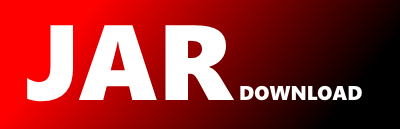
com.intellisrc.web.service.Session.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of web Show documentation
Show all versions of web Show documentation
Create restful HTTP (GET, POST, PUT, DELETE, etc) or SSE / WebSocket application services. Manage JSON data from and to the server easily. It is build on top of Jetty library, so it is very powerful but designed to be elegant and easy to use.
The newest version!
package com.intellisrc.web.service
import groovy.transform.CompileStatic
import jakarta.servlet.http.HttpSession
import org.eclipse.jetty.websocket.api.Session as JettySession
/**
* HTTPSession/WebSocket Session wrapper
* @since 2023/05/25.
*/
@CompileStatic
class Session {
final String id
protected HttpSession httpSession = null
protected JettySession websocketSession = null //This will be set by the WebSocket Server
Session(String id, HttpSession session) {
this.id = id
this.httpSession = session
}
Session(String id, JettySession session) {
this.id = id
this.websocketSession = session
}
Object attribute(String key) {
return httpSession ? httpSession.getAttribute(key) : ""
}
void attribute(String key, Object val) {
if(val == null) {
removeAttribute(key)
} else if(httpSession) {
httpSession.setAttribute(key, val)
}
}
boolean hasAttribute(String key) {
return httpSession ? attributes.contains(key) : false
}
void removeAttribute(String key) {
httpSession?.removeAttribute(key)
}
Set getAttributes() {
return httpSession ? httpSession.attributeNames.toSet() : [] as Set
}
Map getMap() {
return attributes.collectEntries { [(it) : attribute(it) ]}
}
void invalidate() {
if(httpSession) {
httpSession.invalidate()
}
if(websocketSession) {
websocketSession.close()
}
}
boolean isNew() {
return httpSession ? httpSession.new : false
}
boolean isOpen() {
return websocketSession ? websocketSession.open : false
}
HttpSession getHttpSession() {
return httpSession
}
JettySession getWebsocketSession() {
return websocketSession
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy