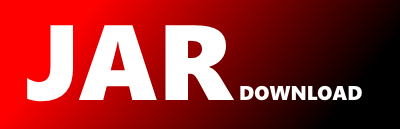
com.intel.gkl.IntelGKLUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of intel-gkl Show documentation
Show all versions of intel-gkl Show documentation
Genomics compute kernels optimized for Intel Architecture
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy