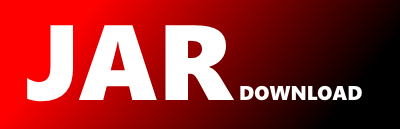
com.inteligr8.activiti.api.ProcessDefinitionApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aps-public-rest-api Show documentation
Show all versions of aps-public-rest-api Show documentation
An APS API library for building REST API clients that support both the CXF and Jersey frameworks
/*
* This program is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or (at your
* option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for
* more details.
*
* You should have received a copy of the GNU General Public License along
* with this program. If not, see .
*/
package com.inteligr8.activiti.api;
import com.inteligr8.activiti.api.ProcessInstanceApi.Sort;
import com.inteligr8.activiti.model.ProcessDefinitionAction;
import com.inteligr8.activiti.model.ResultList;
import com.inteligr8.activiti.model.SortOrder;
import com.inteligr8.activiti.model.ProcessDefinitionAction.ActionValue;
import com.inteligr8.alfresco.activiti.model.ProcessDefinition;
import com.inteligr8.alfresco.activiti.model.ProcessInstance;
import jakarta.ws.rs.Consumes;
import jakarta.ws.rs.GET;
import jakarta.ws.rs.PUT;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.PathParam;
import jakarta.ws.rs.Produces;
import jakarta.ws.rs.QueryParam;
import jakarta.ws.rs.core.MediaType;
@Path("/api/repository/process-definitions")
public interface ProcessDefinitionApi {
@GET
@Path("{processDefinitionId}")
@Produces({ MediaType.APPLICATION_JSON })
ProcessDefinition get(
@PathParam("processDefinitionId") String processDefinitionId);
default void activate(
String processDefinitionId) {
this.act(processDefinitionId, new ProcessDefinitionAction(ActionValue.Activate));
}
default void suspend(
String processDefinitionId) {
this.act(processDefinitionId, new ProcessDefinitionAction(ActionValue.Suspend));
}
@PUT
@Path("{processDefinitionId}")
@Consumes({ MediaType.APPLICATION_JSON })
void act(
@PathParam("processDefinitionId") String processDefinitionId,
ProcessDefinitionAction action);
default ResultList getByTenant(
String category,
String categoryLike,
String categoryNotEquals,
String key,
String keyLike,
String name,
String nameLike,
String resourceName,
String resourceNameLike,
String version,
Boolean suspended,
boolean latest,
String deploymentId,
String startableByUser,
String tenantId,
Sort sort,
SortOrder sortOrder,
Integer pageStart,
Integer pageSize) {
return this.getByAny(category, categoryLike, categoryNotEquals, key, keyLike,
name, nameLike, resourceName, resourceNameLike, version,
suspended, latest, deploymentId, startableByUser,
tenantId, null, sort, sortOrder, pageStart, pageSize);
}
default ResultList getByTenants(
String category,
String categoryLike,
String categoryNotEquals,
String key,
String keyLike,
String name,
String nameLike,
String resourceName,
String resourceNameLike,
String version,
Boolean suspended,
boolean latest,
String deploymentId,
String startableByUser,
String tenantIdLike,
Sort sort,
SortOrder sortOrder,
Integer pageStart,
Integer pageSize) {
return this.getByAny(category, categoryLike, categoryNotEquals, key, keyLike,
name, nameLike, resourceName, resourceNameLike, version,
suspended, latest, deploymentId, startableByUser,
null, tenantIdLike, sort, sortOrder, pageStart, pageSize);
}
@GET
@Produces({ MediaType.APPLICATION_JSON })
public ResultList getByAny(
@QueryParam("category") String category,
@QueryParam("categoryLike") String categoryLike,
@QueryParam("categoryNotEquals") String categoryNotEquals,
@QueryParam("key") String key,
@QueryParam("keyLike") String keyLike,
@QueryParam("name") String name,
@QueryParam("nameLike") String nameLike,
@QueryParam("resourceName") String resourceName,
@QueryParam("resourceNameLike") String resourceNameLike,
@QueryParam("version") String version,
@QueryParam("suspended") Boolean suspended,
@QueryParam("latest") Boolean latest,
@QueryParam("deploymentId") String deploymentId,
@QueryParam("startableByUser") String startableByUser,
@QueryParam("tenantId") String tenantId,
@QueryParam("tenantIdLike") String tenantIdLike,
@QueryParam("sort") Sort sort,
@QueryParam("order") SortOrder sortOrder,
@QueryParam("start") Integer pageStart,
@QueryParam("size") Integer pageSize);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy