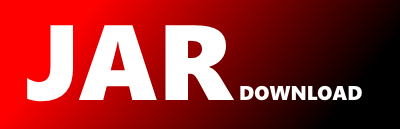
com.inversoft.rest.JSONResponseHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of restify Show documentation
Show all versions of restify Show documentation
The Java 8 REST Client used in our commercial REST Client libraries such as Passport Java Client and our Java 8 Chef Client called Barista
/*
* Copyright (c) 2016, Inversoft Inc., All Rights Reserved
*/
package com.inversoft.rest;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.inversoft.json.JacksonModule;
/**
* Response handler that reads the body as JSON using Jackson. By default, this uses the defaultObjectMapper
variable for
* JSON parsing. You can optionally specify a different ObjectMapper to the constructor. The default ObjectMapper uses Jackson's standard
* ObjectMapper configuration for deserializing. It also uses the JacksonModule from the jackson5
library for handling various
* type conversions.
*
* @author Brian Pontarelli
*/
public class JSONResponseHandler implements RESTClient.ResponseHandler {
public final static ObjectMapper defaultObjectMapper = new ObjectMapper().registerModule(new JacksonModule());
private final ObjectMapper instanceObjectMapper;
private final Class type;
public JSONResponseHandler(Class type) {
this.type = type;
this.instanceObjectMapper = defaultObjectMapper;
}
public JSONResponseHandler(Class type, ObjectMapper objectMapper) {
this.type = type;
this.instanceObjectMapper = objectMapper;
}
@Override
public T apply(InputStream is) throws IOException {
if (is == null) {
return null;
}
// Read a single byte of data to see if the stream is empty but then reset the stream back 0
BufferedInputStream bis = new BufferedInputStream(is, 1024);
bis.mark(1024);
int c = bis.read();
if (c == -1) {
return null;
}
bis.reset();
try {
return instanceObjectMapper.readValue(bis, type);
} catch (IOException e) {
throw new JSONException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy