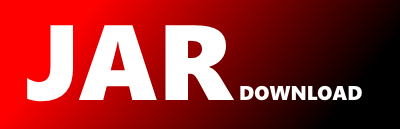
org.apache.arrow.vector.VectorSchemaRoot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arrow-vector Show documentation
Show all versions of arrow-vector Show documentation
An off-heap reference implementation for Arrow columnar data format.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.arrow.vector;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.StreamSupport;
import org.apache.arrow.memory.BufferAllocator;
import org.apache.arrow.util.AutoCloseables;
import org.apache.arrow.util.Preconditions;
import org.apache.arrow.vector.compare.ApproxEqualsVisitor;
import org.apache.arrow.vector.compare.Range;
import org.apache.arrow.vector.compare.VectorEqualsVisitor;
import org.apache.arrow.vector.compare.VectorValueEqualizer;
import org.apache.arrow.vector.compare.util.ValueEpsilonEqualizers;
import org.apache.arrow.vector.types.pojo.Field;
import org.apache.arrow.vector.types.pojo.Schema;
import org.apache.arrow.vector.util.TransferPair;
/**
* Holder for a set of vectors to be loaded/unloaded.
* A VectorSchemaRoot is a container that can hold batches, batches flow through VectorSchemaRoot
* as part of a pipeline. Note this is different from other implementations (i.e. in C++ and Python,
* a RecordBatch is a collection of equal-length vector instances and was created each time for a new batch).
* The recommended usage for VectorSchemaRoot is creating a single VectorSchemaRoot based on the known
* schema and populated data over and over into the same VectorSchemaRoot in a stream of batches rather
* than create a new VectorSchemaRoot instance each time (see Flight or ArrowFileWriter for better understanding).
* Thus at any one point a VectorSchemaRoot may have data or may have no data (say it was transferred downstream
* or not yet populated).
*/
public class VectorSchemaRoot implements AutoCloseable {
private Schema schema;
private int rowCount;
private final List fieldVectors;
private final Map fieldVectorsMap = new HashMap<>();
/**
* Constructs new instance containing each of the vectors.
*/
public VectorSchemaRoot(Iterable vectors) {
this(
StreamSupport.stream(vectors.spliterator(), false).map(t -> t.getField()).collect(Collectors.toList()),
StreamSupport.stream(vectors.spliterator(), false).collect(Collectors.toList())
);
}
/**
* Constructs a new instance containing the children of parent but not the parent itself.
*/
public VectorSchemaRoot(FieldVector parent) {
this(parent.getField().getChildren(), parent.getChildrenFromFields(), parent.getValueCount());
}
/**
* Constructs a new instance.
*
* @param fields The types of each vector.
* @param fieldVectors The data vectors (must be equal in size to fields
.
*/
public VectorSchemaRoot(List fields, List fieldVectors) {
this(new Schema(fields), fieldVectors, fieldVectors.size() == 0 ? 0 : fieldVectors.get(0).getValueCount());
}
/**
* Constructs a new instance.
*
* @param fields The types of each vector.
* @param fieldVectors The data vectors (must be equal in size to fields
.
* @param rowCount The number of rows contained.
*/
public VectorSchemaRoot(List fields, List fieldVectors, int rowCount) {
this(new Schema(fields), fieldVectors, rowCount);
}
/**
* Constructs a new instance.
*
* @param schema The schema for the vectors.
* @param fieldVectors The data vectors.
* @param rowCount The number of rows
*/
public VectorSchemaRoot(Schema schema, List fieldVectors, int rowCount) {
if (schema.getFields().size() != fieldVectors.size()) {
throw new IllegalArgumentException("Fields must match field vectors. Found " +
fieldVectors.size() + " vectors and " + schema.getFields().size() + " fields");
}
this.schema = schema;
this.rowCount = rowCount;
this.fieldVectors = fieldVectors;
for (int i = 0; i < schema.getFields().size(); ++i) {
Field field = schema.getFields().get(i);
FieldVector vector = fieldVectors.get(i);
fieldVectorsMap.put(field.getName(), vector);
}
}
/**
* Creates a new set of empty vectors corresponding to the given schema.
*/
public static VectorSchemaRoot create(Schema schema, BufferAllocator allocator) {
List fieldVectors = new ArrayList<>();
for (Field field : schema.getFields()) {
FieldVector vector = field.createVector(allocator);
fieldVectors.add(vector);
}
if (fieldVectors.size() != schema.getFields().size()) {
throw new IllegalArgumentException("The root vector did not create the right number of children. found " +
fieldVectors.size() + " expected " + schema.getFields().size());
}
return new VectorSchemaRoot(schema, fieldVectors, 0);
}
/** Constructs a new instance from vectors. */
public static VectorSchemaRoot of(FieldVector... vectors) {
return new VectorSchemaRoot(Arrays.stream(vectors).collect(Collectors.toList()));
}
/**
* Do an adaptive allocation of each vector for memory purposes. Sizes will be based on previously
* defined initial allocation for each vector (and subsequent size learned).
*/
public void allocateNew() {
for (FieldVector v : fieldVectors) {
v.allocateNew();
}
rowCount = 0;
}
/**
* Release all the memory for each vector held in this root. This DOES NOT remove vectors from the container.
*/
public void clear() {
for (FieldVector v : fieldVectors) {
v.clear();
}
rowCount = 0;
}
public List getFieldVectors() {
return fieldVectors.stream().collect(Collectors.toList());
}
public FieldVector getVector(String name) {
return fieldVectorsMap.get(name);
}
public FieldVector getVector(int index) {
Preconditions.checkArgument(index >= 0 && index < fieldVectors.size());
return fieldVectors.get(index);
}
/**
* Add vector to the record batch, producing a new VectorSchemaRoot.
* @param index field index
* @param vector vector to be added.
* @return out VectorSchemaRoot with vector added
*/
public VectorSchemaRoot addVector(int index, FieldVector vector) {
Preconditions.checkNotNull(vector);
Preconditions.checkArgument(index >= 0 && index < fieldVectors.size());
List newVectors = new ArrayList<>();
for (int i = 0; i < fieldVectors.size(); i++) {
if (i == index) {
newVectors.add(vector);
}
newVectors.add(fieldVectors.get(i));
}
return new VectorSchemaRoot(newVectors);
}
/**
* Remove vector from the record batch, producing a new VectorSchemaRoot.
* @param index field index
* @return out VectorSchemaRoot with vector removed
*/
public VectorSchemaRoot removeVector(int index) {
Preconditions.checkArgument(index >= 0 && index < fieldVectors.size());
List newVectors = new ArrayList<>();
for (int i = 0; i < fieldVectors.size(); i++) {
if (i != index) {
newVectors.add(fieldVectors.get(i));
}
}
return new VectorSchemaRoot(newVectors);
}
public Schema getSchema() {
return schema;
}
public int getRowCount() {
return rowCount;
}
public void setRootRowCount(int rowCount) {
this.rowCount = rowCount;
}
/**
* Set the row count of all the vectors in this container. Also sets the value
* count for each root level contained FieldVector.
* @param rowCount Number of records.
*/
public void setRowCount(int rowCount) {
this.rowCount = rowCount;
for (FieldVector v : getFieldVectors()) {
v.setValueCount(rowCount);
}
}
@Override
public void close() {
try {
AutoCloseables.close(fieldVectors);
} catch (RuntimeException ex) {
throw ex;
} catch (Exception ex) {
// should never happen since FieldVector.close() doesn't throw IOException
throw new RuntimeException(ex);
}
}
private void printRow(StringBuilder sb, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy