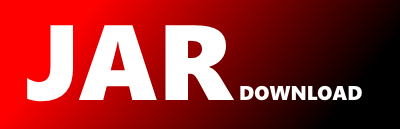
com.inteligr8.alfresco.activiti.model.User Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aps-public-rest-api Show documentation
Show all versions of aps-public-rest-api Show documentation
An APS API library for building REST API clients that support both the CXF and Jersey frameworks
package com.inteligr8.alfresco.activiti.model;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"apps",
"capabilities",
"company",
"created",
"email",
"externalId",
"firstName",
"fullname",
"groups",
"id",
"lastName",
"lastUpdate",
"latestSyncTimeStamp",
"password",
"pictureId",
"primaryGroup",
"status",
"tenantId",
"tenantName",
"tenantPictureId",
"type"
})
public class User {
@JsonProperty("apps")
private List apps = new ArrayList();
@JsonProperty("capabilities")
private List capabilities = new ArrayList();
@JsonProperty("company")
private String company;
@JsonProperty("created")
private String created;
@JsonProperty("email")
private String email;
@JsonProperty("externalId")
private String externalId;
@JsonProperty("firstName")
private String firstName;
@JsonProperty("fullname")
private String fullname;
@JsonProperty("groups")
private List groups = new ArrayList();
@JsonProperty("id")
private Long id;
@JsonProperty("lastName")
private String lastName;
@JsonProperty("lastUpdate")
private String lastUpdate;
@JsonProperty("latestSyncTimeStamp")
private String latestSyncTimeStamp;
@JsonProperty("password")
private String password;
@JsonProperty("pictureId")
private Long pictureId;
@JsonProperty("primaryGroup")
private Group primaryGroup;
@JsonProperty("status")
private String status;
@JsonProperty("tenantId")
private Long tenantId;
@JsonProperty("tenantName")
private String tenantName;
@JsonProperty("tenantPictureId")
private Long tenantPictureId;
@JsonProperty("type")
private String type;
/**
* No args constructor for use in serialization
*/
public User() {
}
public List getApps() {
return apps;
}
public void setApps(List apps) {
this.apps = apps;
}
public User withApps(List apps) {
this.apps = apps;
return this;
}
public List getCapabilities() {
return capabilities;
}
public void setCapabilities(List capabilities) {
this.capabilities = capabilities;
}
public User withCapabilities(List capabilities) {
this.capabilities = capabilities;
return this;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public User withCompany(String company) {
this.company = company;
return this;
}
public String getCreated() {
return created;
}
public void setCreated(String created) {
this.created = created;
}
public User withCreated(String created) {
this.created = created;
return this;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public User withEmail(String email) {
this.email = email;
return this;
}
public String getExternalId() {
return externalId;
}
public void setExternalId(String externalId) {
this.externalId = externalId;
}
public User withExternalId(String externalId) {
this.externalId = externalId;
return this;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public User withFirstName(String firstName) {
this.firstName = firstName;
return this;
}
public String getFullname() {
return fullname;
}
public void setFullname(String fullname) {
this.fullname = fullname;
}
public User withFullname(String fullname) {
this.fullname = fullname;
return this;
}
public List getGroups() {
return groups;
}
public void setGroups(List groups) {
this.groups = groups;
}
public User withGroups(List groups) {
this.groups = groups;
return this;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public User withId(Long id) {
this.id = id;
return this;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public User withLastName(String lastName) {
this.lastName = lastName;
return this;
}
public String getLastUpdate() {
return lastUpdate;
}
public void setLastUpdate(String lastUpdate) {
this.lastUpdate = lastUpdate;
}
public User withLastUpdate(String lastUpdate) {
this.lastUpdate = lastUpdate;
return this;
}
public String getLatestSyncTimeStamp() {
return latestSyncTimeStamp;
}
public void setLatestSyncTimeStamp(String latestSyncTimeStamp) {
this.latestSyncTimeStamp = latestSyncTimeStamp;
}
public User withLatestSyncTimeStamp(String latestSyncTimeStamp) {
this.latestSyncTimeStamp = latestSyncTimeStamp;
return this;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public User withPassword(String password) {
this.password = password;
return this;
}
public Long getPictureId() {
return pictureId;
}
public void setPictureId(Long pictureId) {
this.pictureId = pictureId;
}
public User withPictureId(Long pictureId) {
this.pictureId = pictureId;
return this;
}
public Group getPrimaryGroup() {
return primaryGroup;
}
public void setPrimaryGroup(Group primaryGroup) {
this.primaryGroup = primaryGroup;
}
public User withPrimaryGroup(Group primaryGroup) {
this.primaryGroup = primaryGroup;
return this;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public User withStatus(String status) {
this.status = status;
return this;
}
public Long getTenantId() {
return tenantId;
}
public void setTenantId(Long tenantId) {
this.tenantId = tenantId;
}
public User withTenantId(Long tenantId) {
this.tenantId = tenantId;
return this;
}
public String getTenantName() {
return tenantName;
}
public void setTenantName(String tenantName) {
this.tenantName = tenantName;
}
public User withTenantName(String tenantName) {
this.tenantName = tenantName;
return this;
}
public Long getTenantPictureId() {
return tenantPictureId;
}
public void setTenantPictureId(Long tenantPictureId) {
this.tenantPictureId = tenantPictureId;
}
public User withTenantPictureId(Long tenantPictureId) {
this.tenantPictureId = tenantPictureId;
return this;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public User withType(String type) {
this.type = type;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy