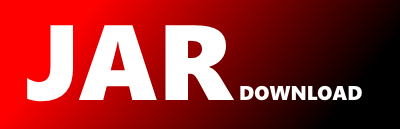
com.iofairy.tuple.EasyTuple Maven / Gradle / Ivy
/*
* Copyright (C) 2021 iofairy,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.iofairy.tuple;
import java.util.*;
/**
* EasyTuple Interface
*
* @since 0.0.5
*/
public interface EasyTuple extends Tuple {
long serialVersionUID = 10065917085L;
/**
* Transform this EasyTuple to List.
* 将 EasyTuple 转成 List
* @param type of elements. 元素的类型
* @return a list
*/
default List toList(){
List ts = new ArrayList<>();
if (arity() > 0) {
for (int i = 0; i < arity(); i++) {
ts.add(element(i));
}
}
return Collections.unmodifiableList(ts);
}
/**
* Create empty tuple
* 创建一个空元组
*
* @return the instance of Tuple0. 返回Tuple0的实例
*/
static EasyTuple0 empty() {
return EasyTuple0.instance();
}
/**
* Create empty EasyTuple
* 创建一个空元组
*
* @return the instance of EasyTuple0. 返回EasyTuple0的实例
*/
static EasyTuple0 of() {
return EasyTuple0.instance();
}
/**
* Create a EasyTuple of 1 element
* 创建1个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param type of element. 元素的类型
* @return the instance of EasyTuple1. 返回EasyTuple1的实例
*/
static EasyTuple1 of(T _1) {
return new EasyTuple1<>(_1);
}
/**
* Create a EasyTuple of 2 elements
* 创建2个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param _2 the 2nd element. 第2个元素
* @param type of elements. 元素的类型
* @return the instance of EasyTuple2. 返回EasyTuple2的实例
*/
static EasyTuple2 of(T _1, T _2) {
return new EasyTuple2<>(_1, _2);
}
/**
* Create a EasyTuple of 3 elements
* 创建3个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param _2 the 2nd element. 第2个元素
* @param _3 the 3rd element. 第3个元素
* @param type of elements. 元素的类型
* @return the instance of EasyTuple3. 返回EasyTuple3的实例
*/
static EasyTuple3 of(T _1, T _2, T _3) {
return new EasyTuple3<>(_1, _2, _3);
}
/**
* Create a EasyTuple of 4 elements
* 创建4个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param _2 the 2nd element. 第2个元素
* @param _3 the 3rd element. 第3个元素
* @param _4 the 4th element. 第4个元素
* @param type of elements. 元素的类型
* @return the instance of EasyTuple4. 返回EasyTuple4的实例
*/
static EasyTuple4 of(T _1, T _2, T _3, T _4) {
return new EasyTuple4<>(_1, _2, _3, _4);
}
/**
* Create a EasyTuple of 5 elements
* 创建5个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param _2 the 2nd element. 第2个元素
* @param _3 the 3rd element. 第3个元素
* @param _4 the 4th element. 第4个元素
* @param _5 the 5th element. 第5个元素
* @param type of elements. 元素的类型
* @return the instance of EasyTuple5. 返回EasyTuple5的实例
*/
static EasyTuple5 of(T _1, T _2, T _3, T _4, T _5) {
return new EasyTuple5<>(_1, _2, _3, _4, _5);
}
/**
* Create a EasyTuple of 6 elements
* 创建6个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param _2 the 2nd element. 第2个元素
* @param _3 the 3rd element. 第3个元素
* @param _4 the 4th element. 第4个元素
* @param _5 the 5th element. 第5个元素
* @param _6 the 6th element. 第6个元素
* @param type of elements. 元素的类型
* @return the instance of EasyTuple6. 返回EasyTuple6的实例
*/
static EasyTuple6 of(T _1, T _2, T _3, T _4, T _5, T _6) {
return new EasyTuple6<>(_1, _2, _3, _4, _5, _6);
}
/**
* Create a EasyTuple of 7 elements
* 创建7个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param _2 the 2nd element. 第2个元素
* @param _3 the 3rd element. 第3个元素
* @param _4 the 4th element. 第4个元素
* @param _5 the 5th element. 第5个元素
* @param _6 the 6th element. 第6个元素
* @param _7 the 7th element. 第7个元素
* @param type of elements. 元素的类型
* @return the instance of EasyTuple7. 返回EasyTuple7的实例
*/
static EasyTuple7 of(T _1, T _2, T _3, T _4, T _5, T _6, T _7) {
return new EasyTuple7<>(_1, _2, _3, _4, _5, _6, _7);
}
/**
* Create a EasyTuple of 8 elements
* 创建8个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param _2 the 2nd element. 第2个元素
* @param _3 the 3rd element. 第3个元素
* @param _4 the 4th element. 第4个元素
* @param _5 the 5th element. 第5个元素
* @param _6 the 6th element. 第6个元素
* @param _7 the 7th element. 第7个元素
* @param _8 the 8th element. 第8个元素
* @param type of elements. 元素的类型
* @return the instance of EasyTuple8. 返回EasyTuple8的实例
*/
static EasyTuple8 of(T _1, T _2, T _3, T _4, T _5, T _6, T _7, T _8) {
return new EasyTuple8<>(_1, _2, _3, _4, _5, _6, _7, _8);
}
/**
* Create a EasyTuple of 9 elements
* 创建9个元素的元组
*
* @param _1 the 1st element. 第1个元素
* @param _2 the 2nd element. 第2个元素
* @param _3 the 3rd element. 第3个元素
* @param _4 the 4th element. 第4个元素
* @param _5 the 5th element. 第5个元素
* @param _6 the 6th element. 第6个元素
* @param _7 the 7th element. 第7个元素
* @param _8 the 8th element. 第8个元素
* @param _9 the 9th element. 第9个元素
* @param type of elements. 元素的类型
* @return the instance of EasyTuple9. 返回EasyTuple9的实例
*/
static EasyTuple9 of(T _1, T _2, T _3, T _4, T _5, T _6, T _7, T _8, T _9) {
return new EasyTuple9<>(_1, _2, _3, _4, _5, _6, _7, _8, _9);
}
/**
* clone a tuple by shallow copy.
* 通过浅拷贝的方式克隆一个tuple
* @param tuple origin tuple
* @return new tuple
*/
static EasyTuple clone(EasyTuple tuple) {
return tuple == null ? tuple : (EasyTuple) tuple.copy();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy