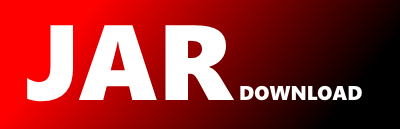
com.inteligr8.alfresco.activiti.model.ModelRepresentation Maven / Gradle / Ivy
Show all versions of aps-public-rest-api Show documentation
package com.inteligr8.alfresco.activiti.model;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonFormat.Shape;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* ModelRepresentation
*
*
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"comment",
"createdBy",
"createdByFullName",
"description",
"favorite",
"id",
"lastUpdated",
"lastUpdatedBy",
"lastUpdatedByFullName",
"latestVersion",
"modelType",
"name",
"permission",
"referenceId",
"stencilSet",
"tenantId",
"version"
})
public class ModelRepresentation {
@JsonProperty("comment")
private String comment;
@JsonProperty("createdBy")
private Long createdBy;
@JsonProperty("createdByFullName")
private String createdByFullName;
@JsonProperty("description")
private String description;
@JsonProperty("favorite")
private Boolean favorite;
@JsonProperty("id")
private Long id;
@JsonProperty("lastUpdated")
@JsonFormat(shape = Shape.STRING, pattern = "yyyy-MM-dd'T'HH:mm:ss.SSSXX")
private OffsetDateTime lastUpdated;
@JsonProperty("lastUpdatedBy")
private Long lastUpdatedBy;
@JsonProperty("lastUpdatedByFullName")
private String lastUpdatedByFullName;
@JsonProperty("latestVersion")
private Boolean latestVersion;
@JsonProperty("modelType")
private Integer modelType;
@JsonProperty("name")
private String name;
@JsonProperty("permission")
private String permission;
@JsonProperty("referenceId")
private Long referenceId;
@JsonProperty("stencilSet")
private Long stencilSet;
@JsonProperty("tenantId")
private Long tenantId;
@JsonProperty("version")
private Integer version;
@JsonIgnore
private Map additionalProperties = new HashMap();
@JsonProperty("comment")
public String getComment() {
return comment;
}
@JsonProperty("comment")
public void setComment(String comment) {
this.comment = comment;
}
public ModelRepresentation withComment(String comment) {
this.comment = comment;
return this;
}
@JsonProperty("createdBy")
public Long getCreatedBy() {
return createdBy;
}
@JsonProperty("createdBy")
public void setCreatedBy(Long createdBy) {
this.createdBy = createdBy;
}
public ModelRepresentation withCreatedBy(Long createdBy) {
this.createdBy = createdBy;
return this;
}
@JsonProperty("createdByFullName")
public String getCreatedByFullName() {
return createdByFullName;
}
@JsonProperty("createdByFullName")
public void setCreatedByFullName(String createdByFullName) {
this.createdByFullName = createdByFullName;
}
public ModelRepresentation withCreatedByFullName(String createdByFullName) {
this.createdByFullName = createdByFullName;
return this;
}
@JsonProperty("description")
public String getDescription() {
return description;
}
@JsonProperty("description")
public void setDescription(String description) {
this.description = description;
}
public ModelRepresentation withDescription(String description) {
this.description = description;
return this;
}
@JsonProperty("favorite")
public Boolean getFavorite() {
return favorite;
}
@JsonProperty("favorite")
public void setFavorite(Boolean favorite) {
this.favorite = favorite;
}
public ModelRepresentation withFavorite(Boolean favorite) {
this.favorite = favorite;
return this;
}
@JsonProperty("id")
public Long getId() {
return id;
}
@JsonProperty("id")
public void setId(Long id) {
this.id = id;
}
public ModelRepresentation withId(Long id) {
this.id = id;
return this;
}
@JsonProperty("lastUpdated")
public OffsetDateTime getLastUpdated() {
return lastUpdated;
}
@JsonProperty("lastUpdated")
public void setLastUpdated(OffsetDateTime lastUpdated) {
this.lastUpdated = lastUpdated;
}
public ModelRepresentation withLastUpdated(OffsetDateTime lastUpdated) {
this.lastUpdated = lastUpdated;
return this;
}
@JsonProperty("lastUpdatedBy")
public Long getLastUpdatedBy() {
return lastUpdatedBy;
}
@JsonProperty("lastUpdatedBy")
public void setLastUpdatedBy(Long lastUpdatedBy) {
this.lastUpdatedBy = lastUpdatedBy;
}
public ModelRepresentation withLastUpdatedBy(Long lastUpdatedBy) {
this.lastUpdatedBy = lastUpdatedBy;
return this;
}
@JsonProperty("lastUpdatedByFullName")
public String getLastUpdatedByFullName() {
return lastUpdatedByFullName;
}
@JsonProperty("lastUpdatedByFullName")
public void setLastUpdatedByFullName(String lastUpdatedByFullName) {
this.lastUpdatedByFullName = lastUpdatedByFullName;
}
public ModelRepresentation withLastUpdatedByFullName(String lastUpdatedByFullName) {
this.lastUpdatedByFullName = lastUpdatedByFullName;
return this;
}
@JsonProperty("latestVersion")
public Boolean getLatestVersion() {
return latestVersion;
}
@JsonProperty("latestVersion")
public void setLatestVersion(Boolean latestVersion) {
this.latestVersion = latestVersion;
}
public ModelRepresentation withLatestVersion(Boolean latestVersion) {
this.latestVersion = latestVersion;
return this;
}
@JsonProperty("modelType")
public Integer getModelType() {
return modelType;
}
@JsonProperty("modelType")
public void setModelType(Integer modelType) {
this.modelType = modelType;
}
public ModelRepresentation withModelType(Integer modelType) {
this.modelType = modelType;
return this;
}
@JsonProperty("name")
public String getName() {
return name;
}
@JsonProperty("name")
public void setName(String name) {
this.name = name;
}
public ModelRepresentation withName(String name) {
this.name = name;
return this;
}
@JsonProperty("permission")
public String getPermission() {
return permission;
}
@JsonProperty("permission")
public void setPermission(String permission) {
this.permission = permission;
}
public ModelRepresentation withPermission(String permission) {
this.permission = permission;
return this;
}
@JsonProperty("referenceId")
public Long getReferenceId() {
return referenceId;
}
@JsonProperty("referenceId")
public void setReferenceId(Long referenceId) {
this.referenceId = referenceId;
}
public ModelRepresentation withReferenceId(Long referenceId) {
this.referenceId = referenceId;
return this;
}
@JsonProperty("stencilSet")
public Long getStencilSet() {
return stencilSet;
}
@JsonProperty("stencilSet")
public void setStencilSet(Long stencilSet) {
this.stencilSet = stencilSet;
}
public ModelRepresentation withStencilSet(Long stencilSet) {
this.stencilSet = stencilSet;
return this;
}
@JsonProperty("tenantId")
public Long getTenantId() {
return tenantId;
}
@JsonProperty("tenantId")
public void setTenantId(Long tenantId) {
this.tenantId = tenantId;
}
public ModelRepresentation withTenantId(Long tenantId) {
this.tenantId = tenantId;
return this;
}
@JsonProperty("version")
public Integer getVersion() {
return version;
}
@JsonProperty("version")
public void setVersion(Integer version) {
this.version = version;
}
public ModelRepresentation withVersion(Integer version) {
this.version = version;
return this;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public ModelRepresentation withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}