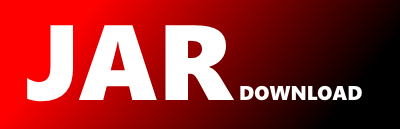
com.iodesystems.xml.tools.XmlLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xmltools Show documentation
Show all versions of xmltools Show documentation
Xmltools contains a flexible jaxb xml loader
package com.iodesystems.xml.tools;
import com.iodesystems.xml.tools.loaded.FileLoadErrored;
import com.iodesystems.xml.tools.loaded.FileLoaded;
import com.iodesystems.xml.tools.loaded.Loaded;
import com.iodesystems.xml.tools.loaded.NodeLoadErrored;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.XMLConstants;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.Unmarshaller.Listener;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchema;
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamReader;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class XmlLoader {
private final Map xmlIdentifierToClass = new HashMap<>();
private final JAXBContext jaxbContext;
private final XMLInputFactory xmlInputFactory;
private final Map schemasByLocation = new HashMap<>();
private final Map, String> classesBySchemaLocation;
public XmlLoader(Map, String> classesBySchemaLocation)
throws JAXBException, MalformedURLException, SAXException {
this.classesBySchemaLocation = classesBySchemaLocation;
jaxbContext =
JAXBContext.newInstance(classesBySchemaLocation.keySet().toArray(new Class>[0]));
xmlInputFactory = XMLInputFactory.newFactory();
// Preload all schemas
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
for (String schemaLocation : classesBySchemaLocation.values()) {
if (schemaLocation == null) {
continue;
}
initSchema(schemaFactory, schemaLocation);
}
// Register xmlIdentifiers
for (Class> cl : classesBySchemaLocation.keySet()) {
XmlRootElement xmlElementName = cl.getAnnotation(XmlRootElement.class);
if (xmlElementName == null) {
continue;
}
String name = xmlElementName.name();
String namespace = xmlElementName.namespace();
if ("##default".equals(namespace)) {
XmlSchema xmlElementNamespace = cl.getPackage().getAnnotation(XmlSchema.class);
if (xmlElementNamespace == null) {
continue;
}
namespace = xmlElementNamespace.namespace();
}
xmlIdentifierToClass.put(namespace + ":" + name, cl);
}
}
public Loaded load(Class cls, String source) throws FileLoadErrored, NodeLoadErrored {
if (source.startsWith("filesystem:")) {
try {
return load(cls, source, new FileInputStream(source.substring("filesystem:".length())));
} catch (FileNotFoundException e) {
throw new FileLoadErrored(source, cls, e, this);
}
} else if (source.startsWith("classpath:")) {
return load(
cls,
source,
getClass().getClassLoader().getResourceAsStream(source.substring("classpath:".length())));
} else {
try {
throw new IllegalArgumentException("Unknown scheme: " + source);
} catch (IllegalArgumentException e) {
throw new FileLoadErrored(source, cls, e, this);
}
}
}
public Loaded load(Class cls, String fileSource, InputStream inputStream)
throws FileLoadErrored, NodeLoadErrored {
try {
XMLStreamReader xsr = xmlInputFactory.createXMLStreamReader(inputStream);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
String validateSchema = classesBySchemaLocation.get(cls);
if (validateSchema != null) {
unmarshaller.setSchema(schemasByLocation.get(validateSchema));
}
Locator locator = new Locator(xsr);
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy