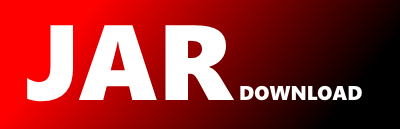
com.intershop.beehive.isml.internal.parser.ISMLtoJSPcompilerTokenManager Maven / Gradle / Ivy
The newest version!
/* Generated By:JavaCC: Do not edit this line. ISMLtoJSPcompilerTokenManager.java */
package com.intershop.beehive.isml.internal.parser;
import com.intershop.beehive.isml.capi.ISMLException;
import com.intershop.beehive.isml.capi.ISMLTemplateConstants;
import com.intershop.beehive.isml.internal.TemplateCompiler;
import com.intershop.beehive.isml.internal.parser.Token;
import java.io.*;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.ArrayList;
/** Token Manager. */
public class ISMLtoJSPcompilerTokenManager implements ISMLtoJSPcompilerConstants
{
int previousState = DEFAULT;
int brackets = 0;
/** Debug output. */
public java.io.PrintStream debugStream = System.out;
/** Set debug output. */
public void setDebugStream(java.io.PrintStream ds) { debugStream = ds; }
private final int jjStopStringLiteralDfa_6(int pos, long active0, long active1, long active2)
{
switch (pos)
{
default :
return -1;
}
}
private final int jjStartNfa_6(int pos, long active0, long active1, long active2)
{
return jjMoveNfa_6(jjStopStringLiteralDfa_6(pos, active0, active1, active2), pos + 1);
}
private int jjStopAtPos(int pos, int kind)
{
jjmatchedKind = kind;
jjmatchedPos = pos;
return pos + 1;
}
private int jjMoveStringLiteralDfa0_6()
{
switch(curChar)
{
case 34:
return jjStopAtPos(0, 148);
case 35:
return jjStopAtPos(0, 149);
case 61:
return jjStartNfaWithStates_6(0, 145, 620);
default :
return jjMoveNfa_6(6, 0);
}
}
private int jjStartNfaWithStates_6(int pos, int kind, int state)
{
jjmatchedKind = kind;
jjmatchedPos = pos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return pos + 1; }
return jjMoveNfa_6(state, pos + 1);
}
static final long[] jjbitVec0 = {
0xfffffffffffffffeL, 0xffffffffffffffffL, 0xffffffffffffffffL, 0xffffffffffffffffL
};
static final long[] jjbitVec2 = {
0x0L, 0x0L, 0xffffffffffffffffL, 0xffffffffffffffffL
};
private int jjMoveNfa_6(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 620;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 6:
if ((0x100002600L & l) != 0L)
{
if (kind > 144)
kind = 144;
jjCheckNAddStates(0, 4);
}
else if (curChar == 61)
jjCheckNAddStates(5, 8);
else if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 19;
else if (curChar == 62)
{
if (kind > 146)
kind = 146;
}
break;
case 620:
if ((0x8ffffff2ffffd9ffL & l) != 0L)
{
if (kind > 143)
kind = 143;
jjCheckNAdd(615);
}
else if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(614, 615);
else if (curChar == 34)
jjCheckNAddTwoStates(612, 613);
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(610, 611);
break;
case 1:
if ((0x100002600L & l) == 0L)
break;
if (kind > 84)
kind = 84;
jjstateSet[jjnewStateCnt++] = 1;
break;
case 8:
if ((0x100002600L & l) == 0L)
break;
if (kind > 87)
kind = 87;
jjstateSet[jjnewStateCnt++] = 8;
break;
case 13:
if ((0x100002600L & l) == 0L)
break;
if (kind > 104)
kind = 104;
jjstateSet[jjnewStateCnt++] = 13;
break;
case 18:
if (curChar == 62 && kind > 146)
kind = 146;
break;
case 19:
if (curChar == 62 && kind > 147)
kind = 147;
break;
case 20:
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 19;
break;
case 23:
if ((0x100002600L & l) == 0L)
break;
if (kind > 70)
kind = 70;
jjstateSet[jjnewStateCnt++] = 23;
break;
case 32:
if ((0x100002600L & l) == 0L)
break;
if (kind > 125)
kind = 125;
jjstateSet[jjnewStateCnt++] = 32;
break;
case 38:
if ((0x100002600L & l) != 0L)
jjAddStates(9, 10);
break;
case 39:
if (curChar != 61)
break;
if (kind > 130)
kind = 130;
jjCheckNAdd(40);
break;
case 40:
if ((0x100002600L & l) == 0L)
break;
if (kind > 130)
kind = 130;
jjCheckNAdd(40);
break;
case 46:
if ((0x100002600L & l) == 0L)
break;
if (kind > 71)
kind = 71;
jjstateSet[jjnewStateCnt++] = 46;
break;
case 61:
if ((0x100002600L & l) == 0L)
break;
if (kind > 103)
kind = 103;
jjstateSet[jjnewStateCnt++] = 61;
break;
case 69:
if ((0x100002600L & l) == 0L)
break;
if (kind > 127)
kind = 127;
jjstateSet[jjnewStateCnt++] = 69;
break;
case 86:
if ((0x100002600L & l) == 0L)
break;
if (kind > 72)
kind = 72;
jjstateSet[jjnewStateCnt++] = 86;
break;
case 93:
if ((0x100002600L & l) == 0L)
break;
if (kind > 73)
kind = 73;
jjstateSet[jjnewStateCnt++] = 93;
break;
case 100:
if ((0x100002600L & l) == 0L)
break;
if (kind > 74)
kind = 74;
jjstateSet[jjnewStateCnt++] = 100;
break;
case 107:
if ((0x100002600L & l) == 0L)
break;
if (kind > 76)
kind = 76;
jjstateSet[jjnewStateCnt++] = 107;
break;
case 116:
if ((0x100002600L & l) != 0L)
jjAddStates(11, 12);
break;
case 117:
if (curChar != 61)
break;
if (kind > 131)
kind = 131;
jjCheckNAdd(118);
break;
case 118:
if ((0x100002600L & l) == 0L)
break;
if (kind > 131)
kind = 131;
jjCheckNAdd(118);
break;
case 125:
if ((0x100002600L & l) != 0L)
jjAddStates(13, 14);
break;
case 126:
if (curChar != 61)
break;
if (kind > 141)
kind = 141;
jjCheckNAdd(127);
break;
case 127:
if ((0x100002600L & l) == 0L)
break;
if (kind > 141)
kind = 141;
jjCheckNAdd(127);
break;
case 133:
if ((0x100002600L & l) == 0L)
break;
if (kind > 75)
kind = 75;
jjstateSet[jjnewStateCnt++] = 133;
break;
case 147:
if ((0x100002600L & l) == 0L)
break;
if (kind > 94)
kind = 94;
jjstateSet[jjnewStateCnt++] = 147;
break;
case 155:
if ((0x100002600L & l) == 0L)
break;
if (kind > 96)
kind = 96;
jjstateSet[jjnewStateCnt++] = 155;
break;
case 160:
if ((0x100002600L & l) == 0L)
break;
if (kind > 77)
kind = 77;
jjstateSet[jjnewStateCnt++] = 160;
break;
case 171:
if ((0x100002600L & l) == 0L)
break;
if (kind > 78)
kind = 78;
jjstateSet[jjnewStateCnt++] = 171;
break;
case 177:
if ((0x100002600L & l) == 0L)
break;
if (kind > 105)
kind = 105;
jjstateSet[jjnewStateCnt++] = 177;
break;
case 189:
if ((0x100002600L & l) == 0L)
break;
if (kind > 106)
kind = 106;
jjstateSet[jjnewStateCnt++] = 189;
break;
case 199:
if ((0x100002600L & l) != 0L)
jjAddStates(15, 16);
break;
case 200:
if (curChar != 61)
break;
if (kind > 140)
kind = 140;
jjCheckNAdd(201);
break;
case 201:
if ((0x100002600L & l) == 0L)
break;
if (kind > 140)
kind = 140;
jjCheckNAdd(201);
break;
case 210:
if ((0x100002600L & l) == 0L)
break;
if (kind > 79)
kind = 79;
jjstateSet[jjnewStateCnt++] = 210;
break;
case 224:
if ((0x100002600L & l) != 0L)
jjAddStates(17, 18);
break;
case 225:
if (curChar != 61)
break;
if (kind > 132)
kind = 132;
jjCheckNAdd(226);
break;
case 226:
if ((0x100002600L & l) == 0L)
break;
if (kind > 132)
kind = 132;
jjCheckNAdd(226);
break;
case 235:
if ((0x100002600L & l) == 0L)
break;
if (kind > 80)
kind = 80;
jjstateSet[jjnewStateCnt++] = 235;
break;
case 239:
if ((0x100002600L & l) == 0L)
break;
if (kind > 82)
kind = 82;
jjstateSet[jjnewStateCnt++] = 239;
break;
case 250:
if ((0x100002600L & l) == 0L)
break;
if (kind > 81)
kind = 81;
jjstateSet[jjnewStateCnt++] = 250;
break;
case 258:
if ((0x100002600L & l) == 0L)
break;
if (kind > 111)
kind = 111;
jjstateSet[jjnewStateCnt++] = 258;
break;
case 265:
if ((0x100002600L & l) == 0L)
break;
if (kind > 83)
kind = 83;
jjstateSet[jjnewStateCnt++] = 265;
break;
case 272:
if ((0x100002600L & l) == 0L)
break;
if (kind > 110)
kind = 110;
jjstateSet[jjnewStateCnt++] = 272;
break;
case 276:
if ((0x100002600L & l) == 0L)
break;
if (kind > 85)
kind = 85;
jjstateSet[jjnewStateCnt++] = 276;
break;
case 282:
if ((0x100002600L & l) == 0L)
break;
if (kind > 86)
kind = 86;
jjstateSet[jjnewStateCnt++] = 282;
break;
case 288:
if ((0x100002600L & l) != 0L)
jjAddStates(19, 20);
break;
case 289:
if (curChar != 61)
break;
if (kind > 135)
kind = 135;
jjCheckNAdd(290);
break;
case 290:
if ((0x100002600L & l) == 0L)
break;
if (kind > 135)
kind = 135;
jjCheckNAdd(290);
break;
case 295:
if ((0x100002600L & l) == 0L)
break;
if (kind > 88)
kind = 88;
jjstateSet[jjnewStateCnt++] = 295;
break;
case 302:
if ((0x100002600L & l) == 0L)
break;
if (kind > 89)
kind = 89;
jjstateSet[jjnewStateCnt++] = 302;
break;
case 310:
if ((0x100002600L & l) == 0L)
break;
if (kind > 90)
kind = 90;
jjstateSet[jjnewStateCnt++] = 310;
break;
case 314:
if ((0x100002600L & l) == 0L)
break;
if (kind > 91)
kind = 91;
jjstateSet[jjnewStateCnt++] = 314;
break;
case 326:
if ((0x100002600L & l) == 0L)
break;
if (kind > 107)
kind = 107;
jjstateSet[jjnewStateCnt++] = 326;
break;
case 334:
if ((0x100002600L & l) == 0L)
break;
if (kind > 108)
kind = 108;
jjstateSet[jjnewStateCnt++] = 334;
break;
case 339:
if (curChar != 48)
break;
if (kind > 112)
kind = 112;
jjCheckNAdd(340);
break;
case 340:
if ((0x100002600L & l) == 0L)
break;
if (kind > 112)
kind = 112;
jjCheckNAdd(340);
break;
case 349:
if (curChar != 49)
break;
if (kind > 113)
kind = 113;
jjCheckNAdd(350);
break;
case 350:
if ((0x100002600L & l) == 0L)
break;
if (kind > 113)
kind = 113;
jjCheckNAdd(350);
break;
case 359:
if (curChar != 50)
break;
if (kind > 114)
kind = 114;
jjCheckNAdd(360);
break;
case 360:
if ((0x100002600L & l) == 0L)
break;
if (kind > 114)
kind = 114;
jjCheckNAdd(360);
break;
case 369:
if (curChar != 51)
break;
if (kind > 115)
kind = 115;
jjCheckNAdd(370);
break;
case 370:
if ((0x100002600L & l) == 0L)
break;
if (kind > 115)
kind = 115;
jjCheckNAdd(370);
break;
case 379:
if (curChar != 52)
break;
if (kind > 116)
kind = 116;
jjCheckNAdd(380);
break;
case 380:
if ((0x100002600L & l) == 0L)
break;
if (kind > 116)
kind = 116;
jjCheckNAdd(380);
break;
case 389:
if (curChar != 53)
break;
if (kind > 117)
kind = 117;
jjCheckNAdd(390);
break;
case 390:
if ((0x100002600L & l) == 0L)
break;
if (kind > 117)
kind = 117;
jjCheckNAdd(390);
break;
case 399:
if (curChar != 54)
break;
if (kind > 118)
kind = 118;
jjCheckNAdd(400);
break;
case 400:
if ((0x100002600L & l) == 0L)
break;
if (kind > 118)
kind = 118;
jjCheckNAdd(400);
break;
case 409:
if (curChar != 55)
break;
if (kind > 119)
kind = 119;
jjCheckNAdd(410);
break;
case 410:
if ((0x100002600L & l) == 0L)
break;
if (kind > 119)
kind = 119;
jjCheckNAdd(410);
break;
case 419:
if (curChar != 56)
break;
if (kind > 120)
kind = 120;
jjCheckNAdd(420);
break;
case 420:
if ((0x100002600L & l) == 0L)
break;
if (kind > 120)
kind = 120;
jjCheckNAdd(420);
break;
case 429:
if (curChar != 57)
break;
if (kind > 121)
kind = 121;
jjCheckNAdd(430);
break;
case 430:
if ((0x100002600L & l) == 0L)
break;
if (kind > 121)
kind = 121;
jjCheckNAdd(430);
break;
case 440:
if ((0x100002600L & l) == 0L)
break;
if (kind > 123)
kind = 123;
jjstateSet[jjnewStateCnt++] = 440;
break;
case 447:
if ((0x100002600L & l) == 0L)
break;
if (kind > 126)
kind = 126;
jjstateSet[jjnewStateCnt++] = 447;
break;
case 460:
if ((0x100002600L & l) == 0L)
break;
if (kind > 128)
kind = 128;
jjstateSet[jjnewStateCnt++] = 460;
break;
case 473:
if ((0x100002600L & l) == 0L)
break;
if (kind > 129)
kind = 129;
jjstateSet[jjnewStateCnt++] = 473;
break;
case 483:
if ((0x100002600L & l) != 0L)
jjAddStates(21, 22);
break;
case 484:
if (curChar != 61)
break;
if (kind > 136)
kind = 136;
jjCheckNAdd(485);
break;
case 485:
if ((0x100002600L & l) == 0L)
break;
if (kind > 136)
kind = 136;
jjCheckNAdd(485);
break;
case 493:
if ((0x100002600L & l) == 0L)
break;
if (kind > 92)
kind = 92;
jjstateSet[jjnewStateCnt++] = 493;
break;
case 498:
if ((0x100002600L & l) == 0L)
break;
if (kind > 93)
kind = 93;
jjstateSet[jjnewStateCnt++] = 498;
break;
case 504:
if ((0x100002600L & l) == 0L)
break;
if (kind > 95)
kind = 95;
jjstateSet[jjnewStateCnt++] = 504;
break;
case 510:
if ((0x100002600L & l) == 0L)
break;
if (kind > 102)
kind = 102;
jjstateSet[jjnewStateCnt++] = 510;
break;
case 516:
if ((0x100002600L & l) == 0L)
break;
if (kind > 109)
kind = 109;
jjstateSet[jjnewStateCnt++] = 516;
break;
case 523:
if ((0x100002600L & l) == 0L)
break;
if (kind > 124)
kind = 124;
jjstateSet[jjnewStateCnt++] = 523;
break;
case 532:
if ((0x100002600L & l) != 0L)
jjAddStates(23, 24);
break;
case 533:
if (curChar != 61)
break;
if (kind > 137)
kind = 137;
jjCheckNAdd(534);
break;
case 534:
if ((0x100002600L & l) == 0L)
break;
if (kind > 137)
kind = 137;
jjCheckNAdd(534);
break;
case 540:
if ((0x100002600L & l) != 0L)
jjAddStates(25, 26);
break;
case 541:
if (curChar != 61)
break;
if (kind > 138)
kind = 138;
jjCheckNAdd(542);
break;
case 542:
if ((0x100002600L & l) == 0L)
break;
if (kind > 138)
kind = 138;
jjCheckNAdd(542);
break;
case 549:
if ((0x100002600L & l) != 0L)
jjAddStates(27, 28);
break;
case 550:
if (curChar != 61)
break;
if (kind > 139)
kind = 139;
jjCheckNAdd(551);
break;
case 551:
if ((0x100002600L & l) == 0L)
break;
if (kind > 139)
kind = 139;
jjCheckNAdd(551);
break;
case 557:
if ((0x100002600L & l) == 0L)
break;
if (kind > 97)
kind = 97;
jjstateSet[jjnewStateCnt++] = 557;
break;
case 560:
if ((0x100002600L & l) == 0L)
break;
if (kind > 98)
kind = 98;
jjstateSet[jjnewStateCnt++] = 560;
break;
case 569:
if ((0x100002600L & l) == 0L)
break;
if (kind > 99)
kind = 99;
jjstateSet[jjnewStateCnt++] = 569;
break;
case 574:
if ((0x100002600L & l) == 0L)
break;
if (kind > 100)
kind = 100;
jjstateSet[jjnewStateCnt++] = 574;
break;
case 582:
if ((0x100002600L & l) == 0L)
break;
if (kind > 101)
kind = 101;
jjstateSet[jjnewStateCnt++] = 582;
break;
case 586:
if ((0x100002600L & l) != 0L)
jjAddStates(29, 30);
break;
case 587:
if (curChar != 61)
break;
if (kind > 133)
kind = 133;
jjCheckNAdd(588);
break;
case 588:
if ((0x100002600L & l) == 0L)
break;
if (kind > 133)
kind = 133;
jjCheckNAdd(588);
break;
case 598:
if ((0x100002600L & l) == 0L)
break;
if (kind > 122)
kind = 122;
jjstateSet[jjnewStateCnt++] = 598;
break;
case 600:
if ((0x100002600L & l) != 0L)
jjAddStates(31, 32);
break;
case 601:
if (curChar != 61)
break;
if (kind > 134)
kind = 134;
jjCheckNAdd(602);
break;
case 602:
if ((0x100002600L & l) == 0L)
break;
if (kind > 134)
kind = 134;
jjCheckNAdd(602);
break;
case 609:
if (curChar == 61)
jjCheckNAddStates(5, 8);
break;
case 610:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(610, 611);
break;
case 611:
if (curChar == 34)
jjCheckNAddTwoStates(612, 613);
break;
case 612:
if ((0xfffffff3ffffffffL & l) != 0L)
jjCheckNAddTwoStates(612, 613);
break;
case 613:
if (curChar == 34 && kind > 142)
kind = 142;
break;
case 614:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(614, 615);
break;
case 615:
if ((0x8ffffff2ffffd9ffL & l) == 0L)
break;
if (kind > 143)
kind = 143;
jjCheckNAdd(615);
break;
case 616:
if ((0x100002600L & l) == 0L)
break;
if (kind > 144)
kind = 144;
jjCheckNAddStates(0, 4);
break;
case 617:
if ((0x100002600L & l) == 0L)
break;
if (kind > 144)
kind = 144;
jjCheckNAdd(617);
break;
case 618:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(618, 18);
break;
case 619:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(619, 20);
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 6:
if ((0x20000000200L & l) != 0L)
jjAddStates(33, 34);
else if ((0x4000000040L & l) != 0L)
jjAddStates(35, 36);
else if ((0x40000000400000L & l) != 0L)
jjAddStates(37, 38);
else if ((0x20000000200000L & l) != 0L)
jjAddStates(39, 40);
else if ((0x8000000080000L & l) != 0L)
jjAddStates(41, 49);
else if ((0x1000000010000L & l) != 0L)
jjAddStates(50, 70);
else if ((0x200000002000L & l) != 0L)
jjAddStates(71, 73);
else if ((0x80000000800L & l) != 0L)
jjAddStates(74, 75);
else if ((0x100000001000L & l) != 0L)
jjAddStates(76, 77);
else if ((0x10000000100L & l) != 0L)
jjAddStates(78, 79);
else if ((0x2000000020L & l) != 0L)
jjAddStates(80, 81);
else if ((0x1000000010L & l) != 0L)
jjAddStates(82, 86);
else if ((0x10000000100000L & l) != 0L)
jjAddStates(87, 89);
else if ((0x800000008L & l) != 0L)
jjAddStates(90, 95);
else if ((0x4000000040000L & l) != 0L)
jjAddStates(96, 98);
else if ((0x200000002L & l) != 0L)
jjAddStates(99, 101);
else if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 16;
else if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 10;
else if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 5;
break;
case 620:
case 615:
if (kind > 143)
kind = 143;
jjCheckNAdd(615);
break;
case 0:
if ((0x10000000100000L & l) == 0L)
break;
if (kind > 84)
kind = 84;
jjstateSet[jjnewStateCnt++] = 1;
break;
case 2:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 0;
break;
case 3:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 2;
break;
case 4:
if ((0x40000000400L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 3;
break;
case 5:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 4;
break;
case 7:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 87)
kind = 87;
jjstateSet[jjnewStateCnt++] = 8;
break;
case 9:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 7;
break;
case 10:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 9;
break;
case 11:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 10;
break;
case 12:
if ((0x8000000080000L & l) == 0L)
break;
if (kind > 104)
kind = 104;
jjstateSet[jjnewStateCnt++] = 13;
break;
case 14:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 12;
break;
case 15:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 14;
break;
case 16:
if ((0x200000002000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 15;
break;
case 17:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 16;
break;
case 21:
if ((0x200000002L & l) != 0L)
jjAddStates(99, 101);
break;
case 22:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 70)
kind = 70;
jjstateSet[jjnewStateCnt++] = 23;
break;
case 24:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 22;
break;
case 25:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 24;
break;
case 26:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 25;
break;
case 27:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 26;
break;
case 28:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 27;
break;
case 29:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 28;
break;
case 30:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 29;
break;
case 31:
if ((0x1000000010L & l) == 0L)
break;
if (kind > 125)
kind = 125;
jjstateSet[jjnewStateCnt++] = 32;
break;
case 33:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 31;
break;
case 34:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 33;
break;
case 35:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 34;
break;
case 36:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 35;
break;
case 37:
if ((0x8000000080000L & l) != 0L)
jjAddStates(9, 10);
break;
case 41:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 37;
break;
case 42:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 41;
break;
case 43:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 42;
break;
case 44:
if ((0x4000000040000L & l) != 0L)
jjAddStates(96, 98);
break;
case 45:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 71)
kind = 71;
jjstateSet[jjnewStateCnt++] = 46;
break;
case 47:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 45;
break;
case 48:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 47;
break;
case 49:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 48;
break;
case 50:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 49;
break;
case 51:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 50;
break;
case 52:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 51;
break;
case 53:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 52;
break;
case 54:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 53;
break;
case 55:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 54;
break;
case 56:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 55;
break;
case 57:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 56;
break;
case 58:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 57;
break;
case 59:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 58;
break;
case 60:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 103)
kind = 103;
jjstateSet[jjnewStateCnt++] = 61;
break;
case 62:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 60;
break;
case 63:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 62;
break;
case 64:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 63;
break;
case 65:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 64;
break;
case 66:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 65;
break;
case 67:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 66;
break;
case 68:
if ((0x8000000080000L & l) == 0L)
break;
if (kind > 127)
kind = 127;
jjstateSet[jjnewStateCnt++] = 69;
break;
case 70:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 68;
break;
case 71:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 70;
break;
case 72:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 71;
break;
case 73:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 72;
break;
case 74:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 73;
break;
case 75:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 74;
break;
case 76:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 75;
break;
case 77:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 76;
break;
case 78:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 77;
break;
case 79:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 78;
break;
case 80:
if ((0x40000000400000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 79;
break;
case 81:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 80;
break;
case 82:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 81;
break;
case 83:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 82;
break;
case 84:
if ((0x800000008L & l) != 0L)
jjAddStates(90, 95);
break;
case 85:
if ((0x10000000100000L & l) == 0L)
break;
if (kind > 72)
kind = 72;
jjstateSet[jjnewStateCnt++] = 86;
break;
case 87:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 85;
break;
case 88:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 87;
break;
case 89:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 88;
break;
case 90:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 89;
break;
case 91:
if ((0x10000000100L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 90;
break;
case 92:
if ((0x10000000100000L & l) == 0L)
break;
if (kind > 73)
kind = 73;
jjstateSet[jjnewStateCnt++] = 93;
break;
case 94:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 92;
break;
case 95:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 94;
break;
case 96:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 95;
break;
case 97:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 96;
break;
case 98:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 97;
break;
case 99:
if ((0x10000000100000L & l) == 0L)
break;
if (kind > 74)
kind = 74;
jjstateSet[jjnewStateCnt++] = 100;
break;
case 101:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 99;
break;
case 102:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 101;
break;
case 103:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 102;
break;
case 104:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 103;
break;
case 105:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 104;
break;
case 106:
if ((0x400000004000L & l) == 0L)
break;
if (kind > 76)
kind = 76;
jjstateSet[jjnewStateCnt++] = 107;
break;
case 108:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 106;
break;
case 109:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 108;
break;
case 110:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 109;
break;
case 111:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 110;
break;
case 112:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 111;
break;
case 113:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 112;
break;
case 114:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 113;
break;
case 115:
if ((0x4000000040000L & l) != 0L)
jjAddStates(11, 12);
break;
case 119:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 115;
break;
case 120:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 119;
break;
case 121:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 120;
break;
case 122:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 121;
break;
case 123:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 122;
break;
case 124:
if ((0x8000000080000L & l) != 0L)
jjAddStates(13, 14);
break;
case 128:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 124;
break;
case 129:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 128;
break;
case 130:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 129;
break;
case 131:
if ((0x10000000100000L & l) != 0L)
jjAddStates(87, 89);
break;
case 132:
if ((0x4000000040000L & l) == 0L)
break;
if (kind > 75)
kind = 75;
jjstateSet[jjnewStateCnt++] = 133;
break;
case 134:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 132;
break;
case 135:
if ((0x80000000800L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 134;
break;
case 136:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 135;
break;
case 137:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 136;
break;
case 138:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 137;
break;
case 139:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 138;
break;
case 140:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 139;
break;
case 141:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 140;
break;
case 142:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 141;
break;
case 143:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 142;
break;
case 144:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 143;
break;
case 145:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 144;
break;
case 146:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 94)
kind = 94;
jjstateSet[jjnewStateCnt++] = 147;
break;
case 148:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 146;
break;
case 149:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 148;
break;
case 150:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 149;
break;
case 151:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 150;
break;
case 152:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 151;
break;
case 153:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 152;
break;
case 154:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 96)
kind = 96;
jjstateSet[jjnewStateCnt++] = 155;
break;
case 156:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 154;
break;
case 157:
if ((0x200000002000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 156;
break;
case 158:
if ((0x1000000010L & l) != 0L)
jjAddStates(82, 86);
break;
case 159:
if ((0x400000004000L & l) == 0L)
break;
if (kind > 77)
kind = 77;
jjstateSet[jjnewStateCnt++] = 160;
break;
case 161:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 159;
break;
case 162:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 161;
break;
case 163:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 162;
break;
case 164:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 163;
break;
case 165:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 164;
break;
case 166:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 165;
break;
case 167:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 166;
break;
case 168:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 167;
break;
case 169:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 168;
break;
case 170:
if ((0x400000004000L & l) == 0L)
break;
if (kind > 78)
kind = 78;
jjstateSet[jjnewStateCnt++] = 171;
break;
case 172:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 170;
break;
case 173:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 172;
break;
case 174:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 173;
break;
case 175:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 174;
break;
case 176:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 105)
kind = 105;
jjstateSet[jjnewStateCnt++] = 177;
break;
case 178:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 176;
break;
case 179:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 178;
break;
case 180:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 179;
break;
case 181:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 180;
break;
case 182:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 181;
break;
case 183:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 182;
break;
case 184:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 183;
break;
case 185:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 184;
break;
case 186:
if ((0x80000000800000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 185;
break;
case 187:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 186;
break;
case 188:
if ((0x200000002000000L & l) == 0L)
break;
if (kind > 106)
kind = 106;
jjstateSet[jjnewStateCnt++] = 189;
break;
case 190:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 188;
break;
case 191:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 190;
break;
case 192:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 191;
break;
case 193:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 192;
break;
case 194:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 193;
break;
case 195:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 194;
break;
case 196:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 195;
break;
case 197:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 196;
break;
case 198:
if ((0x1000000010L & l) != 0L)
jjAddStates(15, 16);
break;
case 202:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 198;
break;
case 203:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 202;
break;
case 204:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 203;
break;
case 205:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 204;
break;
case 206:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 205;
break;
case 207:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 206;
break;
case 208:
if ((0x2000000020L & l) != 0L)
jjAddStates(80, 81);
break;
case 209:
if ((0x10000000100000L & l) == 0L)
break;
if (kind > 79)
kind = 79;
jjstateSet[jjnewStateCnt++] = 210;
break;
case 211:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 209;
break;
case 212:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 211;
break;
case 213:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 212;
break;
case 214:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 213;
break;
case 215:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 214;
break;
case 216:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 215;
break;
case 217:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 216;
break;
case 218:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 217;
break;
case 219:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 218;
break;
case 220:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 219;
break;
case 221:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 220;
break;
case 222:
if ((0x100000001000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 221;
break;
case 223:
if ((0x8000000080L & l) != 0L)
jjAddStates(17, 18);
break;
case 227:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 223;
break;
case 228:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 227;
break;
case 229:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 228;
break;
case 230:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 229;
break;
case 231:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 230;
break;
case 232:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 231;
break;
case 233:
if ((0x10000000100L & l) != 0L)
jjAddStates(78, 79);
break;
case 234:
if ((0x4000000040000L & l) == 0L)
break;
if (kind > 80)
kind = 80;
jjstateSet[jjnewStateCnt++] = 235;
break;
case 236:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 234;
break;
case 237:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 236;
break;
case 238:
if ((0x8000000080000L & l) == 0L)
break;
if (kind > 82)
kind = 82;
jjstateSet[jjnewStateCnt++] = 239;
break;
case 240:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 238;
break;
case 241:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 240;
break;
case 242:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 241;
break;
case 243:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 242;
break;
case 244:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 243;
break;
case 245:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 244;
break;
case 246:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 245;
break;
case 247:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 246;
break;
case 248:
if ((0x100000001000L & l) != 0L)
jjAddStates(76, 77);
break;
case 249:
if ((0x400000004000L & l) == 0L)
break;
if (kind > 81)
kind = 81;
jjstateSet[jjnewStateCnt++] = 250;
break;
case 251:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 249;
break;
case 252:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 251;
break;
case 253:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 252;
break;
case 254:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 253;
break;
case 255:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 254;
break;
case 256:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 255;
break;
case 257:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 111)
kind = 111;
jjstateSet[jjnewStateCnt++] = 258;
break;
case 259:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 257;
break;
case 260:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 259;
break;
case 261:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 260;
break;
case 262:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 261;
break;
case 263:
if ((0x80000000800L & l) != 0L)
jjAddStates(74, 75);
break;
case 264:
if ((0x1000000010L & l) == 0L)
break;
if (kind > 83)
kind = 83;
jjstateSet[jjnewStateCnt++] = 265;
break;
case 266:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 264;
break;
case 267:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 266;
break;
case 268:
if ((0x80000000800000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 267;
break;
case 269:
if ((0x200000002000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 268;
break;
case 270:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 269;
break;
case 271:
if ((0x200000002000000L & l) == 0L)
break;
if (kind > 110)
kind = 110;
jjstateSet[jjnewStateCnt++] = 272;
break;
case 273:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 271;
break;
case 274:
if ((0x200000002000L & l) != 0L)
jjAddStates(71, 73);
break;
case 275:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 85)
kind = 85;
jjstateSet[jjnewStateCnt++] = 276;
break;
case 277:
if ((0x8000000080L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 275;
break;
case 278:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 277;
break;
case 279:
if ((0x100000001000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 278;
break;
case 280:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 279;
break;
case 281:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 86)
kind = 86;
jjstateSet[jjnewStateCnt++] = 282;
break;
case 283:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 281;
break;
case 284:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 283;
break;
case 285:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 284;
break;
case 286:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 285;
break;
case 287:
if ((0x2000000020L & l) != 0L)
jjAddStates(19, 20);
break;
case 291:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 287;
break;
case 292:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 291;
break;
case 293:
if ((0x1000000010000L & l) != 0L)
jjAddStates(50, 70);
break;
case 294:
if ((0x8000000080L & l) == 0L)
break;
if (kind > 88)
kind = 88;
jjstateSet[jjnewStateCnt++] = 295;
break;
case 296:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 294;
break;
case 297:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 296;
break;
case 298:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 297;
break;
case 299:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 298;
break;
case 300:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 299;
break;
case 301:
if ((0x1000000010L & l) == 0L)
break;
if (kind > 89)
kind = 89;
jjstateSet[jjnewStateCnt++] = 302;
break;
case 303:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 301;
break;
case 304:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 303;
break;
case 305:
if ((0x80000000800000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 304;
break;
case 306:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 305;
break;
case 307:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 306;
break;
case 308:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 307;
break;
case 309:
if ((0x10000000100L & l) == 0L)
break;
if (kind > 90)
kind = 90;
jjstateSet[jjnewStateCnt++] = 310;
break;
case 311:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 309;
break;
case 312:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 311;
break;
case 313:
if ((0x1000000010L & l) == 0L)
break;
if (kind > 91)
kind = 91;
jjstateSet[jjnewStateCnt++] = 314;
break;
case 315:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 313;
break;
case 316:
if ((0x400000004000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 315;
break;
case 317:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 316;
break;
case 318:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 317;
break;
case 319:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 318;
break;
case 320:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 319;
break;
case 321:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 320;
break;
case 322:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 321;
break;
case 323:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 322;
break;
case 324:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 323;
break;
case 325:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 107)
kind = 107;
jjstateSet[jjnewStateCnt++] = 326;
break;
case 327:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 325;
break;
case 328:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 327;
break;
case 329:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 328;
break;
case 330:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 329;
break;
case 331:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 330;
break;
case 332:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 331;
break;
case 333:
if ((0x8000000080000L & l) == 0L)
break;
if (kind > 108)
kind = 108;
jjstateSet[jjnewStateCnt++] = 334;
break;
case 335:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 333;
break;
case 336:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 335;
break;
case 337:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 336;
break;
case 338:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 337;
break;
case 341:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 339;
break;
case 342:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 341;
break;
case 343:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 342;
break;
case 344:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 343;
break;
case 345:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 344;
break;
case 346:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 345;
break;
case 347:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 346;
break;
case 348:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 347;
break;
case 351:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 349;
break;
case 352:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 351;
break;
case 353:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 352;
break;
case 354:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 353;
break;
case 355:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 354;
break;
case 356:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 355;
break;
case 357:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 356;
break;
case 358:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 357;
break;
case 361:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 359;
break;
case 362:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 361;
break;
case 363:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 362;
break;
case 364:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 363;
break;
case 365:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 364;
break;
case 366:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 365;
break;
case 367:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 366;
break;
case 368:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 367;
break;
case 371:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 369;
break;
case 372:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 371;
break;
case 373:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 372;
break;
case 374:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 373;
break;
case 375:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 374;
break;
case 376:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 375;
break;
case 377:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 376;
break;
case 378:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 377;
break;
case 381:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 379;
break;
case 382:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 381;
break;
case 383:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 382;
break;
case 384:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 383;
break;
case 385:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 384;
break;
case 386:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 385;
break;
case 387:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 386;
break;
case 388:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 387;
break;
case 391:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 389;
break;
case 392:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 391;
break;
case 393:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 392;
break;
case 394:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 393;
break;
case 395:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 394;
break;
case 396:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 395;
break;
case 397:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 396;
break;
case 398:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 397;
break;
case 401:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 399;
break;
case 402:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 401;
break;
case 403:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 402;
break;
case 404:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 403;
break;
case 405:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 404;
break;
case 406:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 405;
break;
case 407:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 406;
break;
case 408:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 407;
break;
case 411:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 409;
break;
case 412:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 411;
break;
case 413:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 412;
break;
case 414:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 413;
break;
case 415:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 414;
break;
case 416:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 415;
break;
case 417:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 416;
break;
case 418:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 417;
break;
case 421:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 419;
break;
case 422:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 421;
break;
case 423:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 422;
break;
case 424:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 423;
break;
case 425:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 424;
break;
case 426:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 425;
break;
case 427:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 426;
break;
case 428:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 427;
break;
case 431:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 429;
break;
case 432:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 431;
break;
case 433:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 432;
break;
case 434:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 433;
break;
case 435:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 434;
break;
case 436:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 435;
break;
case 437:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 436;
break;
case 438:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 437;
break;
case 439:
if ((0x1000000010L & l) == 0L)
break;
if (kind > 123)
kind = 123;
jjstateSet[jjnewStateCnt++] = 440;
break;
case 441:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 439;
break;
case 442:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 441;
break;
case 443:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 442;
break;
case 444:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 443;
break;
case 445:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 444;
break;
case 446:
if ((0x4000000040000L & l) == 0L)
break;
if (kind > 126)
kind = 126;
jjstateSet[jjnewStateCnt++] = 447;
break;
case 448:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 446;
break;
case 449:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 448;
break;
case 450:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 449;
break;
case 451:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 450;
break;
case 452:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 451;
break;
case 453:
if ((0x40000000400000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 452;
break;
case 454:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 453;
break;
case 455:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 454;
break;
case 456:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 455;
break;
case 457:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 456;
break;
case 458:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 457;
break;
case 459:
if ((0x1000000010L & l) == 0L)
break;
if (kind > 128)
kind = 128;
jjstateSet[jjnewStateCnt++] = 460;
break;
case 461:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 459;
break;
case 462:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 461;
break;
case 463:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 462;
break;
case 464:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 463;
break;
case 465:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 464;
break;
case 466:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 465;
break;
case 467:
if ((0x10000000100L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 466;
break;
case 468:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 467;
break;
case 469:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 468;
break;
case 470:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 469;
break;
case 471:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 470;
break;
case 472:
if ((0x8000000080000L & l) == 0L)
break;
if (kind > 129)
kind = 129;
jjstateSet[jjnewStateCnt++] = 473;
break;
case 474:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 472;
break;
case 475:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 474;
break;
case 476:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 475;
break;
case 477:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 476;
break;
case 478:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 477;
break;
case 479:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 478;
break;
case 480:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 479;
break;
case 481:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 480;
break;
case 482:
if ((0x80000000800000L & l) != 0L)
jjAddStates(21, 22);
break;
case 486:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 482;
break;
case 487:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 486;
break;
case 488:
if ((0x40000000400000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 487;
break;
case 489:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 488;
break;
case 490:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 489;
break;
case 491:
if ((0x8000000080000L & l) != 0L)
jjAddStates(41, 49);
break;
case 492:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 92)
kind = 92;
jjstateSet[jjnewStateCnt++] = 493;
break;
case 494:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 492;
break;
case 495:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 494;
break;
case 496:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 495;
break;
case 497:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 93)
kind = 93;
jjstateSet[jjnewStateCnt++] = 498;
break;
case 499:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 497;
break;
case 500:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 499;
break;
case 501:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 500;
break;
case 502:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 501;
break;
case 503:
if ((0x10000000100000L & l) == 0L)
break;
if (kind > 95)
kind = 95;
jjstateSet[jjnewStateCnt++] = 504;
break;
case 505:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 503;
break;
case 506:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 505;
break;
case 507:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 506;
break;
case 508:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 507;
break;
case 509:
if ((0x200000002000L & l) == 0L)
break;
if (kind > 102)
kind = 102;
jjstateSet[jjnewStateCnt++] = 510;
break;
case 511:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 509;
break;
case 512:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 511;
break;
case 513:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 512;
break;
case 514:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 513;
break;
case 515:
if ((0x8000000080000L & l) == 0L)
break;
if (kind > 109)
kind = 109;
jjstateSet[jjnewStateCnt++] = 516;
break;
case 517:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 515;
break;
case 518:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 517;
break;
case 519:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 518;
break;
case 520:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 519;
break;
case 521:
if ((0x200000002000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 520;
break;
case 522:
if ((0x4000000040000L & l) == 0L)
break;
if (kind > 124)
kind = 124;
jjstateSet[jjnewStateCnt++] = 523;
break;
case 524:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 522;
break;
case 525:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 524;
break;
case 526:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 525;
break;
case 527:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 526;
break;
case 528:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 527;
break;
case 529:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 528;
break;
case 530:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 529;
break;
case 531:
if ((0x2000000020L & l) != 0L)
jjAddStates(23, 24);
break;
case 535:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 531;
break;
case 536:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 535;
break;
case 537:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 536;
break;
case 538:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 537;
break;
case 539:
if ((0x400000004000L & l) != 0L)
jjAddStates(25, 26);
break;
case 543:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 539;
break;
case 544:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 543;
break;
case 545:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 544;
break;
case 546:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 545;
break;
case 547:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 546;
break;
case 548:
if ((0x2000000020L & l) != 0L)
jjAddStates(27, 28);
break;
case 552:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 548;
break;
case 553:
if ((0x200000002000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 552;
break;
case 554:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 553;
break;
case 555:
if ((0x20000000200000L & l) != 0L)
jjAddStates(39, 40);
break;
case 556:
if ((0x100000001000L & l) == 0L)
break;
if (kind > 97)
kind = 97;
jjstateSet[jjnewStateCnt++] = 557;
break;
case 558:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 556;
break;
case 559:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 98)
kind = 98;
jjstateSet[jjnewStateCnt++] = 560;
break;
case 561:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 559;
break;
case 562:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 561;
break;
case 563:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 562;
break;
case 564:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 563;
break;
case 565:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 564;
break;
case 566:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 565;
break;
case 567:
if ((0x40000000400000L & l) != 0L)
jjAddStates(37, 38);
break;
case 568:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 99)
kind = 99;
jjstateSet[jjnewStateCnt++] = 569;
break;
case 570:
if ((0x20000000200000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 568;
break;
case 571:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 570;
break;
case 572:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 571;
break;
case 573:
if ((0x400000004000L & l) == 0L)
break;
if (kind > 100)
kind = 100;
jjstateSet[jjnewStateCnt++] = 574;
break;
case 575:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 573;
break;
case 576:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 575;
break;
case 577:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 576;
break;
case 578:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 577;
break;
case 579:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 578;
break;
case 580:
if ((0x4000000040L & l) != 0L)
jjAddStates(35, 36);
break;
case 581:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 101)
kind = 101;
jjstateSet[jjnewStateCnt++] = 582;
break;
case 583:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 581;
break;
case 584:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 583;
break;
case 585:
if ((0x4000000040000L & l) != 0L)
jjAddStates(29, 30);
break;
case 589:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 585;
break;
case 590:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 589;
break;
case 591:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 590;
break;
case 592:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 591;
break;
case 593:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 592;
break;
case 594:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 593;
break;
case 595:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 594;
break;
case 596:
if ((0x20000000200L & l) != 0L)
jjAddStates(33, 34);
break;
case 597:
if ((0x1000000010L & l) == 0L)
break;
if (kind > 122)
kind = 122;
jjstateSet[jjnewStateCnt++] = 598;
break;
case 599:
if ((0x4000000040000L & l) != 0L)
jjAddStates(31, 32);
break;
case 603:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 599;
break;
case 604:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 603;
break;
case 605:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 604;
break;
case 606:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 605;
break;
case 607:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 606;
break;
case 608:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 607;
break;
case 612:
jjAddStates(102, 103);
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 620:
case 615:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 143)
kind = 143;
jjCheckNAdd(615);
break;
case 612:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjAddStates(102, 103);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 620 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private int jjMoveStringLiteralDfa0_7()
{
return jjMoveNfa_7(0, 0);
}
private int jjMoveNfa_7(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 4;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 0:
if ((0x9ffffff2ffffd9ffL & l) != 0L)
{
if (kind > 151)
kind = 151;
jjCheckNAdd(3);
}
else if (curChar == 34)
jjCheckNAddTwoStates(1, 2);
break;
case 1:
if ((0xfffffffbffffffffL & l) != 0L)
jjCheckNAddTwoStates(1, 2);
break;
case 2:
if (curChar == 34 && kind > 150)
kind = 150;
break;
case 3:
if ((0x9ffffff2ffffd9ffL & l) == 0L)
break;
if (kind > 151)
kind = 151;
jjCheckNAdd(3);
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 0:
case 3:
if (kind > 151)
kind = 151;
jjCheckNAdd(3);
break;
case 1:
jjAddStates(104, 105);
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 0:
case 3:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 151)
kind = 151;
jjCheckNAdd(3);
break;
case 1:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjAddStates(104, 105);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 4 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private final int jjStopStringLiteralDfa_3(int pos, long active0)
{
switch (pos)
{
case 0:
if ((active0 & 0x800000000000000L) != 0L)
{
jjmatchedKind = 58;
return -1;
}
return -1;
case 1:
if ((active0 & 0x800000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 58;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 2:
if ((active0 & 0x800000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 58;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 3:
if ((active0 & 0x800000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 58;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 4:
if ((active0 & 0x800000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 58;
jjmatchedPos = 0;
}
return -1;
}
return -1;
default :
return -1;
}
}
private final int jjStartNfa_3(int pos, long active0)
{
return jjMoveNfa_3(jjStopStringLiteralDfa_3(pos, active0), pos + 1);
}
private int jjMoveStringLiteralDfa0_3()
{
switch(curChar)
{
case 60:
return jjMoveStringLiteralDfa1_3(0x800000000000000L);
default :
return jjMoveNfa_3(1, 0);
}
}
private int jjMoveStringLiteralDfa1_3(long active0)
{
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_3(0, active0);
return 1;
}
switch(curChar)
{
case 47:
return jjMoveStringLiteralDfa2_3(active0, 0x800000000000000L);
default :
break;
}
return jjStartNfa_3(0, active0);
}
private int jjMoveStringLiteralDfa2_3(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_3(0, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_3(1, active0);
return 2;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa3_3(active0, 0x800000000000000L);
default :
break;
}
return jjStartNfa_3(1, active0);
}
private int jjMoveStringLiteralDfa3_3(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_3(1, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_3(2, active0);
return 3;
}
switch(curChar)
{
case 74:
case 106:
return jjMoveStringLiteralDfa4_3(active0, 0x800000000000000L);
default :
break;
}
return jjStartNfa_3(2, active0);
}
private int jjMoveStringLiteralDfa4_3(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_3(2, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_3(3, active0);
return 4;
}
switch(curChar)
{
case 66:
case 98:
return jjMoveStringLiteralDfa5_3(active0, 0x800000000000000L);
default :
break;
}
return jjStartNfa_3(3, active0);
}
private int jjMoveStringLiteralDfa5_3(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_3(3, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_3(4, active0);
return 5;
}
switch(curChar)
{
case 62:
if ((active0 & 0x800000000000000L) != 0L)
return jjStopAtPos(5, 59);
break;
default :
break;
}
return jjStartNfa_3(4, active0);
}
private int jjMoveNfa_3(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 2;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 1:
if ((0xefffffffffffffffL & l) != 0L)
{
if (kind > 58)
kind = 58;
jjCheckNAdd(0);
}
else if (curChar == 60)
{
if (kind > 58)
kind = 58;
}
break;
case 0:
if ((0xefffffffffffffffL & l) == 0L)
break;
kind = 58;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 0:
kind = 58;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 0:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 58)
kind = 58;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 2 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private final int jjStopStringLiteralDfa_0(int pos, long active0)
{
switch (pos)
{
case 0:
if ((active0 & 0x1ffbfff3e38L) != 0L)
{
jjmatchedKind = 53;
return 42;
}
if ((active0 & 0x80000000000L) != 0L)
return 171;
return -1;
case 1:
if ((active0 & 0x10012a60000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return 72;
}
if ((active0 & 0x38L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return -1;
}
if ((active0 & 0xffad593e00L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return 58;
}
return -1;
case 2:
if ((active0 & 0x38L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return -1;
}
if ((active0 & 0xffad593e00L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return 57;
}
if ((active0 & 0x10012a60000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return 71;
}
return -1;
case 3:
if ((active0 & 0x800000000L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 3;
return 78;
}
if ((active0 & 0x10012a60000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return 70;
}
if ((active0 & 0x3600L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 3;
return 56;
}
if ((active0 & 0x38L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return -1;
}
if ((active0 & 0xf7ad590800L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 3;
return 136;
}
return -1;
case 4:
if ((active0 & 0x3000L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 4;
return 55;
}
if ((active0 & 0x10000L) != 0L)
return 136;
if ((active0 & 0xffad580e00L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 4;
return 136;
}
if ((active0 & 0x18L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return -1;
}
if ((active0 & 0x10012a60000L) != 0L)
{
jjmatchedKind = 42;
jjmatchedPos = 4;
return 139;
}
return -1;
case 5:
if ((active0 & 0x400000000L) != 0L)
return 136;
if ((active0 & 0x10L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return -1;
}
if ((active0 & 0xfbad583e00L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 5;
return 136;
}
if ((active0 & 0x10012a60000L) != 0L)
{
jjmatchedKind = 42;
jjmatchedPos = 5;
return 139;
}
return -1;
case 6:
if ((active0 & 0xdba8083e00L) != 0L)
{
if (jjmatchedPos != 6)
{
jjmatchedKind = 41;
jjmatchedPos = 6;
}
return 136;
}
if ((active0 & 0x10012a40000L) != 0L)
{
if (jjmatchedPos != 6)
{
jjmatchedKind = 42;
jjmatchedPos = 6;
}
return 139;
}
if ((active0 & 0x2005500000L) != 0L)
return 136;
if ((active0 & 0x10L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 7:
if ((active0 & 0x80000600L) != 0L)
return 136;
if ((active0 & 0xdb28483800L) != 0L)
{
if (jjmatchedPos != 7)
{
jjmatchedKind = 41;
jjmatchedPos = 7;
}
return 136;
}
if ((active0 & 0x10L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 53;
jjmatchedPos = 0;
}
return -1;
}
if ((active0 & 0x10012a00000L) != 0L)
{
if (jjmatchedPos != 7)
{
jjmatchedKind = 42;
jjmatchedPos = 7;
}
return 139;
}
if ((active0 & 0x40000L) != 0L)
return 139;
return -1;
case 8:
if ((active0 & 0x10010800000L) != 0L)
{
jjmatchedKind = 42;
jjmatchedPos = 8;
return 139;
}
if ((active0 & 0xa28002000L) != 0L)
return 136;
if ((active0 & 0xd100481c00L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 8;
return 136;
}
return -1;
case 9:
if ((active0 & 0xd100400c00L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 9;
return 136;
}
if ((active0 & 0x81000L) != 0L)
return 136;
if ((active0 & 0x10010800000L) != 0L)
{
jjmatchedKind = 42;
jjmatchedPos = 9;
return 139;
}
return -1;
case 10:
if ((active0 & 0x10000800000L) != 0L)
{
jjmatchedKind = 42;
jjmatchedPos = 10;
return 139;
}
if ((active0 & 0x1100000400L) != 0L)
return 136;
if ((active0 & 0xc000400800L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 10;
return 136;
}
return -1;
case 11:
if ((active0 & 0x8000000000L) != 0L)
return 136;
if ((active0 & 0x10000800000L) != 0L)
{
jjmatchedKind = 42;
jjmatchedPos = 11;
return 139;
}
if ((active0 & 0x4000400800L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 11;
return 136;
}
return -1;
case 12:
if ((active0 & 0x4000000000L) != 0L)
{
jjmatchedKind = 41;
jjmatchedPos = 12;
return 136;
}
if ((active0 & 0x400800L) != 0L)
return 136;
if ((active0 & 0x10000800000L) != 0L)
{
jjmatchedKind = 42;
jjmatchedPos = 12;
return 139;
}
return -1;
case 13:
if ((active0 & 0x800000L) != 0L)
{
jjmatchedKind = 42;
jjmatchedPos = 13;
return 139;
}
if ((active0 & 0x4000000000L) != 0L)
return 136;
return -1;
default :
return -1;
}
}
private final int jjStartNfa_0(int pos, long active0)
{
return jjMoveNfa_0(jjStopStringLiteralDfa_0(pos, active0), pos + 1);
}
private int jjMoveStringLiteralDfa0_0()
{
switch(curChar)
{
case 35:
return jjStartNfaWithStates_0(0, 43, 171);
case 60:
return jjMoveStringLiteralDfa1_0(0x1ffbfff3e38L);
default :
return jjMoveNfa_0(3, 0);
}
}
private int jjMoveStringLiteralDfa1_0(long active0)
{
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(0, active0);
return 1;
}
switch(curChar)
{
case 47:
return jjMoveStringLiteralDfa2_0(active0, 0x10012a60000L);
case 66:
case 98:
return jjMoveStringLiteralDfa2_0(active0, 0x8L);
case 69:
case 101:
return jjMoveStringLiteralDfa2_0(active0, 0x20L);
case 73:
case 105:
return jjMoveStringLiteralDfa2_0(active0, 0xffad593e00L);
case 83:
case 115:
return jjMoveStringLiteralDfa2_0(active0, 0x10L);
default :
break;
}
return jjStartNfa_0(0, active0);
}
private int jjMoveStringLiteralDfa2_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(0, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(1, active0);
return 2;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa3_0(active0, 0x18L);
case 73:
case 105:
return jjMoveStringLiteralDfa3_0(active0, 0x10012a60000L);
case 74:
case 106:
return jjMoveStringLiteralDfa3_0(active0, 0x20L);
case 83:
case 115:
return jjMoveStringLiteralDfa3_0(active0, 0xffad593e00L);
default :
break;
}
return jjStartNfa_0(1, active0);
}
private int jjMoveStringLiteralDfa3_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(1, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(2, active0);
return 3;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa4_0(active0, 0x8L);
case 66:
case 98:
if ((active0 & 0x20L) != 0L)
return jjStopAtPos(3, 5);
return jjMoveStringLiteralDfa4_0(active0, 0x800000000L);
case 67:
case 99:
return jjMoveStringLiteralDfa4_0(active0, 0x3600L);
case 68:
case 100:
return jjMoveStringLiteralDfa4_0(active0, 0x800L);
case 70:
case 102:
return jjMoveStringLiteralDfa4_0(active0, 0x5400000L);
case 73:
case 105:
return jjMoveStringLiteralDfa4_0(active0, 0x90000L);
case 76:
case 108:
return jjMoveStringLiteralDfa4_0(active0, 0x100000L);
case 77:
case 109:
return jjMoveStringLiteralDfa4_0(active0, 0x20000000L);
case 80:
case 112:
return jjMoveStringLiteralDfa4_0(active0, 0xd080000000L);
case 82:
case 114:
return jjMoveStringLiteralDfa4_0(active0, 0x108000010L);
case 83:
case 115:
return jjMoveStringLiteralDfa4_0(active0, 0x10612a60000L);
case 84:
case 116:
return jjMoveStringLiteralDfa4_0(active0, 0x2000000000L);
default :
break;
}
return jjStartNfa_0(2, active0);
}
private int jjMoveStringLiteralDfa4_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(2, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(3, active0);
return 4;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa5_0(active0, 0x600L);
case 69:
case 101:
return jjMoveStringLiteralDfa5_0(active0, 0x2708040000L);
case 70:
case 102:
if ((active0 & 0x10000L) != 0L)
return jjStartNfaWithStates_0(4, 16, 136);
return jjMoveStringLiteralDfa5_0(active0, 0x2800000L);
case 73:
case 105:
return jjMoveStringLiteralDfa5_0(active0, 0x1804420800L);
case 76:
case 108:
return jjMoveStringLiteralDfa5_0(active0, 0xc000200000L);
case 78:
case 110:
if ((active0 & 0x8L) != 0L)
return jjStopAtPos(4, 3);
return jjMoveStringLiteralDfa5_0(active0, 0x80000L);
case 79:
case 111:
return jjMoveStringLiteralDfa5_0(active0, 0x21103000L);
case 80:
case 112:
return jjMoveStringLiteralDfa5_0(active0, 0x10000000000L);
case 82:
case 114:
return jjMoveStringLiteralDfa5_0(active0, 0x90000000L);
case 86:
case 118:
return jjMoveStringLiteralDfa5_0(active0, 0x10L);
default :
break;
}
return jjStartNfa_0(3, active0);
}
private int jjMoveStringLiteralDfa5_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(3, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(4, active0);
return 5;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa6_0(active0, 0xc000000000L);
case 67:
case 99:
return jjMoveStringLiteralDfa6_0(active0, 0x80e00L);
case 68:
case 100:
return jjMoveStringLiteralDfa6_0(active0, 0x120000000L);
case 69:
case 101:
return jjMoveStringLiteralDfa6_0(active0, 0x10000000L);
case 70:
case 102:
return jjMoveStringLiteralDfa6_0(active0, 0x20000L);
case 73:
case 105:
return jjMoveStringLiteralDfa6_0(active0, 0x80800000L);
case 76:
case 108:
return jjMoveStringLiteralDfa6_0(active0, 0x10204440010L);
case 78:
case 110:
return jjMoveStringLiteralDfa6_0(active0, 0x808001000L);
case 79:
case 111:
return jjMoveStringLiteralDfa6_0(active0, 0x2302000L);
case 80:
case 112:
return jjMoveStringLiteralDfa6_0(active0, 0x1000000000L);
case 82:
case 114:
return jjMoveStringLiteralDfa6_0(active0, 0x1000000L);
case 84:
case 116:
if ((active0 & 0x400000000L) != 0L)
return jjStartNfaWithStates_0(5, 34, 136);
break;
case 88:
case 120:
return jjMoveStringLiteralDfa6_0(active0, 0x2000000000L);
default :
break;
}
return jjStartNfa_0(4, active0);
}
private int jjMoveStringLiteralDfa6_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(4, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(5, active0);
return 6;
}
switch(curChar)
{
case 62:
if ((active0 & 0x20000L) != 0L)
return jjStopAtPos(6, 17);
break;
case 65:
case 97:
return jjMoveStringLiteralDfa7_0(active0, 0x10800000000L);
case 67:
case 99:
return jjMoveStringLiteralDfa7_0(active0, 0xc000000000L);
case 68:
case 100:
return jjMoveStringLiteralDfa7_0(active0, 0x8000000L);
case 69:
case 101:
if ((active0 & 0x4000000L) != 0L)
{
jjmatchedKind = 26;
jjmatchedPos = 6;
}
return jjMoveStringLiteralDfa7_0(active0, 0x1200400010L);
case 72:
case 104:
return jjMoveStringLiteralDfa7_0(active0, 0x600L);
case 73:
case 105:
return jjMoveStringLiteralDfa7_0(active0, 0x100000000L);
case 75:
case 107:
return jjMoveStringLiteralDfa7_0(active0, 0x2000L);
case 76:
case 108:
return jjMoveStringLiteralDfa7_0(active0, 0x880000L);
case 77:
case 109:
if ((active0 & 0x1000000L) != 0L)
return jjStartNfaWithStates_0(6, 24, 136);
break;
case 78:
case 110:
return jjMoveStringLiteralDfa7_0(active0, 0x90000000L);
case 79:
case 111:
return jjMoveStringLiteralDfa7_0(active0, 0x200000L);
case 80:
case 112:
if ((active0 & 0x100000L) != 0L)
return jjStartNfaWithStates_0(6, 20, 136);
break;
case 82:
case 114:
return jjMoveStringLiteralDfa7_0(active0, 0x2000000L);
case 83:
case 115:
return jjMoveStringLiteralDfa7_0(active0, 0x40000L);
case 84:
case 116:
if ((active0 & 0x2000000000L) != 0L)
return jjStartNfaWithStates_0(6, 37, 136);
return jjMoveStringLiteralDfa7_0(active0, 0x1800L);
case 85:
case 117:
return jjMoveStringLiteralDfa7_0(active0, 0x20000000L);
default :
break;
}
return jjStartNfa_0(5, active0);
}
private int jjMoveStringLiteralDfa7_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(5, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(6, active0);
return 7;
}
switch(curChar)
{
case 66:
case 98:
return jjMoveStringLiteralDfa8_0(active0, 0x400000L);
case 67:
case 99:
return jjMoveStringLiteralDfa8_0(active0, 0x10200000000L);
case 68:
case 100:
return jjMoveStringLiteralDfa8_0(active0, 0x10000000L);
case 69:
case 101:
if ((active0 & 0x200L) != 0L)
{
jjmatchedKind = 9;
jjmatchedPos = 7;
}
else if ((active0 & 0x40000L) != 0L)
return jjStartNfaWithStates_0(7, 18, 139);
return jjMoveStringLiteralDfa8_0(active0, 0xc008801400L);
case 73:
case 105:
return jjMoveStringLiteralDfa8_0(active0, 0x2800L);
case 76:
case 108:
return jjMoveStringLiteralDfa8_0(active0, 0x1020000000L);
case 77:
case 109:
return jjMoveStringLiteralDfa8_0(active0, 0x2000000L);
case 80:
case 112:
return jjMoveStringLiteralDfa8_0(active0, 0x200000L);
case 82:
case 114:
return jjMoveStringLiteralDfa8_0(active0, 0x900000000L);
case 84:
case 116:
if ((active0 & 0x10L) != 0L)
return jjStopAtPos(7, 4);
else if ((active0 & 0x80000000L) != 0L)
return jjStartNfaWithStates_0(7, 31, 136);
break;
case 85:
case 117:
return jjMoveStringLiteralDfa8_0(active0, 0x80000L);
default :
break;
}
return jjStartNfa_0(6, active0);
}
private int jjMoveStringLiteralDfa8_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(6, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(7, active0);
return 8;
}
switch(curChar)
{
case 62:
if ((active0 & 0x200000L) != 0L)
return jjStopAtPos(8, 21);
else if ((active0 & 0x2000000L) != 0L)
return jjStopAtPos(8, 25);
break;
case 66:
case 98:
return jjMoveStringLiteralDfa9_0(active0, 0x800000L);
case 68:
case 100:
return jjMoveStringLiteralDfa9_0(active0, 0x80000L);
case 69:
case 101:
if ((active0 & 0x2000L) != 0L)
return jjStartNfaWithStates_0(8, 13, 136);
else if ((active0 & 0x20000000L) != 0L)
return jjStartNfaWithStates_0(8, 29, 136);
return jjMoveStringLiteralDfa9_0(active0, 0x10110000000L);
case 72:
case 104:
return jjMoveStringLiteralDfa9_0(active0, 0x4000000000L);
case 73:
case 105:
return jjMoveStringLiteralDfa9_0(active0, 0x1000000000L);
case 75:
case 107:
return jjMoveStringLiteralDfa9_0(active0, 0x400L);
case 77:
case 109:
return jjMoveStringLiteralDfa9_0(active0, 0x8000000000L);
case 78:
case 110:
return jjMoveStringLiteralDfa9_0(active0, 0x1000L);
case 79:
case 111:
return jjMoveStringLiteralDfa9_0(active0, 0x800L);
case 82:
case 114:
if ((active0 & 0x8000000L) != 0L)
return jjStartNfaWithStates_0(8, 27, 136);
break;
case 84:
case 116:
if ((active0 & 0x200000000L) != 0L)
return jjStartNfaWithStates_0(8, 33, 136);
break;
case 85:
case 117:
return jjMoveStringLiteralDfa9_0(active0, 0x400000L);
case 89:
case 121:
if ((active0 & 0x800000000L) != 0L)
return jjStartNfaWithStates_0(8, 35, 136);
break;
default :
break;
}
return jjStartNfa_0(7, active0);
}
private int jjMoveStringLiteralDfa9_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(7, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(8, active0);
return 9;
}
switch(curChar)
{
case 67:
case 99:
return jjMoveStringLiteralDfa10_0(active0, 0x100000000L);
case 69:
case 101:
if ((active0 & 0x80000L) != 0L)
return jjStartNfaWithStates_0(9, 19, 136);
return jjMoveStringLiteralDfa10_0(active0, 0x8000000400L);
case 77:
case 109:
return jjMoveStringLiteralDfa10_0(active0, 0x10000000000L);
case 78:
case 110:
return jjMoveStringLiteralDfa10_0(active0, 0x1000400800L);
case 79:
case 111:
return jjMoveStringLiteralDfa10_0(active0, 0x4000000000L);
case 82:
case 114:
return jjMoveStringLiteralDfa10_0(active0, 0x10000000L);
case 84:
case 116:
if ((active0 & 0x1000L) != 0L)
return jjStartNfaWithStates_0(9, 12, 136);
break;
case 85:
case 117:
return jjMoveStringLiteralDfa10_0(active0, 0x800000L);
default :
break;
}
return jjStartNfa_0(8, active0);
}
private int jjMoveStringLiteralDfa10_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(8, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(9, active0);
return 10;
}
switch(curChar)
{
case 62:
if ((active0 & 0x10000000L) != 0L)
return jjStopAtPos(10, 28);
break;
case 65:
case 97:
return jjMoveStringLiteralDfa11_0(active0, 0x800L);
case 68:
case 100:
return jjMoveStringLiteralDfa11_0(active0, 0x400000L);
case 69:
case 101:
if ((active0 & 0x1000000000L) != 0L)
return jjStartNfaWithStates_0(10, 36, 136);
return jjMoveStringLiteralDfa11_0(active0, 0x10000000000L);
case 76:
case 108:
return jjMoveStringLiteralDfa11_0(active0, 0x4000000000L);
case 78:
case 110:
return jjMoveStringLiteralDfa11_0(active0, 0x8000800000L);
case 84:
case 116:
if ((active0 & 0x100000000L) != 0L)
return jjStartNfaWithStates_0(10, 32, 136);
break;
case 89:
case 121:
if ((active0 & 0x400L) != 0L)
return jjStartNfaWithStates_0(10, 10, 136);
break;
default :
break;
}
return jjStartNfa_0(9, active0);
}
private int jjMoveStringLiteralDfa11_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(9, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(10, active0);
return 11;
}
switch(curChar)
{
case 68:
case 100:
return jjMoveStringLiteralDfa12_0(active0, 0x4000800000L);
case 76:
case 108:
return jjMoveStringLiteralDfa12_0(active0, 0x400000L);
case 78:
case 110:
return jjMoveStringLiteralDfa12_0(active0, 0x10000000000L);
case 82:
case 114:
return jjMoveStringLiteralDfa12_0(active0, 0x800L);
case 84:
case 116:
if ((active0 & 0x8000000000L) != 0L)
return jjStartNfaWithStates_0(11, 39, 136);
break;
default :
break;
}
return jjStartNfa_0(10, active0);
}
private int jjMoveStringLiteralDfa12_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(10, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(11, active0);
return 12;
}
switch(curChar)
{
case 69:
case 101:
if ((active0 & 0x400000L) != 0L)
return jjStartNfaWithStates_0(12, 22, 136);
return jjMoveStringLiteralDfa13_0(active0, 0x4000000000L);
case 76:
case 108:
return jjMoveStringLiteralDfa13_0(active0, 0x800000L);
case 84:
case 116:
return jjMoveStringLiteralDfa13_0(active0, 0x10000000000L);
case 89:
case 121:
if ((active0 & 0x800L) != 0L)
return jjStartNfaWithStates_0(12, 11, 136);
break;
default :
break;
}
return jjStartNfa_0(11, active0);
}
private int jjMoveStringLiteralDfa13_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(11, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(12, active0);
return 13;
}
switch(curChar)
{
case 62:
if ((active0 & 0x10000000000L) != 0L)
return jjStopAtPos(13, 40);
break;
case 69:
case 101:
return jjMoveStringLiteralDfa14_0(active0, 0x800000L);
case 82:
case 114:
if ((active0 & 0x4000000000L) != 0L)
return jjStartNfaWithStates_0(13, 38, 136);
break;
default :
break;
}
return jjStartNfa_0(12, active0);
}
private int jjMoveStringLiteralDfa14_0(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_0(12, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_0(13, active0);
return 14;
}
switch(curChar)
{
case 62:
if ((active0 & 0x800000L) != 0L)
return jjStopAtPos(14, 23);
break;
default :
break;
}
return jjStartNfa_0(13, active0);
}
private int jjStartNfaWithStates_0(int pos, int kind, int state)
{
jjmatchedKind = kind;
jjmatchedPos = pos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return pos + 1; }
return jjMoveNfa_0(state, pos + 1);
}
private int jjMoveNfa_0(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 171;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 42:
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 140;
else if (curChar == 33)
jjstateSet[jjnewStateCnt++] = 62;
else if (curChar == 37)
jjCheckNAddTwoStates(44, 45);
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 72;
else if (curChar == 33)
jjstateSet[jjnewStateCnt++] = 41;
break;
case 57:
case 136:
if ((0xaffffffaffffd9ffL & l) == 0L)
break;
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 78:
if ((0xaffffffaffffd9ffL & l) == 0L)
break;
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 56:
if ((0xaffffffaffffd9ffL & l) == 0L)
break;
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 171:
if ((0x3ff000100002600L & l) != 0L)
jjCheckNAddTwoStates(169, 170);
else if (curChar == 38)
{
if (kind > 52)
kind = 52;
}
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddStates(106, 108);
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddStates(109, 111);
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddTwoStates(19, 20);
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddTwoStates(163, 164);
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddStates(112, 114);
break;
case 3:
if ((0xfffffff7ffffffffL & l) != 0L)
{
if (kind > 53)
kind = 53;
}
else if (curChar == 35)
jjCheckNAddStates(115, 121);
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(4, 17);
else if (curChar == 34)
jjCheckNAddTwoStates(4, 155);
else if (curChar == 60)
jjAddStates(122, 137);
else if (curChar == 38)
jjstateSet[jjnewStateCnt++] = 21;
else if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 2;
break;
case 55:
if ((0xaffffffaffffd9ffL & l) == 0L)
break;
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 70:
case 139:
if ((0xaffffffaffffd9ffL & l) == 0L)
break;
if (kind > 42)
kind = 42;
jjCheckNAdd(139);
break;
case 0:
if (curChar == 62 && kind > 7)
kind = 7;
break;
case 1:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 0;
break;
case 2:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 1;
break;
case 4:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(4, 17);
break;
case 6:
if ((0x100002600L & l) != 0L)
jjAddStates(138, 139);
break;
case 7:
if (curChar == 61)
jjCheckNAddTwoStates(8, 9);
break;
case 8:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(8, 9);
break;
case 9:
if (curChar == 34)
jjCheckNAddTwoStates(10, 11);
break;
case 10:
if ((0xfffffffbffffffffL & l) != 0L)
jjCheckNAddTwoStates(10, 11);
break;
case 11:
if (curChar == 34 && kind > 44)
kind = 44;
break;
case 19:
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddTwoStates(19, 20);
break;
case 20:
if (curChar == 59 && kind > 48)
kind = 48;
break;
case 21:
if (curChar == 35)
jjstateSet[jjnewStateCnt++] = 18;
break;
case 22:
if (curChar == 38)
jjstateSet[jjnewStateCnt++] = 21;
break;
case 23:
if (curChar == 35)
jjCheckNAdd(24);
break;
case 24:
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddStates(140, 142);
break;
case 25:
if (curChar == 59)
jjCheckNAdd(27);
break;
case 26:
if (curChar == 34 && kind > 49)
kind = 49;
break;
case 28:
if (curChar == 34)
jjstateSet[jjnewStateCnt++] = 23;
break;
case 30:
if ((0xfffffff7ffffffffL & l) != 0L && kind > 53)
kind = 53;
break;
case 31:
if (curChar == 60)
jjAddStates(122, 137);
break;
case 32:
if (curChar == 35)
jjCheckNAddTwoStates(33, 34);
break;
case 33:
if ((0xffffdfffffffffffL & l) != 0L)
jjCheckNAddTwoStates(33, 34);
break;
case 34:
if (curChar == 45)
jjCheckNAddStates(143, 146);
break;
case 35:
if (curChar == 45)
jjCheckNAddTwoStates(35, 36);
break;
case 36:
if ((0xbfffffffffffffffL & l) != 0L)
jjCheckNAddTwoStates(33, 34);
break;
case 37:
if (curChar == 45)
jjCheckNAddTwoStates(37, 39);
break;
case 38:
if (curChar == 62 && kind > 1)
kind = 1;
break;
case 39:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 38;
break;
case 40:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 32;
break;
case 41:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 40;
break;
case 43:
if (curChar == 37)
jjCheckNAddTwoStates(44, 45);
break;
case 44:
if ((0xffffffdfffffffffL & l) != 0L)
jjCheckNAddTwoStates(44, 45);
break;
case 45:
if (curChar == 37)
jjCheckNAddStates(147, 150);
break;
case 46:
if (curChar == 37)
jjCheckNAddTwoStates(46, 47);
break;
case 47:
if ((0xbfffffffffffffffL & l) != 0L)
jjCheckNAddTwoStates(44, 45);
break;
case 48:
if (curChar == 37)
jjCheckNAddTwoStates(48, 49);
break;
case 49:
if (curChar == 62 && kind > 2)
kind = 2;
break;
case 50:
if (curChar == 62 && kind > 6)
kind = 6;
break;
case 60:
if (curChar == 45 && kind > 6)
kind = 6;
break;
case 61:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 60;
break;
case 62:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 61;
break;
case 63:
if (curChar == 33)
jjstateSet[jjnewStateCnt++] = 62;
break;
case 73:
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 72;
break;
case 74:
if (curChar == 62 && kind > 8)
kind = 8;
break;
case 83:
if ((0x100002600L & l) != 0L)
jjAddStates(151, 152);
break;
case 84:
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 74;
break;
case 91:
if (curChar == 62 && kind > 14)
kind = 14;
break;
case 99:
if ((0x100002600L & l) != 0L)
jjAddStates(153, 154);
break;
case 100:
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 91;
break;
case 120:
if (curChar == 62 && kind > 30)
kind = 30;
break;
case 128:
if ((0x100002600L & l) != 0L)
jjAddStates(155, 156);
break;
case 129:
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 120;
break;
case 141:
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 140;
break;
case 142:
if (curChar == 62)
jjCheckNAddTwoStates(143, 149);
break;
case 143:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(143, 149);
break;
case 144:
if (curChar == 62 && kind > 45)
kind = 45;
break;
case 149:
if (curChar == 60)
jjstateSet[jjnewStateCnt++] = 148;
break;
case 154:
if (curChar == 34)
jjCheckNAddTwoStates(4, 155);
break;
case 155:
if (curChar == 35)
jjCheckNAdd(156);
break;
case 156:
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddStates(109, 111);
break;
case 157:
if ((0x100000200L & l) != 0L)
jjCheckNAddTwoStates(157, 158);
break;
case 158:
if (curChar == 61 && kind > 50)
kind = 50;
break;
case 159:
if (curChar == 35)
jjCheckNAddStates(115, 121);
break;
case 160:
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddStates(112, 114);
break;
case 161:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(161, 162);
break;
case 162:
if ((0x4000000400000000L & l) != 0L && kind > 46)
kind = 46;
break;
case 163:
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddTwoStates(163, 164);
break;
case 164:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(164, 165);
break;
case 165:
if ((0x3ff000000000000L & l) != 0L && kind > 47)
kind = 47;
break;
case 166:
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddStates(106, 108);
break;
case 167:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(167, 168);
break;
case 169:
if ((0x3ff000100002600L & l) != 0L)
jjCheckNAddTwoStates(169, 170);
break;
case 170:
if (curChar == 38 && kind > 52)
kind = 52;
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 42:
if ((0x10000000100L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 152;
else if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 135;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 133;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 125;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 118;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 111;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 104;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 96;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 89;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 80;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 58;
break;
case 57:
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 131;
else if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 116;
else if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 87;
else if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 56;
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 123;
else if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 109;
else if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 78;
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 102;
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 94;
break;
case 78:
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 86;
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 77;
break;
case 56:
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 55;
break;
case 71:
if ((0x8000000080000L & l) != 0L)
jjCheckNAdd(139);
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 70;
break;
case 171:
if ((0x7fffffe07fffffeL & l) != 0L)
jjCheckNAddStates(109, 111);
if ((0x7fffffe07fffffeL & l) != 0L)
jjCheckNAddTwoStates(163, 164);
if ((0x7fffffe07fffffeL & l) != 0L)
jjCheckNAddStates(112, 114);
if ((0x7e0000007eL & l) != 0L)
jjCheckNAddTwoStates(169, 170);
if ((0x7e0000007eL & l) != 0L)
jjCheckNAddStates(106, 108);
if ((0x7e0000007eL & l) != 0L)
jjCheckNAddTwoStates(19, 20);
break;
case 3:
if (kind > 53)
kind = 53;
if (curChar == 92)
jjstateSet[jjnewStateCnt++] = 28;
break;
case 55:
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 54;
break;
case 58:
if ((0x8000000080000L & l) != 0L)
jjCheckNAdd(136);
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 132;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 124;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 117;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 110;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 103;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 95;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 88;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 79;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 57;
break;
case 72:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 138;
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 71;
break;
case 70:
if (kind > 42)
kind = 42;
jjCheckNAdd(139);
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 69;
break;
case 5:
if ((0x80000000800000L & l) != 0L)
jjAddStates(138, 139);
break;
case 10:
jjAddStates(157, 158);
break;
case 12:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 5;
break;
case 13:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 12;
break;
case 14:
if ((0x40000000400000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 13;
break;
case 15:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 14;
break;
case 16:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 15;
break;
case 17:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 16;
break;
case 18:
if ((0x100000001000000L & l) != 0L)
jjCheckNAdd(19);
break;
case 19:
if ((0x7e0000007eL & l) != 0L)
jjCheckNAddTwoStates(19, 20);
break;
case 24:
if ((0x7e0000007eL & l) != 0L)
jjAddStates(140, 142);
break;
case 27:
if (curChar == 92)
jjstateSet[jjnewStateCnt++] = 26;
break;
case 29:
if (curChar == 92)
jjstateSet[jjnewStateCnt++] = 28;
break;
case 30:
if (kind > 53)
kind = 53;
break;
case 33:
case 36:
jjCheckNAddTwoStates(33, 34);
break;
case 44:
case 47:
jjCheckNAddTwoStates(44, 45);
break;
case 51:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 50;
break;
case 52:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 51;
break;
case 53:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 52;
break;
case 54:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 53;
break;
case 59:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 58;
break;
case 64:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 0;
break;
case 65:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 64;
break;
case 66:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 65;
break;
case 67:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 66;
break;
case 68:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 67;
break;
case 69:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 68;
break;
case 75:
if ((0x80000000800L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 74;
break;
case 76:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 75;
break;
case 77:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 76;
break;
case 79:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 78;
break;
case 80:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 79;
break;
case 81:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 80;
break;
case 82:
if ((0x80000000800L & l) != 0L)
jjAddStates(151, 152);
break;
case 85:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 82;
break;
case 86:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 85;
break;
case 87:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 86;
break;
case 88:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 87;
break;
case 89:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 88;
break;
case 90:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 89;
break;
case 92:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 91;
break;
case 93:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 92;
break;
case 94:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 93;
break;
case 95:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 94;
break;
case 96:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 95;
break;
case 97:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 96;
break;
case 98:
if ((0x2000000020L & l) != 0L)
jjAddStates(153, 154);
break;
case 101:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 98;
break;
case 102:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 101;
break;
case 103:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 102;
break;
case 104:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 103;
break;
case 105:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 104;
break;
case 106:
if ((0x4000000040L & l) != 0L && kind > 15)
kind = 15;
break;
case 107:
case 113:
if ((0x20000000200L & l) != 0L)
jjCheckNAdd(106);
break;
case 108:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 107;
break;
case 109:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 108;
break;
case 110:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 109;
break;
case 111:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 110;
break;
case 112:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 111;
break;
case 114:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 113;
break;
case 115:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 114;
break;
case 116:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 115;
break;
case 117:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 116;
break;
case 118:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 117;
break;
case 119:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 118;
break;
case 121:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 120;
break;
case 122:
if ((0x100000001000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 121;
break;
case 123:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 122;
break;
case 124:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 123;
break;
case 125:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 124;
break;
case 126:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 125;
break;
case 127:
if ((0x10000000100000L & l) != 0L)
jjAddStates(155, 156);
break;
case 130:
if ((0x100000001000000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 127;
break;
case 131:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 130;
break;
case 132:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 131;
break;
case 133:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 132;
break;
case 134:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 133;
break;
case 135:
if ((0x8000000080000L & l) != 0L)
jjCheckNAdd(136);
break;
case 136:
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 137:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 135;
break;
case 138:
if ((0x8000000080000L & l) != 0L)
jjCheckNAdd(139);
break;
case 139:
if (kind > 42)
kind = 42;
jjCheckNAdd(139);
break;
case 140:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 138;
break;
case 145:
if ((0x1000000010L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 144;
break;
case 146:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 145;
break;
case 147:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 146;
break;
case 148:
if ((0x10000000100L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 147;
break;
case 150:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 142;
break;
case 151:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 150;
break;
case 152:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 151;
break;
case 153:
if ((0x10000000100L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 152;
break;
case 156:
if ((0x7fffffe07fffffeL & l) != 0L)
jjCheckNAddStates(109, 111);
break;
case 160:
if ((0x7fffffe07fffffeL & l) != 0L)
jjCheckNAddStates(112, 114);
break;
case 163:
if ((0x7fffffe07fffffeL & l) != 0L)
jjCheckNAddTwoStates(163, 164);
break;
case 165:
if ((0x7fffffe07fffffeL & l) != 0L && kind > 47)
kind = 47;
break;
case 166:
if ((0x7e0000007eL & l) != 0L)
jjCheckNAddStates(106, 108);
break;
case 168:
if (curChar == 125 && kind > 51)
kind = 51;
break;
case 169:
if ((0x7e0000007eL & l) != 0L)
jjCheckNAddTwoStates(169, 170);
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 57:
case 136:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 78:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 56:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 3:
if (jjCanMove_0(hiByte, i1, i2, l1, l2) && kind > 53)
kind = 53;
break;
case 55:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 41)
kind = 41;
jjCheckNAdd(136);
break;
case 70:
case 139:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 42)
kind = 42;
jjCheckNAdd(139);
break;
case 10:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjAddStates(157, 158);
break;
case 33:
case 36:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjCheckNAddTwoStates(33, 34);
break;
case 44:
case 47:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjCheckNAddTwoStates(44, 45);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 171 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private final int jjStopStringLiteralDfa_4(int pos, long active0)
{
switch (pos)
{
case 0:
if ((active0 & 0x2000000000000000L) != 0L)
{
jjmatchedKind = 60;
return -1;
}
return -1;
case 1:
if ((active0 & 0x2000000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 60;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 2:
if ((active0 & 0x2000000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 60;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 3:
if ((active0 & 0x2000000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 60;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 4:
if ((active0 & 0x2000000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 60;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 5:
if ((active0 & 0x2000000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 60;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 6:
if ((active0 & 0x2000000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 60;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 7:
if ((active0 & 0x2000000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 60;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 8:
if ((active0 & 0x2000000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 60;
jjmatchedPos = 0;
}
return -1;
}
return -1;
default :
return -1;
}
}
private final int jjStartNfa_4(int pos, long active0)
{
return jjMoveNfa_4(jjStopStringLiteralDfa_4(pos, active0), pos + 1);
}
private int jjMoveStringLiteralDfa0_4()
{
switch(curChar)
{
case 60:
return jjMoveStringLiteralDfa1_4(0x2000000000000000L);
default :
return jjMoveNfa_4(1, 0);
}
}
private int jjMoveStringLiteralDfa1_4(long active0)
{
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(0, active0);
return 1;
}
switch(curChar)
{
case 47:
return jjMoveStringLiteralDfa2_4(active0, 0x2000000000000000L);
default :
break;
}
return jjStartNfa_4(0, active0);
}
private int jjMoveStringLiteralDfa2_4(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_4(0, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(1, active0);
return 2;
}
switch(curChar)
{
case 83:
case 115:
return jjMoveStringLiteralDfa3_4(active0, 0x2000000000000000L);
default :
break;
}
return jjStartNfa_4(1, active0);
}
private int jjMoveStringLiteralDfa3_4(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_4(1, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(2, active0);
return 3;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa4_4(active0, 0x2000000000000000L);
default :
break;
}
return jjStartNfa_4(2, active0);
}
private int jjMoveStringLiteralDfa4_4(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_4(2, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(3, active0);
return 4;
}
switch(curChar)
{
case 82:
case 114:
return jjMoveStringLiteralDfa5_4(active0, 0x2000000000000000L);
default :
break;
}
return jjStartNfa_4(3, active0);
}
private int jjMoveStringLiteralDfa5_4(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_4(3, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(4, active0);
return 5;
}
switch(curChar)
{
case 86:
case 118:
return jjMoveStringLiteralDfa6_4(active0, 0x2000000000000000L);
default :
break;
}
return jjStartNfa_4(4, active0);
}
private int jjMoveStringLiteralDfa6_4(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_4(4, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(5, active0);
return 6;
}
switch(curChar)
{
case 76:
case 108:
return jjMoveStringLiteralDfa7_4(active0, 0x2000000000000000L);
default :
break;
}
return jjStartNfa_4(5, active0);
}
private int jjMoveStringLiteralDfa7_4(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_4(5, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(6, active0);
return 7;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa8_4(active0, 0x2000000000000000L);
default :
break;
}
return jjStartNfa_4(6, active0);
}
private int jjMoveStringLiteralDfa8_4(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_4(6, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(7, active0);
return 8;
}
switch(curChar)
{
case 84:
case 116:
return jjMoveStringLiteralDfa9_4(active0, 0x2000000000000000L);
default :
break;
}
return jjStartNfa_4(7, active0);
}
private int jjMoveStringLiteralDfa9_4(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_4(7, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_4(8, active0);
return 9;
}
switch(curChar)
{
case 62:
if ((active0 & 0x2000000000000000L) != 0L)
return jjStopAtPos(9, 61);
break;
default :
break;
}
return jjStartNfa_4(8, active0);
}
private int jjMoveNfa_4(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 2;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 1:
if ((0xefffffffffffffffL & l) != 0L)
{
if (kind > 60)
kind = 60;
jjCheckNAdd(0);
}
else if (curChar == 60)
{
if (kind > 60)
kind = 60;
}
break;
case 0:
if ((0xefffffffffffffffL & l) == 0L)
break;
kind = 60;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 0:
kind = 60;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 0:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 60)
kind = 60;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 2 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private int jjMoveStringLiteralDfa0_9()
{
return jjMoveNfa_9(0, 0);
}
private int jjMoveNfa_9(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 1;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 0:
kind = 222;
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 0:
kind = 222;
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 0:
if (jjCanMove_0(hiByte, i1, i2, l1, l2) && kind > 222)
kind = 222;
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 1 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private final int jjStopStringLiteralDfa_2(int pos, long active0)
{
switch (pos)
{
case 0:
if ((active0 & 0x200000000000000L) != 0L)
{
jjmatchedKind = 56;
return -1;
}
return -1;
case 1:
if ((active0 & 0x200000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 56;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 2:
if ((active0 & 0x200000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 56;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 3:
if ((active0 & 0x200000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 56;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 4:
if ((active0 & 0x200000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 56;
jjmatchedPos = 0;
}
return -1;
}
return -1;
case 5:
if ((active0 & 0x200000000000000L) != 0L)
{
if (jjmatchedPos == 0)
{
jjmatchedKind = 56;
jjmatchedPos = 0;
}
return -1;
}
return -1;
default :
return -1;
}
}
private final int jjStartNfa_2(int pos, long active0)
{
return jjMoveNfa_2(jjStopStringLiteralDfa_2(pos, active0), pos + 1);
}
private int jjMoveStringLiteralDfa0_2()
{
switch(curChar)
{
case 60:
return jjMoveStringLiteralDfa1_2(0x200000000000000L);
default :
return jjMoveNfa_2(1, 0);
}
}
private int jjMoveStringLiteralDfa1_2(long active0)
{
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_2(0, active0);
return 1;
}
switch(curChar)
{
case 47:
return jjMoveStringLiteralDfa2_2(active0, 0x200000000000000L);
default :
break;
}
return jjStartNfa_2(0, active0);
}
private int jjMoveStringLiteralDfa2_2(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_2(0, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_2(1, active0);
return 2;
}
switch(curChar)
{
case 66:
case 98:
return jjMoveStringLiteralDfa3_2(active0, 0x200000000000000L);
default :
break;
}
return jjStartNfa_2(1, active0);
}
private int jjMoveStringLiteralDfa3_2(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_2(1, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_2(2, active0);
return 3;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa4_2(active0, 0x200000000000000L);
default :
break;
}
return jjStartNfa_2(2, active0);
}
private int jjMoveStringLiteralDfa4_2(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_2(2, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_2(3, active0);
return 4;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa5_2(active0, 0x200000000000000L);
default :
break;
}
return jjStartNfa_2(3, active0);
}
private int jjMoveStringLiteralDfa5_2(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_2(3, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_2(4, active0);
return 5;
}
switch(curChar)
{
case 78:
case 110:
return jjMoveStringLiteralDfa6_2(active0, 0x200000000000000L);
default :
break;
}
return jjStartNfa_2(4, active0);
}
private int jjMoveStringLiteralDfa6_2(long old0, long active0)
{
if (((active0 &= old0)) == 0L)
return jjStartNfa_2(4, old0);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_2(5, active0);
return 6;
}
switch(curChar)
{
case 62:
if ((active0 & 0x200000000000000L) != 0L)
return jjStopAtPos(6, 57);
break;
default :
break;
}
return jjStartNfa_2(5, active0);
}
private int jjMoveNfa_2(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 2;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 1:
if ((0xefffffffffffffffL & l) != 0L)
{
if (kind > 56)
kind = 56;
jjCheckNAdd(0);
}
else if (curChar == 60)
{
if (kind > 56)
kind = 56;
}
break;
case 0:
if ((0xefffffffffffffffL & l) == 0L)
break;
kind = 56;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 0:
kind = 56;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 0:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 56)
kind = 56;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 2 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private final int jjStopStringLiteralDfa_8(int pos, long active0, long active1, long active2, long active3)
{
switch (pos)
{
case 0:
if ((active2 & 0xffffdf0000000000L) != 0L || (active3 & 0x7ffbfL) != 0L)
{
jjmatchedKind = 217;
return 34;
}
if ((active2 & 0x5000000000L) != 0L)
return 6;
if ((active3 & 0x40L) != 0L)
{
jjmatchedKind = 217;
return 60;
}
if ((active2 & 0x8000000000L) != 0L)
return 29;
return -1;
case 1:
if ((active2 & 0xb0000000000L) != 0L)
return 34;
if ((active3 & 0x40L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 1;
return 59;
}
if ((active2 & 0xffffd40000000000L) != 0L || (active3 & 0x7ffbfL) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 1;
return 34;
}
return -1;
case 2:
if ((active2 & 0x6106140000000000L) != 0L)
return 34;
if ((active2 & 0x9ef9c00000000000L) != 0L || (active3 & 0x7ffbfL) != 0L)
{
if (jjmatchedPos != 2)
{
jjmatchedKind = 217;
jjmatchedPos = 2;
}
return 34;
}
if ((active3 & 0x40L) != 0L)
{
if (jjmatchedPos != 2)
{
jjmatchedKind = 217;
jjmatchedPos = 2;
}
return 58;
}
return -1;
case 3:
if ((active2 & 0x400000000000000L) != 0L)
return 34;
if ((active2 & 0x9afdc00000000000L) != 0L || (active3 & 0x7ffffL) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 3;
return 34;
}
return -1;
case 4:
if ((active2 & 0x1804000000000000L) != 0L || (active3 & 0x100L) != 0L)
return 34;
if ((active2 & 0x82f9c00000000000L) != 0L || (active3 & 0x7feffL) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 4;
return 34;
}
return -1;
case 5:
if ((active2 & 0x8000000000000L) != 0L)
return 34;
if ((active2 & 0x82f1c00000000000L) != 0L || (active3 & 0x7feffL) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 5;
return 34;
}
return -1;
case 6:
if ((active2 & 0x20000000000000L) != 0L || (active3 & 0x4c5L) != 0L)
return 34;
if ((active2 & 0x82d1c00000000000L) != 0L || (active3 & 0x7fa3aL) != 0L)
{
if (jjmatchedPos != 6)
{
jjmatchedKind = 217;
jjmatchedPos = 6;
}
return 34;
}
return -1;
case 7:
if ((active2 & 0x1000000000000L) != 0L || (active3 & 0x1020L) != 0L)
return 34;
if ((active2 & 0x82d0c00000000000L) != 0L || (active3 & 0x7ee1aL) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 7;
return 34;
}
return -1;
case 8:
if ((active2 & 0x8050800000000000L) != 0L || (active3 & 0x20400L) != 0L)
return 34;
if ((active2 & 0x280400000000000L) != 0L || (active3 & 0x5ea1aL) != 0L)
{
if (jjmatchedPos != 8)
{
jjmatchedKind = 217;
jjmatchedPos = 8;
}
return 34;
}
return -1;
case 9:
if ((active3 & 0x2802L) != 0L)
return 34;
if ((active2 & 0x280400000000000L) != 0L || (active3 & 0x7c218L) != 0L)
{
if (jjmatchedPos != 9)
{
jjmatchedKind = 217;
jjmatchedPos = 9;
}
return 34;
}
return -1;
case 10:
if ((active2 & 0x200000000000000L) != 0L || (active3 & 0x40000L) != 0L)
return 34;
if ((active2 & 0x80400000000000L) != 0L || (active3 & 0x3ca18L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 10;
return 34;
}
return -1;
case 11:
if ((active2 & 0x80400000000000L) != 0L || (active3 & 0x18800L) != 0L)
return 34;
if ((active3 & 0x24218L) != 0L)
{
if (jjmatchedPos != 11)
{
jjmatchedKind = 217;
jjmatchedPos = 11;
}
return 34;
}
return -1;
case 12:
if ((active3 & 0x20000L) != 0L)
return 34;
if ((active3 & 0x14218L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 12;
return 34;
}
return -1;
case 13:
if ((active3 & 0x10218L) != 0L)
return 34;
if ((active3 & 0x4000L) != 0L)
{
if (jjmatchedPos != 13)
{
jjmatchedKind = 217;
jjmatchedPos = 13;
}
return 34;
}
return -1;
case 14:
if ((active3 & 0x4010L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 14;
return 34;
}
return -1;
case 15:
if ((active3 & 0x10L) != 0L)
return 34;
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 15;
return 34;
}
return -1;
case 16:
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 16;
return 34;
}
return -1;
case 17:
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 17;
return 34;
}
return -1;
case 18:
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 18;
return 34;
}
return -1;
case 19:
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 19;
return 34;
}
return -1;
case 20:
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 20;
return 34;
}
return -1;
case 21:
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 21;
return 34;
}
return -1;
case 22:
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 22;
return 34;
}
return -1;
case 23:
if ((active3 & 0x4000L) != 0L)
{
jjmatchedKind = 217;
jjmatchedPos = 23;
return 34;
}
return -1;
default :
return -1;
}
}
private final int jjStartNfa_8(int pos, long active0, long active1, long active2, long active3)
{
return jjMoveNfa_8(jjStopStringLiteralDfa_8(pos, active0, active1, active2, active3), pos + 1);
}
private int jjMoveStringLiteralDfa0_8()
{
switch(curChar)
{
case 33:
return jjMoveStringLiteralDfa1_8(0x400000000L, 0x0L);
case 35:
return jjStopAtPos(0, 211);
case 37:
return jjStopAtPos(0, 160);
case 40:
return jjStopAtPos(0, 152);
case 41:
return jjStopAtPos(0, 153);
case 42:
return jjStopAtPos(0, 158);
case 43:
return jjStopAtPos(0, 156);
case 44:
return jjStopAtPos(0, 216);
case 45:
return jjStopAtPos(0, 157);
case 46:
return jjStartNfaWithStates_8(0, 167, 29);
case 47:
return jjStopAtPos(0, 159);
case 58:
return jjStopAtPos(0, 215);
case 60:
jjmatchedKind = 166;
return jjMoveStringLiteralDfa1_8(0x1000000000L, 0x0L);
case 61:
return jjMoveStringLiteralDfa1_8(0x200000000L, 0x0L);
case 62:
jjmatchedKind = 165;
return jjMoveStringLiteralDfa1_8(0x800000000L, 0x0L);
case 65:
case 97:
return jjMoveStringLiteralDfa1_8(0x8040000000000L, 0x0L);
case 67:
case 99:
return jjMoveStringLiteralDfa1_8(0x0L, 0x802L);
case 69:
case 101:
return jjMoveStringLiteralDfa1_8(0x10000000000L, 0x40200L);
case 71:
case 103:
return jjMoveStringLiteralDfa1_8(0x8001800000000000L, 0x4000L);
case 72:
case 104:
return jjMoveStringLiteralDfa1_8(0x0L, 0x40L);
case 73:
case 105:
return jjMoveStringLiteralDfa1_8(0x40400000000000L, 0x0L);
case 76:
case 108:
return jjMoveStringLiteralDfa1_8(0x2800000000000000L, 0x18000L);
case 78:
case 110:
return jjMoveStringLiteralDfa1_8(0x120000000000L, 0x0L);
case 79:
case 111:
return jjMoveStringLiteralDfa1_8(0x80000000000L, 0x0L);
case 80:
case 112:
return jjMoveStringLiteralDfa1_8(0x110000000000000L, 0x23020L);
case 82:
case 114:
return jjMoveStringLiteralDfa1_8(0x0L, 0x80L);
case 83:
case 115:
return jjMoveStringLiteralDfa1_8(0x280000000000000L, 0x11dL);
case 84:
case 116:
return jjMoveStringLiteralDfa1_8(0x400000000000000L, 0x0L);
case 85:
case 117:
return jjMoveStringLiteralDfa1_8(0x1006000000000000L, 0x0L);
case 86:
case 118:
return jjMoveStringLiteralDfa1_8(0x4000000000000000L, 0x0L);
case 87:
case 119:
return jjMoveStringLiteralDfa1_8(0x20000000000000L, 0x400L);
default :
return jjMoveNfa_8(7, 0);
}
}
private int jjMoveStringLiteralDfa1_8(long active2, long active3)
{
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(0, 0L, 0L, active2, active3);
return 1;
}
switch(curChar)
{
case 61:
if ((active2 & 0x200000000L) != 0L)
return jjStopAtPos(1, 161);
else if ((active2 & 0x400000000L) != 0L)
return jjStopAtPos(1, 162);
else if ((active2 & 0x800000000L) != 0L)
return jjStopAtPos(1, 163);
else if ((active2 & 0x1000000000L) != 0L)
return jjStopAtPos(1, 164);
break;
case 65:
case 97:
return jjMoveStringLiteralDfa2_8(active2, 0x4110000000000000L, active3, 0x23040L);
case 67:
case 99:
return jjMoveStringLiteralDfa2_8(active2, 0x1808000000000000L, active3, 0L);
case 69:
case 101:
if ((active2 & 0x20000000000L) != 0L)
return jjStartNfaWithStates_8(1, 169, 34);
return jjMoveStringLiteralDfa2_8(active2, 0xa021800000000000L, active3, 0x449dL);
case 73:
case 105:
return jjMoveStringLiteralDfa2_8(active2, 0L, active3, 0x20L);
case 78:
case 110:
return jjMoveStringLiteralDfa2_8(active2, 0x40000000000L, active3, 0x40000L);
case 79:
case 111:
return jjMoveStringLiteralDfa2_8(active2, 0x100000000000L, active3, 0x18802L);
case 80:
case 112:
return jjMoveStringLiteralDfa2_8(active2, 0L, active3, 0x100L);
case 81:
case 113:
if ((active2 & 0x10000000000L) != 0L)
return jjStartNfaWithStates_8(1, 168, 34);
break;
case 82:
case 114:
if ((active2 & 0x80000000000L) != 0L)
return jjStartNfaWithStates_8(1, 171, 34);
return jjMoveStringLiteralDfa2_8(active2, 0x406000000000000L, active3, 0L);
case 83:
case 115:
return jjMoveStringLiteralDfa2_8(active2, 0x40400000000000L, active3, 0L);
case 84:
case 116:
return jjMoveStringLiteralDfa2_8(active2, 0x280000000000000L, active3, 0L);
case 88:
case 120:
return jjMoveStringLiteralDfa2_8(active2, 0L, active3, 0x200L);
default :
break;
}
return jjStartNfa_8(0, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa2_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(0, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(1, 0L, 0L, active2, active3);
return 2;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa3_8(active2, 0x1800000000000000L, active3, 0L);
case 66:
case 98:
return jjMoveStringLiteralDfa3_8(active2, 0x20000000000000L, active3, 0x400L);
case 67:
case 99:
return jjMoveStringLiteralDfa3_8(active2, 0L, active3, 0x58000L);
case 68:
case 100:
if ((active2 & 0x40000000000L) != 0L)
return jjStartNfaWithStates_8(2, 170, 34);
else if ((active2 & 0x100000000000000L) != 0L)
return jjStartNfaWithStates_8(2, 184, 34);
return jjMoveStringLiteralDfa3_8(active2, 0x40000000000000L, active3, 0L);
case 73:
case 105:
return jjMoveStringLiteralDfa3_8(active2, 0x400000000000000L, active3, 0x200L);
case 76:
case 108:
if ((active2 & 0x2000000000000L) != 0L)
{
jjmatchedKind = 177;
jjmatchedPos = 2;
}
else if ((active2 & 0x4000000000000000L) != 0L)
return jjStartNfaWithStates_8(2, 190, 34);
return jjMoveStringLiteralDfa3_8(active2, 0x4000000000000L, active3, 0x100L);
case 78:
case 110:
if ((active2 & 0x2000000000000000L) != 0L)
return jjStartNfaWithStates_8(2, 189, 34);
return jjMoveStringLiteralDfa3_8(active2, 0L, active3, 0x802L);
case 80:
case 112:
return jjMoveStringLiteralDfa3_8(active2, 0L, active3, 0xa0L);
case 82:
case 114:
return jjMoveStringLiteralDfa3_8(active2, 0x290000000000000L, active3, 0x23005L);
case 83:
case 115:
return jjMoveStringLiteralDfa3_8(active2, 0x400000000000L, active3, 0x58L);
case 84:
case 116:
if ((active2 & 0x100000000000L) != 0L)
return jjStartNfaWithStates_8(2, 172, 34);
return jjMoveStringLiteralDfa3_8(active2, 0x8009800000000000L, active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(1, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa3_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(1, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(2, 0L, 0L, active2, active3);
return 3;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa4_8(active2, 0x10000000000000L, active3, 0x3b000L);
case 67:
case 99:
return jjMoveStringLiteralDfa4_8(active2, 0x8000000000000000L, active3, 0L);
case 69:
case 101:
return jjMoveStringLiteralDfa4_8(active2, 0x44000000000000L, active3, 0x20L);
case 72:
case 104:
return jjMoveStringLiteralDfa4_8(active2, 0x800000000000L, active3, 0L);
case 73:
case 105:
return jjMoveStringLiteralDfa4_8(active2, 0x288000000000000L, active3, 0x100L);
case 76:
case 108:
return jjMoveStringLiteralDfa4_8(active2, 0L, active3, 0x80L);
case 77:
case 109:
if ((active2 & 0x400000000000000L) != 0L)
return jjStartNfaWithStates_8(3, 186, 34);
break;
case 78:
case 110:
return jjMoveStringLiteralDfa4_8(active2, 0L, active3, 0x40L);
case 79:
case 111:
return jjMoveStringLiteralDfa4_8(active2, 0L, active3, 0x40000L);
case 82:
case 114:
return jjMoveStringLiteralDfa4_8(active2, 0x20000000000000L, active3, 0x400L);
case 83:
case 115:
return jjMoveStringLiteralDfa4_8(active2, 0x1800400000000000L, active3, 0x218L);
case 84:
case 116:
return jjMoveStringLiteralDfa4_8(active2, 0L, active3, 0x4802L);
case 86:
case 118:
return jjMoveStringLiteralDfa4_8(active2, 0x1000000000000L, active3, 0x5L);
default :
break;
}
return jjStartNfa_8(2, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa4_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(2, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(3, 0L, 0L, active2, active3);
return 4;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa5_8(active2, 0x1000000000000L, active3, 0x80L);
case 68:
case 100:
return jjMoveStringLiteralDfa5_8(active2, 0L, active3, 0x40000L);
case 69:
case 101:
if ((active2 & 0x800000000000000L) != 0L)
return jjStartNfaWithStates_8(4, 187, 34);
else if ((active2 & 0x1000000000000000L) != 0L)
return jjStartNfaWithStates_8(4, 188, 34);
return jjMoveStringLiteralDfa5_8(active2, 0x800000000000L, active3, 0x4842L);
case 70:
case 102:
return jjMoveStringLiteralDfa5_8(active2, 0x40000000000000L, active3, 0L);
case 73:
case 105:
return jjMoveStringLiteralDfa5_8(active2, 0L, active3, 0x1cL);
case 76:
case 108:
return jjMoveStringLiteralDfa5_8(active2, 0L, active3, 0x18021L);
case 77:
case 109:
return jjMoveStringLiteralDfa5_8(active2, 0x10000000000000L, active3, 0x23000L);
case 78:
case 110:
return jjMoveStringLiteralDfa5_8(active2, 0x280000000000000L, active3, 0L);
case 79:
case 111:
return jjMoveStringLiteralDfa5_8(active2, 0x8028000000000000L, active3, 0x400L);
case 83:
case 115:
return jjMoveStringLiteralDfa5_8(active2, 0x400000000000L, active3, 0L);
case 84:
case 116:
if ((active3 & 0x100L) != 0L)
return jjStartNfaWithStates_8(4, 200, 34);
return jjMoveStringLiteralDfa5_8(active2, 0L, active3, 0x200L);
case 88:
case 120:
if ((active2 & 0x4000000000000L) != 0L)
return jjStartNfaWithStates_8(4, 178, 34);
break;
default :
break;
}
return jjStartNfa_8(3, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa5_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(3, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(4, 0L, 0L, active2, active3);
return 5;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa6_8(active2, 0x800000000000L, active3, 0L);
case 67:
case 99:
return jjMoveStringLiteralDfa6_8(active2, 0L, active3, 0x84L);
case 69:
case 101:
return jjMoveStringLiteralDfa6_8(active2, 0x10400000000000L, active3, 0x62001L);
case 71:
case 103:
return jjMoveStringLiteralDfa6_8(active2, 0x280000000000000L, active3, 0L);
case 73:
case 105:
return jjMoveStringLiteralDfa6_8(active2, 0x40000000000000L, active3, 0x18020L);
case 76:
case 108:
return jjMoveStringLiteralDfa6_8(active2, 0x1000000000000L, active3, 0L);
case 77:
case 109:
return jjMoveStringLiteralDfa6_8(active2, 0L, active3, 0x5000L);
case 78:
case 110:
if ((active2 & 0x8000000000000L) != 0L)
return jjStartNfaWithStates_8(5, 179, 34);
return jjMoveStringLiteralDfa6_8(active2, 0L, active3, 0x802L);
case 79:
case 111:
return jjMoveStringLiteralDfa6_8(active2, 0x8020000000000000L, active3, 0x418L);
case 83:
case 115:
return jjMoveStringLiteralDfa6_8(active2, 0L, active3, 0x200L);
case 88:
case 120:
return jjMoveStringLiteralDfa6_8(active2, 0L, active3, 0x40L);
default :
break;
}
return jjStartNfa_8(4, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa6_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(4, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(5, 0L, 0L, active2, active3);
return 6;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa7_8(active2, 0L, active3, 0x1000L);
case 68:
case 100:
return jjMoveStringLiteralDfa7_8(active2, 0x800000000000L, active3, 0L);
case 69:
case 101:
if ((active3 & 0x4L) != 0L)
return jjStartNfaWithStates_8(6, 194, 34);
else if ((active3 & 0x80L) != 0L)
return jjStartNfaWithStates_8(6, 199, 34);
break;
case 75:
case 107:
return jjMoveStringLiteralDfa7_8(active2, 0x8000000000000000L, active3, 0L);
case 78:
case 110:
return jjMoveStringLiteralDfa7_8(active2, 0x40400000000000L, active3, 0x2038L);
case 80:
case 112:
return jjMoveStringLiteralDfa7_8(active2, 0L, active3, 0x4000L);
case 84:
case 116:
if ((active2 & 0x20000000000000L) != 0L)
{
jjmatchedKind = 181;
jjmatchedPos = 6;
}
else if ((active3 & 0x1L) != 0L)
return jjStartNfaWithStates_8(6, 192, 34);
else if ((active3 & 0x40L) != 0L)
return jjStartNfaWithStates_8(6, 198, 34);
return jjMoveStringLiteralDfa7_8(active2, 0x290000000000000L, active3, 0x20e02L);
case 85:
case 117:
return jjMoveStringLiteralDfa7_8(active2, 0x1000000000000L, active3, 0L);
case 86:
case 118:
return jjMoveStringLiteralDfa7_8(active2, 0L, active3, 0x40000L);
case 90:
case 122:
return jjMoveStringLiteralDfa7_8(active2, 0L, active3, 0x18000L);
default :
break;
}
return jjStartNfa_8(5, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa7_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(5, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(6, 0L, 0L, active2, active3);
return 7;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa8_8(active2, 0x400000000000L, active3, 0x40000L);
case 69:
case 101:
if ((active2 & 0x1000000000000L) != 0L)
return jjStartNfaWithStates_8(7, 176, 34);
else if ((active3 & 0x20L) != 0L)
return jjStartNfaWithStates_8(7, 197, 34);
return jjMoveStringLiteralDfa8_8(active2, 0x50800000000000L, active3, 0x38600L);
case 73:
case 105:
return jjMoveStringLiteralDfa8_8(active2, 0x8000000000000000L, active3, 0L);
case 76:
case 108:
return jjMoveStringLiteralDfa8_8(active2, 0L, active3, 0x4018L);
case 79:
case 111:
return jjMoveStringLiteralDfa8_8(active2, 0x280000000000000L, active3, 0L);
case 80:
case 112:
if ((active3 & 0x1000L) != 0L)
return jjStartNfaWithStates_8(7, 204, 34);
break;
case 84:
case 116:
return jjMoveStringLiteralDfa8_8(active2, 0L, active3, 0x2000L);
case 85:
case 117:
return jjMoveStringLiteralDfa8_8(active2, 0L, active3, 0x802L);
default :
break;
}
return jjStartNfa_8(6, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa8_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(6, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(7, 0L, 0L, active2, active3);
return 8;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa9_8(active2, 0L, active3, 0x4000L);
case 66:
case 98:
return jjMoveStringLiteralDfa9_8(active2, 0x400000000000L, active3, 0L);
case 68:
case 100:
if ((active2 & 0x40000000000000L) != 0L)
return jjStartNfaWithStates_8(8, 182, 34);
break;
case 69:
case 101:
if ((active2 & 0x8000000000000000L) != 0L)
return jjStartNfaWithStates_8(8, 191, 34);
return jjMoveStringLiteralDfa9_8(active2, 0L, active3, 0x18L);
case 72:
case 104:
return jjMoveStringLiteralDfa9_8(active2, 0x80000000000000L, active3, 0L);
case 76:
case 108:
return jjMoveStringLiteralDfa9_8(active2, 0L, active3, 0x40000L);
case 77:
case 109:
return jjMoveStringLiteralDfa9_8(active2, 0L, active3, 0x200L);
case 82:
case 114:
if ((active2 & 0x800000000000L) != 0L)
return jjStartNfaWithStates_8(8, 175, 34);
else if ((active2 & 0x10000000000000L) != 0L)
{
jjmatchedKind = 180;
jjmatchedPos = 8;
}
return jjMoveStringLiteralDfa9_8(active2, 0L, active3, 0x22802L);
case 84:
case 116:
return jjMoveStringLiteralDfa9_8(active2, 0L, active3, 0x18000L);
case 88:
case 120:
if ((active3 & 0x400L) != 0L)
return jjStartNfaWithStates_8(8, 202, 34);
return jjMoveStringLiteralDfa9_8(active2, 0x200000000000000L, active3, 0L);
default :
break;
}
return jjStartNfa_8(7, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa9_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(7, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(8, 0L, 0L, active2, active3);
return 9;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa10_8(active2, 0L, active3, 0x18000L);
case 76:
case 108:
if ((active3 & 0x2L) != 0L)
{
jjmatchedKind = 193;
jjmatchedPos = 9;
}
return jjMoveStringLiteralDfa10_8(active2, 0x400000000000L, active3, 0x20800L);
case 77:
case 109:
return jjMoveStringLiteralDfa10_8(active2, 0x200000000000000L, active3, 0L);
case 80:
case 112:
return jjMoveStringLiteralDfa10_8(active2, 0L, active3, 0x200L);
case 83:
case 115:
return jjMoveStringLiteralDfa10_8(active2, 0L, active3, 0x18L);
case 84:
case 116:
return jjMoveStringLiteralDfa10_8(active2, 0x80000000000000L, active3, 0x4000L);
case 85:
case 117:
return jjMoveStringLiteralDfa10_8(active2, 0L, active3, 0x40000L);
case 89:
case 121:
if ((active3 & 0x2000L) != 0L)
return jjStartNfaWithStates_8(9, 205, 34);
break;
default :
break;
}
return jjStartNfa_8(8, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa10_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(8, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(9, 0L, 0L, active2, active3);
return 10;
}
switch(curChar)
{
case 69:
case 101:
if ((active3 & 0x40000L) != 0L)
return jjStartNfaWithStates_8(10, 210, 34);
return jjMoveStringLiteralDfa11_8(active2, 0x400000000000L, active3, 0x4800L);
case 73:
case 105:
return jjMoveStringLiteralDfa11_8(active2, 0L, active3, 0x20000L);
case 76:
case 108:
if ((active2 & 0x200000000000000L) != 0L)
return jjStartNfaWithStates_8(10, 185, 34);
return jjMoveStringLiteralDfa11_8(active2, 0L, active3, 0x200L);
case 77:
case 109:
return jjMoveStringLiteralDfa11_8(active2, 0x80000000000000L, active3, 0L);
case 83:
case 115:
return jjMoveStringLiteralDfa11_8(active2, 0L, active3, 0x18L);
case 88:
case 120:
return jjMoveStringLiteralDfa11_8(active2, 0L, active3, 0x18000L);
default :
break;
}
return jjStartNfa_8(9, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa11_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(9, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(10, 0L, 0L, active2, active3);
return 11;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa12_8(active2, 0L, active3, 0x200L);
case 68:
case 100:
if ((active2 & 0x400000000000L) != 0L)
return jjStartNfaWithStates_8(11, 174, 34);
break;
case 76:
case 108:
if ((active2 & 0x80000000000000L) != 0L)
return jjStartNfaWithStates_8(11, 183, 34);
break;
case 83:
case 115:
return jjMoveStringLiteralDfa12_8(active2, 0L, active3, 0x24000L);
case 84:
case 116:
if ((active3 & 0x8000L) != 0L)
{
jjmatchedKind = 207;
jjmatchedPos = 11;
}
return jjMoveStringLiteralDfa12_8(active2, 0L, active3, 0x10000L);
case 85:
case 117:
return jjMoveStringLiteralDfa12_8(active2, 0L, active3, 0x18L);
case 88:
case 120:
if ((active3 & 0x800L) != 0L)
return jjStartNfaWithStates_8(11, 203, 34);
break;
default :
break;
}
return jjStartNfa_8(10, 0L, 0L, active2, active3);
}
private int jjMoveStringLiteralDfa12_8(long old2, long active2, long old3, long active3)
{
if (((active2 &= old2) | (active3 &= old3)) == 0L)
return jjStartNfa_8(10, 0L, 0L, old2, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(11, 0L, 0L, 0L, active3);
return 12;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa13_8(active3, 0x10000L);
case 79:
case 111:
return jjMoveStringLiteralDfa13_8(active3, 0x4000L);
case 82:
case 114:
return jjMoveStringLiteralDfa13_8(active3, 0x18L);
case 84:
case 116:
if ((active3 & 0x20000L) != 0L)
return jjStartNfaWithStates_8(12, 209, 34);
return jjMoveStringLiteralDfa13_8(active3, 0x200L);
default :
break;
}
return jjStartNfa_8(11, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa13_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(11, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(12, 0L, 0L, 0L, active3);
return 13;
}
switch(curChar)
{
case 69:
case 101:
if ((active3 & 0x200L) != 0L)
return jjStartNfaWithStates_8(13, 201, 34);
break;
case 76:
case 108:
if ((active3 & 0x8L) != 0L)
{
jjmatchedKind = 195;
jjmatchedPos = 13;
}
return jjMoveStringLiteralDfa14_8(active3, 0x10L);
case 85:
case 117:
return jjMoveStringLiteralDfa14_8(active3, 0x4000L);
case 88:
case 120:
if ((active3 & 0x10000L) != 0L)
return jjStartNfaWithStates_8(13, 208, 34);
break;
default :
break;
}
return jjStartNfa_8(12, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa14_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(12, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(13, 0L, 0L, 0L, active3);
return 14;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa15_8(active3, 0x10L);
case 82:
case 114:
return jjMoveStringLiteralDfa15_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(13, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa15_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(13, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(14, 0L, 0L, 0L, active3);
return 15;
}
switch(curChar)
{
case 67:
case 99:
return jjMoveStringLiteralDfa16_8(active3, 0x4000L);
case 88:
case 120:
if ((active3 & 0x10L) != 0L)
return jjStartNfaWithStates_8(15, 196, 34);
break;
default :
break;
}
return jjStartNfa_8(14, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa16_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(14, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(15, 0L, 0L, 0L, active3);
return 16;
}
switch(curChar)
{
case 69:
case 101:
return jjMoveStringLiteralDfa17_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(15, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa17_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(15, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(16, 0L, 0L, 0L, active3);
return 17;
}
switch(curChar)
{
case 76:
case 108:
return jjMoveStringLiteralDfa18_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(16, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa18_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(16, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(17, 0L, 0L, 0L, active3);
return 18;
}
switch(curChar)
{
case 79:
case 111:
return jjMoveStringLiteralDfa19_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(17, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa19_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(17, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(18, 0L, 0L, 0L, active3);
return 19;
}
switch(curChar)
{
case 67:
case 99:
return jjMoveStringLiteralDfa20_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(18, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa20_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(18, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(19, 0L, 0L, 0L, active3);
return 20;
}
switch(curChar)
{
case 65:
case 97:
return jjMoveStringLiteralDfa21_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(19, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa21_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(19, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(20, 0L, 0L, 0L, active3);
return 21;
}
switch(curChar)
{
case 84:
case 116:
return jjMoveStringLiteralDfa22_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(20, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa22_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(20, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(21, 0L, 0L, 0L, active3);
return 22;
}
switch(curChar)
{
case 73:
case 105:
return jjMoveStringLiteralDfa23_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(21, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa23_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(21, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(22, 0L, 0L, 0L, active3);
return 23;
}
switch(curChar)
{
case 79:
case 111:
return jjMoveStringLiteralDfa24_8(active3, 0x4000L);
default :
break;
}
return jjStartNfa_8(22, 0L, 0L, 0L, active3);
}
private int jjMoveStringLiteralDfa24_8(long old3, long active3)
{
if (((active3 &= old3)) == 0L)
return jjStartNfa_8(22, 0L, 0L, 0L, old3);
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) {
jjStopStringLiteralDfa_8(23, 0L, 0L, 0L, active3);
return 24;
}
switch(curChar)
{
case 78:
case 110:
if ((active3 & 0x4000L) != 0L)
return jjStartNfaWithStates_8(24, 206, 34);
break;
default :
break;
}
return jjStartNfa_8(23, 0L, 0L, 0L, active3);
}
private int jjStartNfaWithStates_8(int pos, int kind, int state)
{
jjmatchedKind = kind;
jjmatchedPos = pos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return pos + 1; }
return jjMoveNfa_8(state, pos + 1);
}
private int jjMoveNfa_8(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 70;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 59:
case 34:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
break;
case 58:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
break;
case 60:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
break;
case 7:
if ((0x3ff000000000000L & l) != 0L)
{
if (kind > 214)
kind = 214;
jjCheckNAddStates(159, 162);
}
else if (curChar == 46)
jjCheckNAdd(29);
else if (curChar == 34)
jjCheckNAddStates(163, 167);
else if (curChar == 39)
jjCheckNAddStates(168, 172);
else if (curChar == 36)
jjstateSet[jjnewStateCnt++] = 8;
else if (curChar == 60)
jjstateSet[jjnewStateCnt++] = 6;
break;
case 0:
if (curChar == 61)
jjCheckNAddStates(173, 175);
break;
case 1:
if ((0xffffffdfffffffffL & l) != 0L)
jjCheckNAddStates(173, 175);
break;
case 2:
if (curChar == 37)
jjCheckNAddTwoStates(2, 3);
break;
case 3:
if ((0xbfffffffffffffffL & l) != 0L)
jjCheckNAddStates(173, 175);
break;
case 4:
if (curChar == 62 && kind > 154)
kind = 154;
break;
case 5:
if (curChar == 37)
jjstateSet[jjnewStateCnt++] = 4;
break;
case 6:
if (curChar == 37)
jjstateSet[jjnewStateCnt++] = 0;
break;
case 9:
jjAddStates(176, 177);
break;
case 11:
if (curChar == 36)
jjstateSet[jjnewStateCnt++] = 8;
break;
case 12:
case 15:
if (curChar == 39)
jjCheckNAddStates(168, 172);
break;
case 14:
case 17:
if ((0xffffff7fffffffffL & l) != 0L)
jjCheckNAddStates(168, 172);
break;
case 18:
if (curChar == 39)
jjstateSet[jjnewStateCnt++] = 15;
break;
case 19:
if (curChar == 39 && kind > 212)
kind = 212;
break;
case 20:
case 23:
if (curChar == 34)
jjCheckNAddStates(163, 167);
break;
case 22:
case 25:
if ((0xfffffffbffffffffL & l) != 0L)
jjCheckNAddStates(163, 167);
break;
case 26:
if (curChar == 34)
jjstateSet[jjnewStateCnt++] = 23;
break;
case 27:
if (curChar == 34 && kind > 213)
kind = 213;
break;
case 28:
if (curChar == 46)
jjCheckNAdd(29);
break;
case 29:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 214)
kind = 214;
jjCheckNAddTwoStates(29, 30);
break;
case 31:
if ((0x280000000000L & l) != 0L)
jjCheckNAdd(32);
break;
case 32:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 214)
kind = 214;
jjCheckNAdd(32);
break;
case 35:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 214)
kind = 214;
jjCheckNAddStates(159, 162);
break;
case 36:
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddTwoStates(36, 37);
break;
case 37:
if (curChar != 46)
break;
if (kind > 214)
kind = 214;
jjCheckNAddTwoStates(38, 39);
break;
case 38:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 214)
kind = 214;
jjCheckNAddTwoStates(38, 39);
break;
case 40:
if ((0x280000000000L & l) != 0L)
jjCheckNAdd(41);
break;
case 41:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 214)
kind = 214;
jjCheckNAdd(41);
break;
case 42:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 214)
kind = 214;
jjCheckNAddTwoStates(42, 43);
break;
case 44:
if ((0x280000000000L & l) != 0L)
jjCheckNAdd(45);
break;
case 45:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 214)
kind = 214;
jjCheckNAdd(45);
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 59:
if ((0x7fffffe87fffffeL & l) != 0L)
{
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
}
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 67;
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 58;
break;
case 58:
if ((0x7fffffe87fffffeL & l) != 0L)
{
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
}
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 66;
else if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 57;
break;
case 60:
if ((0x7fffffe87fffffeL & l) != 0L)
{
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
}
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 68;
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 59;
break;
case 7:
if ((0x7fffffe07fffffeL & l) != 0L)
{
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
}
if ((0x10000000100L & l) != 0L)
jjAddStates(178, 179);
break;
case 1:
case 3:
jjCheckNAddStates(173, 175);
break;
case 8:
if (curChar == 123)
jjCheckNAddTwoStates(9, 10);
break;
case 9:
if ((0xdfffffffffffffffL & l) != 0L)
jjCheckNAddTwoStates(9, 10);
break;
case 10:
if (curChar == 125 && kind > 155)
kind = 155;
break;
case 13:
if (curChar == 92)
jjstateSet[jjnewStateCnt++] = 14;
break;
case 14:
jjCheckNAddStates(168, 172);
break;
case 16:
if (curChar == 92)
jjstateSet[jjnewStateCnt++] = 15;
break;
case 17:
if ((0xffffffffefffffffL & l) != 0L)
jjCheckNAddStates(168, 172);
break;
case 21:
if (curChar == 92)
jjstateSet[jjnewStateCnt++] = 22;
break;
case 22:
jjCheckNAddStates(163, 167);
break;
case 24:
if (curChar == 92)
jjstateSet[jjnewStateCnt++] = 23;
break;
case 25:
if ((0xffffffffefffffffL & l) != 0L)
jjCheckNAddStates(163, 167);
break;
case 30:
if ((0x2000000020L & l) != 0L)
jjAddStates(180, 181);
break;
case 33:
if ((0x7fffffe07fffffeL & l) == 0L)
break;
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
break;
case 34:
if ((0x7fffffe87fffffeL & l) == 0L)
break;
if (kind > 217)
kind = 217;
jjCheckNAdd(34);
break;
case 39:
if ((0x2000000020L & l) != 0L)
jjAddStates(182, 183);
break;
case 43:
if ((0x2000000020L & l) != 0L)
jjAddStates(184, 185);
break;
case 46:
if ((0x10000000100L & l) != 0L)
jjAddStates(178, 179);
break;
case 47:
if ((0x8000000080000L & l) != 0L && kind > 173)
kind = 173;
break;
case 48:
case 61:
if ((0x10000000100000L & l) != 0L)
jjCheckNAdd(47);
break;
case 49:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 48;
break;
case 50:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 49;
break;
case 51:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 50;
break;
case 52:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 51;
break;
case 53:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 52;
break;
case 54:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 53;
break;
case 55:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 54;
break;
case 56:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 55;
break;
case 57:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 56;
break;
case 62:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 61;
break;
case 63:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 62;
break;
case 64:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 63;
break;
case 65:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 64;
break;
case 66:
if ((0x100000001000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 65;
break;
case 67:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 66;
break;
case 68:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 67;
break;
case 69:
if ((0x200000002L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 68;
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 3:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjCheckNAddStates(173, 175);
break;
case 9:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjAddStates(176, 177);
break;
case 14:
case 17:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjCheckNAddStates(168, 172);
break;
case 22:
case 25:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjCheckNAddStates(163, 167);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 70 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private int jjMoveStringLiteralDfa0_1()
{
return jjMoveNfa_1(1, 0);
}
private int jjMoveNfa_1(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 17;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 1:
if ((0xefffdfffffffffffL & l) != 0L)
{
if (kind > 54)
kind = 54;
jjCheckNAdd(0);
}
else if ((0x1000200000000000L & l) != 0L)
{
if (kind > 54)
kind = 54;
}
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 15;
else if (curChar == 60)
jjstateSet[jjnewStateCnt++] = 12;
break;
case 0:
if ((0xefffdfffffffffffL & l) == 0L)
break;
if (kind > 54)
kind = 54;
jjCheckNAdd(0);
break;
case 2:
if (curChar == 62 && kind > 55)
kind = 55;
break;
case 12:
if (curChar == 47)
jjstateSet[jjnewStateCnt++] = 11;
break;
case 13:
if (curChar == 60)
jjstateSet[jjnewStateCnt++] = 12;
break;
case 14:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 2;
break;
case 15:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 14;
break;
case 16:
if (curChar == 45)
jjstateSet[jjnewStateCnt++] = 15;
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 0:
if (kind > 54)
kind = 54;
jjCheckNAdd(0);
break;
case 3:
if ((0x10000000100000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 2;
break;
case 4:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 3;
break;
case 5:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 4;
break;
case 6:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 5;
break;
case 7:
if ((0x200000002000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 6;
break;
case 8:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 7;
break;
case 9:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 8;
break;
case 10:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 9;
break;
case 11:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 10;
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 1:
case 0:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 54)
kind = 54;
jjCheckNAdd(0);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 17 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
private final int jjStopStringLiteralDfa_5(int pos, long active0, long active1)
{
switch (pos)
{
default :
return -1;
}
}
private final int jjStartNfa_5(int pos, long active0, long active1)
{
return jjMoveNfa_5(jjStopStringLiteralDfa_5(pos, active0, active1), pos + 1);
}
private int jjMoveStringLiteralDfa0_5()
{
switch(curChar)
{
case 34:
return jjStopAtPos(0, 68);
case 35:
return jjStopAtPos(0, 69);
case 61:
return jjStartNfaWithStates_5(0, 66, 14);
default :
return jjMoveNfa_5(0, 0);
}
}
private int jjStartNfaWithStates_5(int pos, int kind, int state)
{
jjmatchedKind = kind;
jjmatchedPos = pos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return pos + 1; }
return jjMoveNfa_5(state, pos + 1);
}
private int jjMoveNfa_5(int startState, int curPos)
{
int startsAt = 0;
jjnewStateCnt = 14;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;)
{
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64)
{
long l = 1L << curChar;
do
{
switch(jjstateSet[--i])
{
case 0:
if ((0x100002600L & l) != 0L)
{
if (kind > 65)
kind = 65;
jjCheckNAddStates(186, 188);
}
else if (curChar == 61)
jjCheckNAddStates(189, 192);
else if (curChar == 62)
{
if (kind > 67)
kind = 67;
}
break;
case 14:
if ((0xdffffff2ffffd9ffL & l) != 0L)
{
if (kind > 64)
kind = 64;
jjCheckNAdd(10);
}
else if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(9, 10);
else if (curChar == 34)
jjCheckNAddTwoStates(7, 8);
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(5, 6);
break;
case 1:
if ((0x3ff200000000000L & l) == 0L)
break;
if (kind > 62)
kind = 62;
jjCheckNAddTwoStates(1, 2);
break;
case 2:
if ((0x100002600L & l) == 0L)
break;
if (kind > 62)
kind = 62;
jjCheckNAdd(2);
break;
case 3:
if (curChar == 62 && kind > 67)
kind = 67;
break;
case 4:
if (curChar == 61)
jjCheckNAddStates(189, 192);
break;
case 5:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(5, 6);
break;
case 6:
if (curChar == 34)
jjCheckNAddTwoStates(7, 8);
break;
case 7:
if ((0xfffffff3ffffffffL & l) != 0L)
jjCheckNAddTwoStates(7, 8);
break;
case 8:
if (curChar == 34 && kind > 63)
kind = 63;
break;
case 9:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(9, 10);
break;
case 10:
if ((0xdffffff2ffffd9ffL & l) == 0L)
break;
if (kind > 64)
kind = 64;
jjCheckNAdd(10);
break;
case 11:
if ((0x100002600L & l) == 0L)
break;
if (kind > 65)
kind = 65;
jjCheckNAddStates(186, 188);
break;
case 12:
if ((0x100002600L & l) == 0L)
break;
if (kind > 65)
kind = 65;
jjCheckNAdd(12);
break;
case 13:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(13, 3);
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 0:
if ((0x7fffffe07fffffeL & l) == 0L)
break;
if (kind > 62)
kind = 62;
jjCheckNAddTwoStates(1, 2);
break;
case 14:
case 10:
if (kind > 64)
kind = 64;
jjCheckNAdd(10);
break;
case 1:
if ((0x7fffffe87fffffeL & l) == 0L)
break;
if (kind > 62)
kind = 62;
jjCheckNAddTwoStates(1, 2);
break;
case 7:
jjAddStates(193, 194);
break;
default : break;
}
} while(i != startsAt);
}
else
{
int hiByte = (int)(curChar >> 8);
int i1 = hiByte >> 6;
long l1 = 1L << (hiByte & 077);
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 14:
case 10:
if (!jjCanMove_0(hiByte, i1, i2, l1, l2))
break;
if (kind > 64)
kind = 64;
jjCheckNAdd(10);
break;
case 7:
if (jjCanMove_0(hiByte, i1, i2, l1, l2))
jjAddStates(193, 194);
break;
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 14 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = input_stream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
static final int[] jjnextStates = {
617, 618, 18, 619, 20, 610, 611, 614, 615, 38, 39, 116, 117, 125, 126, 199,
200, 224, 225, 288, 289, 483, 484, 532, 533, 540, 541, 549, 550, 586, 587, 600,
601, 597, 608, 584, 595, 572, 579, 558, 566, 496, 502, 508, 514, 521, 530, 538,
547, 554, 300, 308, 312, 324, 332, 338, 348, 358, 368, 378, 388, 398, 408, 418,
428, 438, 445, 458, 471, 481, 490, 280, 286, 292, 270, 273, 256, 262, 237, 247,
222, 232, 169, 175, 187, 197, 207, 145, 153, 157, 91, 98, 105, 114, 123, 130,
59, 67, 83, 30, 36, 43, 612, 613, 1, 2, 166, 167, 168, 156, 157, 158,
160, 161, 162, 160, 163, 19, 156, 166, 169, 170, 42, 43, 59, 63, 73, 81,
90, 97, 105, 112, 119, 126, 134, 137, 141, 153, 6, 7, 24, 25, 27, 33,
35, 37, 39, 46, 47, 48, 49, 83, 84, 99, 100, 128, 129, 10, 11, 36,
37, 42, 43, 21, 24, 25, 26, 27, 13, 16, 17, 18, 19, 1, 2, 5,
9, 10, 60, 69, 31, 32, 40, 41, 44, 45, 12, 13, 3, 5, 6, 9,
10, 7, 8,
};
private static final boolean jjCanMove_0(int hiByte, int i1, int i2, long l1, long l2)
{
switch(hiByte)
{
case 0:
return ((jjbitVec2[i2] & l2) != 0L);
default :
if ((jjbitVec0[i1] & l1) != 0L)
return true;
return false;
}
}
/** Token literal values. */
public static final String[] jjstrLiteralImages = {
"", null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, "\43", null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, "\75", null,
"\42", "\43", null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, "\75", null, null, "\42", "\43", null,
null, "\50", "\51", null, null, "\53", "\55", "\52", "\57", "\45", "\75\75",
"\41\75", "\76\75", "\74\75", "\76", "\74", "\56", null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, "\43", null, null, null,
"\72", "\54", null, null, null, null, null, null, };
/** Lexer state names. */
public static final String[] lexStateNames = {
"DEFAULT",
"COMMENT",
"BEAN",
"EJB",
"SERVLET",
"CUST_TAG",
"IS_TAG",
"ATTVALUE",
"EXPRESSION",
"SKIPPER",
};
/** Lex State array. */
public static final int[] jjnewLexState = {
-1, -1, -1, 2, 4, 3, 1, -1, -1, 6, 6, 6, 6, 6, -1, 6, 6, -1, -1, 6, 6, -1, 6, -1, 5,
-1, 6, 5, -1, 6, -1, 6, 6, 6, 6, 6, 6, 6, 6, 6, -1, 5, 5, 8, -1, -1, -1, -1, -1, -1,
-1, -1, -1, -1, -1, 0, -1, 0, -1, 0, -1, 0, -1, -1, -1, -1, -1, 0, -1, 8, -1, -1, -1, -1, -1,
-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1,
-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1,
-1, -1, -1, -1, -1, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, -1, -1, -1, -1, 0, 0, -1, 8,
6, 6, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1,
-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1,
-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1,
};
static final long[] jjtoToken = {
0xffffffffffffffffL, 0xffffffffffffffffL, 0xffffffffffffffffL, 0x43ffffffL,
};
static final long[] jjtoSkip = {
0x0L, 0x0L, 0x0L, 0x3c000000L,
};
protected JavaCharStream input_stream;
private final int[] jjrounds = new int[620];
private final int[] jjstateSet = new int[1240];
private final StringBuffer jjimage = new StringBuffer();
private StringBuffer image = jjimage;
private int jjimageLen;
private int lengthOfMatch;
protected char curChar;
/** Constructor. */
public ISMLtoJSPcompilerTokenManager(JavaCharStream stream){
if (JavaCharStream.staticFlag)
throw new Error("ERROR: Cannot use a static CharStream class with a non-static lexical analyzer.");
input_stream = stream;
}
/** Constructor. */
public ISMLtoJSPcompilerTokenManager(JavaCharStream stream, int lexState){
this(stream);
SwitchTo(lexState);
}
/** Reinitialise parser. */
public void ReInit(JavaCharStream stream)
{
jjmatchedPos = jjnewStateCnt = 0;
curLexState = defaultLexState;
input_stream = stream;
ReInitRounds();
}
private void ReInitRounds()
{
int i;
jjround = 0x80000001;
for (i = 620; i-- > 0;)
jjrounds[i] = 0x80000000;
}
/** Reinitialise parser. */
public void ReInit(JavaCharStream stream, int lexState)
{
ReInit(stream);
SwitchTo(lexState);
}
/** Switch to specified lex state. */
public void SwitchTo(int lexState)
{
if (lexState >= 10 || lexState < 0)
throw new TokenMgrError("Error: Ignoring invalid lexical state : " + lexState + ". State unchanged.", TokenMgrError.INVALID_LEXICAL_STATE);
else
curLexState = lexState;
}
protected Token jjFillToken()
{
final Token t;
final String curTokenImage;
final int beginLine;
final int endLine;
final int beginColumn;
final int endColumn;
String im = jjstrLiteralImages[jjmatchedKind];
curTokenImage = (im == null) ? input_stream.GetImage() : im;
beginLine = input_stream.getBeginLine();
beginColumn = input_stream.getBeginColumn();
endLine = input_stream.getEndLine();
endColumn = input_stream.getEndColumn();
t = Token.newToken(jjmatchedKind, curTokenImage);
t.beginLine = beginLine;
t.endLine = endLine;
t.beginColumn = beginColumn;
t.endColumn = endColumn;
return t;
}
int curLexState = 0;
int defaultLexState = 0;
int jjnewStateCnt;
int jjround;
int jjmatchedPos;
int jjmatchedKind;
/** Get the next Token. */
public Token getNextToken()
{
Token matchedToken;
int curPos = 0;
EOFLoop :
for (;;)
{
try
{
curChar = input_stream.BeginToken();
}
catch(java.io.IOException e)
{
jjmatchedKind = 0;
matchedToken = jjFillToken();
return matchedToken;
}
image = jjimage;
image.setLength(0);
jjimageLen = 0;
switch(curLexState)
{
case 0:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_0();
break;
case 1:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_1();
break;
case 2:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_2();
break;
case 3:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_3();
break;
case 4:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_4();
break;
case 5:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_5();
break;
case 6:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_6();
break;
case 7:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_7();
break;
case 8:
try { input_stream.backup(0);
while (curChar <= 32 && (0x100002600L & (1L << curChar)) != 0L)
curChar = input_stream.BeginToken();
}
catch (java.io.IOException e1) { continue EOFLoop; }
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_8();
break;
case 9:
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_9();
break;
}
if (jjmatchedKind != 0x7fffffff)
{
if (jjmatchedPos + 1 < curPos)
input_stream.backup(curPos - jjmatchedPos - 1);
if ((jjtoToken[jjmatchedKind >> 6] & (1L << (jjmatchedKind & 077))) != 0L)
{
matchedToken = jjFillToken();
TokenLexicalActions(matchedToken);
if (jjnewLexState[jjmatchedKind] != -1)
curLexState = jjnewLexState[jjmatchedKind];
return matchedToken;
}
else
{
if (jjnewLexState[jjmatchedKind] != -1)
curLexState = jjnewLexState[jjmatchedKind];
continue EOFLoop;
}
}
int error_line = input_stream.getEndLine();
int error_column = input_stream.getEndColumn();
String error_after = null;
boolean EOFSeen = false;
try { input_stream.readChar(); input_stream.backup(1); }
catch (java.io.IOException e1) {
EOFSeen = true;
error_after = curPos <= 1 ? "" : input_stream.GetImage();
if (curChar == '\n' || curChar == '\r') {
error_line++;
error_column = 0;
}
else
error_column++;
}
if (!EOFSeen) {
input_stream.backup(1);
error_after = curPos <= 1 ? "" : input_stream.GetImage();
}
throw new TokenMgrError(EOFSeen, curLexState, error_line, error_column, error_after, curChar, TokenMgrError.LEXICAL_ERROR);
}
}
void TokenLexicalActions(Token matchedToken)
{
switch(jjmatchedKind)
{
case 43 :
image.append(jjstrLiteralImages[43]);
lengthOfMatch = jjstrLiteralImages[43].length();
previousState = DEFAULT;
break;
case 69 :
image.append(jjstrLiteralImages[69]);
lengthOfMatch = jjstrLiteralImages[69].length();
previousState = CUST_TAG;
break;
case 149 :
image.append(jjstrLiteralImages[149]);
lengthOfMatch = jjstrLiteralImages[149].length();
previousState = IS_TAG;
break;
case 152 :
image.append(jjstrLiteralImages[152]);
lengthOfMatch = jjstrLiteralImages[152].length();
brackets++;
break;
case 153 :
image.append(jjstrLiteralImages[153]);
lengthOfMatch = jjstrLiteralImages[153].length();
brackets--;
break;
case 211 :
image.append(jjstrLiteralImages[211]);
lengthOfMatch = jjstrLiteralImages[211].length();
SwitchTo(previousState);
break;
default :
break;
}
}
private void jjCheckNAdd(int state)
{
if (jjrounds[state] != jjround)
{
jjstateSet[jjnewStateCnt++] = state;
jjrounds[state] = jjround;
}
}
private void jjAddStates(int start, int end)
{
do {
jjstateSet[jjnewStateCnt++] = jjnextStates[start];
} while (start++ != end);
}
private void jjCheckNAddTwoStates(int state1, int state2)
{
jjCheckNAdd(state1);
jjCheckNAdd(state2);
}
private void jjCheckNAddStates(int start, int end)
{
do {
jjCheckNAdd(jjnextStates[start]);
} while (start++ != end);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy