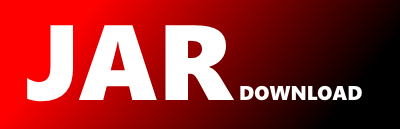
com.inversoft.rest.RESTClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of restify Show documentation
Show all versions of restify Show documentation
The Java 8 REST Client used in our commercial REST Client libraries such as Passport Java Client and our Java 8 Chef Client called Barista
/*
* Copyright (c) 2016-2017, Inversoft Inc., All Rights Reserved
*/
package com.inversoft.rest;
import javax.net.ssl.HttpsURLConnection;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URI;
import java.net.URL;
import java.net.URLEncoder;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import java.util.Base64;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Objects;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.inversoft.net.ssl.SSLTools;
/**
* RESTful WebService call builder. This provides the ability to call RESTful WebServices using a builder pattern to
* set up all the necessary request information and parse the response.
*
* @author Brian Pontarelli
*/
public class RESTClient {
private static final Logger logger = LoggerFactory.getLogger(RESTClient.class);
public final Map headers = new HashMap<>();
public final Map> parameters = new LinkedHashMap<>();
public final StringBuilder url = new StringBuilder();
public BodyHandler bodyHandler;
public String certificate;
public int connectTimeout = 2000;
public ResponseHandler errorResponseHandler;
public Class errorType;
public String key;
public HTTPMethod method;
public int readTimeout = 2000;
public ResponseHandler successResponseHandler;
public Class successType;
public RESTClient(Class successType, Class errorType) {
if (successType == Void.class || errorType == Void.class) {
throw new IllegalArgumentException("Void.class isn't valid. Use Void.TYPE instead.");
}
this.successType = successType;
this.errorType = errorType;
}
public RESTClient authorization(String key) {
this.headers.put("Authorization", key);
return this;
}
public RESTClient basicAuthorization(String username, String password) {
if (username != null && password != null) {
String credentials = username + ":" + password;
Base64.Encoder encoder = Base64.getEncoder();
String encoded = encoder.encodeToString(credentials.getBytes());
this.headers.put("Authorization", "Basic " + encoded);
}
return this;
}
public RESTClient bodyHandler(BodyHandler bodyHandler) {
this.bodyHandler = bodyHandler;
return this;
}
public RESTClient certificate(String certificate) {
this.certificate = certificate;
return this;
}
public RESTClient connectTimeout(int connectTimeout) {
this.connectTimeout = connectTimeout;
return this;
}
public RESTClient delete() {
this.method = HTTPMethod.DELETE;
return this;
}
public RESTClient errorResponseHandler(ResponseHandler errorResponseHandler) {
this.errorResponseHandler = errorResponseHandler;
return this;
}
public RESTClient get() {
this.method = HTTPMethod.GET;
return this;
}
public URI getURI() {
return URI.create(url.toString());
}
public ClientResponse go() {
if (url.length() == 0) {
throw new IllegalStateException("You must specify a URL");
}
Objects.requireNonNull(method, "You must specify a HTTP method");
if (successType != Void.TYPE && successResponseHandler == null) {
throw new IllegalStateException("You specified a success response type, you must then provide a success response handler.");
}
if (errorType != Void.TYPE && errorResponseHandler == null) {
throw new IllegalStateException("You specified an error response type, you must then provide an error response handler.");
}
ClientResponse response = new ClientResponse<>();
response.request = (bodyHandler != null) ? bodyHandler.getBodyObject() : null;
response.method = method;
HttpURLConnection huc;
try {
if (parameters.size() > 0) {
if (url.indexOf("?") == -1) {
url.append("?");
}
for (Iterator>> i = parameters.entrySet().iterator(); i.hasNext(); ) {
Entry> entry = i.next();
for (Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy