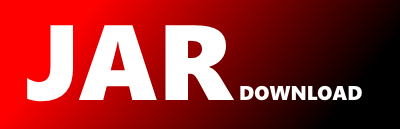
com.intellifylearning.Options Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of intellisense Show documentation
Show all versions of intellisense Show documentation
IntelliSense Sensor API for Java
package com.intellifylearning;
import org.apache.commons.lang.StringUtils;
/**
* IntelliSense client options
*
*/
public class Options {
/**
* The REST API endpoint (with scheme)
*/
private String host;
/**
* Stop accepting messages after the queue reaches this capacity
*/
private int maxQueueSize;
/**
* If true, an IntellifyQueueFullException is thrown immediately when any message is put to a
* full queue
*/
private boolean errorOnQueueFull;
/**
* Time in milliseconds to block on a full queue before throwing an
* IntellifyQueueFullTimeoutException
*/
private int queueWaitTimeout;
/**
* Specify batch size
*/
private int batchSize = 0;
/**
* The amount of milliseconds that passes before a request is marked as
* timed out
*/
private int timeout;
/**
* Intellify metadata - sensorId representing this instance of the sensor
*/
private String sensorId;
/**
* Intellify metadata - correlationId - used to correlate data payloads
* between sensor and intellistore
*/
private String correlationId;
/**
* Enable in test mode - echos output to request bin
*/
private boolean enableTestMode;
/**
* Creates a default options
*/
public Options() {
this(Defaults.HOST, Defaults.MAX_QUEUE_SIZE, Defaults.QUEUE_WAIT_TIMEOUT, Defaults.CONNECTION_TIMEOUT);
}
/**
* Creates an option with the provided settings
*/
Options(String host, int maxQueueSize, int queueWaitTimeout, int timeout) {
setHost(host);
setMaxQueueSize(maxQueueSize);
setQueueWaitTimeout(queueWaitTimeout);
setTimeout(timeout);
}
public String getHost() {
return host;
}
public int getMaxQueueSize() {
return maxQueueSize;
}
public int getTimeout() {
return timeout;
}
public int getQueueWaitTimeout() {
return queueWaitTimeout;
}
public boolean isErrorOnQueueFull() {
return errorOnQueueFull;
}
/**
* Sets the maximum queue capacity, which is an emergency pressure relief
* valve. If we're unable to flush messages fast enough, the queue will stop
* accepting messages after this capacity is reached.
*
* @param maxQueueSize queue size
* @return this for chaining
*/
public Options setMaxQueueSize(int maxQueueSize) {
if (maxQueueSize < 1) {
throw new IllegalArgumentException(
"IntellifyBase#option#maxQueueSize must be greater than 0.");
}
this.maxQueueSize = maxQueueSize;
return this;
}
/**
* Sets the REST API endpoint
*
* @param host host
* @return this for chaining
*/
public Options setHost(String host) {
if (StringUtils.isEmpty(host)) {
throw new IllegalArgumentException(
"IntellifyBase#option#host must be a valid host, like 'https://api.IntelliSense'.");
}
this.host = host;
return this;
}
/**
* Sets the milliseconds to wait before a flush is marked as timed out.
*
* @param timeout timeout in milliseconds.
* @return this for chaining
*/
public Options setTimeout(int timeout) {
if (timeout < 1000) {
throw new IllegalArgumentException(
"IntellifyBase#option#timeout must be at least 1000 milliseconds.");
}
this.timeout = timeout;
return this;
}
public Options setQueueWaitTimeout(int timeout) {
if (timeout < 0) {
throw new IllegalArgumentException("IntellifyBase#option#queueWaitTimeout must be greater than 0.");
}
this.queueWaitTimeout = timeout;
return this;
}
public Options setErrorOnQueueFull(boolean errorOnQueueFull) {
this.errorOnQueueFull = errorOnQueueFull;
return this;
}
/**
* @return the sensorId
*/
public String getSensorId() {
return sensorId;
}
/**
* @param sensorId the sensorId to set
*/
public void setSensorId(String sensorId) {
this.sensorId = sensorId;
}
/**
* @return the correlationId
*/
public String getCorrelationId() {
return correlationId;
}
/**
* @param correlationId the correlationId to set
*/
public void setCorrelationId(String correlationId) {
this.correlationId = correlationId;
}
/**
* @return the enableTestMode
*/
public boolean isEnableTestMode() {
return enableTestMode;
}
/**
* @param enableTestMode the enableTestMode to set
*/
public void setEnableTestMode(boolean enableTestMode) {
this.enableTestMode = enableTestMode;
}
/**
* @return the batchSize
*/
public int getBatchSize() {
return batchSize;
}
/**
* @param batchSize the batchSize to set
*/
public void setBatchSize(int batchSize) {
this.batchSize = batchSize;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy