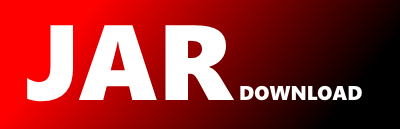
com.invms.x.entities.basis.HtmlEntity Maven / Gradle / Ivy
The newest version!
package com.invms.x.entities.basis;
import java.io.Serializable;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.ForeignKey;
import javax.persistence.Id;
import javax.persistence.Index;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.Table;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.invms.x.builders.QueryBuilder;
import com.invms.x.filters.EntityFilter;
import com.invms.x.filters.FieldFilter;
import com.invms.x.filters.HtmlFilter;
import com.invms.x.models.basis.HtmlModel;
import com.invms.x.utils.ComUtils;
import com.invms.x.utils.XUtils;
@FieldFilter(markOnly = true)
@EntityFilter(viewEnable = true, code = HtmlEntity.BASE_CODE, name = "网页", sortIndex = HtmlEntity.BASE_SORT_INDEX, sortOptions = "sortName:'sortIndex',sortOrder:'asc'", parentCode = IBasisEntity.BASE_CODE)
@Table(name = "t_" + HtmlEntity.BASE_CODE, indexes = { @Index(name = "i_html_code", columnList = "c_code"), @Index(name = "i_html_category", columnList = "c_category"), @Index(name = "i_html_sort_index", columnList = "c_sort_index"), @Index(name = "i_html_deleted", columnList = "c_deleted") })
@Entity
public class HtmlEntity extends BaseHierarchicEntity implements Serializable {
private static final long serialVersionUID = -4161079333373506688L;
public static final String BASE_CODE = IBasisEntity.BASE_CODE + "_html";
public static final long BASE_SORT_INDEX = IBasisEntity.BASE_SORT_INDEX + 9600;
public static void setQueryBuilder(Class> resultClass, String queryMethod, QueryBuilder queryBuilder) {
StringBuilder stringBuilder = new StringBuilder();
if (resultClass != null && HtmlModel.class.isAssignableFrom(resultClass)) {
stringBuilder.append("select new " + resultClass.getName());
stringBuilder.append("(t.id, t.code, t.name, t.category");
stringBuilder.append(", t.description, t.keywords");
stringBuilder.append(", t.hierarchy, t.parentId, t.remark, t.sortIndex");
stringBuilder.append(", t.updateUser, t.updateTime, t.insertUser, t.insertTime, t.deleted");
stringBuilder.append(", tParent.code, tParent.name)");
stringBuilder.append(" from HtmlEntity t");
}
switch (queryMethod) {
case EntityFilter.METHOD_INSERT:
case EntityFilter.METHOD_UPDATE:
case EntityFilter.METHOD_DELETE:
case EntityFilter.METHOD_CLEAR:
break;
case EntityFilter.METHOD_COUNT:
case EntityFilter.METHOD_EXIST:
case EntityFilter.METHOD_SINGLE:
case EntityFilter.METHOD_RANGE:
case EntityFilter.METHOD_PAGINATE:
stringBuilder.append(" left join t.parent tParent");
break;
}
queryBuilder.setQuery(stringBuilder.toString());
}
public static HtmlEntity fromFilter(Class> entityClass, EntityFilter entityFilter, HtmlEntity entity, HtmlFilter filter) throws Exception {
entity.setCode(XUtils.buildEntityFilter(entityClass, entityFilter, entity.getCode()));
boolean mustCover = entityFilter.mustCover();
if (mustCover && !ComUtils.empty(entityFilter.name())) {
entity.setName(entityFilter.name());
} else {
entity.setName(XUtils.buildEntityFilter(entityClass, entityFilter, entity.getName()));
}
if (entity.getSortIndex() == null && entityFilter.sortIndex() != 0) {
entity.setSortIndex(entityFilter.sortIndex());
}
return entity;
}
public static HtmlEntity fromFilter(HtmlFilter filter, HtmlEntity entity) throws Exception {
if (entity == null) {
entity = new HtmlEntity();
}
if (!ComUtils.empty(filter.code())) {
entity.setCode(filter.code());
}
if (!ComUtils.empty(filter.name())) {
entity.setName(filter.name());
}
if (!ComUtils.empty(filter.category())) {
entity.setCategory(filter.category());
}
if (!ComUtils.empty(filter.description())) {
entity.setDescription(filter.description());
}
if (!ComUtils.empty(filter.keywords())) {
entity.setKeywords(filter.keywords());
}
if (!ComUtils.empty(filter.remark())) {
entity.setRemark(filter.remark());
}
if (filter.sortIndex() != 0) {
entity.setSortIndex(filter.sortIndex());
}
entity.setDeleted(filter.deleted());
return entity;
}
@FieldFilter(code = BASE_CODE + "_id", name = "网页主键", dataType = FieldFilter.DATA_TYPE_STRING, outputEnable = true, sortIndex = BASE_SORT_INDEX + 1, parentCode = BASE_CODE)
@Id
@Column(name = "p_id", length = 32, nullable = false)
protected String id;
@FieldFilter(code = BASE_CODE + "_description", name = "网页描述", dataType = FieldFilter.DATA_TYPE_STRING, queryEnable = true, listEnable = true, listVisible = true, sortEnable = true, detailEnable = true, insertEnable = true, updateEnable = true, inputEnable = true, outputEnable = true, sortIndex = BASE_SORT_INDEX + 5, parentCode = BASE_CODE)
@Column(name = "c_description", length = 256)
protected String description;
@FieldFilter(code = BASE_CODE + "_keywords", name = "网页关键字", dataType = FieldFilter.DATA_TYPE_STRING, queryEnable = true, listEnable = true, listVisible = true, sortEnable = true, detailEnable = true, insertEnable = true, updateEnable = true, inputEnable = true, outputEnable = true, sortIndex = BASE_SORT_INDEX + 6, parentCode = BASE_CODE)
@Column(name = "c_keywords", length = 128)
protected String keywords;
@JsonIgnore
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "f_parent_id", foreignKey = @ForeignKey(name = "i_html_parent_id"), insertable = false, updatable = false)
private HtmlEntity parent;
@Override
public String getId() {
return id;
}
@Override
public void setId(String id) {
this.id = id;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getKeywords() {
return keywords;
}
public void setKeywords(String keywords) {
this.keywords = keywords;
}
public HtmlEntity() {
id = XUtils.buildId();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy