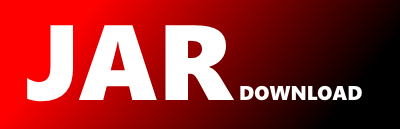
com.io7m.changelog.core.CVersionStandard Maven / Gradle / Ivy
package com.io7m.changelog.core;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Generated;
/**
*
* The type of standard version numbers of the form {@code M.N.P-S},
* where {@code M} is the major version, {@code N} is the minor
* version, {@code P} is the patch number, and {@code S} is an
* arbitrary qualifier string.
*
*/
@SuppressWarnings({"all"})
@Generated({"Immutables.generator", "CVersionStandardType"})
public final class CVersionStandard implements CVersionStandardType {
private final int major;
private final int minor;
private final int patch;
private final String qualifier;
private CVersionStandard(int major, int minor, int patch, String qualifier) {
this.major = major;
this.minor = minor;
this.patch = patch;
this.qualifier = Objects.requireNonNull(qualifier, "qualifier");
}
private CVersionStandard(CVersionStandard original, int major, int minor, int patch, String qualifier) {
this.major = major;
this.minor = minor;
this.patch = patch;
this.qualifier = qualifier;
}
/**
* @return The major version number
*/
@Override
public int major() {
return major;
}
/**
* @return The minor version number
*/
@Override
public int minor() {
return minor;
}
/**
* @return The patch number
*/
@Override
public int patch() {
return patch;
}
/**
* @return The qualifier component of the version number
*/
@Override
public String qualifier() {
return qualifier;
}
/**
* Copy the current immutable object by setting a value for the {@link CVersionStandardType#major() major} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for major
* @return A modified copy of the {@code this} object
*/
public final CVersionStandard withMajor(int value) {
if (this.major == value) return this;
return validate(new CVersionStandard(this, value, this.minor, this.patch, this.qualifier));
}
/**
* Copy the current immutable object by setting a value for the {@link CVersionStandardType#minor() minor} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for minor
* @return A modified copy of the {@code this} object
*/
public final CVersionStandard withMinor(int value) {
if (this.minor == value) return this;
return validate(new CVersionStandard(this, this.major, value, this.patch, this.qualifier));
}
/**
* Copy the current immutable object by setting a value for the {@link CVersionStandardType#patch() patch} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for patch
* @return A modified copy of the {@code this} object
*/
public final CVersionStandard withPatch(int value) {
if (this.patch == value) return this;
return validate(new CVersionStandard(this, this.major, this.minor, value, this.qualifier));
}
/**
* Copy the current immutable object by setting a value for the {@link CVersionStandardType#qualifier() qualifier} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for qualifier
* @return A modified copy of the {@code this} object
*/
public final CVersionStandard withQualifier(String value) {
if (this.qualifier.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "qualifier");
return validate(new CVersionStandard(this, this.major, this.minor, this.patch, newValue));
}
/**
* This instance is equal to all instances of {@code CVersionStandard} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof CVersionStandard
&& equalTo((CVersionStandard) another);
}
private boolean equalTo(CVersionStandard another) {
return major == another.major
&& minor == another.minor
&& patch == another.patch
&& qualifier.equals(another.qualifier);
}
/**
* Computes a hash code from attributes: {@code major}, {@code minor}, {@code patch}, {@code qualifier}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + major;
h = h * 17 + minor;
h = h * 17 + patch;
h = h * 17 + qualifier.hashCode();
return h;
}
/**
* Prints the immutable value {@code CVersionStandard} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "CVersionStandard{"
+ "major=" + major
+ ", minor=" + minor
+ ", patch=" + patch
+ ", qualifier=" + qualifier
+ "}";
}
/**
* Construct a new immutable {@code CVersionStandard} instance.
* @param major The value for the {@code major} attribute
* @param minor The value for the {@code minor} attribute
* @param patch The value for the {@code patch} attribute
* @param qualifier The value for the {@code qualifier} attribute
* @return An immutable CVersionStandard instance
*/
public static CVersionStandard of(int major, int minor, int patch, String qualifier) {
return validate(new CVersionStandard(major, minor, patch, qualifier));
}
private static CVersionStandard validate(CVersionStandard instance) {
instance.checkPreconditions();
return instance;
}
/**
* Creates an immutable copy of a {@link CVersionStandardType} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CVersionStandard instance
*/
public static CVersionStandard copyOf(CVersionStandardType instance) {
if (instance instanceof CVersionStandard) {
return (CVersionStandard) instance;
}
return CVersionStandard.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link CVersionStandard CVersionStandard}.
* @return A new CVersionStandard builder
*/
public static CVersionStandard.Builder builder() {
return new CVersionStandard.Builder();
}
/**
* Builds instances of type {@link CVersionStandard CVersionStandard}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_MAJOR = 0x1L;
private static final long INIT_BIT_MINOR = 0x2L;
private static final long INIT_BIT_PATCH = 0x4L;
private static final long INIT_BIT_QUALIFIER = 0x8L;
private long initBits = 0xfL;
private int major;
private int minor;
private int patch;
private String qualifier;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code CVersionStandardType} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CVersionStandardType instance) {
Objects.requireNonNull(instance, "instance");
setMajor(instance.major());
setMinor(instance.minor());
setPatch(instance.patch());
setQualifier(instance.qualifier());
return this;
}
/**
* Initializes the value for the {@link CVersionStandardType#major() major} attribute.
* @param major The value for major
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setMajor(int major) {
this.major = major;
initBits &= ~INIT_BIT_MAJOR;
return this;
}
/**
* Initializes the value for the {@link CVersionStandardType#minor() minor} attribute.
* @param minor The value for minor
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setMinor(int minor) {
this.minor = minor;
initBits &= ~INIT_BIT_MINOR;
return this;
}
/**
* Initializes the value for the {@link CVersionStandardType#patch() patch} attribute.
* @param patch The value for patch
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPatch(int patch) {
this.patch = patch;
initBits &= ~INIT_BIT_PATCH;
return this;
}
/**
* Initializes the value for the {@link CVersionStandardType#qualifier() qualifier} attribute.
* @param qualifier The value for qualifier
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setQualifier(String qualifier) {
this.qualifier = Objects.requireNonNull(qualifier, "qualifier");
initBits &= ~INIT_BIT_QUALIFIER;
return this;
}
/**
* Builds a new {@link CVersionStandard CVersionStandard}.
* @return An immutable instance of CVersionStandard
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public CVersionStandard build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return CVersionStandard.validate(new CVersionStandard(null, major, minor, patch, qualifier));
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList();
if ((initBits & INIT_BIT_MAJOR) != 0) attributes.add("major");
if ((initBits & INIT_BIT_MINOR) != 0) attributes.add("minor");
if ((initBits & INIT_BIT_PATCH) != 0) attributes.add("patch");
if ((initBits & INIT_BIT_QUALIFIER) != 0) attributes.add("qualifier");
return "Cannot build CVersionStandard, some of required attributes are not set " + attributes;
}
}
}