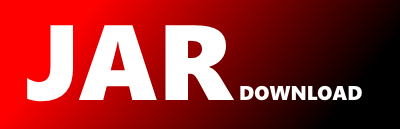
com.github.gg_a.text.StringExtractor Maven / Gradle / Ivy
The newest version!
package com.github.gg_a.text;
import com.github.gg_a.tuple.Tuple;
import com.github.gg_a.tuple.Tuple2;
import com.github.gg_a.tuple.Tuple3;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class StringExtractor {
/**
* ${} will parse to $ (String literal).
* 遇到 ${} 则解析成字符 $
*/
private final static String $ = "${}";
private final static String DEFAULT_VALUE_DELIMITER = ": ";
/**
* Regex for extracting expression from ${}, but { or } can't be included in ${}
*/
private final static Pattern PATTERN = Pattern.compile("\\$\\{((?![{}]).)*}", Pattern.MULTILINE);
/**
* 根据 ${} 分割字符串
*
* @param source 待插值的字符串
* @return 列表。Tuple3:_1 字符串类型; _2 字符串; _3 原始字符串
*/
public static List> split(String source) {
List> stringTs = new ArrayList<>();
final Matcher matcher = PATTERN.matcher(source);
int startIndex = 0;
while (matcher.find()) {
String matchStr = matcher.group();
int start = matcher.start(); // 起始字符位置
int end = matcher.end(); // 结束字符+1 的位置
if ($.equals(matchStr)) {
String value = source.substring(startIndex, start + 1);
stringTs.add(Tuple.of(StringType.STRING, value, value));
} else {
if (start != startIndex) { // 不相等说明${}前面有一段常量还未添加进列表
String value = source.substring(startIndex, start);
stringTs.add(Tuple.of(StringType.STRING, value, value));
}
String strInBrace = matchStr.substring(2, matchStr.length() - 1);
int index = strInBrace.indexOf(DEFAULT_VALUE_DELIMITER);
if (index > -1) {
String defaultValue = "";
if (index + DEFAULT_VALUE_DELIMITER.length() < strInBrace.length()) {
defaultValue = strInBrace.substring(index + DEFAULT_VALUE_DELIMITER.length());
stringTs.add(Tuple.of(StringType.VALUE, strInBrace.substring(0, index), defaultValue));
}
}else {
stringTs.add(Tuple.of(StringType.VALUE, strInBrace, matchStr));
}
}
startIndex = end;
}
if (startIndex < source.length()) {
String value = source.substring(startIndex);
stringTs.add(Tuple.of(StringType.STRING, value, value));
}
return stringTs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy