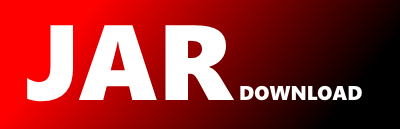
com.io7m.smfj.format.binary.v1.SMFBV1AttributeByteBuffered Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of io7m-smfj-format-binary Show documentation
Show all versions of io7m-smfj-format-binary Show documentation
Sequential mesh format (Binary format)
The newest version!
package com.io7m.smfj.format.binary.v1;
import com.io7m.jpra.runtime.java.JPRACursorByteReadableType;
import com.io7m.jpra.runtime.java.JPRAStringCursorByteBuffered;
import com.io7m.jpra.runtime.java.JPRAStringCursorReadableType;
import com.io7m.jpra.runtime.java.JPRAStringCursorType;
import com.io7m.jpra.runtime.java.JPRATypeModel;
import java.lang.Override;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import java.util.Objects;
/**
* A {@code ByteBuffer} based implementation of the {@code SMFBV1Attribute} record type. */
public final class SMFBV1AttributeByteBuffered implements SMFBV1AttributeType {
private static final int SIZE_OCTETS = 80;
private static final int FIELD_NAME_OFFSET_OCTETS = 0;
private static final int FIELD_COMPONENT_KIND_OFFSET_OCTETS = 68;
private static final int FIELD_COMPONENT_COUNT_OFFSET_OCTETS = 72;
private static final int FIELD_COMPONENT_SIZE_OFFSET_OCTETS = 76;
private final ByteBuffer buffer;
private final int base_offset;
private final JPRACursorByteReadableType pointer;
private final JPRAStringCursorType field_name;
private final JPRATypeModel.JPRAString meta_type_name;
private final JPRATypeModel.JPRAIntegerUnsigned meta_type_component_kind;
private final JPRATypeModel.JPRAIntegerUnsigned meta_type_component_count;
private final JPRATypeModel.JPRAIntegerUnsigned meta_type_component_size;
private SMFBV1AttributeByteBuffered(final ByteBuffer in_buffer, final JPRACursorByteReadableType in_pointer, final int in_base_offset) {
this.buffer = Objects.requireNonNull(in_buffer, "Buffer");
this.pointer = Objects.requireNonNull(in_pointer, "Pointer");
this.base_offset = in_base_offset;
this.field_name = JPRAStringCursorByteBuffered.newString(in_buffer, FIELD_NAME_OFFSET_OCTETS + in_base_offset, in_pointer, Charset.forName("UTF-8"), 64);
this.meta_type_name = JPRATypeModel.JPRAString.of(64, "UTF-8");
this.meta_type_component_kind = JPRATypeModel.JPRAIntegerUnsigned.of(32);
this.meta_type_component_count = JPRATypeModel.JPRAIntegerUnsigned.of(32);
this.meta_type_component_size = JPRATypeModel.JPRAIntegerUnsigned.of(32);
}
/**
* Construct a view of a type.
* @param in_buffer A byte buffer
* @param in_pointer A cursor
* @param in_base_offset The base offset from the cursor
* @return A view of a type
*/
public static SMFBV1AttributeType newValueWithOffset(final ByteBuffer in_buffer, final JPRACursorByteReadableType in_pointer, final int in_base_offset) {
return new SMFBV1AttributeByteBuffered(in_buffer, in_pointer, in_base_offset);
}
/**
* Construct a view of a type.
* @param in_buffer A byte buffer
* @param in_pointer A cursor
* @return A view of a type
*/
public static SMFBV1AttributeType newValue(final ByteBuffer in_buffer, final JPRACursorByteReadableType in_pointer) {
return new SMFBV1AttributeByteBuffered(in_buffer, in_pointer, 0);
}
@Override
public int sizeOctets() {
return SIZE_OCTETS;
}
/**
* @return The size of the type in octets
*/
public static int sizeInOctets() {
return SIZE_OCTETS;
}
private int getByteOffsetFor(final int field_offset) {
final int b = (int) this.pointer.getByteOffsetObservable().get();
return b + this.base_offset + field_offset;
}
@Override
public int metaNameOffsetFromType() {
return FIELD_NAME_OFFSET_OCTETS;
}
/**
* @return The offset in octets of field {@code name} from the start of the type
*/
public static int metaNameStaticOffsetFromType() {
return FIELD_NAME_OFFSET_OCTETS;
}
@Override
public int metaNameOffsetFromCursor() {
return FIELD_NAME_OFFSET_OCTETS + this.base_offset;
}
@Override
public JPRATypeModel.JPRAString metaNameType() {
return this.meta_type_name;
}
@Override
public JPRAStringCursorReadableType getNameReadable() {
return this.field_name;
}
@Override
public JPRAStringCursorType getNameWritable() {
return this.field_name;
}
@Override
public int metaComponentKindOffsetFromType() {
return FIELD_COMPONENT_KIND_OFFSET_OCTETS;
}
/**
* @return The offset in octets of field {@code component_kind} from the start of the type
*/
public static int metaComponentKindStaticOffsetFromType() {
return FIELD_COMPONENT_KIND_OFFSET_OCTETS;
}
@Override
public int metaComponentKindOffsetFromCursor() {
return FIELD_COMPONENT_KIND_OFFSET_OCTETS + this.base_offset;
}
@Override
public JPRATypeModel.JPRAIntegerUnsigned metaComponentKindType() {
return this.meta_type_component_kind;
}
@Override
public int getComponentKind() {
return this.buffer.getInt(this.getByteOffsetFor(FIELD_COMPONENT_KIND_OFFSET_OCTETS));
}
@Override
public void setComponentKind(final int x) {
this.buffer.putInt(this.getByteOffsetFor(FIELD_COMPONENT_KIND_OFFSET_OCTETS), x);
}
@Override
public int metaComponentCountOffsetFromType() {
return FIELD_COMPONENT_COUNT_OFFSET_OCTETS;
}
/**
* @return The offset in octets of field {@code component_count} from the start of the type
*/
public static int metaComponentCountStaticOffsetFromType() {
return FIELD_COMPONENT_COUNT_OFFSET_OCTETS;
}
@Override
public int metaComponentCountOffsetFromCursor() {
return FIELD_COMPONENT_COUNT_OFFSET_OCTETS + this.base_offset;
}
@Override
public JPRATypeModel.JPRAIntegerUnsigned metaComponentCountType() {
return this.meta_type_component_count;
}
@Override
public int getComponentCount() {
return this.buffer.getInt(this.getByteOffsetFor(FIELD_COMPONENT_COUNT_OFFSET_OCTETS));
}
@Override
public void setComponentCount(final int x) {
this.buffer.putInt(this.getByteOffsetFor(FIELD_COMPONENT_COUNT_OFFSET_OCTETS), x);
}
@Override
public int metaComponentSizeOffsetFromType() {
return FIELD_COMPONENT_SIZE_OFFSET_OCTETS;
}
/**
* @return The offset in octets of field {@code component_size} from the start of the type
*/
public static int metaComponentSizeStaticOffsetFromType() {
return FIELD_COMPONENT_SIZE_OFFSET_OCTETS;
}
@Override
public int metaComponentSizeOffsetFromCursor() {
return FIELD_COMPONENT_SIZE_OFFSET_OCTETS + this.base_offset;
}
@Override
public JPRATypeModel.JPRAIntegerUnsigned metaComponentSizeType() {
return this.meta_type_component_size;
}
@Override
public int getComponentSize() {
return this.buffer.getInt(this.getByteOffsetFor(FIELD_COMPONENT_SIZE_OFFSET_OCTETS));
}
@Override
public void setComponentSize(final int x) {
this.buffer.putInt(this.getByteOffsetFor(FIELD_COMPONENT_SIZE_OFFSET_OCTETS), x);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy