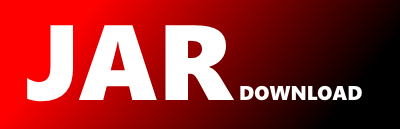
com.intershop.beehive.isml.internal.parser.ISMLparser.jj Maven / Gradle / Ivy
The newest version!
options {
JAVA_UNICODE_ESCAPE = true;
IGNORE_CASE = true;
STATIC = false;
}
PARSER_BEGIN(ISMLtoJSPcompiler)
package com.intershop.beehive.isml.internal.parser;
import com.intershop.beehive.isml.capi.ISMLException;
import com.intershop.beehive.isml.capi.ISMLTemplateConstants;
import com.intershop.beehive.isml.internal.TemplateCompiler;
import com.intershop.beehive.isml.internal.parser.Token;
import java.io.*;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.ArrayList;
public class ISMLtoJSPcompiler implements TemplateCompiler
{
boolean isSSSenabled = false;
int startline = 1;
int startcolumn = 1;
File in = null;
InputStream inStream = null;
/* JSP tags */
protected static String INLINE_SCRIPTING_START = "<%=";
protected static String INLINE_SCRIPTING_END = "%>";
protected static String SCRIPTING_START = "<% ";
protected static String SCRIPTING_END = " %>";
protected static String DIRECTIVE_START = "<%@ ";
protected static String DIRECTIVE_END = " %>";
public boolean compileTemplate( int securityLevel,
OutputStreamWriter out,
File inputfile, InputStream instr)
throws ISMLException
{
/* stack to test correct nesting and ending of IF's and LOOP's */
List nestingTable = new LinkedList();
try
{
if ( securityLevel==ALLOW_ALL )
{
isSSSenabled = true;
}
else
{
isSSSenabled = false;
}
in = inputfile;
inStream = instr;
CompactingWriter result = new CompactingWriter(new BufferedWriter(out,
ISMLTemplateConstants.DEFAULT_TEMPLATE_BUFFERSIZE), out.getEncoding());
result.print(DIRECTIVE_START);
result.print(" page buffer=\"none\"");
result.print(" import=\"java.util.*,java.io.*" +
",com.intershop.beehive.core.internal.template.*" +
",com.intershop.beehive.core.internal.template.isml.*" +
",com.intershop.beehive.core.capi.log.*" +
",com.intershop.beehive.core.capi.resource.*" +
",com.intershop.beehive.core.capi.util.UUIDMgr,com.intershop.beehive.core.capi.util.XMLHelper" +
",com.intershop.beehive.foundation.util.*" +
",com.intershop.beehive.core.internal.url.*" +
",com.intershop.beehive.core.internal.resource.*" +
",com.intershop.beehive.core.capi.pipeline.PipelineDictionary" +
",com.intershop.beehive.core.capi.naming.NamingMgr" +
",com.intershop.beehive.core.capi.pagecache.PageCacheMgr" +
",com.intershop.beehive.core.capi.request.SessionMgr" +
",com.intershop.beehive.core.internal.request.SessionMgrImpl" +
",com.intershop.beehive.core.pipelet.PipelineConstants\"");
result.print(" extends=\"com.intershop.beehive.core.internal.template.AbstractTemplate\"");
result.print(DIRECTIVE_END);
result.print(SCRIPTING_START);
result.print("\nboolean _boolean_result=false;\n");
// replace the original JSP writer with a Wrapper implementation to prevent NPE for NULL string writing
result.print("out = new WrappedJspWriter(out);\n");
result.print("TemplateExecutionConfig context = getTemplateExecutionConfig();\n");
result.print("createTemplatePageConfig(context.getServletRequest());\n");
result.print("printHeader(out);\n");
result.print(SCRIPTING_END);
result.flush();
this.specification(result, nestingTable);
if (nestingTable.size()>0)
{
throw new ParseException("Error in template " + in.getName() + " : 1 or more ISIF or ISLOOP statement without or end tag.");
}
if (token_source.brackets != 0)
{
throw new ParseException("Error in template " + in.getName() + " : Check for lost opening or closing brackets (\"(\" or \")\").");
}
result.print(SCRIPTING_START);
result.print("printFooter(out);");
result.print(SCRIPTING_END);
result.flush();
return true;
}
catch (ParseException e)
{
throw new ISMLException(e.getMessage());
}
catch (TokenMgrError e)
{
throw new ISMLException(e.getMessage());
}
catch (IOException e)
{
throw new ISMLException(e.getMessage());
}
}
}
PARSER_END(ISMLtoJSPcompiler)
TOKEN_MGR_DECLS :
{
int previousState = DEFAULT;
int brackets = 0;
}
/* Tokens outside of tags */
TOKEN :
{
< INC_TAG : "") > : DEFAULT
| < ISBREAK : ("") | (")? "/>") >
| < ISCACHE : " : IS_TAG
| < ISCACHEKEY : " : IS_TAG
| < ISDICTIONARY : " : IS_TAG
| < ISCONTENT : " : IS_TAG
| < ISCOOKIE : " : IS_TAG
| < ISELSE : ("") | (")? "/>") >
| < ISELSIF : (" : IS_TAG
| < ISIF : " : IS_TAG
| < ISIF_END : " " >
| < CLOSING_ISELSE : "
| < ISINCLUDE : " : IS_TAG
| < ISLOOP : " : IS_TAG
| < ISLOOP_END : " " >
| < ISFILEBUNDLE : " : IS_TAG
| < ISFILEBUNDLE_END : " " >
| < ISFORM : " : CUST_TAG
| < ISFORM_END : " " >
| < ISFILE : " : IS_TAG
| < ISRENDER : " : CUST_TAG
| < ISRENDER_END : " " >
| < ISMODULE : " : IS_TAG
| < ISNEXT : ("") | (")? "/>") >
| < ISPRINT : " : IS_TAG
| < ISREDIRECT : " : IS_TAG
| < ISSELECT : " : IS_TAG
| < ISSET : " : IS_TAG
| < ISBINARY : " : IS_TAG
| < ISPIPELINE : " : IS_TAG
| < ISTEXT : " : IS_TAG
| < ISPLACEHOLDER : " : IS_TAG
| < ISPLACEMENT : " : IS_TAG
| < ISPLACEMENT_END : " " >
| < ISX : ""])+ > : CUST_TAG
| < ISX_END : " "])+ > : CUST_TAG
| < IS_EXPRESSION : "#" > { previousState = DEFAULT; } : EXPRESSION
| < IS_EXP_PREVIEW : ("\"")?
([" ","\t","\n","\r"])+
"preview"
([" ","\t","\n","\r"])*
"="
([" ","\t","\n","\r"])*
"\"" (~["\""])* "\"" >
| < POWER_HOOK : ""([" ","\t","\n","\r"])* "" >
| < NO_EXPRESSION_1 : ( "#" (["0"-"9","A"-"Z"])+ ([" ","\t","\n","\r"])* (["\"",">"]) ) >
| < NO_EXPRESSION_2 : ( "#" (["0"-"9","A"-"Z"])+ ([" ","\t","\n","\r"])+ (["0"-"9","A"-"Z"]) ) >
| < NO_EXPRESSION_3 : ( ("" | "#") (["0"-"9","A"-"F"])+ ";" ) >
| < NO_EXPRESSION_4 : ( "\\\"#" (["0"-"9","A"-"F"])+ (";")? "\\\"" ) >
| < NO_EXPRESSION_5 : ( ("\"")? "#" (["0"-"9","A"-"Z"])+ ([" ","\t"])* "=") >
| < NO_EXPRESSION_6 : ( "#" (["0"-"9","A"-"F"])+ ([" ","\t","\n","\r"])* "}" ) >
| < NO_EXPRESSION_7 : ( "#" (["0"-"9","A"-"F"," ","\t","\n","\r"])* "&" ) >
| < CONTENT : ( ~["#"] ) >
}
/* comment token */
TOKEN :
{
< COMMENT_CONTENT : ((( ~["<","-"])+)|("<")|("-")) >
| < ISCOMMENT_END : ("") | ("--->") > : DEFAULT
}
/* === BEGIN SSS tokens === */
/* bean token */
TOKEN :
{
< BEAN_CONTENT: ((( ~["<"])+)|("<")) >
| < BEAN_ETAG: " " > : DEFAULT
}
/* enterprice java bean token */
TOKEN :
{
< EJB_CONTENT: ((( ~["<"])+)|("<")) >
| < EJB_ETAG: " " > : DEFAULT
}
/* serlet token */
TOKEN :
{
< SERVLET_CONTENT: ((( ~["<"])+)|("<")) >
| < SERVLET_ETAG: " " > : DEFAULT
}
/* tokens for custom tag handling */
TOKEN :
{
< CUST_ATT_NAME : ["A"-"Z"] (["_","0"-"9","A"-"Z","-"])* ()? >
| < CUST_ATT_VALUE1 : ()? (~["\"","#"])* >
| < CUST_ATT_VALUE2 : ()? (~["\"","#","="," ","\r","\n","\t"])+ >
| < CUST_SPACE : ([" ","\r","\n","\t"])+ >
| < CUST_ASSIGN : "=" >
| < CUST_END : ()? ">" > : DEFAULT
| < CUST_QUOTE : "\"" >
| < CUST_EXPR : "#" > { previousState = CUST_TAG; } : EXPRESSION
}
TOKEN :
{
< ATT_ATTRIBUTE : "attribute" ()? >
| < ATT_RETURNATTRIBUTE : "returnattribute" ()? >
| < ATT_CHARSET : "charset" ()? >
| < ATT_COMMENT : "comment" ()? >
| < ATT_COMPACT : "compact" ()? >
| < ATT_TEMPLATEMARKER: "templatemarker" ()? >
| < ATT_CONDITION : "condition" ()? >
| < ATT_DESCRIPTION: "description" ()? >
| < ATT_DOMAIN : "domain" ()? >
| < ATT_EXTENSIONPOINT: "extensionpoint" ()? >
| < ATT_HOUR : "hour" ()? >
| < ATT_LOCATION : "location" ()? >
| < ATT_HTTPSTATUS : "httpstatus" ()? >
| < ATT_KEYWORD : "keyword" ()? >
| < ATT_OBJECT : "object" ()? >
| < ATT_MAXAGE : "maxage" ()? >
| < ATT_MINUTE : "minute" ()? >
| < ATT_NAME : "name" ()? >
| < ATT_PADDING : "padding" ()? >
| < ATT_PASSWORD : "password" ()? >
| < ATT_PATH : "path" ()? >
| < ATT_PERSONALIZED: "personalized" ()? >
| < ATT_SCOPE : "scope" ()? >
| < ATT_SOURCE : "source" ()? >
| < ATT_TEMPLATE : "template" ()? >
| < ATT_STRICT : "strict" ()? >
| < ATT_TYPE : "type" ()? >
| < ATT_URL : "url" ()? >
| < ATT_USERNAME : "username" ()? >
| < ATT_VALUE : "value" ()? >
| < ATT_VERSION : "version" ()? >
| < ATT_FILE : "file" ()? >
| < ATT_STREAM : "stream" ()? >
| < ATT_RESOURCE : "resource" ()? >
| < ATT_BYTES : "bytes" ()? >
| < ATT_DOWNLOADNAME: "downloadname" ()? >
| < ATT_DICTIONARY : "dictionary" ()? >
| < ATT_PIPELINE : "pipeline" ()? >
| < ATT_PARAMS : "params" ()? >
| < ATT_SYMBOLS : "symbols" ()? >
| < ATT_KEY : "key" ()? >
| < ATT_LOCALE : "locale" ()? >
| < ATT_PARAMETER0 : "parameter0" ()? >
| < ATT_PARAMETER1 : "parameter1" ()? >
| < ATT_PARAMETER2 : "parameter2" ()? >
| < ATT_PARAMETER3 : "parameter3" ()? >
| < ATT_PARAMETER4 : "parameter4" ()? >
| < ATT_PARAMETER5 : "parameter5" ()? >
| < ATT_PARAMETER6 : "parameter6" ()? >
| < ATT_PARAMETER7 : "parameter7" ()? >
| < ATT_PARAMETER8 : "parameter8" ()? >
| < ATT_PARAMETER9 : "parameter9" ()? >
| < ATT_ID : "id" ()? >
| < ATT_PREPEND : "prepend" ()? >
| < ATT_SEPARATOR : "separator" ()? >
| < ATT_APPEND : "append" ()? >
| < ATT_PRESERVEORDER : "preserveorder" ()? >
| < ATT_REMOVEDUPLICATES : "removeduplicates" ()? >
| < ATT_PLACEHOLDERID : "placeholderid" ()? >
| < ATT_PROCESSORS : "processors" ()? >
| < ATT_ALIAS : "alias" ()? ()? > : ATTVALUE
| < ATT_COUNTER : "counter" ()? ()? > : ATTVALUE
| < ATT_ENCODE : "encoding" ()? ()? > : ATTVALUE
| < ATT_FORMAT : "formatter" ()? ()? > : ATTVALUE
| < ATT_ITERATOR : "iterator" ()? ()? > : ATTVALUE
| < ATT_MODE : "mode" ()? ()? > : ATTVALUE
| < ATT_PREVIEW : "preview" ()? ()? > : ATTVALUE
| < ATT_SECURE : "secure" ()? ()? > : ATTVALUE
| < ATT_SESSION : "session" ()? ()? > : ATTVALUE
| < ATT_STYLE : "style" ()? ()? > : ATTVALUE
| < ATT_DISABLED : "disabled" ()? ()? > : ATTVALUE
| < ATT_CLASS : "class" ()? ()? > : ATTVALUE
| < ATT_VALUE1 : ()? (~["\"","#"])* >
| < ATT_VALUE2 : ()? (~["\"","#","="," ","\r","\n","\t",">","<"])+ >
| < TAG_SPACE : ([" ","\r","\n","\t"])+ >
| < ASSIGN : "=" >
| < END_TAG : ()? ">" > : DEFAULT
| < EMPTY_ELEMENT_END_TAG: ()? "/>" > : DEFAULT
| < QUOTE : "\"" >
| < ATT_EXPR : "#" > { previousState = IS_TAG; } : EXPRESSION
}
TOKEN :
{
< VALUE_Q : "\"" (~["\""])* "\"" > : IS_TAG
| < VALUE_N : (~[" ","\r","\n","\t","\"",">","#","="])+ > : IS_TAG
}
TOKEN:
{
< BRACKET_OPEN: ("(") >{brackets++;}
| < BRACKET_CLOSE: (")") >{brackets--;}
| < JSP_EMBEDDED_EXPRESSION: "<%=" ((~["%"])|(("%")+ ~[">"]))* "%>" >
| < JSP_EMBEDDED_EL: "${" (~["}"])* "}" >
| < ADD: ("+") >
| < SUB: ("-") >
| < MUL: ("*") >
| < DIV: ("/") >
| < MOD: ("%") >
| < I_EG: ("==") >
| < I_NE: ("!=") >
| < I_GE: (">=") >
| < I_LE: ("<=") >
| < I_GT: (">") >
| < I_LT: ("<") >
| < CAT: (".") >
| < EQ: ("EQ") >
| < NE: ("NE") >
| < AND: ("AND") >
| < OR: ("OR") >
| < NOT: ("NOT") >
| < F1: ("hasLoopElements")|("hasElements") >
| < F2: ("isSSSenabled") >
| < F3: ("getHeader") >
| < F4: ("getValue") >
| < F5: ("URL") >
| < F6: ("URLEx") >
| < F7: ("Action") >
| < F8: ("Parameter") >
| < F9: ("WebRoot") >
| < F10: ("isDefined") >
| < F11: ("stringToHtml") >
| < F12: ("pad") >
| < F13: ("stringToXml") >
| < F14: ("trim") >
| < F15: ("lcase") >
| < F16: ("ucase") >
| < F17: ("len") >
| < F18: ("val") >
| < F19: ("getCookie") >
| < F20: ("Servlet") >
| < F21: ("ContentURL") >
| < F22: ("Service") >
| < F25: ("sessionlessURL") >
| < F26: ("sessionlessURLEx") >
| < F27: ("pipeline") >
| < F34: ("hasNext") >
| < F35: ("replace") >
| < F36: ("split") >
| < F37: ("existsTemplate") >
| < F38: ("WebRootEx") >
| < F39: ("ContentURLEx") >
| < F40: ("ParamMap") >
| < F41: ("ParamEntry") >
| < F42: ("getTemplateSourceLocation") >
| < F43: ("localizeText") >
| < F44: ("localizeTextEx") >
| < F45: ("ParameterList") >
| < F46: ("encodeValue") >
| < EXP_STOP: ("#") > { SwitchTo(previousState); }
| < STRING_SINGLEQUOTED: "\'"
( ("\\" (~["\'"]))
|("\\\'")
|(~["\'","\\"])
|("\'\'")
)*
"\'" >
| < STRING_DOUBLEQUOTED: "\""
( ("\\" (~["\""]))
|("\\\"")
|(~["\"","\\"])
|("\"\"")
)*
"\"" >
| < DOUBLE: (["0"-"9"])+ "." (["0"-"9"])* ( "e" (["+","-"])? (["0"-"9"])+)?
| "." (["0"-"9"])+ ( "e" (["+","-"])? (["0"-"9"])+)?
| (["0"-"9"])+ ( "e" (["+","-"])? (["0"-"9"])+)? >
| < COLON: ":" >
| < COMMA: "," >
| < VARNAME: ["A"-"Z"] (["_","0"-"9","A"-"Z"])* >
}
SKIP : { " " | "\t" | "\n" | "\r" }
TOKEN :
{
< ALL : ((~["0"])|(["0"])) >
}
/* Main production rule */
void specification(CompactingWriter result, List nestingTable) throws IOException :
{
Token token = null;
boolean previewAttributeRemoveFlag = false;
}
{
( ( token = )
{
result.print(token);
result.print(SCRIPTING_START);
result.print("insertIntershopSignature(request,(com.intershop.beehive.core.capi.request.ServletResponse)response);");
result.print(SCRIPTING_END);
}
| (stuff(result))
{
previewAttributeRemoveFlag = false;
}
| (sss(result))
{ previewAttributeRemoveFlag = false; }
| (isTag(result, nestingTable))
{ previewAttributeRemoveFlag = false; }
| ( (token = )
{
startline = token.beginLine;
startcolumn = token.beginColumn;
}
previewAttributeRemoveFlag = outExpression(result)
)
| (token = )
{
if (!previewAttributeRemoveFlag)
{
// print usual HTML content
result.print(token);
}
else if (token.toString().charAt(0) == '\"')
{
result.print("\"");
}
previewAttributeRemoveFlag = false;
}
| ( ()* )
{
/* comment handling */
}
| ( )
{
throw new ParseException("Error in template " + in.getName() + " : Check comment usage, nesting is not allowed and there must be a starting \"\" (or \"\").");
}
| ( )
{
throw new ParseException("Error in template " + in.getName() + " : Unexpected token
encountered!");
}
)*
}
/* rule for ISML expressions outside ISML tags */
boolean outExpression(CompactingWriter result) throws IOException :
{
StringBuilder buffer = new StringBuilder();
}
{
try
{
(is_expression(buffer) )
{
result.print(INLINE_SCRIPTING_START);
result.print("context.getFormattedValue(" + buffer + ",null)");
result.print(INLINE_SCRIPTING_END);
return true;
}
}
catch (ParseException e)
{
/* this is a workaround for single #'s (as in color codes) */
/* if is_expression fails the input stream is parsed again */
/* from the beginning to process all tokens after the '#' */
/* with scope default */
if (e.getMessage().indexOf('#') < 0)
{
throw new ParseException("Error in template " + in.getName() + " : " + e.getMessage());
}
try
{
InputStream ins = inStream;
if (ins != null && ins.markSupported())
{
ins.reset();
}
else
{
ins = new BufferedInputStream(new FileInputStream(in), ISMLTemplateConstants.DEFAULT_TEMPLATE_BUFFERSIZE);
}
this.ReInit(ins);
token_source.SwitchTo(SKIPPER);
Token skipperToken = getNextToken();
while ( (skipperToken.beginLine &
are just a workarounds for the "single #'s" -
workaround to speed up template processing significantly
is a special case for CSS color codes
is for color codes enclosed in escaped double quotes */
void stuff(CompactingWriter result) throws IOException :
{
Token stuff;
}
{
( stuff=
|stuff=
|stuff=
|stuff=
|stuff=
|stuff=
|stuff=
|stuff=)
{
// print usual HTML content
result.printCompact(stuff);
}
}
/**
* Server side scripting rules
**/
void sss(CompactingWriter result) throws IOException :
{
Token stuff;
}
{
( ( stuff = | stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
| beanTag(result)
| servletTag(result)
| ejbTag(result)
)
}
void beanTag(CompactingWriter result) throws IOException :
{
Token stuff;
}
{ ( stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
( (stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
)*
( stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
}
void servletTag(CompactingWriter result) throws IOException :
{
Token stuff;
}
{ ( stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
( (stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
)*
( stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
}
void ejbTag(CompactingWriter result) throws IOException :
{
Token stuff;
}
{ ( stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
( (stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
)*
( stuff = )
{
if (isSSSenabled) { result.print(stuff); }
}
}
/**
* ISML tag rule
*/
void isTag(CompactingWriter result, List nestingTable) :
{
/**
* the tag factory - map tags to Server Side Scripting
*/
Token tag = null;
HashMap attributes = new HashMap();
}
{
( ( tag= | tag=
| tag= | tag=
| tag= | tag=
| tag= | tag=
| tag=
)
| ( ( tag= )
( ( tagAttribute2(attributes, ATT_ATTRIBUTE, ATT_RETURNATTRIBUTE))+ ( | ) )
)
| ( ( tag= | tag= | tag= | tag=
| tag= | tag= | tag=
| tag= | tag= | tag= | tag=
| tag= | tag= | tag= | tag=
| tag= | tag=
)
( ( tagAttribute(attributes))+ ( | ) )
)
| ( ( tag= | tag= | tag=
)
( ( tagAttribute(attributes))+ )
)
| ( ( tag= | tag=
)
( ( customAttribute(attributes))* )
)
| ( ( tag=
)
( ( formAttribute(attributes))* )
)
| ( tag= )
)
{
ISMLTagCompiler.compileTag(tag, result, attributes, nestingTable);
}
}
/**
* ISML tag attribute rules
**/
void tagAttribute(HashMap attributes) : {}
{
{tagAttribute2(attributes, -1, -1);}
}
void tagAttribute2(HashMap attributes, int multiValueAttr1, int multiValueAttr2) :
{
/**
* the attribute factory - parses tag-attributes
*/
Token name=null,value=null;
String buf;
StringBuilder buffer1 = new StringBuilder();
}
{
( ( ( name= | name= | name=
| name= | name=| name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name= | name=
)
( ( ()?
( ( (is_expression(buffer1)) )
| ( (is_expression(buffer1)) )
)
)
{
storeAttribute(attributes, '#' + String.valueOf(name.kind),buffer1.toString(),name.kind==multiValueAttr1 || name.kind==multiValueAttr2);
}
| ( value= | value= )
{
String realValue = value.toString();
realValue = realValue.substring(1).trim();
if (value.kind == ATT_VALUE1)
{
storeAttribute(attributes, String.valueOf(name.kind),
realValue.substring(1,realValue.length()-1), name.kind==multiValueAttr1 || name.kind==multiValueAttr2);
}
else
{
storeAttribute(attributes, String.valueOf(name.kind),realValue, name.kind==multiValueAttr1 || name.kind==multiValueAttr2);
}
}
)
)
| ( ( name= | name= | name=
| name= | name= | name=
| name= | name= | name=
| name= | name=| name=
)
(value=|value=)
)
{
buf = value.toString();
if (value.kind==VALUE_Q)
{
buf = buf.substring(1,buf.length()-1);
}
storeAttribute(attributes, String.valueOf(name.kind),buf, name.kind==multiValueAttr1 || name.kind==multiValueAttr2);
}
)
}
void storeAttribute(HashMap attributes, String name, String value, boolean isMultivalue) : {}
{
{
if (!isMultivalue)
{
attributes.put(name, value);
}
else
{
@SuppressWarnings("unchecked")
ArrayList v = (ArrayList)attributes.get(name);
if (v == null)
{
v = new ArrayList();
attributes.put(name, v);
}
v.add(value);
}
}
}
/**
* ISML form tag attribute rule
**/
void formAttribute(HashMap attributes) :
{
/**
* the attribute factory - parses tag-attributes
*/
Token name=null,value=null;
StringBuilder buffer1 = new StringBuilder();
}
{
( name=
( ( ()?
(
( (is_expression(buffer1)) )
| ( (is_expression(buffer1)) )
)
)
{
attributes.put('#' + name.toString().trim(),buffer1);
}
| ( value= | value= )
{
String realValue = value.toString();
realValue = realValue.substring(1).trim();
if (value.kind == CUST_ATT_VALUE1)
{
attributes.put(name.toString().trim(),
realValue.substring(1,realValue.length()-1));
}
else
{
attributes.put(name.toString().trim(),realValue);
}
}
)
)
}
/**
* ISML custom tag attribute rule
**/
void customAttribute(HashMap attributes) :
{
/**
* the attribute factory - parses tag-attributes
*/
Token name=null,value=null;
StringBuilder buffer1 = new StringBuilder();
}
{
( name=
( ( ()?
(
( (is_expression(buffer1)) )
| ( (is_expression(buffer1)) )
)
)
{
attributes.put(name.toString().trim(),buffer1);
}
| ( value= | value= )
{
String realValue = value.toString();
realValue = realValue.substring(1).trim();
if (value.kind == CUST_ATT_VALUE1)
{
attributes.put(name.toString().trim(),
realValue.substring(1,realValue.length()-1));
}
else
{
attributes.put(name.toString().trim(),realValue);
}
}
)
)
}
/*
* ISML Action syntax rule
*/
void is_action(StringBuilder jsp_buffer) :
{
StringBuilder buffer = new StringBuilder();
}
{
( ( ()
{
// start Method
jsp_buffer.append("(new URLPipelineAction(");
}
[ (is_expression(buffer))
{
// parameter action
jsp_buffer.append("context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter server group
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter domain
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter locale
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter currency
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter appID
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
}
]
]
]
]
]
]
()
{
jsp_buffer.append("))");
}
)
)
|
(
( ()
{
// start Method
jsp_buffer.append("(new URLServletAction(");
}
[ (is_expression(buffer))
{
// parameter servlet name
jsp_buffer.append("context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter server group
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
]
]
()
{
jsp_buffer.append("))");
}
)
)
|
(
( ()
{
// start Method
jsp_buffer.append("(new URLServiceAction(");
}
[ (is_expression(buffer))
{
// parameter action
jsp_buffer.append("context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter server group
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter domain
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter locale
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter currency
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
buffer.setLength(0);
}
[ ( is_expression(buffer))
{
// parameter appID
jsp_buffer.append(",context.getFormattedValue(" + buffer + ",null)");
}
]
]
]
]
]
]
()
{
jsp_buffer.append("))");
}
)
)
}
/*
* ISML expression syntax rule
*/
void is_expression(StringBuilder jsp_buffer) :
{
StringBuilder buffer2 = new StringBuilder();
boolean flag = true;
}
{
( (
operator(buffer2)
[flag = boolean_expr(jsp_buffer,buffer2,true) ]
)
{
if (flag)
{
/* special handling of case (NOT operator()) */
jsp_buffer.append("(!Boolean.valueOf(String.valueOf(" + buffer2+ "))) ");
}
}
| ( operator(buffer2)
[ flag = arithmetic_comp(jsp_buffer,buffer2)
| flag = string_comp(jsp_buffer,buffer2)
| flag = boolean_expr(jsp_buffer,buffer2,false)
| flag = arithmetic_expr(jsp_buffer,buffer2)
| flag = string_expr(jsp_buffer,buffer2)
]
{
if (flag)
{
/* special handling if expression is just a single operator */
jsp_buffer.append(buffer2.toString());
}
}
)
)
}
/* addition to ISML expression syntax rule */
void operator(StringBuilder jsp_buffer) :
{ }
{
(LOOKAHEAD(3) function(jsp_buffer)
| jsp_embedded_expression(jsp_buffer)
| jsp_embedded_el(jsp_buffer)
| var(jsp_buffer)
| constant(jsp_buffer)
| bracket(jsp_buffer)
)
}
/* rule for arithmetic comparisation operations */
boolean arithmetic_comp(StringBuilder jsp_buffer,StringBuilder buffer2) :
{
Token op = null;
}
{
( ( op = | op = | op = | op = | op = | op = )
{
jsp_buffer.append("((");
/* String to double conversions not supported yet
jsp_buffer.append(" ((("+buffer2+")instanceof Number)?
jsp_buffer.append("(((Number) " + buffer2 + ").doubleValue()):");
jsp_buffer.append("((Double.valueOf(context.getFormattedValue(" + buffer2 + ",null))).doubleValue())) " + op.toString());
*/
jsp_buffer.append(" ((Number) " + buffer2 + ").doubleValue() " + op.toString());
/* clearbuffer */
buffer2.setLength(0);
}
operator(buffer2)
)
{
jsp_buffer.append("((Number)("+buffer2+")).doubleValue()) ? Boolean.TRUE : Boolean.FALSE)");
return false; /* more than one operator */
}
}
/* rule for string comparisation operations */
boolean string_comp(StringBuilder jsp_buffer,StringBuilder buffer2) :
{
Token op = null;
}
{
( ( op = | op = )
{
jsp_buffer.append("((");
if (op.kind==NE)
{
jsp_buffer.append("!");
}
jsp_buffer.append("(context.getFormattedValue(" + buffer2 + ",null).equals(context.getFormattedValue(");
/* clearbuffer */
buffer2.setLength(0);
}
operator(buffer2)
)
{
jsp_buffer.append(buffer2 + ",null)))) ? Boolean.TRUE : Boolean.FALSE)");
return false; /* more than one operator */
}
}
/* rule for arithmetic operations */
boolean arithmetic_expr(StringBuilder jsp_buffer,StringBuilder buffer2) :
{
int i = 0;
Token op = null;
}
{
( ( op = | op = | op = | op = | op = )
{
if (i==0)
{
jsp_buffer.append("(Double.valueOf(");
}
/* String to double conversions not supported yet
jsp_buffer.append(" ((("+buffer2+")instanceof Number)?
jsp_buffer.append("(((Number) " + buffer2 + ").doubleValue()):");
jsp_buffer.append("((Double.valueOf(context.getFormattedValue(" + buffer2 + ",null))).doubleValue())) " + op.toString());
*/
jsp_buffer.append(" ((Number) " + buffer2 + ").doubleValue() " + op.toString());
buffer2.setLength(0);
i++;
}
operator(buffer2)
)+
{
jsp_buffer.append("((Number) " + buffer2 + ").doubleValue()))");
return false; /* more than one operator */
}
}
/* rule for string operations */
boolean string_expr(StringBuilder jsp_buffer,StringBuilder buffer2) :
{ }
{
(
{
jsp_buffer.append("context.getFormattedValue(" + buffer2 + ",null) + ");
buffer2.setLength(0);
}
operator(buffer2)
)+
{
jsp_buffer.append("context.getFormattedValue(" + buffer2 + ",null)");
return false; /* more than one operator */
}
}
/* rule for boolean operations */
boolean boolean_expr(StringBuilder jsp_buffer,StringBuilder buffer2,boolean notSwitch) :
{
int i = 0;
Token op = null;
}
{
( ( op = | op = )
{
if (i==0)
{
jsp_buffer.append("((");
}
if (notSwitch)
{
jsp_buffer.append("!");
}
jsp_buffer.append("Boolean.parseBoolean(String.valueOf(" + buffer2 + ")) ");
jsp_buffer.append((op.kind==AND)?"&& ":"|| ");
/* clearbuffer */
buffer2.setLength(0);
notSwitch = false;
i=1;
}
[ ( )
{ notSwitch = true; }
]
operator(buffer2)
)+
{
if (notSwitch)
{
jsp_buffer.append("!");
}
jsp_buffer.append("Boolean.valueOf(String.valueOf(" + buffer2 + "))))");
return false; /* more than one operator */
}
}
/* rule for paranthising */
void bracket(StringBuilder jsp_buffer) :
{}
{
()
{
jsp_buffer.append("(");
}
(is_expression(jsp_buffer))
{
jsp_buffer.append(")");
}
}
/* rules for ISML expression constants */
void constant(StringBuilder jsp_buffer) :
{ }
{
stringConstant(jsp_buffer)
| numberConstant(jsp_buffer)
}
void stringConstant(StringBuilder jsp_buffer) :
{
Token value = null;
}
{
(value= | value =)
{
boolean hasPreviousSlash = false;
boolean hasPreviousQuote = false;
char currentChar;
String stringValue = value.toString();
jsp_buffer.append('\"');
for (int i=1; i<(stringValue.length()-1); i++)
{
currentChar = stringValue.charAt(i);
// handling of special characters
switch (currentChar)
{
case '\\':
hasPreviousSlash = !hasPreviousSlash;
jsp_buffer.append('\\');
break;
case 'd':
if (hasPreviousSlash)
{
jsp_buffer.append('\"');
hasPreviousSlash = false;
}
else
{
jsp_buffer.append('d');
}
break;
case 's':
if (hasPreviousSlash)
{
jsp_buffer.append('\'');
hasPreviousSlash = false;
}
else
{
jsp_buffer.append('s');
}
break;
case '\'':
if (value.kind == STRING_SINGLEQUOTED)
{
if (hasPreviousQuote)
{
jsp_buffer.append('\\');
jsp_buffer.append('\'');
hasPreviousQuote = false;
}
else if (hasPreviousSlash)
{
jsp_buffer.append('\'');
hasPreviousSlash = false;
}
else
{
hasPreviousQuote = true;
}
}
else
{
if (hasPreviousSlash)
{
jsp_buffer.append('\'');
hasPreviousSlash = false;
}
else
{
jsp_buffer.append('\\');
jsp_buffer.append('\'');
}
}
break;
case '\"':
if (value.kind == STRING_DOUBLEQUOTED)
{
if (hasPreviousQuote)
{
jsp_buffer.append('\\');
jsp_buffer.append('\"');
hasPreviousQuote = false;
}
else if (hasPreviousSlash)
{
jsp_buffer.append('\"');
hasPreviousSlash = false;
}
else
{
hasPreviousQuote = true;
}
}
else
{
if (hasPreviousSlash)
{
jsp_buffer.append('\"');
hasPreviousSlash = false;
}
else
{
jsp_buffer.append('\\');
jsp_buffer.append('\"');
}
}
break;
default:
jsp_buffer.append(currentChar);
hasPreviousSlash = false;
hasPreviousQuote = false;
}
}
jsp_buffer.append('\"');
}
}
void numberConstant(StringBuilder jsp_buffer) :
{
Token value = null;
Token sign = null;
}
{
[sign=|sign=] value=
{
if (sign==null)
{
jsp_buffer.append("Double.valueOf(" + value + ")");
}
else
{
jsp_buffer.append("Double.valueOf(" + sign + value + ")");
}
}
}
/* rule for ISML expression functions */
void function(StringBuilder jsp_buffer) :
{
Token value = null;
StringBuilder buffer = null;
boolean flag = false;
}
{
(
( ( )
{
jsp_buffer.append("(hasLoopElements(\"");
}
( var_name(jsp_buffer) )
{
jsp_buffer.append("\") ? Boolean.TRUE : Boolean.FALSE)");
}
)
| ( )
{
jsp_buffer.append("isSSSenabled()");
}
| ( ( )
{
// getHeader
jsp_buffer.append("(request.getHeader(context.getFormattedValue(");
}
( is_expression(jsp_buffer)