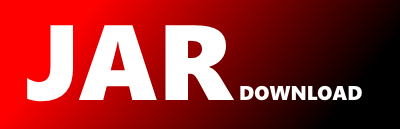
com.inteligr8.alfresco.activiti.model.UserLight Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aps-public-rest-api Show documentation
Show all versions of aps-public-rest-api Show documentation
An APS API library for building REST API clients that support both the CXF and Jersey frameworks
package com.inteligr8.alfresco.activiti.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"company",
"email",
"externalId",
"firstName",
"id",
"lastName",
"pictureId"
})
public class UserLight {
@JsonProperty("company")
private String company;
@JsonProperty("email")
private String email;
@JsonProperty("externalId")
private String externalId;
@JsonProperty("firstName")
private String firstName;
@JsonProperty("id")
private Long id;
@JsonProperty("lastName")
private String lastName;
@JsonProperty("pictureId")
private Long pictureId;
/**
* No args constructor for use in serialization
*/
public UserLight() {
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public UserLight withCompany(String company) {
this.setCompany(company);
return this;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public UserLight withEmail(String email) {
this.setEmail(email);
return this;
}
public String getExternalId() {
return externalId;
}
public void setExternalId(String externalId) {
this.externalId = externalId;
}
public UserLight withExternalId(String externalId) {
this.setExternalId(externalId);
return this;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public UserLight withFirstName(String firstName) {
this.setFirstName(firstName);
return this;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public UserLight withId(Long id) {
this.setId(id);
return this;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public UserLight withLastName(String lastName) {
this.setLastName(lastName);
return this;
}
public Long getPictureId() {
return pictureId;
}
public void setPictureId(Long pictureId) {
this.pictureId = pictureId;
}
public UserLight withPictureId(Long pictureId) {
this.setPictureId(pictureId);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy