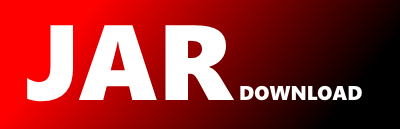
com.intellifylearning.Intellify Maven / Gradle / Ivy
package com.intellifylearning;
import com.intellifylearning.metrics.models.Agent;
import com.intellifylearning.models.Callback;
import com.intellifylearning.stats.IntelliSenseStatistics;
public class Intellify {
private static Client defaultClient;
/**
* Creates a new IntelliSense client.
*
* The client is an HTTP wrapper over the IntelliSense REST API. It will
* allow you to conveniently consume the API without making any HTTP
* requests yourself.
*
* This client is also designed to be thread-safe and to not block each of
* your calls to make a HTTP request. It uses batching to efficiently send
* your requests on a separate resource-constrained thread pool.
*
* This method is thread-safe.
*
* @param apiKey Your IntelliSense apiKey. You can get one of these by
* registering for a project at http://www.intellifylearning.com
*/
public static synchronized void initialize(String apiKey) {
if (defaultClient == null) {
defaultClient = new Client(apiKey, new Options());
}
}
/**
* Creates a new IntelliSense client.
*
* The client is an HTTP wrapper over the IntelliSense REST API. It will
* allow you to conveniently consume the API without making any HTTP
* requests yourself.
*
* This client is also designed to be thread-safe and to not block each of
* your calls to make a HTTP request. It uses batching to efficiently send
* your requests on a separate resource-constrained thread pool.
*
* This method is thread-safe.
*
* @param apiKey Your IntelliSense apiKey. You can get one of these by
* registering for a project at http://www.intellifylearning.com
*
* @param options Options to configure the behavior of the IntelliSense client
*
*/
public static synchronized void initialize(String apiKey, Options options) {
if (defaultClient == null) {
defaultClient = new Client(apiKey, options);
}
}
private static void checkInitialized() {
if (defaultClient == null) {
throw new IllegalStateException("IntellifyBase client is "
+ "not initialized. Please call IntellifyBase.iniitalize(..); "
+ "before calling identify / track / or flush.");
}
}
/**
* Caliper Sensor API (Caliper Beta)
*
* Describe a {@link Agent} that is part of the learning graph
*
* @param entity data to send
*
*/
public static void describe(
com.intellify.api.caliper.impl.entities.Entity entity) {
checkInitialized();
defaultClient.identify(entity);
}
public static void describeSync(com.intellify.api.caliper.impl.entities.Entity entity) {
checkInitialized();
defaultClient.identifySync(entity);
}
/**
* Caliper Sensor API (1.0 beta)
*
* Measure a CaliperEvent
*
* @param event the Caliper CaliperEvent
*
*/
public static void measure(
com.intellify.api.caliper.impl.events.Event event) {
checkInitialized();
defaultClient.measure(event);
}
public static void measureSync(com.intellify.api.caliper.impl.events.Event event) {
checkInitialized();
defaultClient.measureSync(event);
}
/**
* Caliper Sensor API (1.0)
*
* Measure a CaliperEvent
*
* @param event the Caliper CaliperEvent
*
*/
public static void send(com.intellify.api.caliper.impl.events.Event event) {
checkInitialized();
defaultClient.measure(event);
}
public static void sendSync(com.intellify.api.caliper.impl.events.Event event) {
checkInitialized();
defaultClient.measureSync(event);
}
/**
* Sends the given event JSON synchronously.
*
* @param eventJson a caliper-compliant event message
*/
public static void sendEventJsonSync(String eventJson) {
checkInitialized();
defaultClient.sendEventJsonSync(eventJson);
}
/**
* Sends the given events in a batch synchronously.
*
* @param eventsJsonArray a json array of caliper-compliant event messages
*/
public static void sendBatchEventsJsonSync(String eventsJsonArray) {
checkInitialized();
defaultClient.sendBatchEventsJsonSync(eventsJsonArray);
}
/**
* Sends the given entity JSON synchronously
*
* @param entityJson a caliper-compliant entity message
*/
public static void sendEntityJsonSync(String entityJson) {
checkInitialized();
defaultClient.sendEntityJsonSync(entityJson);
}
/**
* Sends the given entities in a batch synchronously.
*
* @param entitiesJsonArray a json array of caliper-compliant entity messages
*/
public static void sendBatchEntitiesJsonSync(String entitiesJsonArray) {
checkInitialized();
defaultClient.sendBatchEntitiesJsonSync(entitiesJsonArray);
}
/**
* Register callback for debugging
*
* @param callback Callback
*/
public static void registerCallback(Callback callback) {
checkInitialized();
defaultClient.registerCallback(callback);
}
/**
* Blocks until all messages in the queue are flushed.
*/
public static void flush() {
checkInitialized();
defaultClient.flush();
}
/**
* Closes the threads associated with the client
*/
public static void close() {
checkInitialized();
defaultClient.close();
}
/**
* Flushes all messages and closes the threads associated with the client
*/
public static void flushAndClose() {
flush();
close();
}
/**
* Returns statistics for the IntelliSense client
*
* @return statistics
*/
public static IntelliSenseStatistics getStatistics() {
checkInitialized();
return defaultClient.getStatistics();
}
/**
* Fetches the default intellisense client singleton
*
* @return default client
*/
public static Client getDefaultClient() {
return defaultClient;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy