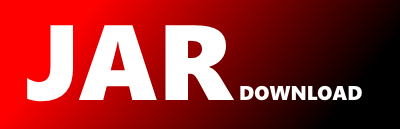
com.io7m.jstructural.annotated.SADocumentWithSections Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of io7m-jstructural-core Show documentation
Show all versions of io7m-jstructural-core Show documentation
Java implementation of the structural document language (Core types)
/*
* Copyright © 2014 http://io7m.com
*
* Permission to use, copy, modify, and/or distribute this software for any
* purpose with or without fee is hereby granted, provided that the above
* copyright notice and this permission notice appear in all copies.
*
* THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
* WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
* MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY
* SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
* WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
* ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
* IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
*/
package com.io7m.jstructural.annotated;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.io7m.jfunctional.Option;
import com.io7m.jfunctional.OptionType;
import com.io7m.jnull.NullCheck;
import com.io7m.jnull.Nullable;
import com.io7m.jstructural.core.SDocumentContents;
import com.io7m.jstructural.core.SDocumentStyle;
import com.io7m.jstructural.core.SNonEmptyList;
import com.io7m.junreachable.UnreachableCodeException;
/**
* A document with sections.
*/
public final class SADocumentWithSections extends SADocument
{
private final Map numbered_sections;
private final SNonEmptyList sections;
/**
* Construct a new document with sections.
*
* @param in_ids
* The set of mappings from IDs to elements
* @param in_title
* The title
* @param in_contents
* Whether or not the document has a table of contents
* @param in_style
* The style
* @param in_content
* The list of sections
* @param in_footnotes
* The list of footnotes
* @param in_formals
* The formal items
*/
public SADocumentWithSections(
final SAIDMap in_ids,
final SADocumentTitle in_title,
final OptionType in_contents,
final OptionType in_style,
final SNonEmptyList in_content,
final List in_footnotes,
final SAFormalItemsByKind in_formals)
{
super(in_ids, in_title, in_contents, in_style, in_footnotes, in_formals);
this.sections = NullCheck.notNull(in_content, "Content");
this.numbered_sections = new HashMap();
for (final SASection s : this.sections.getElements()) {
assert this.numbered_sections.containsKey(s.getNumber()) == false;
this.numbered_sections.put(s.getNumber(), s);
}
}
@Override public A documentAccept(
final SADocumentVisitor v)
throws Exception
{
return v.visitDocumentWithSections(this);
}
@Override public boolean equals(
final @Nullable Object obj)
{
if (this == obj) {
return true;
}
if (!super.equals(obj)) {
return false;
}
if (obj == null) {
return false;
}
if (this.getClass() != obj.getClass()) {
return false;
}
final SADocumentWithSections other = (SADocumentWithSections) obj;
return this.sections.equals(other.sections);
}
@Override public OptionType getSection(
final SASectionNumber n)
{
NullCheck.notNull(n, "Number");
if (this.numbered_sections.containsKey(n)) {
final SASection r = this.numbered_sections.get(n);
assert r != null;
return Option.some(r);
}
return Option.none();
}
/**
* @return The document sections
*/
public SNonEmptyList getSections()
{
return this.sections;
}
@Override public int hashCode()
{
final int prime = 31;
int result = super.hashCode();
result = (prime * result) + this.sections.hashCode();
return result;
}
@Override public SASegmentNumber segmentGetFirst()
{
return this.sections.getElements().get(0).getNumber();
}
@Override public OptionType segmentGetNext(
final SASegmentNumber n)
{
try {
final List section_list = this.sections.getElements();
return n
.segmentNumberAccept(new SASegmentNumberVisitor>() {
@Override public OptionType visitPartNumber(
final SAPartNumber pn)
throws Exception
{
throw new UnreachableCodeException();
}
@Override public OptionType visitSectionNumber(
final SASectionNumber pn)
throws Exception
{
return pn
.sectionNumberAccept(new SASectionNumberVisitor>() {
@Override public
OptionType
visitSectionNumberWithoutPart(
final SASectionNumberS p)
throws Exception
{
if (p.getSection() >= section_list.size()) {
return Option.none();
}
final SASectionNumberS next =
new SASectionNumberS(p.getSection() + 1);
return Option.some((SASegmentNumber) next);
}
@Override public
OptionType
visitSectionNumberWithPart(
final SASectionNumberPS p)
throws Exception
{
throw new UnreachableCodeException();
}
});
}
});
} catch (final Exception e) {
throw new UnreachableCodeException(e);
}
}
@Override public OptionType segmentGetPrevious(
final SASegmentNumber n)
{
try {
return n
.segmentNumberAccept(new SASegmentNumberVisitor>() {
@Override public OptionType visitPartNumber(
final SAPartNumber pn)
throws Exception
{
throw new UnreachableCodeException();
}
@Override public OptionType visitSectionNumber(
final SASectionNumber pn)
throws Exception
{
return pn
.sectionNumberAccept(new SASectionNumberVisitor>() {
@Override public
OptionType
visitSectionNumberWithoutPart(
final SASectionNumberS p)
throws Exception
{
if (p.getSection() == 1) {
return Option.none();
}
final SASectionNumberS prev =
new SASectionNumberS(p.getSection() - 1);
return Option.some((SASegmentNumber) prev);
}
@Override public
OptionType
visitSectionNumberWithPart(
final SASectionNumberPS p)
throws Exception
{
throw new UnreachableCodeException();
}
});
}
});
} catch (final Exception e) {
throw new UnreachableCodeException(e);
}
}
@Override public OptionType segmentGetUp(
final SASegmentNumber n)
{
return Option.none();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy