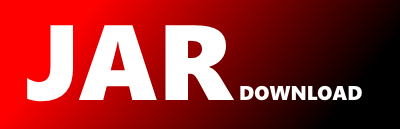
com.inteligr8.activiti.api.ExecutionApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aps-public-rest-api Show documentation
Show all versions of aps-public-rest-api Show documentation
An APS API library for building REST API clients that support both the CXF and Jersey frameworks
/*
* This program is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or (at your
* option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for
* more details.
*
* You should have received a copy of the GNU General Public License along
* with this program. If not, see .
*/
package com.inteligr8.activiti.api;
import java.util.List;
import jakarta.ws.rs.Consumes;
import jakarta.ws.rs.GET;
import jakarta.ws.rs.POST;
import jakarta.ws.rs.PUT;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.PathParam;
import jakarta.ws.rs.Produces;
import jakarta.ws.rs.QueryParam;
import jakarta.ws.rs.core.MediaType;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.inteligr8.activiti.model.ProcessInstanceAction;
import com.inteligr8.activiti.model.ProcessInstanceAction.ActionValue;
import com.inteligr8.activiti.model.Execution;
import com.inteligr8.activiti.model.ResultList;
import com.inteligr8.activiti.model.SignalEventAction;
import com.inteligr8.activiti.model.SortOrder;
import com.inteligr8.activiti.model.Variable;
@Path("/api/runtime/executions")
public interface ExecutionApi {
public enum Sort {
@JsonProperty("id")
ProcessInstanceId,
@JsonProperty("processDefinitionId")
ProcessDefinitionId,
@JsonProperty("tenantId")
TenantId,
@JsonProperty("processDefinitionKey")
ProcessDefinitionKey;
}
public enum VariableScope {
@JsonProperty("local")
Local,
@JsonProperty("global")
Global,
}
@GET
@Path("{executionId}")
@Produces({ MediaType.APPLICATION_JSON })
Execution get(
@PathParam("executionId") String executionId);
@GET
@Path("{executionId}/activities")
@Produces({ MediaType.APPLICATION_JSON })
List getActiveActivities(
@PathParam("executionId") String executionId);
default Execution signal(
String processInstanceId,
List variables) {
ProcessInstanceAction action = new ProcessInstanceAction(ActionValue.Signal);
if (variables != null && !variables.isEmpty())
action.setVariables(variables);
return this.execute(processInstanceId, action);
}
default Execution signalReceived(
String processInstanceId,
String signal,
List variables) {
ProcessInstanceAction action = new SignalEventAction(ActionValue.SignalReceived, signal);
if (variables != null && !variables.isEmpty())
action.setVariables(variables);
return this.execute(processInstanceId, action);
}
default Execution messageReceived(
String processInstanceId,
String message,
List variables) {
ProcessInstanceAction action = new SignalEventAction(ActionValue.MessageReceived, message);
if (variables != null && !variables.isEmpty())
action.setVariables(variables);
return this.execute(processInstanceId, action);
}
@PUT
@Path("{executionId}")
@Consumes({ MediaType.APPLICATION_JSON })
@Produces({ MediaType.APPLICATION_JSON })
Execution execute(
@PathParam("executionId") String executionId,
ProcessInstanceAction action);
default ResultList getWithoutTenant(
String executionId,
String activityId,
String processDefinitionKey,
String processDefinitionId,
String processInstanceId,
String messageEventSubscriptionName,
String signalEventSubscriptionName,
String parentId,
Sort sort,
SortOrder sortOrder,
Integer pageStart,
Integer pageSize) {
return this.getByAny(executionId, activityId, processDefinitionKey, processDefinitionId, processInstanceId,
messageEventSubscriptionName, signalEventSubscriptionName, parentId, null, null, true,
sort, sortOrder, pageStart, pageSize);
}
default ResultList getByTenant(
String executionId,
String activityId,
String processDefinitionKey,
String processDefinitionId,
String processInstanceId,
String messageEventSubscriptionName,
String signalEventSubscriptionName,
String parentId,
String tenantId,
Sort sort,
SortOrder sortOrder,
Integer pageStart,
Integer pageSize) {
return this.getByAny(executionId, activityId, processDefinitionKey, processDefinitionId, processInstanceId,
messageEventSubscriptionName, signalEventSubscriptionName, parentId, tenantId, null, false,
sort, sortOrder, pageStart, pageSize);
}
default ResultList getByTenants(
String executionId,
String activityId,
String processDefinitionKey,
String processDefinitionId,
String processInstanceId,
String messageEventSubscriptionName,
String signalEventSubscriptionName,
String parentId,
String tenantIdLike,
Sort sort,
SortOrder sortOrder,
Integer pageStart,
Integer pageSize) {
return this.getByAny(executionId, activityId, processDefinitionKey, processDefinitionId, processInstanceId,
messageEventSubscriptionName, signalEventSubscriptionName, parentId, null, tenantIdLike, false,
sort, sortOrder, pageStart, pageSize);
}
@GET
@Produces({ MediaType.APPLICATION_JSON })
ResultList getByAny(
@QueryParam("id") String executionId,
@QueryParam("activityId") String activityId,
@QueryParam("processDefinitionKey") String processDefinitionKey,
@QueryParam("processDefinitionId") String processDefinitionId,
@QueryParam("processInstanceId") String processInstanceId,
@QueryParam("messageEventSubscriptionName") String messageEventSubscriptionName,
@QueryParam("signalEventSubscriptionName") String signalEventSubscriptionName,
@QueryParam("parentId") String parentId,
@QueryParam("tenantId") String tenantId,
@QueryParam("tenantIdLike") String tenantIdLike,
@QueryParam("withoutTenantId") Boolean withoutTenantId,
@QueryParam("sort") Sort sort,
@QueryParam("order") SortOrder sortOrder,
@QueryParam("start") Integer pageStart,
@QueryParam("size") Integer pageSize);
@GET
@Path("{executionId}/variables")
@Produces({ MediaType.APPLICATION_JSON })
List getVariables(
@PathParam("executionId") String executionId,
@QueryParam("scope") VariableScope scope);
@GET
@Path("{executionId}/variables/{variableName}")
@Produces({ MediaType.APPLICATION_JSON })
Variable getVariable(
@PathParam("executionId") String executionId,
@PathParam("variableName") String variableName);
@POST
@Path("{executionId}/variables")
@Consumes({ MediaType.APPLICATION_JSON })
void createVariables(
@PathParam("executionId") String executionId,
List variables);
@PUT
@Path("{executionId}/variables")
@Consumes({ MediaType.APPLICATION_JSON })
void updateVariables(
@PathParam("executionId") String executionId,
List variables);
default void updateVariable(
String executionId,
Variable variable) {
this.updateVariable(executionId, variable.getName(), variable);
}
@PUT
@Path("{executionId}/variables/{variableName}")
@Consumes({ MediaType.APPLICATION_JSON })
void updateVariable(
@PathParam("executionId") String executionId,
@PathParam("variableName") String variableName,
Variable variable);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy