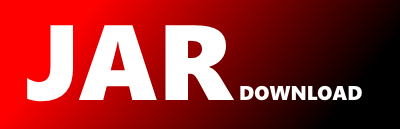
com.invms.x.entities.basis.OrganizationEntity Maven / Gradle / Ivy
The newest version!
package com.invms.x.entities.basis;
import java.io.Serializable;
import java.util.List;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.ForeignKey;
import javax.persistence.Id;
import javax.persistence.Index;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.Table;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.invms.x.builders.ArgsBuilder;
import com.invms.x.builders.QueryBuilder;
import com.invms.x.filters.EntityFilter;
import com.invms.x.filters.FieldFilter;
import com.invms.x.filters.OrganizationFilter;
import com.invms.x.models.basis.OrganizationModel;
import com.invms.x.repositories.basis.BasisRepository;
import com.invms.x.services.basis.AuthorizeService;
import com.invms.x.utils.ComUtils;
import com.invms.x.utils.XUtils;
@FieldFilter(markOnly = true)
@EntityFilter(viewEnable = true, code = OrganizationEntity.BASE_CODE, name = "机构", sortIndex = OrganizationEntity.BASE_SORT_INDEX, sortOptions = "sortName:'sortIndex',sortOrder:'asc'", parentCode = IBasisEntity.BASE_CODE)
@Table(name = "t_" + OrganizationEntity.BASE_CODE, indexes = { @Index(name = "i_organization_code", columnList = "c_code"), @Index(name = "i_organization_category", columnList = "c_category"), @Index(name = "i_organization_sort_index", columnList = "c_sort_index"), @Index(name = "i_organization_deleted", columnList = "c_deleted") })
@Entity
public class OrganizationEntity extends BaseHierarchicEntity implements Serializable {
private static final long serialVersionUID = -540507259916433279L;
public static final String BASE_CODE = IBasisEntity.BASE_CODE + "_organization";
public static final long BASE_SORT_INDEX = IBasisEntity.BASE_SORT_INDEX + 9100;
public static void setQueryBuilder(Class> resultClass, String queryMethod, QueryBuilder queryBuilder) {
StringBuilder stringBuilder = new StringBuilder();
if (resultClass != null && OrganizationModel.class.isAssignableFrom(resultClass)) {
stringBuilder.append("select new " + resultClass.getName());
stringBuilder.append("(t.id, t.code, t.name, t.category");
stringBuilder.append(", t.shortName, t.contactName, t.contactPhone, t.contactAddress, t.divisionId");
stringBuilder.append(", t.hierarchy, t.parentId, t.remark, t.sortIndex");
stringBuilder.append(", t.updateUser, t.updateTime, t.insertUser, t.insertTime, t.deleted");
stringBuilder.append(", tParent.code, tParent.name, tDivision.code, tDivision.name, tDivision.shortName)");
stringBuilder.append(" from OrganizationEntity t");
}
switch (queryMethod) {
case EntityFilter.METHOD_INSERT:
case EntityFilter.METHOD_UPDATE:
case EntityFilter.METHOD_DELETE:
case EntityFilter.METHOD_CLEAR:
break;
case EntityFilter.METHOD_COUNT:
case EntityFilter.METHOD_EXIST:
case EntityFilter.METHOD_SINGLE:
case EntityFilter.METHOD_RANGE:
case EntityFilter.METHOD_PAGINATE:
stringBuilder.append(" left join t.parent tParent");
stringBuilder.append(" left join t.division tDivision");
break;
}
queryBuilder.setQuery(stringBuilder.toString());
}
public static void setArgsBuilder(String queryMethod, ArgsBuilder argsBuilder) throws Exception {
switch (queryMethod) {
case EntityFilter.METHOD_INSERT:
case EntityFilter.METHOD_UPDATE:
case EntityFilter.METHOD_DELETE:
break;
case EntityFilter.METHOD_CLEAR:
case EntityFilter.METHOD_COUNT:
case EntityFilter.METHOD_EXIST:
case EntityFilter.METHOD_SINGLE:
case EntityFilter.METHOD_RANGE:
case EntityFilter.METHOD_PAGINATE:
AuthorizeService authorizeService = XUtils.getBean(AuthorizeService.class);
if (authorizeService.isRequireAuthorize(argsBuilder) && authorizeService.isOrganizationPowerEnable()) {
List ids = authorizeService.getLoggedOrganizationIds(argsBuilder);
if (ids.isEmpty()) {
argsBuilder.setValue("id_in", new String[] { "" });
} else {
argsBuilder.setValue("id_in", ids.toArray(new String[ids.size()]));
}
}
break;
}
}
public static void setInsert(OrganizationEntity entity, ArgsBuilder argsBuilder) throws Exception {
AuthorizeService authorizeService = XUtils.getBean(AuthorizeService.class);
if (authorizeService.isOrganizationPowerEnable()) {
BasisRepository basisRepository = XUtils.getBean(BasisRepository.class);
basisRepository.setLoggedPower(entity, argsBuilder);
}
}
public static OrganizationEntity fromFilter(OrganizationFilter filter, OrganizationEntity entity) throws Exception {
if (entity == null) {
entity = new OrganizationEntity();
}
if (!ComUtils.empty(filter.code())) {
entity.setCode(filter.code());
}
if (!ComUtils.empty(filter.name())) {
entity.setName(filter.name());
}
if (!ComUtils.empty(filter.category())) {
entity.setCategory(filter.category());
}
if (!ComUtils.empty(filter.shortName())) {
entity.setShortName(filter.shortName());
}
if (!ComUtils.empty(filter.contactName())) {
entity.setContactName(filter.contactName());
}
if (!ComUtils.empty(filter.contactPhone())) {
entity.setContactPhone(filter.contactPhone());
}
if (!ComUtils.empty(filter.contactAddress())) {
entity.setContactAddress(filter.contactAddress());
}
if (!ComUtils.empty(filter.remark())) {
entity.setRemark(filter.remark());
}
if (filter.sortIndex() != 0) {
entity.setSortIndex(filter.sortIndex());
}
entity.setDeleted(filter.deleted());
return entity;
}
@FieldFilter(code = BASE_CODE + "_id", name = "机构主键", dataType = FieldFilter.DATA_TYPE_STRING, outputEnable = true, sortIndex = BASE_SORT_INDEX + 1, parentCode = BASE_CODE)
@Id
@Column(name = "p_id", length = 32, nullable = false)
protected String id;
@FieldFilter(code = BASE_CODE + "_shortName", name = "机构简称", dataType = FieldFilter.DATA_TYPE_STRING, queryEnable = true, listEnable = true, listVisible = true, sortEnable = true, detailEnable = true, insertEnable = true, updateEnable = true, inputEnable = true, outputEnable = true, sortIndex = BASE_SORT_INDEX + 5, parentCode = BASE_CODE)
@Column(name = "c_short_name", length = 32)
protected String shortName;
@FieldFilter(code = BASE_CODE + "_contactName", name = "联系人员", dataType = FieldFilter.DATA_TYPE_STRING, queryEnable = true, listEnable = true, listVisible = true, sortEnable = true, detailEnable = true, insertEnable = true, updateEnable = true, inputEnable = true, outputEnable = true, sortIndex = BASE_SORT_INDEX + 6, parentCode = BASE_CODE)
@Column(name = "c_contact_name", length = 32)
protected String contactName;
@FieldFilter(code = BASE_CODE + "_contactPhone", name = "联系电话", dataType = FieldFilter.DATA_TYPE_STRING, queryEnable = true, listEnable = true, listVisible = true, sortEnable = true, detailEnable = true, insertEnable = true, updateEnable = true, inputEnable = true, outputEnable = true, sortIndex = BASE_SORT_INDEX + 7, parentCode = BASE_CODE)
@Column(name = "c_contact_phone", length = 32)
protected String contactPhone;
@FieldFilter(code = BASE_CODE + "_contactAddress", name = "联系地址", dataType = FieldFilter.DATA_TYPE_STRING, queryEnable = true, listEnable = true, sortEnable = true, detailEnable = true, insertEnable = true, updateEnable = true, inputEnable = true, outputEnable = true, sortIndex = BASE_SORT_INDEX + 8, parentCode = BASE_CODE)
@Column(name = "c_contact_address", length = 256)
protected String contactAddress;
@FieldFilter(code = BASE_CODE + "_divisionId", name = "区域主键", category = "所属区域", dataType = FieldFilter.DATA_TYPE_FOREIGN, detailEnable = true, insertEnable = true, updateEnable = true, inputEnable = true, outputEnable = true, foreignCode = DivisionEntity.BASE_CODE, foreignOptions = "url:'{apiRoot}/entity/basis/division/simple'", sortIndex = BASE_SORT_INDEX + 90, parentCode = BASE_CODE)
@Column(name = "f_division_id", length = 32)
protected String divisionId;
@JsonIgnore
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "f_division_id", foreignKey = @ForeignKey(name = "i_organization_division_id"), insertable = false, updatable = false)
private DivisionEntity division;
@JsonIgnore
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "f_parent_id", foreignKey = @ForeignKey(name = "i_organization_parent_id"), insertable = false, updatable = false)
private OrganizationEntity parent;
@Override
public String getId() {
return id;
}
@Override
public void setId(String id) {
this.id = id;
}
@Override
public String getText() {
return ComUtils.getNotEmpty(text, shortName, name, code);
}
public String getShortName() {
return shortName;
}
public void setShortName(String shortName) {
this.shortName = shortName;
}
public String getContactName() {
return contactName;
}
public void setContactName(String contactName) {
this.contactName = contactName;
}
public String getContactPhone() {
return contactPhone;
}
public void setContactPhone(String contactPhone) {
this.contactPhone = contactPhone;
}
public String getContactAddress() {
return contactAddress;
}
public void setContactAddress(String contactAddress) {
this.contactAddress = contactAddress;
}
public String getDivisionId() {
return divisionId;
}
public void setDivisionId(String divisionId) {
this.divisionId = divisionId;
}
public OrganizationEntity() {
id = XUtils.buildId();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy