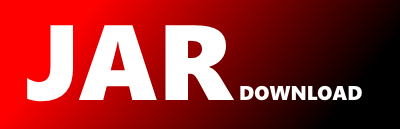
com.io7m.cardant.tests.main.CAMainCommandsDocumentation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.io7m.cardant.tests Show documentation
Show all versions of com.io7m.cardant.tests Show documentation
Inventory system (Test suite)
The newest version!
/*
* Copyright © 2023 Mark Raynsford https://www.io7m.com
*
* Permission to use, copy, modify, and/or distribute this software for any
* purpose with or without fee is hereby granted, provided that the above
* copyright notice and this permission notice appear in all copies.
*
* THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
* WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
* MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY
* SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
* WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
* ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
* IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
*/
package com.io7m.cardant.tests.main;
import com.io7m.cardant.main.CAMain;
import com.io7m.cardant.shell.CAShellValueConverters;
import com.io7m.cardant.strings.CAStrings;
import com.io7m.quarrel.core.QCommandGroupType;
import com.io7m.quarrel.core.QCommandOrGroupType;
import com.io7m.quarrel.core.QCommandParserConfiguration;
import com.io7m.quarrel.core.QCommandParsers;
import com.io7m.quarrel.core.QCommandType;
import com.io7m.quarrel.ext.xstructural.QCommandXS;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
import java.util.Locale;
public final class CAMainCommandsDocumentation
{
private CAMainCommandsDocumentation()
{
}
private record CACommandPath(
List path,
QCommandType command)
{
}
private static void commands(
final ArrayList outputs,
final ArrayList path,
final QCommandOrGroupType cg)
{
switch (cg) {
case final QCommandGroupType g -> {
try {
path.add(cg.metadata().name());
for (final var c : g.commandTree().values()) {
commands(outputs, path, c);
}
} finally {
path.removeLast();
}
}
case final QCommandType c -> {
try {
path.add(c.metadata().name());
outputs.add(new CACommandPath(List.copyOf(path), c));
} finally {
path.removeLast();
}
}
};
}
public static void main(
final String[] args)
throws Exception
{
final var parsers =
new QCommandParsers();
final var xs =
new QCommandXS("xs", true);
final var parserConfig =
new QCommandParserConfiguration(
CAShellValueConverters.create(CAStrings.create(Locale.ROOT)),
QCommandParsers.emptyResources()
);
final var main =
new CAMain(new String[] { });
final var app =
main.application();
final var outputs =
new ArrayList();
final var path =
new ArrayList();
for (final var e : app.commandTree().entrySet()) {
commands(outputs, path, e.getValue());
}
for (final var command : outputs) {
try {
final var parser =
parsers.create(parserConfig);
{
final var textWriter =
new StringWriter();
final var writer =
new PrintWriter(textWriter);
final var parameters = new ArrayList();
parameters.add("--type");
parameters.add("parameters");
parameters.addAll(command.path);
final var context =
parser.execute(
app.commandTree(),
writer,
xs,
parameters
);
context.execute();
writer.println();
writer.flush();
final var name =
String.join("-", command.path());
final var paramsFile =
Paths.get("/shared-tmp/mcmd-%s-parameters.xml".formatted(name));
Files.writeString(
paramsFile,
textWriter.toString(),
StandardCharsets.UTF_8
);
}
} catch (final Exception e) {
e.printStackTrace();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy