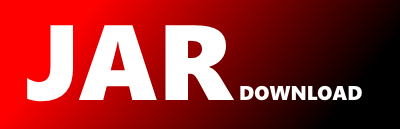
com.io7m.ethermaker.core.MACAddress Maven / Gradle / Ivy
Show all versions of com.io7m.ethermaker.core Show documentation
package com.io7m.ethermaker.core;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* A MAC address.
*/
@SuppressWarnings({"all"})
public final class MACAddress extends MACAddressType {
private final int octet0;
private final int octet1;
private final int octet2;
private final int octet3;
private final int octet4;
private final int octet5;
private MACAddress(int octet0, int octet1, int octet2, int octet3, int octet4, int octet5) {
this.octet0 = octet0;
this.octet1 = octet1;
this.octet2 = octet2;
this.octet3 = octet3;
this.octet4 = octet4;
this.octet5 = octet5;
}
/**
* @return Octet 0
*/
@Override
public int octet0() {
return octet0;
}
/**
* @return Octet 1
*/
@Override
public int octet1() {
return octet1;
}
/**
* @return Octet 2
*/
@Override
public int octet2() {
return octet2;
}
/**
* @return Octet 3
*/
@Override
public int octet3() {
return octet3;
}
/**
* @return Octet 4
*/
@Override
public int octet4() {
return octet4;
}
/**
* @return Octet 5
*/
@Override
public int octet5() {
return octet5;
}
/**
* Copy the current immutable object by setting a value for the {@link MACAddressType#octet0() octet0} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for octet0
* @return A modified copy of the {@code this} object
*/
public final MACAddress withOctet0(int value) {
if (this.octet0 == value) return this;
return validate(new MACAddress(value, this.octet1, this.octet2, this.octet3, this.octet4, this.octet5));
}
/**
* Copy the current immutable object by setting a value for the {@link MACAddressType#octet1() octet1} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for octet1
* @return A modified copy of the {@code this} object
*/
public final MACAddress withOctet1(int value) {
if (this.octet1 == value) return this;
return validate(new MACAddress(this.octet0, value, this.octet2, this.octet3, this.octet4, this.octet5));
}
/**
* Copy the current immutable object by setting a value for the {@link MACAddressType#octet2() octet2} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for octet2
* @return A modified copy of the {@code this} object
*/
public final MACAddress withOctet2(int value) {
if (this.octet2 == value) return this;
return validate(new MACAddress(this.octet0, this.octet1, value, this.octet3, this.octet4, this.octet5));
}
/**
* Copy the current immutable object by setting a value for the {@link MACAddressType#octet3() octet3} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for octet3
* @return A modified copy of the {@code this} object
*/
public final MACAddress withOctet3(int value) {
if (this.octet3 == value) return this;
return validate(new MACAddress(this.octet0, this.octet1, this.octet2, value, this.octet4, this.octet5));
}
/**
* Copy the current immutable object by setting a value for the {@link MACAddressType#octet4() octet4} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for octet4
* @return A modified copy of the {@code this} object
*/
public final MACAddress withOctet4(int value) {
if (this.octet4 == value) return this;
return validate(new MACAddress(this.octet0, this.octet1, this.octet2, this.octet3, value, this.octet5));
}
/**
* Copy the current immutable object by setting a value for the {@link MACAddressType#octet5() octet5} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for octet5
* @return A modified copy of the {@code this} object
*/
public final MACAddress withOctet5(int value) {
if (this.octet5 == value) return this;
return validate(new MACAddress(this.octet0, this.octet1, this.octet2, this.octet3, this.octet4, value));
}
/**
* This instance is equal to all instances of {@code MACAddress} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof MACAddress
&& equalTo(0, (MACAddress) another);
}
private boolean equalTo(int synthetic, MACAddress another) {
return octet0 == another.octet0
&& octet1 == another.octet1
&& octet2 == another.octet2
&& octet3 == another.octet3
&& octet4 == another.octet4
&& octet5 == another.octet5;
}
/**
* Computes a hash code from attributes: {@code octet0}, {@code octet1}, {@code octet2}, {@code octet3}, {@code octet4}, {@code octet5}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + octet0;
h += (h << 5) + octet1;
h += (h << 5) + octet2;
h += (h << 5) + octet3;
h += (h << 5) + octet4;
h += (h << 5) + octet5;
return h;
}
private static MACAddress validate(MACAddress instance) {
instance.checkPreconditions();
return instance;
}
/**
* Creates an immutable copy of a {@link MACAddressType} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable MACAddress instance
*/
public static MACAddress copyOf(MACAddressType instance) {
if (instance instanceof MACAddress) {
return (MACAddress) instance;
}
return MACAddress.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link MACAddress MACAddress}.
*
* MACAddress.builder()
* .setOctet0(int) // required {@link MACAddressType#octet0() octet0}
* .setOctet1(int) // required {@link MACAddressType#octet1() octet1}
* .setOctet2(int) // required {@link MACAddressType#octet2() octet2}
* .setOctet3(int) // required {@link MACAddressType#octet3() octet3}
* .setOctet4(int) // required {@link MACAddressType#octet4() octet4}
* .setOctet5(int) // required {@link MACAddressType#octet5() octet5}
* .build();
*
* @return A new MACAddress builder
*/
public static MACAddress.Builder builder() {
return new MACAddress.Builder();
}
/**
* Builds instances of type {@link MACAddress MACAddress}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_OCTET0 = 0x1L;
private static final long INIT_BIT_OCTET1 = 0x2L;
private static final long INIT_BIT_OCTET2 = 0x4L;
private static final long INIT_BIT_OCTET3 = 0x8L;
private static final long INIT_BIT_OCTET4 = 0x10L;
private static final long INIT_BIT_OCTET5 = 0x20L;
private long initBits = 0x3fL;
private int octet0;
private int octet1;
private int octet2;
private int octet3;
private int octet4;
private int octet5;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code MACAddressType} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(MACAddressType instance) {
Objects.requireNonNull(instance, "instance");
this.setOctet0(instance.octet0());
this.setOctet1(instance.octet1());
this.setOctet2(instance.octet2());
this.setOctet3(instance.octet3());
this.setOctet4(instance.octet4());
this.setOctet5(instance.octet5());
return this;
}
/**
* Initializes the value for the {@link MACAddressType#octet0() octet0} attribute.
* @param octet0 The value for octet0
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOctet0(int octet0) {
this.octet0 = octet0;
initBits &= ~INIT_BIT_OCTET0;
return this;
}
/**
* Initializes the value for the {@link MACAddressType#octet1() octet1} attribute.
* @param octet1 The value for octet1
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOctet1(int octet1) {
this.octet1 = octet1;
initBits &= ~INIT_BIT_OCTET1;
return this;
}
/**
* Initializes the value for the {@link MACAddressType#octet2() octet2} attribute.
* @param octet2 The value for octet2
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOctet2(int octet2) {
this.octet2 = octet2;
initBits &= ~INIT_BIT_OCTET2;
return this;
}
/**
* Initializes the value for the {@link MACAddressType#octet3() octet3} attribute.
* @param octet3 The value for octet3
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOctet3(int octet3) {
this.octet3 = octet3;
initBits &= ~INIT_BIT_OCTET3;
return this;
}
/**
* Initializes the value for the {@link MACAddressType#octet4() octet4} attribute.
* @param octet4 The value for octet4
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOctet4(int octet4) {
this.octet4 = octet4;
initBits &= ~INIT_BIT_OCTET4;
return this;
}
/**
* Initializes the value for the {@link MACAddressType#octet5() octet5} attribute.
* @param octet5 The value for octet5
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOctet5(int octet5) {
this.octet5 = octet5;
initBits &= ~INIT_BIT_OCTET5;
return this;
}
/**
* Builds a new {@link MACAddress MACAddress}.
* @return An immutable instance of MACAddress
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public MACAddress build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return MACAddress.validate(new MACAddress(octet0, octet1, octet2, octet3, octet4, octet5));
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_OCTET0) != 0) attributes.add("octet0");
if ((initBits & INIT_BIT_OCTET1) != 0) attributes.add("octet1");
if ((initBits & INIT_BIT_OCTET2) != 0) attributes.add("octet2");
if ((initBits & INIT_BIT_OCTET3) != 0) attributes.add("octet3");
if ((initBits & INIT_BIT_OCTET4) != 0) attributes.add("octet4");
if ((initBits & INIT_BIT_OCTET5) != 0) attributes.add("octet5");
return "Cannot build MACAddress, some of required attributes are not set " + attributes;
}
}
}