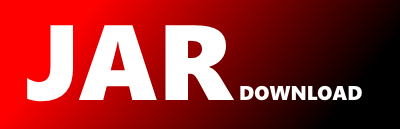
com.iofairy.pattern.matcher.Null4Matcher Maven / Gradle / Ivy
/*
* Copyright (C) 2021 iofairy,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.iofairy.pattern.matcher;
import com.iofairy.lambda.R1;
import com.iofairy.lambda.RT1;
import com.iofairy.pattern.type.*;
import com.iofairy.tuple.*;
import java.util.Objects;
/**
* Null4Matcher
*
* @since 0.2.0
*/
public class Null4Matcher implements NullMatcher {
private final R msg;
private final T1 value1;
private final T2 value2;
private final T3 value3;
private final boolean isMatch;
Null4Matcher(boolean isMatch, T1 value1, T2 value2, T3 value3, R msg) {
this.isMatch = isMatch;
this.value1 = value1;
this.value2 = value2;
this.value3 = value3;
this.msg = msg;
}
public Null5Matcher whenV(V matchValue, R1 super V, ? extends NV> computeValue, R1 super NV, Boolean> action, R1, ? extends R> msgAction) {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(msgAction);
if (!isMatch) {
R msg = msgAction.$(Tuple.of(value1, value2, value3));
if (matchValue == null) {
return new Null5Matcher<>(true, value1, value2, value3, null, msg);
}
NV newValue = computeValue.$(matchValue);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher whenV(V matchValue, R1 super V, ? extends NV> computeValue, R1, ? extends R> msgAction) {
return whenV(matchValue, computeValue, null, msgAction);
}
public Null5Matcher whenV(V matchValue, R1 super V, ? extends NV> computeValue, R1 super NV, Boolean> action, R msg) {
Objects.requireNonNull(computeValue);
if (!isMatch) {
if (matchValue == null) {
return new Null5Matcher<>(true, value1, value2, value3, null, msg);
}
NV newValue = computeValue.$(matchValue);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher whenV(V matchValue, R1 super V, ? extends NV> computeValue, R msg) {
return whenV(matchValue, computeValue, null, msg);
}
public Null5Matcher whenW(PatternNull1 patternNull, R1 super T1, ? extends NV> computeValue, R1 super NV, Boolean> action, R1, ? extends R> msgAction) {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
Objects.requireNonNull(msgAction);
if (!isMatch) {
R msg = msgAction.$(Tuple.of(value1, value2, value3));
NV newValue = computeValue.$(value1);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher whenW(PatternNull1 patternNull, R1 super T1, ? extends NV> computeValue, R1, ? extends R> msgAction) {
return whenW(patternNull, computeValue, null, msgAction);
}
public Null5Matcher whenW(PatternNull1 patternNull, R1 super T1, ? extends NV> computeValue, R1 super NV, Boolean> action, R msg) {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
if (!isMatch) {
NV newValue = computeValue.$(value1);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher whenW(PatternNull1 patternNull, R1 super T1, ? extends NV> computeValue, R msg) {
return whenW(patternNull, computeValue, null, msg);
}
public Null5Matcher whenW(PatternNull2 patternNull, R1 super T2, ? extends NV> computeValue, R1 super NV, Boolean> action, R1, ? extends R> msgAction) {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
Objects.requireNonNull(msgAction);
if (!isMatch) {
R msg = msgAction.$(Tuple.of(value1, value2, value3));
NV newValue = computeValue.$(value2);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher whenW(PatternNull2 patternNull, R1 super T2, ? extends NV> computeValue, R1, ? extends R> msgAction) {
return whenW(patternNull, computeValue, null, msgAction);
}
public Null5Matcher whenW(PatternNull2 patternNull, R1 super T2, ? extends NV> computeValue, R1 super NV, Boolean> action, R msg) {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
if (!isMatch) {
NV newValue = computeValue.$(value2);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher whenW(PatternNull2 patternNull, R1 super T2, ? extends NV> computeValue, R msg) {
return whenW(patternNull, computeValue, null, msg);
}
public Null5Matcher whenW(PatternNull3 patternNull, R1 super T3, ? extends NV> computeValue, R1 super NV, Boolean> action, R1, ? extends R> msgAction) {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
Objects.requireNonNull(msgAction);
if (!isMatch) {
R msg = msgAction.$(Tuple.of(value1, value2, value3));
NV newValue = computeValue.$(value3);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher whenW(PatternNull3 patternNull, R1 super T3, ? extends NV> computeValue, R1, ? extends R> msgAction) {
return whenW(patternNull, computeValue, null, msgAction);
}
public Null5Matcher whenW(PatternNull3 patternNull, R1 super T3, ? extends NV> computeValue, R1 super NV, Boolean> action, R msg) {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
if (!isMatch) {
NV newValue = computeValue.$(value3);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher whenW(PatternNull3 patternNull, R1 super T3, ? extends NV> computeValue, R msg) {
return whenW(patternNull, computeValue, null, msg);
}
/*
* ########################################################################
* ************************************************************************
* ################ NullMatcher with throwing exception ###############
* ************************************************************************
* ########################################################################
*/
public Null5Matcher withV(V matchValue, RT1 super V, ? extends NV, E> computeValue, RT1 super NV, Boolean, E> action, RT1, ? extends R, E> msgAction) throws E {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(msgAction);
if (!isMatch) {
R msg = msgAction.$(Tuple.of(value1, value2, value3));
if (matchValue == null) {
return new Null5Matcher<>(true, value1, value2, value3, null, msg);
}
NV newValue = computeValue.$(matchValue);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher withV(V matchValue, RT1 super V, ? extends NV, E> computeValue, RT1, ? extends R, E> msgAction) throws E {
return withV(matchValue, computeValue, null, msgAction);
}
public Null5Matcher withV(V matchValue, RT1 super V, ? extends NV, E> computeValue, RT1 super NV, Boolean, E> action, R msg) throws E {
Objects.requireNonNull(computeValue);
if (!isMatch) {
if (matchValue == null) {
return new Null5Matcher<>(true, value1, value2, value3, null, msg);
}
NV newValue = computeValue.$(matchValue);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher withV(V matchValue, RT1 super V, ? extends NV, E> computeValue, R msg) throws E {
return withV(matchValue, computeValue, null, msg);
}
public Null5Matcher withW(PatternNull1 patternNull, RT1 super T1, ? extends NV, E> computeValue, RT1 super NV, Boolean, E> action, RT1, ? extends R, E> msgAction) throws E {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
Objects.requireNonNull(msgAction);
if (!isMatch) {
R msg = msgAction.$(Tuple.of(value1, value2, value3));
NV newValue = computeValue.$(value1);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher withW(PatternNull1 patternNull, RT1 super T1, ? extends NV, E> computeValue, RT1, ? extends R, E> msgAction) throws E {
return withW(patternNull, computeValue, null, msgAction);
}
public Null5Matcher withW(PatternNull1 patternNull, RT1 super T1, ? extends NV, E> computeValue, RT1 super NV, Boolean, E> action, R msg) throws E {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
if (!isMatch) {
NV newValue = computeValue.$(value1);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher withW(PatternNull1 patternNull, RT1 super T1, ? extends NV, E> computeValue, R msg) throws E {
return withW(patternNull, computeValue, null, msg);
}
public Null5Matcher withW(PatternNull2 patternNull, RT1 super T2, ? extends NV, E> computeValue, RT1 super NV, Boolean, E> action, RT1, ? extends R, E> msgAction) throws E {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
Objects.requireNonNull(msgAction);
if (!isMatch) {
R msg = msgAction.$(Tuple.of(value1, value2, value3));
NV newValue = computeValue.$(value2);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher withW(PatternNull2 patternNull, RT1 super T2, ? extends NV, E> computeValue, RT1, ? extends R, E> msgAction) throws E {
return withW(patternNull, computeValue, null, msgAction);
}
public Null5Matcher withW(PatternNull2 patternNull, RT1 super T2, ? extends NV, E> computeValue, RT1 super NV, Boolean, E> action, R msg) throws E {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
if (!isMatch) {
NV newValue = computeValue.$(value2);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher withW(PatternNull2 patternNull, RT1 super T2, ? extends NV, E> computeValue, R msg) throws E {
return withW(patternNull, computeValue, null, msg);
}
public Null5Matcher withW(PatternNull3 patternNull, RT1 super T3, ? extends NV, E> computeValue, RT1 super NV, Boolean, E> action, RT1, ? extends R, E> msgAction) throws E {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
Objects.requireNonNull(msgAction);
if (!isMatch) {
R msg = msgAction.$(Tuple.of(value1, value2, value3));
NV newValue = computeValue.$(value3);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher withW(PatternNull3 patternNull, RT1 super T3, ? extends NV, E> computeValue, RT1, ? extends R, E> msgAction) throws E {
return withW(patternNull, computeValue, null, msgAction);
}
public Null5Matcher withW(PatternNull3 patternNull, RT1 super T3, ? extends NV, E> computeValue, RT1 super NV, Boolean, E> action, R msg) throws E {
Objects.requireNonNull(computeValue);
Objects.requireNonNull(patternNull);
if (!isMatch) {
NV newValue = computeValue.$(value3);
boolean doBreak = newValue == null || (action != null && action.$(newValue));
R localMsg = doBreak ? msg : null;
return new Null5Matcher<>(doBreak, value1, value2, value3, newValue, localMsg);
}
return new Null5Matcher<>(true, value1, value2, value3, null, this.msg);
}
public Null5Matcher withW(PatternNull3 patternNull, RT1 super T3, ? extends NV, E> computeValue, R msg) throws E {
return withW(patternNull, computeValue, null, msg);
}
public Tuple4 orElse(R msg) {
R finalMsg = !isMatch ? msg : this.msg;
return Tuple.of(value1, value2, value3, finalMsg);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy