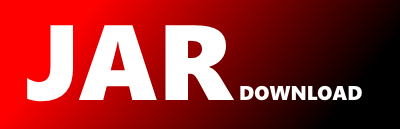
com.intellifylearning.request.BlockingRequester Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of intellisense Show documentation
Show all versions of intellisense Show documentation
IntelliSense Sensor API for Java
package com.intellifylearning.request;
import java.io.Closeable;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import org.apache.http.HttpResponse;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.ByteArrayEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.util.EntityUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.fasterxml.jackson.core.JsonGenerationException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.collect.Iterables;
import com.intellify.api.caliper.BatchDescribes;
import com.intellify.api.caliper.BatchEvents;
import com.intellify.api.caliper.CaliperEntity;
import com.intellify.api.caliper.DescribeData;
import com.intellify.api.caliper.LearningEventData;
import com.intellify.api.caliper.impl.BatchEntityData;
import com.intellify.api.caliper.impl.BatchEventData;
import com.intellify.api.caliper.impl.EntityData;
import com.intellify.api.caliper.impl.EventData;
import com.intellify.api.caliper.impl.IntellifyBase;
import com.intellifylearning.Client;
import com.intellifylearning.Constants;
import com.intellifylearning.models.Batch;
import com.intellifylearning.models.BatchIntellifyBase;
public class BlockingRequester implements IRequester, Closeable {
private static final Logger logger = LoggerFactory
.getLogger(Constants.LOGGER);
private static final String describeURI = "/v1/describe/batch";
private static final String learningEventURI = "/v1/learningevent/batch";
private static final String entityDataURI = "/v1custom/entitydata/batch";
private static final String eventDataURI = "/v1custom/eventdata/batch";
private final Client client;
private final CloseableHttpClient httpClient;
public BlockingRequester(Client client) {
this.client = client;
if (client.getOptions().isEnableTestMode()) {
logger.info("#### RUNNING IN TEST MODE");
}
int requestTimeout = client.getOptions().getTimeout();
RequestConfig requestConfig = RequestConfig.custom()
.setStaleConnectionCheckEnabled(true)
.setConnectTimeout(requestTimeout)
.setSocketTimeout(requestTimeout)
.setConnectionRequestTimeout(requestTimeout).build();
this.httpClient = HttpClientBuilder.create()
.setDefaultRequestConfig(requestConfig).build();
}
@Override
public void send(Batch batch) {
logger.debug(
"############ Sending describes/events in batch of size ... "
+ batch.getBatch().size());
BatchDescribes batchDescribes = getDescribes(batch);
BatchEvents batchEvents = getEvents(batch);
if (!Iterables.isEmpty(batchDescribes.getDescribes())) {
logger.debug(
"Sending " + Iterables.size(batchDescribes.getDescribes())
+ " describes");
String json = jacksonJson(batchDescribes);
sendBatch(json, describeURI, batchDescribes);
} else {
logger.debug("No describes found in batch...");
}
if (!Iterables.isEmpty(batchEvents.getEvents())) {
logger.debug("Sending " + Iterables.size(batchEvents.getEvents())
+ " events");
String json = jacksonJson(batchEvents);
sendBatch(json, learningEventURI, batchEvents);
} else {
logger.debug("No events found in batch...");
}
}
@Override
public void sendIntellifyBaseBatch(BatchIntellifyBase batch) {
logger.debug(
"############ Sending describes/events in batch of size ... "
+ batch.getBatch().size());
BatchEntityData batchEntityData = getBatchEntityData(batch);
BatchEventData batchEventData = getBatchEventData(batch);
if (!Iterables.isEmpty(batchEntityData.getEntityData())) {
logger.debug(
"Sending " + Iterables.size(batchEntityData.getEntityData())
+ " entity data objects");
String json = jacksonJson(batchEntityData);
sendBatch(json, entityDataURI, batchEntityData);
} else {
logger.debug("No entity data objects found in batch...");
}
if (!Iterables.isEmpty(batchEventData.getEventData())) {
logger.debug(
"Sending " + Iterables.size(batchEventData.getEventData())
+ " events");
String json = jacksonJson(batchEventData);
sendBatch(json, eventDataURI, batchEventData);
} else {
logger.debug("No event data objects found in batch...");
}
}
private void sendBatch(String json, String uri,
BatchDescribes batchDescribes) {
if (sendBatch(json, uri)) {
reportWithoutCallback(batchDescribes, true);
} else {
reportWithoutCallback(batchDescribes, false);
}
}
private void sendBatch(String json, String uri, BatchEvents batchEvents) {
if (sendBatch(json, uri)) {
reportWithoutCallback(batchEvents, true);
} else {
reportWithoutCallback(batchEvents, false);
}
}
private void sendBatch(String json, String uri,
BatchEntityData batchEntityData) {
if (sendBatch(json, uri)) {
reportWithoutCallback(batchEntityData, true);
} else {
reportWithoutCallback(batchEntityData, false);
}
}
private void sendBatch(String json, String uri,
BatchEventData batchEventData) {
if (sendBatch(json, uri)) {
reportWithoutCallback(batchEventData, true);
} else {
reportWithoutCallback(batchEventData, false);
}
}
private boolean sendBatch(String json, String uri) {
boolean successful = false;
try {
long start = System.currentTimeMillis();
HttpPut put = new HttpPut(client.getOptions().getHost() + uri);
put.addHeader("Content-Type", "application/json; charset=utf-8");
ByteArrayEntity baEntity = new ByteArrayEntity(
json.getBytes("UTF-8"));
put.setEntity(baEntity);
logger.debug("About to send request - " + put.toString());
logger.debug("entity in request - " + json);
logger.debug("ACTUAL entity in request - " + baEntity.toString());
HttpResponse response = null;
try {
response = httpClient.execute(put);
successful = processResponse(response);
long duration = System.currentTimeMillis() - start;
client.getStatistics().updateRequestTime(duration);
} finally {
if (response != null) {
EntityUtils.consumeQuietly(response.getEntity());
}
}
} catch (IOException e) {
String message = "Failed intellisense response." + e.getMessage();
logger.error(message, e);
return false;
}
return successful;
}
private boolean processResponse(HttpResponse response)
throws IllegalStateException, IOException {
String responseBody = EntityUtils.toString(response.getEntity(), StandardCharsets.UTF_8);
;
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == 200 || statusCode == 201) {
String message = "Successful intellisense request. [code = "
+ statusCode + "]. Response = " + responseBody;
logger.debug(message);
return true;
} else {
String message = "Failed intellisense response [code = "
+ statusCode + "]. Response = " + responseBody;
logger.error(message);
return false;
}
}
private BatchDescribes getDescribes(Batch batch) {
BatchDescribes batchDescribes = new BatchDescribes();
for (CaliperEntity entity : batch.getBatch()) {
if (entity instanceof DescribeData) {
batchDescribes.getDescribes().add((DescribeData) entity);
}
}
return batchDescribes;
}
private BatchEvents getEvents(Batch batch) {
BatchEvents batchEvents = new BatchEvents();
for (CaliperEntity entity : batch.getBatch()) {
if (entity instanceof LearningEventData) {
batchEvents.getEvents().add((LearningEventData) entity);
}
}
return batchEvents;
}
private BatchEntityData getBatchEntityData(
BatchIntellifyBase batchIntellifyBase) {
BatchEntityData batchEntityData = new BatchEntityData();
for (IntellifyBase entity : batchIntellifyBase.getBatch()) {
if (entity instanceof EntityData) {
batchEntityData.getEntityData().add((EntityData) entity);
}
}
return batchEntityData;
}
private BatchEventData getBatchEventData(
BatchIntellifyBase batchIntellifyBase) {
BatchEventData batchEventData = new BatchEventData();
for (IntellifyBase event : batchIntellifyBase.getBatch()) {
if (event instanceof EventData) {
batchEventData.getEventData().add((EventData) event);
}
}
return batchEventData;
}
private String jacksonJson(BatchDescribes batchDescribes) {
ObjectMapper mapper = new ObjectMapper();
// mapper.enableDefaultTyping(ObjectMapper.DefaultTyping.OBJECT_AND_NON_CONCRETE);
try {
String json = mapper.writeValueAsString(batchDescribes);
logger.debug("#$#$#$ BatchDescribe as JSON = " + json);
return json;
} catch (JsonGenerationException | JsonMappingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
private String jacksonJson(BatchEvents batchEvents) {
ObjectMapper mapper = new ObjectMapper();
// mapper.enableDefaultTyping(ObjectMapper.DefaultTyping.OBJECT_AND_NON_CONCRETE);
try {
return mapper.writeValueAsString(batchEvents);
} catch (JsonGenerationException | JsonMappingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
private String jacksonJson(BatchEventData batchEventData) {
ObjectMapper mapper = new ObjectMapper();
// mapper.enableDefaultTyping(ObjectMapper.DefaultTyping.OBJECT_AND_NON_CONCRETE);
// mapper.registerModule(new EventModule());
try {
String json = mapper.writeValueAsString(batchEventData);
logger.debug("#$#$#$ BatchEventData as JSON = " + json);
return json;
} catch (JsonGenerationException | JsonMappingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
private String jacksonJson(BatchEntityData batchEntityData) {
ObjectMapper mapper = new ObjectMapper();
// mapper.enableDefaultTyping(ObjectMapper.DefaultTyping.OBJECT_AND_NON_CONCRETE);
// mapper.registerModule(new EntityModule());
try {
logger.debug("#$#$#$ Starting serialization of BatchEntityData = ");
String json = mapper.writeValueAsString(batchEntityData);
logger.debug("#$#$#$ BatchEntityData as JSON = " + json);
return json;
} catch (JsonGenerationException | JsonMappingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
private void reportWithoutCallback(BatchEvents batch, boolean success) {
for (CaliperEntity event : batch.getEvents()) {
if (success) {
client.getStatistics().updateSuccessful(1);
} else {
client.getStatistics().updateFailed(1);
}
}
}
private void reportWithoutCallback(BatchDescribes batch, boolean success) {
for (CaliperEntity describe : batch.getDescribes()) {
if (success) {
client.getStatistics().updateSuccessful(1);
} else {
client.getStatistics().updateFailed(1);
}
}
}
private void reportWithoutCallback(BatchEventData batchEventData,
boolean success) {
for (IntellifyBase event : batchEventData.getEventData()) {
if (success) {
client.getStatistics().updateSuccessful(1);
} else {
client.getStatistics().updateFailed(1);
}
if (client.getOptions().isEnableTestMode()) {
client.notifyEventSend(success, ((EventData) event).getEvent());
}
}
}
private void reportWithoutCallback(BatchEntityData batchEntityData,
boolean success) {
for (IntellifyBase describe : batchEntityData.getEntityData()) {
if (success) {
client.getStatistics().updateSuccessful(1);
} else {
client.getStatistics().updateFailed(1);
}
if (client.getOptions().isEnableTestMode()) {
client.notifyEntitySend(success,
((EntityData) describe).getEntity());
}
}
}
@Override
public void close() {
try {
httpClient.close();
} catch (IOException e) {
logger.debug("Error closing http client", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy