImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geda.spring-integration Show documentation
Show all versions of geda.spring-integration Show documentation
Provides GeDA core integration for Spring framework
/*
* This code is distributed under The GNU Lesser General Public License (LGPLv3)
* Please visit GNU site for LGPLv3 http://www.gnu.org/copyleft/lesser.html
*
* Copyright Denis Pavlov 2009
* Web: http://www.inspire-software.com
* SVN: https://geda-genericdto.svn.sourceforge.net/svnroot/geda-genericdto
*/
package com.inspiresoftware.lib.dto.geda.interceptor.impl;
import com.inspiresoftware.lib.dto.geda.annotations.Occurrence;
import com.inspiresoftware.lib.dto.geda.interceptor.AdviceConfig;
import com.inspiresoftware.lib.dto.geda.interceptor.AdviceConfigResolver;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Basic implementation of the resolved that examines method annotations in order to
* produce configuration objects. Provides method signature cache to store the
* configuration information in a permanent cache.
*
* Additionally this resolver keeps track of the methods that are not applicable and
* thus are blacklisted.
*
* User: denispavlov
* Date: Jan 26, 2012
* Time: 1:03:36 PM
*/
public class RuntimeAdviceConfigResolverImpl implements AdviceConfigResolver {
private static final Logger LOG = LoggerFactory.getLogger(RuntimeAdviceConfigResolverImpl.class);
private final Map blacklist = new ConcurrentHashMap();
private final Map> cache = new ConcurrentHashMap>();
public RuntimeAdviceConfigResolverImpl() {
}
/** {@inheritDoc} */
public Map resolve(final Method method, final Class> targetClass) {
final Integer methodCacheKey = methodCacheKey(method, targetClass);
if (isBlacklisted(methodCacheKey)) {
return Collections.emptyMap();
}
if (isCached(methodCacheKey)) {
return this.cache.get(methodCacheKey);
}
final Map cfg = resolveConfiguration(method, targetClass);
if (cfg.isEmpty()) {
this.blacklist.put(methodCacheKey, Boolean.TRUE);
} else {
cache.put(methodCacheKey, cfg);
if (LOG.isInfoEnabled()) {
LOG.info("Added GeDA configuration for method: {}.{}[p={}]... {} total mappings so far",
new Object[] {
targetClass == null ? method.getDeclaringClass().getCanonicalName() : targetClass.getCanonicalName(),
method.getName(),
method.getParameterTypes().length,
cache.size() });
}
}
return cfg;
}
boolean isCached(final Integer methodCacheKey) {
return this.cache.containsKey(methodCacheKey);
}
boolean isBlacklisted(final Integer methodCacheKey) {
return this.blacklist.containsKey(methodCacheKey);
}
Map resolveConfiguration(final Method method,
final Class> targetClass) {
return TransferableUtils.resolveConfiguration(method, targetClass);
}
Integer methodCacheKey(final Method method, final Class> targetClass) {
int hashKey = targetClass != null ? targetClass.getCanonicalName().hashCode() : method.getDeclaringClass().getCanonicalName().hashCode();
hashKey = 31 * hashKey + method.getName().hashCode();
final Class[] args = method.getParameterTypes();
if (args.length > 0) {
for (Class arg : args) {
hashKey = 31 * hashKey + arg.hashCode();
}
}
return Integer.valueOf(hashKey);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy