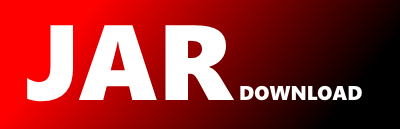
com.intuit.ipp.data.AdvancedInventoryPrefs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ipp-v3-java-data Show documentation
Show all versions of ipp-v3-java-data Show documentation
IPP Java V3 DevKit Data project - FMS Entities generation
//
// This file was generated by the Eclipse Implementation of JAXB, v2.3.7
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.07.12 at 12:19:07 PM IST
//
package com.intuit.ipp.data;
import java.io.Serializable;
import java.util.Date;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.intuit.sb.cdm.util.v3.DateAdapter;
import org.jvnet.jaxb2_commons.lang.Equals2;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy2;
import org.jvnet.jaxb2_commons.lang.HashCode2;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy2;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* QBW: only. Defines advance inventory Prefs details
*
*
* Java class for AdvancedInventoryPrefs complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="AdvancedInventoryPrefs">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="MLIAvailable" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="MLIEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="EnhancedInventoryReceivingEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="TrackingSerialOrLotNumber" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="TrackingOnSalesTransactionsEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="TrackingOnPurchaseTransactionsEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="TrackingOnInventoryAdjustmentEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="TrackingOnBuildAssemblyEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="FIFOEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="FIFOEffectiveDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* <element name="RowShelfBinEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="BarcodeEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AdvancedInventoryPrefs", propOrder = {
"mliAvailable",
"mliEnabled",
"enhancedInventoryReceivingEnabled",
"trackingSerialOrLotNumber",
"trackingOnSalesTransactionsEnabled",
"trackingOnPurchaseTransactionsEnabled",
"trackingOnInventoryAdjustmentEnabled",
"trackingOnBuildAssemblyEnabled",
"fifoEnabled",
"fifoEffectiveDate",
"rowShelfBinEnabled",
"barcodeEnabled"
})
public class AdvancedInventoryPrefs implements Serializable, Equals2, HashCode2
{
private final static long serialVersionUID = 1L;
@XmlElement(name = "MLIAvailable")
protected Boolean mliAvailable;
@XmlElement(name = "MLIEnabled")
protected Boolean mliEnabled;
@XmlElement(name = "EnhancedInventoryReceivingEnabled")
protected Boolean enhancedInventoryReceivingEnabled;
@XmlElement(name = "TrackingSerialOrLotNumber")
protected Boolean trackingSerialOrLotNumber;
@XmlElement(name = "TrackingOnSalesTransactionsEnabled")
protected Boolean trackingOnSalesTransactionsEnabled;
@XmlElement(name = "TrackingOnPurchaseTransactionsEnabled")
protected Boolean trackingOnPurchaseTransactionsEnabled;
@XmlElement(name = "TrackingOnInventoryAdjustmentEnabled")
protected Boolean trackingOnInventoryAdjustmentEnabled;
@XmlElement(name = "TrackingOnBuildAssemblyEnabled")
protected Boolean trackingOnBuildAssemblyEnabled;
@XmlElement(name = "FIFOEnabled")
protected Boolean fifoEnabled;
@XmlElement(name = "FIFOEffectiveDate", type = String.class)
@XmlJavaTypeAdapter(DateAdapter.class)
@XmlSchemaType(name = "date")
protected Date fifoEffectiveDate;
@XmlElement(name = "RowShelfBinEnabled")
protected Boolean rowShelfBinEnabled;
@XmlElement(name = "BarcodeEnabled")
protected Boolean barcodeEnabled;
/**
* Gets the value of the mliAvailable property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isMLIAvailable() {
return mliAvailable;
}
/**
* Sets the value of the mliAvailable property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setMLIAvailable(Boolean value) {
this.mliAvailable = value;
}
/**
* Gets the value of the mliEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isMLIEnabled() {
return mliEnabled;
}
/**
* Sets the value of the mliEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setMLIEnabled(Boolean value) {
this.mliEnabled = value;
}
/**
* Gets the value of the enhancedInventoryReceivingEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isEnhancedInventoryReceivingEnabled() {
return enhancedInventoryReceivingEnabled;
}
/**
* Sets the value of the enhancedInventoryReceivingEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setEnhancedInventoryReceivingEnabled(Boolean value) {
this.enhancedInventoryReceivingEnabled = value;
}
/**
* Gets the value of the trackingSerialOrLotNumber property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTrackingSerialOrLotNumber() {
return trackingSerialOrLotNumber;
}
/**
* Sets the value of the trackingSerialOrLotNumber property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTrackingSerialOrLotNumber(Boolean value) {
this.trackingSerialOrLotNumber = value;
}
/**
* Gets the value of the trackingOnSalesTransactionsEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTrackingOnSalesTransactionsEnabled() {
return trackingOnSalesTransactionsEnabled;
}
/**
* Sets the value of the trackingOnSalesTransactionsEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTrackingOnSalesTransactionsEnabled(Boolean value) {
this.trackingOnSalesTransactionsEnabled = value;
}
/**
* Gets the value of the trackingOnPurchaseTransactionsEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTrackingOnPurchaseTransactionsEnabled() {
return trackingOnPurchaseTransactionsEnabled;
}
/**
* Sets the value of the trackingOnPurchaseTransactionsEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTrackingOnPurchaseTransactionsEnabled(Boolean value) {
this.trackingOnPurchaseTransactionsEnabled = value;
}
/**
* Gets the value of the trackingOnInventoryAdjustmentEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTrackingOnInventoryAdjustmentEnabled() {
return trackingOnInventoryAdjustmentEnabled;
}
/**
* Sets the value of the trackingOnInventoryAdjustmentEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTrackingOnInventoryAdjustmentEnabled(Boolean value) {
this.trackingOnInventoryAdjustmentEnabled = value;
}
/**
* Gets the value of the trackingOnBuildAssemblyEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTrackingOnBuildAssemblyEnabled() {
return trackingOnBuildAssemblyEnabled;
}
/**
* Sets the value of the trackingOnBuildAssemblyEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTrackingOnBuildAssemblyEnabled(Boolean value) {
this.trackingOnBuildAssemblyEnabled = value;
}
/**
* Gets the value of the fifoEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isFIFOEnabled() {
return fifoEnabled;
}
/**
* Sets the value of the fifoEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setFIFOEnabled(Boolean value) {
this.fifoEnabled = value;
}
/**
* Gets the value of the fifoEffectiveDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public Date getFIFOEffectiveDate() {
return fifoEffectiveDate;
}
/**
* Sets the value of the fifoEffectiveDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFIFOEffectiveDate(Date value) {
this.fifoEffectiveDate = value;
}
/**
* Gets the value of the rowShelfBinEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRowShelfBinEnabled() {
return rowShelfBinEnabled;
}
/**
* Sets the value of the rowShelfBinEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRowShelfBinEnabled(Boolean value) {
this.rowShelfBinEnabled = value;
}
/**
* Gets the value of the barcodeEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isBarcodeEnabled() {
return barcodeEnabled;
}
/**
* Sets the value of the barcodeEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setBarcodeEnabled(Boolean value) {
this.barcodeEnabled = value;
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy2 strategy) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final AdvancedInventoryPrefs that = ((AdvancedInventoryPrefs) object);
{
Boolean lhsMLIAvailable;
lhsMLIAvailable = this.isMLIAvailable();
Boolean rhsMLIAvailable;
rhsMLIAvailable = that.isMLIAvailable();
if (!strategy.equals(LocatorUtils.property(thisLocator, "mliAvailable", lhsMLIAvailable), LocatorUtils.property(thatLocator, "mliAvailable", rhsMLIAvailable), lhsMLIAvailable, rhsMLIAvailable, (this.mliAvailable!= null), (that.mliAvailable!= null))) {
return false;
}
}
{
Boolean lhsMLIEnabled;
lhsMLIEnabled = this.isMLIEnabled();
Boolean rhsMLIEnabled;
rhsMLIEnabled = that.isMLIEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "mliEnabled", lhsMLIEnabled), LocatorUtils.property(thatLocator, "mliEnabled", rhsMLIEnabled), lhsMLIEnabled, rhsMLIEnabled, (this.mliEnabled!= null), (that.mliEnabled!= null))) {
return false;
}
}
{
Boolean lhsEnhancedInventoryReceivingEnabled;
lhsEnhancedInventoryReceivingEnabled = this.isEnhancedInventoryReceivingEnabled();
Boolean rhsEnhancedInventoryReceivingEnabled;
rhsEnhancedInventoryReceivingEnabled = that.isEnhancedInventoryReceivingEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "enhancedInventoryReceivingEnabled", lhsEnhancedInventoryReceivingEnabled), LocatorUtils.property(thatLocator, "enhancedInventoryReceivingEnabled", rhsEnhancedInventoryReceivingEnabled), lhsEnhancedInventoryReceivingEnabled, rhsEnhancedInventoryReceivingEnabled, (this.enhancedInventoryReceivingEnabled!= null), (that.enhancedInventoryReceivingEnabled!= null))) {
return false;
}
}
{
Boolean lhsTrackingSerialOrLotNumber;
lhsTrackingSerialOrLotNumber = this.isTrackingSerialOrLotNumber();
Boolean rhsTrackingSerialOrLotNumber;
rhsTrackingSerialOrLotNumber = that.isTrackingSerialOrLotNumber();
if (!strategy.equals(LocatorUtils.property(thisLocator, "trackingSerialOrLotNumber", lhsTrackingSerialOrLotNumber), LocatorUtils.property(thatLocator, "trackingSerialOrLotNumber", rhsTrackingSerialOrLotNumber), lhsTrackingSerialOrLotNumber, rhsTrackingSerialOrLotNumber, (this.trackingSerialOrLotNumber!= null), (that.trackingSerialOrLotNumber!= null))) {
return false;
}
}
{
Boolean lhsTrackingOnSalesTransactionsEnabled;
lhsTrackingOnSalesTransactionsEnabled = this.isTrackingOnSalesTransactionsEnabled();
Boolean rhsTrackingOnSalesTransactionsEnabled;
rhsTrackingOnSalesTransactionsEnabled = that.isTrackingOnSalesTransactionsEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "trackingOnSalesTransactionsEnabled", lhsTrackingOnSalesTransactionsEnabled), LocatorUtils.property(thatLocator, "trackingOnSalesTransactionsEnabled", rhsTrackingOnSalesTransactionsEnabled), lhsTrackingOnSalesTransactionsEnabled, rhsTrackingOnSalesTransactionsEnabled, (this.trackingOnSalesTransactionsEnabled!= null), (that.trackingOnSalesTransactionsEnabled!= null))) {
return false;
}
}
{
Boolean lhsTrackingOnPurchaseTransactionsEnabled;
lhsTrackingOnPurchaseTransactionsEnabled = this.isTrackingOnPurchaseTransactionsEnabled();
Boolean rhsTrackingOnPurchaseTransactionsEnabled;
rhsTrackingOnPurchaseTransactionsEnabled = that.isTrackingOnPurchaseTransactionsEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "trackingOnPurchaseTransactionsEnabled", lhsTrackingOnPurchaseTransactionsEnabled), LocatorUtils.property(thatLocator, "trackingOnPurchaseTransactionsEnabled", rhsTrackingOnPurchaseTransactionsEnabled), lhsTrackingOnPurchaseTransactionsEnabled, rhsTrackingOnPurchaseTransactionsEnabled, (this.trackingOnPurchaseTransactionsEnabled!= null), (that.trackingOnPurchaseTransactionsEnabled!= null))) {
return false;
}
}
{
Boolean lhsTrackingOnInventoryAdjustmentEnabled;
lhsTrackingOnInventoryAdjustmentEnabled = this.isTrackingOnInventoryAdjustmentEnabled();
Boolean rhsTrackingOnInventoryAdjustmentEnabled;
rhsTrackingOnInventoryAdjustmentEnabled = that.isTrackingOnInventoryAdjustmentEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "trackingOnInventoryAdjustmentEnabled", lhsTrackingOnInventoryAdjustmentEnabled), LocatorUtils.property(thatLocator, "trackingOnInventoryAdjustmentEnabled", rhsTrackingOnInventoryAdjustmentEnabled), lhsTrackingOnInventoryAdjustmentEnabled, rhsTrackingOnInventoryAdjustmentEnabled, (this.trackingOnInventoryAdjustmentEnabled!= null), (that.trackingOnInventoryAdjustmentEnabled!= null))) {
return false;
}
}
{
Boolean lhsTrackingOnBuildAssemblyEnabled;
lhsTrackingOnBuildAssemblyEnabled = this.isTrackingOnBuildAssemblyEnabled();
Boolean rhsTrackingOnBuildAssemblyEnabled;
rhsTrackingOnBuildAssemblyEnabled = that.isTrackingOnBuildAssemblyEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "trackingOnBuildAssemblyEnabled", lhsTrackingOnBuildAssemblyEnabled), LocatorUtils.property(thatLocator, "trackingOnBuildAssemblyEnabled", rhsTrackingOnBuildAssemblyEnabled), lhsTrackingOnBuildAssemblyEnabled, rhsTrackingOnBuildAssemblyEnabled, (this.trackingOnBuildAssemblyEnabled!= null), (that.trackingOnBuildAssemblyEnabled!= null))) {
return false;
}
}
{
Boolean lhsFIFOEnabled;
lhsFIFOEnabled = this.isFIFOEnabled();
Boolean rhsFIFOEnabled;
rhsFIFOEnabled = that.isFIFOEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "fifoEnabled", lhsFIFOEnabled), LocatorUtils.property(thatLocator, "fifoEnabled", rhsFIFOEnabled), lhsFIFOEnabled, rhsFIFOEnabled, (this.fifoEnabled!= null), (that.fifoEnabled!= null))) {
return false;
}
}
{
Date lhsFIFOEffectiveDate;
lhsFIFOEffectiveDate = this.getFIFOEffectiveDate();
Date rhsFIFOEffectiveDate;
rhsFIFOEffectiveDate = that.getFIFOEffectiveDate();
if (!strategy.equals(LocatorUtils.property(thisLocator, "fifoEffectiveDate", lhsFIFOEffectiveDate), LocatorUtils.property(thatLocator, "fifoEffectiveDate", rhsFIFOEffectiveDate), lhsFIFOEffectiveDate, rhsFIFOEffectiveDate, (this.fifoEffectiveDate!= null), (that.fifoEffectiveDate!= null))) {
return false;
}
}
{
Boolean lhsRowShelfBinEnabled;
lhsRowShelfBinEnabled = this.isRowShelfBinEnabled();
Boolean rhsRowShelfBinEnabled;
rhsRowShelfBinEnabled = that.isRowShelfBinEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "rowShelfBinEnabled", lhsRowShelfBinEnabled), LocatorUtils.property(thatLocator, "rowShelfBinEnabled", rhsRowShelfBinEnabled), lhsRowShelfBinEnabled, rhsRowShelfBinEnabled, (this.rowShelfBinEnabled!= null), (that.rowShelfBinEnabled!= null))) {
return false;
}
}
{
Boolean lhsBarcodeEnabled;
lhsBarcodeEnabled = this.isBarcodeEnabled();
Boolean rhsBarcodeEnabled;
rhsBarcodeEnabled = that.isBarcodeEnabled();
if (!strategy.equals(LocatorUtils.property(thisLocator, "barcodeEnabled", lhsBarcodeEnabled), LocatorUtils.property(thatLocator, "barcodeEnabled", rhsBarcodeEnabled), lhsBarcodeEnabled, rhsBarcodeEnabled, (this.barcodeEnabled!= null), (that.barcodeEnabled!= null))) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy2 strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public int hashCode(ObjectLocator locator, HashCodeStrategy2 strategy) {
int currentHashCode = 1;
{
Boolean theMLIAvailable;
theMLIAvailable = this.isMLIAvailable();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "mliAvailable", theMLIAvailable), currentHashCode, theMLIAvailable, (this.mliAvailable!= null));
}
{
Boolean theMLIEnabled;
theMLIEnabled = this.isMLIEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "mliEnabled", theMLIEnabled), currentHashCode, theMLIEnabled, (this.mliEnabled!= null));
}
{
Boolean theEnhancedInventoryReceivingEnabled;
theEnhancedInventoryReceivingEnabled = this.isEnhancedInventoryReceivingEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "enhancedInventoryReceivingEnabled", theEnhancedInventoryReceivingEnabled), currentHashCode, theEnhancedInventoryReceivingEnabled, (this.enhancedInventoryReceivingEnabled!= null));
}
{
Boolean theTrackingSerialOrLotNumber;
theTrackingSerialOrLotNumber = this.isTrackingSerialOrLotNumber();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "trackingSerialOrLotNumber", theTrackingSerialOrLotNumber), currentHashCode, theTrackingSerialOrLotNumber, (this.trackingSerialOrLotNumber!= null));
}
{
Boolean theTrackingOnSalesTransactionsEnabled;
theTrackingOnSalesTransactionsEnabled = this.isTrackingOnSalesTransactionsEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "trackingOnSalesTransactionsEnabled", theTrackingOnSalesTransactionsEnabled), currentHashCode, theTrackingOnSalesTransactionsEnabled, (this.trackingOnSalesTransactionsEnabled!= null));
}
{
Boolean theTrackingOnPurchaseTransactionsEnabled;
theTrackingOnPurchaseTransactionsEnabled = this.isTrackingOnPurchaseTransactionsEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "trackingOnPurchaseTransactionsEnabled", theTrackingOnPurchaseTransactionsEnabled), currentHashCode, theTrackingOnPurchaseTransactionsEnabled, (this.trackingOnPurchaseTransactionsEnabled!= null));
}
{
Boolean theTrackingOnInventoryAdjustmentEnabled;
theTrackingOnInventoryAdjustmentEnabled = this.isTrackingOnInventoryAdjustmentEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "trackingOnInventoryAdjustmentEnabled", theTrackingOnInventoryAdjustmentEnabled), currentHashCode, theTrackingOnInventoryAdjustmentEnabled, (this.trackingOnInventoryAdjustmentEnabled!= null));
}
{
Boolean theTrackingOnBuildAssemblyEnabled;
theTrackingOnBuildAssemblyEnabled = this.isTrackingOnBuildAssemblyEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "trackingOnBuildAssemblyEnabled", theTrackingOnBuildAssemblyEnabled), currentHashCode, theTrackingOnBuildAssemblyEnabled, (this.trackingOnBuildAssemblyEnabled!= null));
}
{
Boolean theFIFOEnabled;
theFIFOEnabled = this.isFIFOEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "fifoEnabled", theFIFOEnabled), currentHashCode, theFIFOEnabled, (this.fifoEnabled!= null));
}
{
Date theFIFOEffectiveDate;
theFIFOEffectiveDate = this.getFIFOEffectiveDate();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "fifoEffectiveDate", theFIFOEffectiveDate), currentHashCode, theFIFOEffectiveDate, (this.fifoEffectiveDate!= null));
}
{
Boolean theRowShelfBinEnabled;
theRowShelfBinEnabled = this.isRowShelfBinEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "rowShelfBinEnabled", theRowShelfBinEnabled), currentHashCode, theRowShelfBinEnabled, (this.rowShelfBinEnabled!= null));
}
{
Boolean theBarcodeEnabled;
theBarcodeEnabled = this.isBarcodeEnabled();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "barcodeEnabled", theBarcodeEnabled), currentHashCode, theBarcodeEnabled, (this.barcodeEnabled!= null));
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy2 strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy