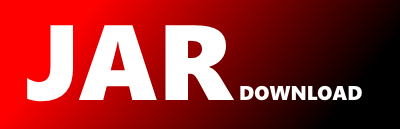
com.io7m.smfj.format.binary.v1.SMFBV1SchemaIDByteBuffered Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of io7m-smfj-format-binary Show documentation
Show all versions of io7m-smfj-format-binary Show documentation
Sequential mesh format (Binary format)
The newest version!
package com.io7m.smfj.format.binary.v1;
import com.io7m.jpra.runtime.java.JPRACursorByteReadableType;
import com.io7m.jpra.runtime.java.JPRATypeModel;
import java.lang.Override;
import java.nio.ByteBuffer;
import java.util.Objects;
/**
* A {@code ByteBuffer} based implementation of the {@code SMFBV1SchemaID} record type. */
public final class SMFBV1SchemaIDByteBuffered implements SMFBV1SchemaIDType {
private static final int SIZE_OCTETS = 16;
private static final int FIELD_VENDOR_ID_OFFSET_OCTETS = 0;
private static final int FIELD_SCHEMA_ID_OFFSET_OCTETS = 4;
private static final int FIELD_SCHEMA_VERSION_MAJOR_OFFSET_OCTETS = 8;
private static final int FIELD_SCHEMA_VERSION_MINOR_OFFSET_OCTETS = 12;
private final ByteBuffer buffer;
private final int base_offset;
private final JPRACursorByteReadableType pointer;
private final JPRATypeModel.JPRAIntegerUnsigned meta_type_vendor_id;
private final JPRATypeModel.JPRAIntegerUnsigned meta_type_schema_id;
private final JPRATypeModel.JPRAIntegerUnsigned meta_type_schema_version_major;
private final JPRATypeModel.JPRAIntegerUnsigned meta_type_schema_version_minor;
private SMFBV1SchemaIDByteBuffered(final ByteBuffer in_buffer, final JPRACursorByteReadableType in_pointer, final int in_base_offset) {
this.buffer = Objects.requireNonNull(in_buffer, "Buffer");
this.pointer = Objects.requireNonNull(in_pointer, "Pointer");
this.base_offset = in_base_offset;
this.meta_type_vendor_id = JPRATypeModel.JPRAIntegerUnsigned.of(32);
this.meta_type_schema_id = JPRATypeModel.JPRAIntegerUnsigned.of(32);
this.meta_type_schema_version_major = JPRATypeModel.JPRAIntegerUnsigned.of(32);
this.meta_type_schema_version_minor = JPRATypeModel.JPRAIntegerUnsigned.of(32);
}
/**
* Construct a view of a type.
* @param in_buffer A byte buffer
* @param in_pointer A cursor
* @param in_base_offset The base offset from the cursor
* @return A view of a type
*/
public static SMFBV1SchemaIDType newValueWithOffset(final ByteBuffer in_buffer, final JPRACursorByteReadableType in_pointer, final int in_base_offset) {
return new SMFBV1SchemaIDByteBuffered(in_buffer, in_pointer, in_base_offset);
}
/**
* Construct a view of a type.
* @param in_buffer A byte buffer
* @param in_pointer A cursor
* @return A view of a type
*/
public static SMFBV1SchemaIDType newValue(final ByteBuffer in_buffer, final JPRACursorByteReadableType in_pointer) {
return new SMFBV1SchemaIDByteBuffered(in_buffer, in_pointer, 0);
}
@Override
public int sizeOctets() {
return SIZE_OCTETS;
}
/**
* @return The size of the type in octets
*/
public static int sizeInOctets() {
return SIZE_OCTETS;
}
private int getByteOffsetFor(final int field_offset) {
final int b = (int) this.pointer.getByteOffsetObservable().get();
return b + this.base_offset + field_offset;
}
@Override
public int metaVendorIdOffsetFromType() {
return FIELD_VENDOR_ID_OFFSET_OCTETS;
}
/**
* @return The offset in octets of field {@code vendor_id} from the start of the type
*/
public static int metaVendorIdStaticOffsetFromType() {
return FIELD_VENDOR_ID_OFFSET_OCTETS;
}
@Override
public int metaVendorIdOffsetFromCursor() {
return FIELD_VENDOR_ID_OFFSET_OCTETS + this.base_offset;
}
@Override
public JPRATypeModel.JPRAIntegerUnsigned metaVendorIdType() {
return this.meta_type_vendor_id;
}
@Override
public int getVendorId() {
return this.buffer.getInt(this.getByteOffsetFor(FIELD_VENDOR_ID_OFFSET_OCTETS));
}
@Override
public void setVendorId(final int x) {
this.buffer.putInt(this.getByteOffsetFor(FIELD_VENDOR_ID_OFFSET_OCTETS), x);
}
@Override
public int metaSchemaIdOffsetFromType() {
return FIELD_SCHEMA_ID_OFFSET_OCTETS;
}
/**
* @return The offset in octets of field {@code schema_id} from the start of the type
*/
public static int metaSchemaIdStaticOffsetFromType() {
return FIELD_SCHEMA_ID_OFFSET_OCTETS;
}
@Override
public int metaSchemaIdOffsetFromCursor() {
return FIELD_SCHEMA_ID_OFFSET_OCTETS + this.base_offset;
}
@Override
public JPRATypeModel.JPRAIntegerUnsigned metaSchemaIdType() {
return this.meta_type_schema_id;
}
@Override
public int getSchemaId() {
return this.buffer.getInt(this.getByteOffsetFor(FIELD_SCHEMA_ID_OFFSET_OCTETS));
}
@Override
public void setSchemaId(final int x) {
this.buffer.putInt(this.getByteOffsetFor(FIELD_SCHEMA_ID_OFFSET_OCTETS), x);
}
@Override
public int metaSchemaVersionMajorOffsetFromType() {
return FIELD_SCHEMA_VERSION_MAJOR_OFFSET_OCTETS;
}
/**
* @return The offset in octets of field {@code schema_version_major} from the start of the type
*/
public static int metaSchemaVersionMajorStaticOffsetFromType() {
return FIELD_SCHEMA_VERSION_MAJOR_OFFSET_OCTETS;
}
@Override
public int metaSchemaVersionMajorOffsetFromCursor() {
return FIELD_SCHEMA_VERSION_MAJOR_OFFSET_OCTETS + this.base_offset;
}
@Override
public JPRATypeModel.JPRAIntegerUnsigned metaSchemaVersionMajorType() {
return this.meta_type_schema_version_major;
}
@Override
public int getSchemaVersionMajor() {
return this.buffer.getInt(this.getByteOffsetFor(FIELD_SCHEMA_VERSION_MAJOR_OFFSET_OCTETS));
}
@Override
public void setSchemaVersionMajor(final int x) {
this.buffer.putInt(this.getByteOffsetFor(FIELD_SCHEMA_VERSION_MAJOR_OFFSET_OCTETS), x);
}
@Override
public int metaSchemaVersionMinorOffsetFromType() {
return FIELD_SCHEMA_VERSION_MINOR_OFFSET_OCTETS;
}
/**
* @return The offset in octets of field {@code schema_version_minor} from the start of the type
*/
public static int metaSchemaVersionMinorStaticOffsetFromType() {
return FIELD_SCHEMA_VERSION_MINOR_OFFSET_OCTETS;
}
@Override
public int metaSchemaVersionMinorOffsetFromCursor() {
return FIELD_SCHEMA_VERSION_MINOR_OFFSET_OCTETS + this.base_offset;
}
@Override
public JPRATypeModel.JPRAIntegerUnsigned metaSchemaVersionMinorType() {
return this.meta_type_schema_version_minor;
}
@Override
public int getSchemaVersionMinor() {
return this.buffer.getInt(this.getByteOffsetFor(FIELD_SCHEMA_VERSION_MINOR_OFFSET_OCTETS));
}
@Override
public void setSchemaVersionMinor(final int x) {
this.buffer.putInt(this.getByteOffsetFor(FIELD_SCHEMA_VERSION_MINOR_OFFSET_OCTETS), x);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy