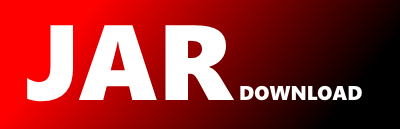
com.iofairy.pattern.mapping.ActionNoneMatcherMapping Maven / Gradle / Ivy
/*
* Copyright (C) 2021 iofairy,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.iofairy.pattern.mapping;
import com.iofairy.base.None;
import com.iofairy.lambda.*;
import com.iofairy.pattern.PatternIn;
import com.iofairy.pattern.matcher.ActionNoneRMatcher;
import com.iofairy.pattern.matcher.ActionNoneVMatcher;
/**
* ActionNone Matcher Mapping
* @since 0.0.1
*/
public class ActionNoneMatcherMapping extends PatternMatcherMapping {
protected final R1 super T, Boolean> preAction;
public ActionNoneMatcherMapping(None value, R1 super T, Boolean> preAction) {
super(value);
this.preAction = preAction;
}
public ActionNoneVMatcher when(T matchValue, V1 super T> action) {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.when(matchValue, action);
}
public ActionNoneVMatcher whenNext(T matchValue, V1 super T> action) {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.whenNext(matchValue, action);
}
public ActionNoneRMatcher when(T matchValue, R1 super T, ? extends R> action) {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.when(matchValue, action);
}
public ActionNoneRMatcher whenNext(T matchValue, R1 super T, ? extends R> action) {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.whenNext(matchValue, action);
}
public ActionNoneVMatcher when(PatternIn matchValues, V1 super T> action) {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.when(matchValues, action);
}
public ActionNoneVMatcher whenNext(PatternIn matchValues, V1 super T> action) {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.whenNext(matchValues, action);
}
public ActionNoneRMatcher when(PatternIn matchValues, R1 super T, ? extends R> action) {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.when(matchValues, action);
}
public ActionNoneRMatcher whenNext(PatternIn matchValues, R1 super T, ? extends R> action) {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.whenNext(matchValues, action);
}
public ActionNoneVMatcher when(boolean matchValue, V1 super T> action) {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.when(matchValue, action);
}
public ActionNoneVMatcher whenNext(boolean matchValue, V1 super T> action) {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.whenNext(matchValue, action);
}
public ActionNoneRMatcher when(boolean matchValue, R1 super T, ? extends R> action) {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.when(matchValue, action);
}
public ActionNoneRMatcher whenNext(boolean matchValue, R1 super T, ? extends R> action) {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.whenNext(matchValue, action);
}
/*
* ######################################################
* ******************************************************
* ##### MatcherMapping with throwing exception #####
* ******************************************************
* ######################################################
*/
public ActionNoneVMatcher with(T matchValue, VT1 super T, E> action) throws E {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.with(matchValue, action);
}
public ActionNoneVMatcher withNext(T matchValue, VT1 super T, E> action) throws E {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.withNext(matchValue, action);
}
public ActionNoneRMatcher with(T matchValue, RT1 super T, ? extends R, E> action) throws E {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.with(matchValue, action);
}
public ActionNoneRMatcher withNext(T matchValue, RT1 super T, ? extends R, E> action) throws E {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.withNext(matchValue, action);
}
public ActionNoneVMatcher with(PatternIn matchValues, VT1 super T, E> action) throws E {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.with(matchValues, action);
}
public ActionNoneVMatcher withNext(PatternIn matchValues, VT1 super T, E> action) throws E {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.withNext(matchValues, action);
}
public ActionNoneRMatcher with(PatternIn matchValues, RT1 super T, ? extends R, E> action) throws E {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.with(matchValues, action);
}
public ActionNoneRMatcher withNext(PatternIn matchValues, RT1 super T, ? extends R, E> action) throws E {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.withNext(matchValues, action);
}
public ActionNoneVMatcher with(boolean matchValue, VT1 super T, E> action) throws E {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.with(matchValue, action);
}
public ActionNoneVMatcher withNext(boolean matchValue, VT1 super T, E> action) throws E {
ActionNoneVMatcher actionNoneVMatcher = new ActionNoneVMatcher<>(value, preAction);
return actionNoneVMatcher.withNext(matchValue, action);
}
public ActionNoneRMatcher with(boolean matchValue, RT1 super T, ? extends R, E> action) throws E {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.with(matchValue, action);
}
public ActionNoneRMatcher withNext(boolean matchValue, RT1 super T, ? extends R, E> action) throws E {
ActionNoneRMatcher actionNoneRMatcher = new ActionNoneRMatcher<>(value, preAction);
return actionNoneRMatcher.withNext(matchValue, action);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy