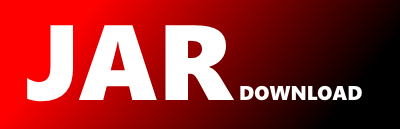
com.instaclustr.cassandra.backup.guice.BackupRestoreBindings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of instaclustr-backup-restore Show documentation
Show all versions of instaclustr-backup-restore Show documentation
Backup and restoration tooling for Cassandra
package com.instaclustr.cassandra.backup.guice;
import static com.google.inject.multibindings.MapBinder.newMapBinder;
import com.google.inject.Binder;
import com.google.inject.TypeLiteral;
import com.google.inject.assistedinject.FactoryModuleBuilder;
import com.google.inject.multibindings.MapBinder;
import com.google.inject.multibindings.Multibinder;
import com.google.inject.util.Types;
import com.instaclustr.cassandra.backup.impl.BucketService;
import com.instaclustr.cassandra.backup.impl.backup.Backuper;
import com.instaclustr.cassandra.backup.impl.restore.Restorer;
public class BackupRestoreBindings {
public static
void installBindings(final Binder binder,
final String typeId,
final Class restorerClass,
final Class backuperClass,
final Class bucketServiceClass) {
@SuppressWarnings("unchecked")
final TypeLiteral> restorerFactoryType =
(TypeLiteral>) TypeLiteral.get(Types.newParameterizedType(RestorerFactory.class, restorerClass));
@SuppressWarnings("unchecked")
final TypeLiteral> backuperFactoryType =
(TypeLiteral>) TypeLiteral.get(Types.newParameterizedType(BackuperFactory.class, backuperClass));
@SuppressWarnings("unchecked")
final TypeLiteral> bucketServiceFactoryType =
(TypeLiteral>) TypeLiteral.get(Types.newParameterizedType(BucketServiceFactory.class, bucketServiceClass));
binder.install(new FactoryModuleBuilder()
.implement(Restorer.class, restorerClass)
.build(restorerFactoryType));
binder.install(new FactoryModuleBuilder()
.implement(Backuper.class, backuperClass)
.build(backuperFactoryType));
binder.install(new FactoryModuleBuilder()
.implement(BucketService.class, bucketServiceClass)
.build(bucketServiceFactoryType));
// add an entry to the Map for the factory created above
MapBinder.newMapBinder(binder, TypeLiteral.get(String.class), TypeLiteral.get(RestorerFactory.class))
.addBinding(typeId).to(restorerFactoryType);
// add an entry to the Map for the factory created above
MapBinder.newMapBinder(binder, TypeLiteral.get(String.class), TypeLiteral.get(BackuperFactory.class))
.addBinding(typeId).to(backuperFactoryType);
// add an entry to the Map for the factory created above
MapBinder.newMapBinder(binder, TypeLiteral.get(String.class), TypeLiteral.get(BucketServiceFactory.class))
.addBinding(typeId).to(bucketServiceFactoryType);
// Map
newMapBinder(binder,
new TypeLiteral() {},
restorerFactoryType)
.addBinding(typeId).to(restorerFactoryType);
// Map
newMapBinder(binder,
new TypeLiteral() {},
backuperFactoryType)
.addBinding(typeId).to(backuperFactoryType);
// Map
newMapBinder(binder,
new TypeLiteral() {},
bucketServiceFactoryType)
.addBinding(typeId).to(bucketServiceFactoryType);
Multibinder.newSetBinder(binder, String.class, StorageProviders.class).addBinding().toInstance(typeId);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy