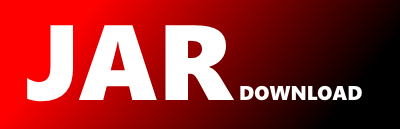
com.intel.analytics.bigdl.ppml.examples.tpch.Q12.scala Maven / Gradle / Ivy
The newest version!
// scalastyle:off
/*
* This file is copied from:
* https://github.com/ssavvides/tpch-spark/blob/master/src/main/scala/Q12.scala
*
* Copyright (c) 2015 Savvas Savvides, [email protected], [email protected]
*
* Licensed under the The MIT License:
*
* Permission is hereby granted, free of charge, to any person
* obtaining a copy of this software and associated documentation
* files (the "Software"), to deal in the Software without
* restriction, including without limitation the rights to use,
* copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the
* Software is furnished to do so, subject to the following
* conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
* OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
* HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
* WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
* OTHER DEALINGS IN THE SOFTWARE.
*/
// scalastyle:on
package com.intel.analytics.bigdl.ppml.examples.tpch
import org.apache.spark.sql.DataFrame
import org.apache.spark.SparkContext
import org.apache.spark.sql.functions.sum
import org.apache.spark.sql.functions.udf
import com.intel.analytics.bigdl.ppml.PPMLContext
/**
* TPC-H Query 12
* Savvas Savvides
*
*/
class Q12 extends TpchQuery {
override def execute(sc: PPMLContext, schemaProvider: TpchSchemaProvider): DataFrame = {
// this is used to implicitly convert an RDD to a DataFrame.
val sqlContext = sc.getSparkSession.sqlContext
import sqlContext.implicits._
import schemaProvider._
val mul = udf { (x: Double, y: Double) => x * y }
val highPriority = udf { (x: String) => if (x == "1-URGENT" || x == "2-HIGH") 1 else 0 }
val lowPriority = udf { (x: String) => if (x != "1-URGENT" && x != "2-HIGH") 1 else 0 }
lineitem.filter((
$"l_shipmode" === "MAIL" || $"l_shipmode" === "SHIP") &&
$"l_commitdate" < $"l_receiptdate" &&
$"l_shipdate" < $"l_commitdate" &&
$"l_receiptdate" >= "1994-01-01" && $"l_receiptdate" < "1995-01-01")
.join(order, $"l_orderkey" === order("o_orderkey"))
.select($"l_shipmode", $"o_orderpriority")
.groupBy($"l_shipmode")
.agg(sum(highPriority($"o_orderpriority")).as("sum_highorderpriority"),
sum(lowPriority($"o_orderpriority")).as("sum_loworderpriority"))
.sort($"l_shipmode")
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy