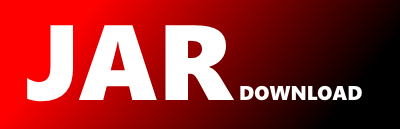
com.iohao.profile.Profile Maven / Gradle / Ivy
package com.iohao.profile;
import com.iohao.profile.able.Profileable;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* profile
* create time : 2019-02-02 11:14
*
* @author [email protected]
*/
@ToString
public class Profile {
private static final String TRUE = "true";
private static final String FALSE = "false";
String key;
@Getter
Map map = new HashMap<>();
@Getter
@Setter
Profileable profileable = new Profileable() {
@Override
public String get(String key, String defVal) {
return defVal;
}
@Override
public boolean getBool(String key, boolean defVal) {
return defVal;
}
@Override
public int getInt(String key, int defVal) {
return defVal;
}
};
Profile() {
}
public void put(String key, Boolean val) {
map.put(key, val);
}
public void put(String key, Integer val) {
map.put(key, val);
}
public void put(String key, String val) {
map.put(key, val);
}
/**
* 添加值
*
* boolean 类型 : 添加到 bool put
* int 类型 : 添加到 int put
* str 类型 : 添加到 str put
*
*
* @param key key
* @param value 值
*/
public void putValue(String key, Object value) {
if (Objects.isNull(value)) {
return;
}
String strValue = value.toString().trim();
if (strValue.length() == 0) {
return;
}
if (isNumeric(strValue)) {
put(key, Integer.valueOf(strValue));
} else if (Objects.equals(strValue, TRUE) || Objects.equals(strValue, FALSE)) {
put(key, Boolean.valueOf(strValue));
} else {
put(key, strValue);
}
}
/**
* 移除属性
*
* @param key key
* @return 移除的值
*/
public Object remove(String key) {
return map.remove(key);
}
/**
* 获取 string 值
*
* @param key key
* @return 不存在返回 "" 空字符串
*/
public String get(String key) {
return get(key, "");
}
/**
* 获取 string 值
*
* @param key key
* @param defVal 默认值
* @return key 不存在, 返回默认值
*/
public String get(String key, String defVal) {
Object value = map.get(key);
return Objects.isNull(value) ? profileable.get(key, defVal) : String.valueOf(value);
}
/**
* 获取 bool 值
*
* @param key key
* @return key 不存在, 返回false
*/
public boolean getBool(String key) {
return getBool(key, false);
}
/**
* 获取 bool 值
*
* @param key key
* @param defVal 默认值
* @return key 不存在, 返回默认值
*/
public boolean getBool(String key, boolean defVal) {
Object value = map.get(key);
if (Objects.isNull(value)) {
return profileable.getBool(key, defVal);
}
return value instanceof Boolean ? (boolean) value : defVal;
}
/**
* 获取 int 值
*
* @param key key
* @return key 不存在, 返回0
*/
public int getInt(String key) {
return getInt(key, 0);
}
/**
* 获取 int 值
*
* @param key key
* @param defVal 默认值
* @return key 不存在, 返回默认值
*/
public int getInt(String key, int defVal) {
Object value = map.get(key);
if (Objects.isNull(value)) {
return profileable.getInt(key, defVal);
}
return value instanceof Integer ? (int) value : defVal;
}
private boolean isNumeric(String str) {
char[] chars = str.toCharArray();
for (int i = 0; i < chars.length; i++) {
if (chars[i] < 48 || chars[i] > 57) {
return false;
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy