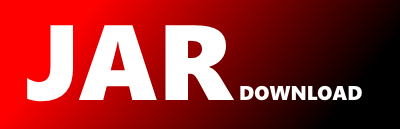
com.intellifylearning.Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of intellisense Show documentation
Show all versions of intellisense Show documentation
IntelliSense Sensor API for Java
package com.intellifylearning;
import java.util.List;
import org.apache.commons.lang.StringUtils;
import org.joda.time.DateTime;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.intellify.api.caliper.CaliperEntity;
import com.intellify.api.caliper.DescribeData;
import com.intellify.api.caliper.LearningEventData;
import com.intellify.api.caliper.impl.EntityData;
import com.intellify.api.caliper.impl.EventData;
import com.intellify.api.caliper.impl.IntellifyBase;
import com.intellifylearning.flush.Flusher;
import com.intellifylearning.flush.IBatchFactory;
import com.intellifylearning.metrics.events.CaliperEvent;
import com.intellifylearning.metrics.models.Agent;
import com.intellifylearning.metrics.models.DigitalResource;
import com.intellifylearning.models.Batch;
import com.intellifylearning.models.BatchIntellifyBase;
import com.intellifylearning.models.Callback;
import com.intellifylearning.request.BlockingRequester;
import com.intellifylearning.stats.IntelliSenseStatistics;
/**
* The IntelliSense Client - Instantiate this to use the IntelliSense API.
*
* The client is an HTTP wrapper over the IntelliSense REST API. It will allow
* you to conveniently consume the API without making any HTTP requests
* yourself.
*
* This client is also designed to be thread-safe and to not block each of your
* calls to make a HTTP request. It uses batching to efficiently send your
* requests on a separate resource-constrained thread pool.
*
*/
public final class Client {
private static final Logger logger = LoggerFactory
.getLogger(Constants.LOGGER);
private String apiKey;
private Options options;
private Flusher flusher;
private BlockingRequester requester;
private IntelliSenseStatistics statistics;
private Callback callback;
/**
* Creates a new IntelliSense client.
*
* The client is an HTTP wrapper over the IntelliSense REST API. It will
* allow you to conveniently consume the API without making any HTTP
* requests yourself.
*
* This client is also designed to be thread-safe and to not block each of
* your calls to make a HTTP request. It uses batching to efficiently send
* your requests on a separate resource-constrained thread pool.
*
*
* @param apiKey Your IntelliSense apiKey. You can get one of these by
* registering for a project at http://www.intellifylearning.com
*
*/
public Client(String apiKey) {
this(apiKey, new Options());
}
/**
* Creates a new IntelliSense client.
*
* The client is an HTTP wrapper over the IntelliSense REST API. It will
* allow you to conveniently consume the API without making any HTTP
* requests yourself.
*
* This client is also designed to be thread-safe and to not block each of
* your calls to make a HTTP request. It uses batching to efficiently send
* your requests on a separate resource-constrained thread pool.
*
*
* @param apiKey Your IntelliSense apiKey. You can get one of these by
* registering for a project at http://www.intellifylearning.com
*
* @param options Options to configure the behavior of the IntelliSense client
*
*
*/
public Client(String apiKey, Options options) {
String errorPrefix = "intellisense-java client must be initialized with a valid ";
if (StringUtils.isEmpty(apiKey)) {
throw new IllegalArgumentException(errorPrefix + "apiKey.");
}
if (options == null) {
throw new IllegalArgumentException(errorPrefix + "options.");
}
this.apiKey = apiKey;
this.options = options;
this.statistics = new IntelliSenseStatistics();
this.requester = new BlockingRequester(this);
this.flusher = new Flusher(this, factory, requester);
this.flusher.start();
Runtime.getRuntime().addShutdownHook(new Thread() {
@Override
public void run() {
flusher.flush();
}
});
logger.debug(
"Successfully initialized intellisense-java-client with apiKey = "
+ this.apiKey + " and host = " + this.options.getHost()
+ " and sensorId = " + this.options.getSensorId());
}
private IBatchFactory factory = new IBatchFactory() {
@Override
public Batch create(List batch) {
return new Batch(apiKey, batch);
}
@Override
public BatchIntellifyBase createIntellifyBaseBatch(
List batch) {
return new BatchIntellifyBase(apiKey, batch);
}
};
//
// API Calls
//
//
// Identify
//
public void describe(DigitalResource digitalResource) {
identify(digitalResource);
}
public void identify(DigitalResource digitalResource) {
DescribeData describeData = new DescribeData();
describeData.setEntityId(digitalResource.getId());
describeData.setProperties(digitalResource.getProperties());
describeData.setType(digitalResource.getType());
if (digitalResource.getLastModifiedAt() != 0L) {
describeData.setTimestamp(digitalResource.getLastModifiedAt());
} else {
describeData.setTimestamp(DateTime.now().getMillis());
}
addIntellifyMetadata(describeData);
flusher.enqueue(describeData);
logger.debug("Enqueued describe for DigitalResource: " + describeData);
statistics.updateIdentifies(1);
}
public void describe(Agent agent) {
identify(agent);
}
public void identify(Agent agent) {
DescribeData describeData = new DescribeData();
describeData.setEntityId(agent.getId());
describeData.setProperties(agent.getProperties());
describeData.setType(agent.getType());
if (agent.getLastModifiedAt() != 0L) {
describeData.setTimestamp(agent.getLastModifiedAt());
} else {
describeData.setTimestamp(DateTime.now().getMillis());
}
addIntellifyMetadata(describeData);
flusher.enqueue(describeData);
logger.debug("Enqueued describe for Agent: " + describeData);
statistics.updateIdentifies(1);
}
public void describe(
com.intellify.api.caliper.impl.entities.Entity entity) {
identify(entity);
}
public void identify(
com.intellify.api.caliper.impl.entities.Entity entity) {
EntityData entityData = new EntityData();
entityData.setEntity(entity);
entityData.setEntityId(entity.getId());
entityData.setType(entity.getType());
if (entity.getDateModified() != 0L) {
entityData.setTimestamp(entity.getDateModified());
} else {
entityData.setTimestamp(DateTime.now().getMillis());
}
entityData.setEntity(entity);
addIntellifyMetadata(entityData);
flusher.enqueue(entityData);
logger.debug("Enqueued describe for Entity: " + entityData);
statistics.updateIdentifies(1);
}
public void measure(CaliperEvent caliperEvent) {
LearningEventData event = new LearningEventData();
event.setAction(caliperEvent.getAction());
event.setActivityContext(caliperEvent.getActivityContext());
event.setLearningContext(caliperEvent.getLearningContext());
if (caliperEvent.getTimestamp() != 0L) {
event.setTimestamp(caliperEvent.getTimestamp());
} else {
event.setTimestamp(DateTime.now().getMillis());
}
addIntellifyMetadata(event);
flusher.enqueue(event);
logger.debug("Enqueued event : " + event);
statistics.updateTracks(1);
}
public void measure(com.intellify.api.caliper.impl.events.Event event) {
EventData eventData = new EventData();
eventData.setEvent(event);
if (event.getStartedAtTime() != 0L) {
eventData.setTimestamp(event.getStartedAtTime());
} else {
eventData.setTimestamp(DateTime.now().getMillis());
}
addIntellifyMetadata(eventData);
flusher.enqueue(eventData);
logger.debug("Enqueued event data : " + eventData);
statistics.updateTracks(1);
}
private void addIntellifyMetadata(IntellifyBase intellifyBaseObj) {
intellifyBaseObj.setApiKey(getapiKey());
intellifyBaseObj.setSensorId(getOptions().getSensorId());
intellifyBaseObj.setCorrelationId(getOptions().getCorrelationId());
}
private void addIntellifyMetadata(CaliperEntity caliperEntity) {
caliperEntity.setApiKey(getapiKey());
caliperEntity.setIl_sensorId(getOptions().getSensorId());
caliperEntity.setIl_correlationId(getOptions().getCorrelationId());
}
//
// Actions
//
/**
* Blocks until all messages in the queue are flushed.
*/
public void flush() {
this.flusher.flush();
}
/**
* Closes the queue and the threads associated with flushing the queue
*/
public void close() {
this.flusher.close();
this.requester.close();
}
//
// Getters and Setters
//
public String getapiKey() {
return apiKey;
}
public void setapiKey(String apiKey) {
this.apiKey = apiKey;
}
public Options getOptions() {
return options;
}
public IntelliSenseStatistics getStatistics() {
return statistics;
}
protected void registerCallback(Callback callback) {
this.callback = callback;
}
public void notifyEntitySend(boolean success,
com.intellify.api.caliper.impl.entities.Entity entity) {
this.callback.onResponse(success, entity);
}
public void notifyEventSend(boolean success,
com.intellify.api.caliper.impl.events.Event event) {
this.callback.onResponse(success, event);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy