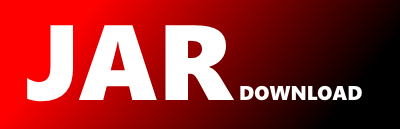
com.instaclustr.cassandra.backup.gcp.GCPBackuper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of instaclustr-backup-restore Show documentation
Show all versions of instaclustr-backup-restore Show documentation
Backup and restoration tooling for Cassandra
package com.instaclustr.cassandra.backup.gcp;
import static com.google.cloud.storage.Storage.PredefinedAcl.BUCKET_OWNER_FULL_CONTROL;
import java.io.InputStream;
import java.nio.channels.Channels;
import java.nio.channels.ReadableByteChannel;
import java.nio.file.Path;
import com.google.cloud.WriteChannel;
import com.google.cloud.storage.BlobId;
import com.google.cloud.storage.BlobInfo;
import com.google.cloud.storage.Storage;
import com.google.cloud.storage.StorageException;
import com.google.common.io.ByteStreams;
import com.google.inject.assistedinject.Assisted;
import com.google.inject.assistedinject.AssistedInject;
import com.instaclustr.cassandra.backup.gcp.GCPModule.GoogleStorageFactory;
import com.instaclustr.cassandra.backup.impl.OperationProgressTracker;
import com.instaclustr.cassandra.backup.impl.RemoteObjectReference;
import com.instaclustr.cassandra.backup.impl.backup.BackupCommitLogsOperationRequest;
import com.instaclustr.cassandra.backup.impl.backup.BackupOperationRequest;
import com.instaclustr.cassandra.backup.impl.backup.Backuper;
import com.instaclustr.threading.Executors.ExecutorServiceSupplier;
public class GCPBackuper extends Backuper {
private final Storage storage;
@AssistedInject
public GCPBackuper(final GoogleStorageFactory storageFactory,
final ExecutorServiceSupplier executorServiceSupplier,
@Assisted final BackupOperationRequest backupOperationRequest) {
super(backupOperationRequest, executorServiceSupplier);
this.storage = storageFactory.build(backupOperationRequest);
}
@AssistedInject
public GCPBackuper(final GoogleStorageFactory storageFactory,
final ExecutorServiceSupplier executorServiceSupplier,
@Assisted final BackupCommitLogsOperationRequest backupOperationRequest) {
super(backupOperationRequest, executorServiceSupplier);
this.storage = storageFactory.build(backupOperationRequest);
}
@Override
public RemoteObjectReference objectKeyToRemoteReference(final Path objectKey) {
return new GCPRemoteObjectReference(objectKey, resolveRemotePath(objectKey), request.storageLocation.bucket);
}
@Override
public FreshenResult freshenRemoteObject(final RemoteObjectReference object) {
final BlobId blobId = ((GCPRemoteObjectReference) object).blobId;
try {
storage.copy(new Storage.CopyRequest.Builder()
.setSource(blobId)
.setTarget(BlobInfo.newBuilder(blobId).build(), Storage.BlobTargetOption.predefinedAcl(BUCKET_OWNER_FULL_CONTROL))
.build());
return FreshenResult.FRESHENED;
} catch (final StorageException e) {
if (e.getCode() != 404) {
throw e;
}
return FreshenResult.UPLOAD_REQUIRED;
}
}
@Override
public void uploadFile(final long size,
final InputStream localFileStream,
final RemoteObjectReference object,
final OperationProgressTracker operationProgressTracker) throws Exception {
final BlobId blobId = ((GCPRemoteObjectReference) object).blobId;
try (final WriteChannel outputChannel = storage.writer(BlobInfo.newBuilder(blobId).build(), Storage.BlobWriteOption.predefinedAcl(BUCKET_OWNER_FULL_CONTROL));
final ReadableByteChannel inputChannel = Channels.newChannel(localFileStream)) {
ByteStreams.copy(inputChannel, outputChannel);
} finally {
operationProgressTracker.update();
}
}
@Override
public void cleanup() throws Exception {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy