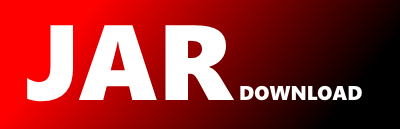
com.intuit.fuzzymatcher.component.ElementMatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fuzzy-matcher Show documentation
Show all versions of fuzzy-matcher Show documentation
A java library to determine probability of objects being similar
package com.intuit.fuzzymatcher.component;
import com.intuit.fuzzymatcher.domain.Element;
import com.intuit.fuzzymatcher.domain.Match;
import com.intuit.fuzzymatcher.domain.Token;
import org.apache.commons.lang3.BooleanUtils;
import java.util.*;
public class ElementMatch {
private final TokenRepo tokenRepo;
public ElementMatch() {
this.tokenRepo = new TokenRepo();
}
public Set> matchElement(Element element) {
Set> matchElements = new HashSet<>();
Map elementTokenScore = new HashMap<>();
List tokens = element.getTokens();
tokens.stream()
.filter(token -> BooleanUtils.isNotFalse(element.getDocument().isSource()))
.forEach(token -> {
elementThresholdMatching(token, elementTokenScore, matchElements);
});
tokens.forEach(token -> tokenRepo.put(token));
return matchElements;
}
private void elementThresholdMatching(Token token, Map elementTokenScore, Set> matchingElements) {
Set matchElements = tokenRepo.get(token);
Element element = token.getElement();
// Token Match Found
if (matchElements != null) {
matchElements.forEach(matchElement -> {
int score = elementTokenScore.getOrDefault(matchElement, 0) + 1;
elementTokenScore.put(matchElement, score);
// Element Score above threshold
double elementScore = element.getScore(score, matchElement);
// Element match Found
if (elementScore > element.getThreshold()) {
Match elementMatch = new Match<>(element, matchElement, elementScore);
matchingElements.remove(elementMatch);
matchingElements.add(elementMatch);
}
});
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy